Cells Si SOI 220nm#
add_fiber_array_sc#
- cspdk.si220.cells.add_fiber_array_sc(component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_sc', grating_coupler='grating_coupler_elliptical_sc', gc_port_name: str = 'o1', component_name: str | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', gc_rotation: float = -90, **kwargs) Component [source]#
Returns component with south routes and grating_couplers.
You can also use pads or other terminations instead of grating couplers.
- Parameters:
component – component spec to connect to grating couplers.
grating_coupler – spec for route terminations.
gc_port_name – grating coupler input port name.
component_name – optional for the label.
cross_section – cross_section function.
gc_rotation – fiber coupler rotation in degrees. Defaults to -90.
kwargs – additional arguments.
- Keyword Arguments:
bend – bend spec.
straight – straight spec.
fanout_length – if None, automatic calculation of fanout length.
max_y0_optical – in um.
with_loopback – True, adds loopback structures.
with_loopback_inside – True, adds loopback structures inside the component.
straight_separation – from edge to edge.
list_port_labels – None, adds TM labels to port indices in this list.
connected_port_list_ids – names of ports only for type 0 optical routing.
nb_optical_ports_lines – number of grating coupler lines.
force_manhattan – False
excluded_ports – list of port names to exclude when adding gratings.
grating_indices – list of grating coupler indices.
routing_straight – function to route.
routing_method – route_single.
optical_routing_type – None: auto, 0: no extension, 1: standard, 2: check.
input_port_indexes – to connect.
pitch – in um.
radius – optional radius of the bend. Defaults to the cross_section.
radius_loopback – optional radius of the loopback bend. Defaults to the cross_section.
route_backwards – route from component to grating coupler or vice-versa.
import gdsfactory as gf c = gf.components.crossing() cc = gf.routing.add_fiber_array( component=c, optical_routing_type=2, grating_coupler=gf.components.grating_coupler_elliptical_te, with_loopback=False ) cc.plot()
import cspdk
c = cspdk.si220.cells.add_fiber_array_sc(component='straight_sc', grating_coupler='grating_coupler_elliptical_sc', gc_port_name='o1', cross_section='xs_sc', gc_rotation=-90).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
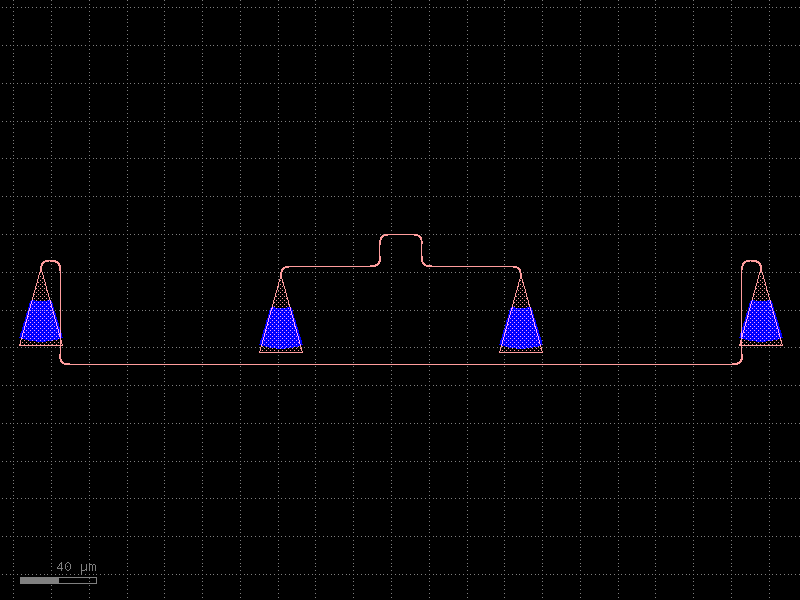
add_fiber_array_so#
- cspdk.si220.cells.add_fiber_array_so(*, component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_so', grating_coupler='grating_coupler_elliptical_so', gc_port_name: str = 'o1', component_name: str | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', gc_rotation: float = -90, **kwargs) Component #
Returns component with south routes and grating_couplers.
You can also use pads or other terminations instead of grating couplers.
- Parameters:
component – component spec to connect to grating couplers.
grating_coupler – spec for route terminations.
gc_port_name – grating coupler input port name.
component_name – optional for the label.
cross_section – cross_section function.
gc_rotation – fiber coupler rotation in degrees. Defaults to -90.
kwargs – additional arguments.
- Keyword Arguments:
bend – bend spec.
straight – straight spec.
fanout_length – if None, automatic calculation of fanout length.
max_y0_optical – in um.
with_loopback – True, adds loopback structures.
with_loopback_inside – True, adds loopback structures inside the component.
straight_separation – from edge to edge.
list_port_labels – None, adds TM labels to port indices in this list.
connected_port_list_ids – names of ports only for type 0 optical routing.
nb_optical_ports_lines – number of grating coupler lines.
force_manhattan – False
excluded_ports – list of port names to exclude when adding gratings.
grating_indices – list of grating coupler indices.
routing_straight – function to route.
routing_method – route_single.
optical_routing_type – None: auto, 0: no extension, 1: standard, 2: check.
input_port_indexes – to connect.
pitch – in um.
radius – optional radius of the bend. Defaults to the cross_section.
radius_loopback – optional radius of the loopback bend. Defaults to the cross_section.
route_backwards – route from component to grating coupler or vice-versa.
import gdsfactory as gf c = gf.components.crossing() cc = gf.routing.add_fiber_array( component=c, optical_routing_type=2, grating_coupler=gf.components.grating_coupler_elliptical_te, with_loopback=False ) cc.plot()
import cspdk
c = cspdk.si220.cells.add_fiber_array_so(component='straight_so', grating_coupler='grating_coupler_elliptical_so', gc_port_name='o1', cross_section='xs_so', gc_rotation=-90).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
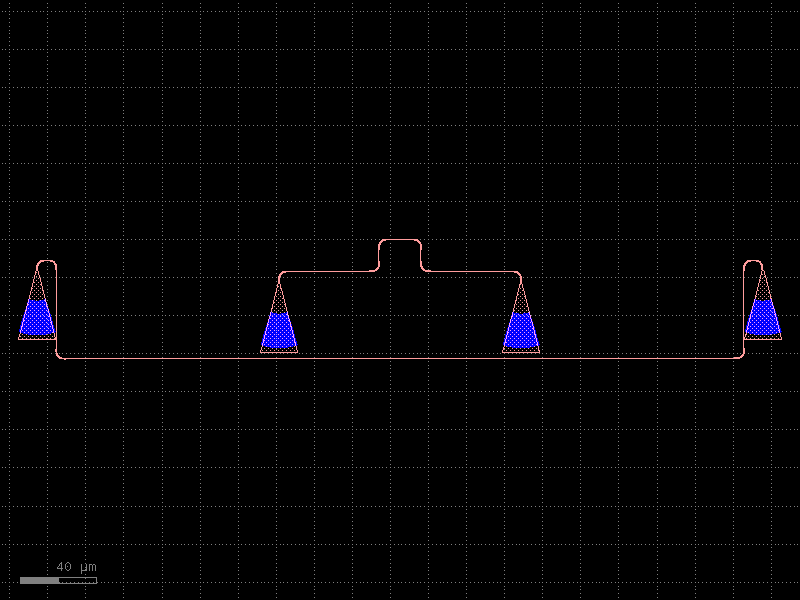
add_fiber_single_sc#
- cspdk.si220.cells.add_fiber_single_sc(component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_sc', grating_coupler='grating_coupler_elliptical_sc', gc_port_name: str = 'o1', component_name: str | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', taper: str | Callable[[...], Component] | dict[str, Any] | KCell | None = None, input_port_names: list[str] | tuple[str, ...] | None = None, fiber_spacing: float = 70, with_loopback: bool = True, loopback_spacing: float = 100.0, **kwargs) Component [source]#
Returns component with south routes and grating_couplers.
You can also use pads or other terminations instead of grating couplers.
- Parameters:
component – component spec to connect to grating couplers.
grating_coupler – spec for route terminations.
gc_port_name – grating coupler input port name.
component_name – optional for the label.
cross_section – cross_section function.
taper – taper spec.
input_port_names – list of input port names to connect to grating couplers.
fiber_spacing – spacing between fibers.
with_loopback – adds loopback structures.
loopback_spacing – spacing between loopback and test structure.
kwargs – additional arguments.
- Keyword Arguments:
bend – bend spec.
straight – straight spec.
fanout_length – if None, automatic calculation of fanout length.
max_y0_optical – in um.
with_loopback – True, adds loopback structures.
straight_separation – from edge to edge.
list_port_labels – None, adds TM labels to port indices in this list.
connected_port_list_ids – names of ports only for type 0 optical routing.
nb_optical_ports_lines – number of grating coupler lines.
force_manhattan – False
excluded_ports – list of port names to exclude when adding gratings.
grating_indices – list of grating coupler indices.
routing_straight – function to route.
routing_method – route_single.
optical_routing_type – None: auto, 0: no extension, 1: standard, 2: check.
gc_rotation – fiber coupler rotation in degrees. Defaults to -90.
input_port_indexes – to connect.
import gdsfactory as gf c = gf.components.crossing() cc = gf.routing.add_fiber_array( component=c, optical_routing_type=2, grating_coupler=gf.components.grating_coupler_elliptical_te, with_loopback=False ) cc.plot()
import cspdk
c = cspdk.si220.cells.add_fiber_single_sc(component='straight_sc', grating_coupler='grating_coupler_elliptical_sc', gc_port_name='o1', cross_section='xs_sc', fiber_spacing=70, with_loopback=True, loopback_spacing=100.0).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
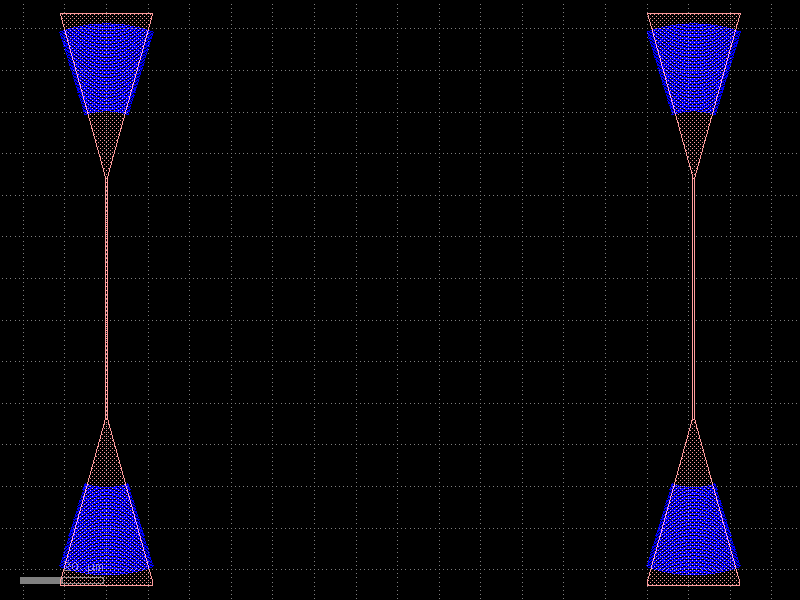
add_fiber_single_so#
- cspdk.si220.cells.add_fiber_single_so(*, component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_so', grating_coupler='grating_coupler_elliptical_so', gc_port_name: str = 'o1', component_name: str | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', taper: str | Callable[[...], Component] | dict[str, Any] | KCell | None = None, input_port_names: list[str] | tuple[str, ...] | None = None, fiber_spacing: float = 70, with_loopback: bool = True, loopback_spacing: float = 100.0, **kwargs) Component #
Returns component with south routes and grating_couplers.
You can also use pads or other terminations instead of grating couplers.
- Parameters:
component – component spec to connect to grating couplers.
grating_coupler – spec for route terminations.
gc_port_name – grating coupler input port name.
component_name – optional for the label.
cross_section – cross_section function.
taper – taper spec.
input_port_names – list of input port names to connect to grating couplers.
fiber_spacing – spacing between fibers.
with_loopback – adds loopback structures.
loopback_spacing – spacing between loopback and test structure.
kwargs – additional arguments.
- Keyword Arguments:
bend – bend spec.
straight – straight spec.
fanout_length – if None, automatic calculation of fanout length.
max_y0_optical – in um.
with_loopback – True, adds loopback structures.
straight_separation – from edge to edge.
list_port_labels – None, adds TM labels to port indices in this list.
connected_port_list_ids – names of ports only for type 0 optical routing.
nb_optical_ports_lines – number of grating coupler lines.
force_manhattan – False
excluded_ports – list of port names to exclude when adding gratings.
grating_indices – list of grating coupler indices.
routing_straight – function to route.
routing_method – route_single.
optical_routing_type – None: auto, 0: no extension, 1: standard, 2: check.
gc_rotation – fiber coupler rotation in degrees. Defaults to -90.
input_port_indexes – to connect.
import gdsfactory as gf c = gf.components.crossing() cc = gf.routing.add_fiber_array( component=c, optical_routing_type=2, grating_coupler=gf.components.grating_coupler_elliptical_te, with_loopback=False ) cc.plot()
import cspdk
c = cspdk.si220.cells.add_fiber_single_so(component='straight_so', grating_coupler='grating_coupler_elliptical_so', gc_port_name='o1', cross_section='xs_so', fiber_spacing=70, with_loopback=True, loopback_spacing=100.0).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
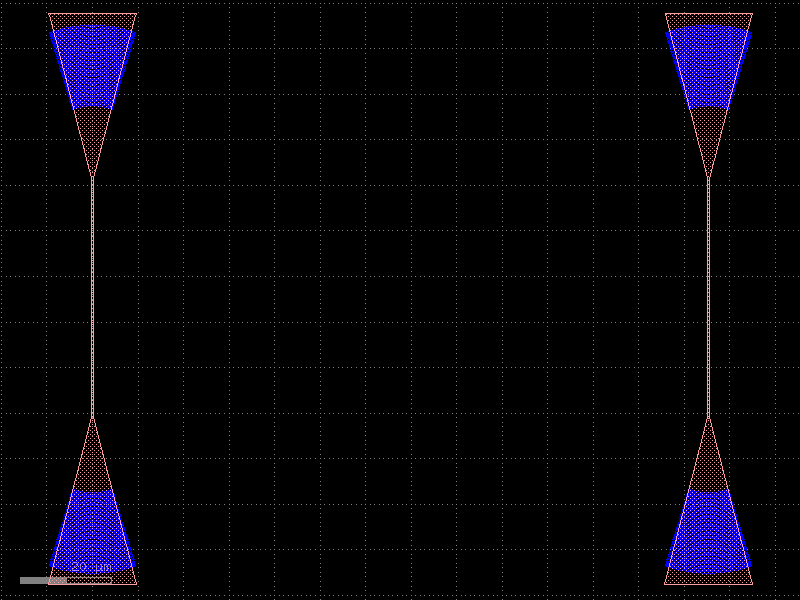
add_pads_top#
- cspdk.si220.cells.add_pads_top(component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_heater_metal_sc', port_names: Sequence[str] | None = None, component_name: str | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'metal_routing', pad_port_name: str = 'e1', pad: str | Callable[[...], Component] | dict[str, Any] | KCell = 'pad', bend: str | Callable[[...], Component] | dict[str, Any] | KCell = 'wire_corner', straight_separation: float = 15.0, pad_pitch: float = 100.0, taper: str | Callable[[...], Component] | dict[str, Any] | KCell | None = None, port_type: str = 'electrical', allow_width_mismatch: bool = True, fanout_length: float | None = 80, route_width: float | list[float] | None = 0, **kwargs) Component [source]#
Returns new component with ports connected top pads.
- Parameters:
component – component spec to connect to.
port_names – list of port names to connect to pads.
component_name – optional for the label.
cross_section – cross_section function.
pad_port_name – pad port name.
pad – pad function.
bend – bend function.
straight_separation – from edge to edge.
pad_pitch – spacing between pads.
taper – taper function.
port_type – port type.
allow_width_mismatch – if True, allows width mismatch.
fanout_length – length of the fanout.
route_width – width of the route.
kwargs – additional arguments.
import gdsfactory as gf c = gf.c.nxn( xsize=600, ysize=200, north=2, south=3, wg_width=10, layer="M3", port_type="electrical", ) cc = gf.routing.add_pads_top(component=c, port_names=("e1", "e4"), fanout_length=50) cc.plot()
import cspdk
c = cspdk.si220.cells.add_pads_top(component='straight_heater_metal_sc', cross_section='metal_routing', pad_port_name='e1', pad='pad', bend='wire_corner', straight_separation=15.0, pad_pitch=100.0, port_type='electrical', allow_width_mismatch=True, fanout_length=80, route_width=0).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
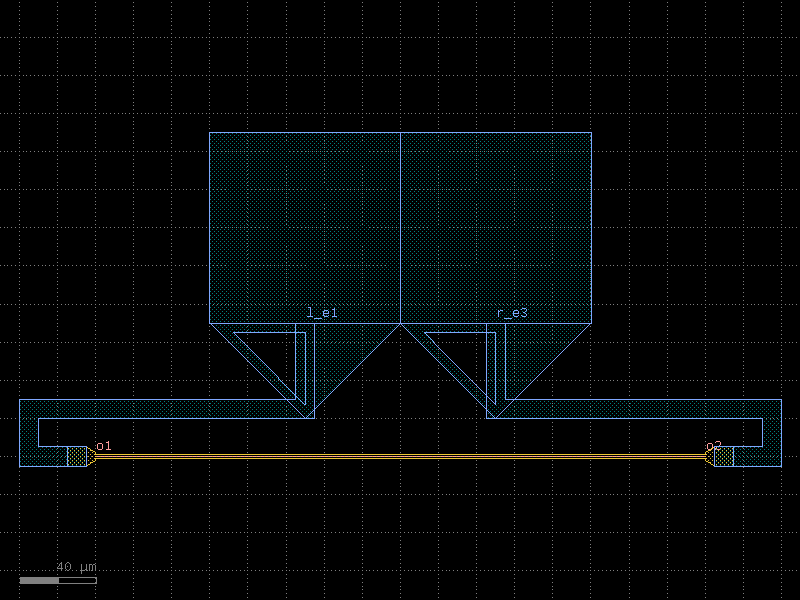
array#
- cspdk.si220.cells.array(component='pad', spacing: tuple[float, float] = (150.0, 150.0), columns: int = 6, rows: int = 1, add_ports: bool = True, size=None, centered: bool = False) Component [source]#
An array of components.
- Parameters:
component – the component of which to create an array
spacing – the x and y spacing by which to place the component
columns – the number of components to place in the x-direction
rows – the number of components to place in the y-direction
add_ports – add ports to the component
size – Optional x, y size. Overrides columns and rows.
centered – center the array around the origin.
import cspdk
c = cspdk.si220.cells.array(component='pad', spacing=(150.0, 150.0), columns=6, rows=1, add_ports=True, centered=False).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
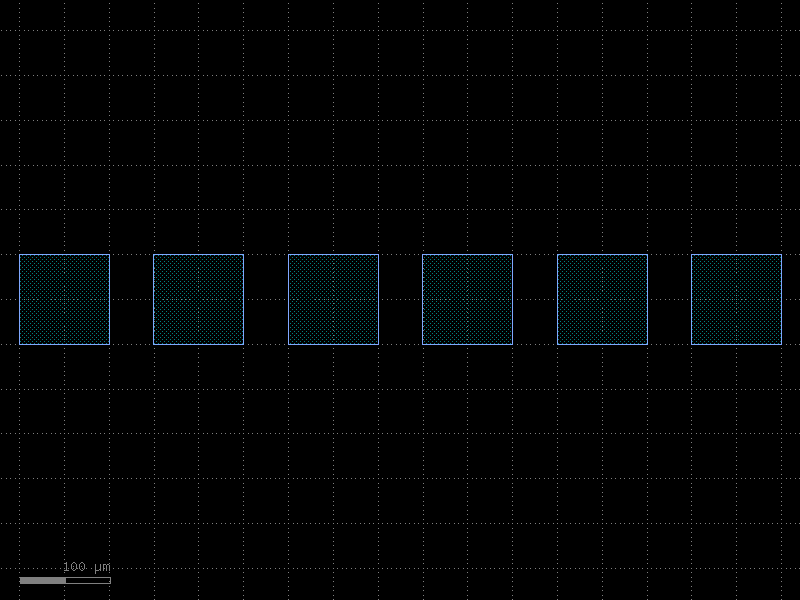
bend_euler#
- cspdk.si220.cells.bend_euler(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_euler(angle=90.0, p=0.5, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
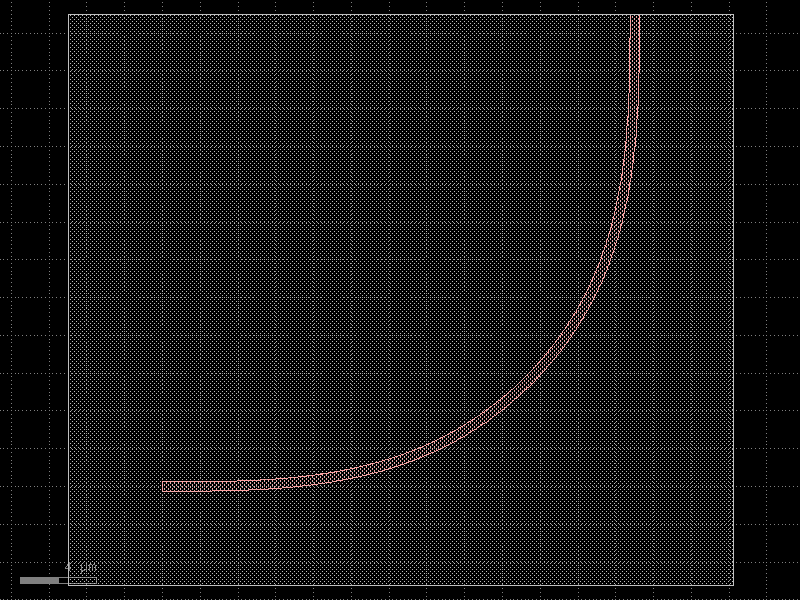
bend_euler_rc#
- cspdk.si220.cells.bend_euler_rc(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc') Component #
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_euler_rc(angle=90.0, p=0.5, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
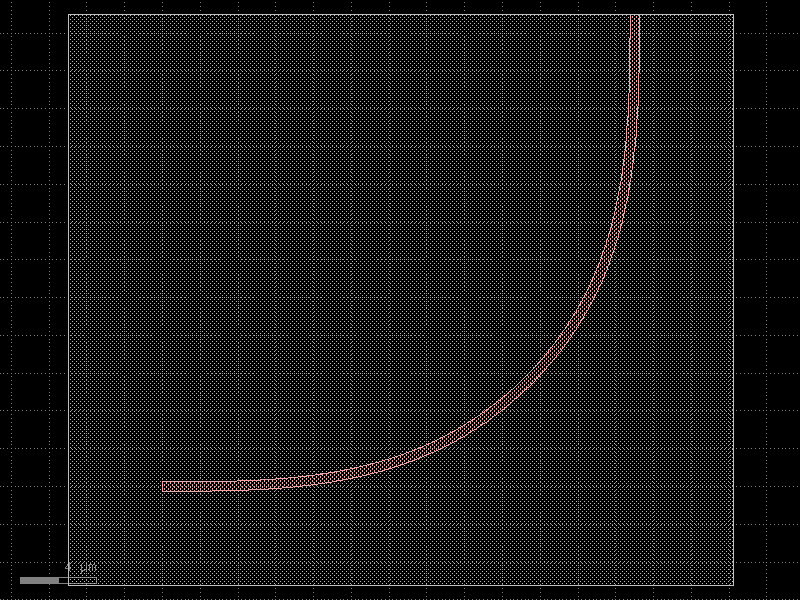
bend_euler_ro#
- cspdk.si220.cells.bend_euler_ro(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro') Component #
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_euler_ro(angle=90.0, p=0.5, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
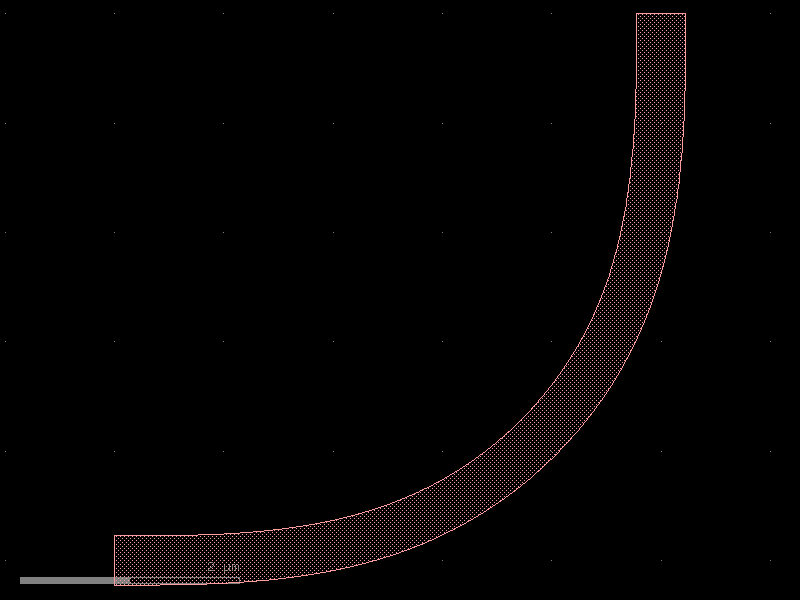
bend_euler_sc#
- cspdk.si220.cells.bend_euler_sc(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_euler_sc(angle=90.0, p=0.5, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
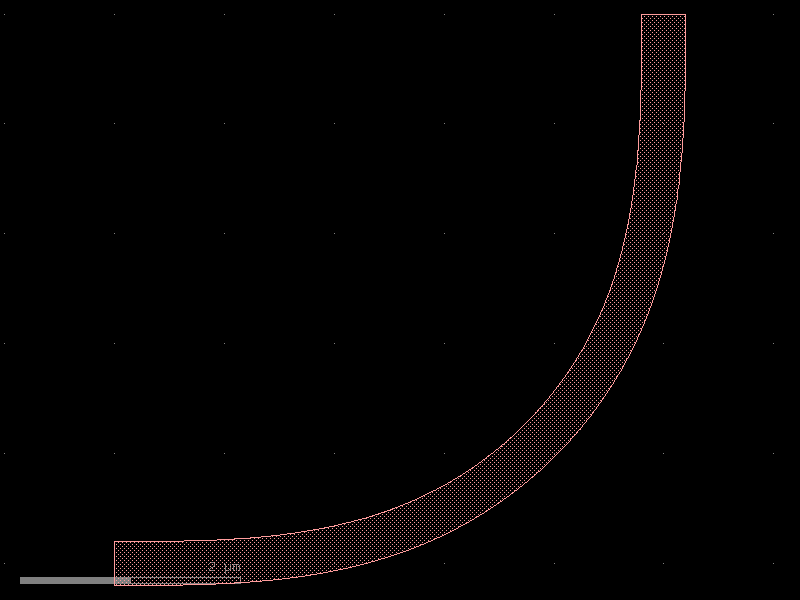
bend_euler_so#
- cspdk.si220.cells.bend_euler_so(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_euler_so(angle=90.0, p=0.5, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
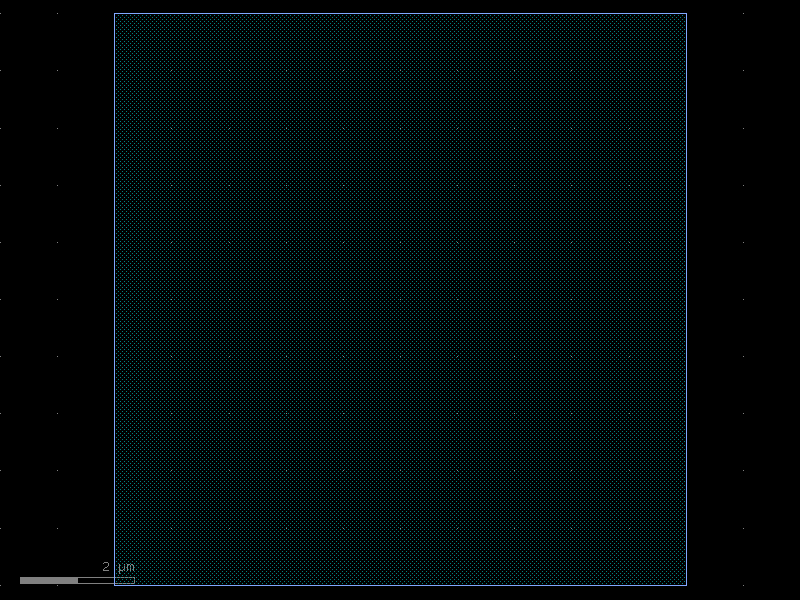
bend_metal#
- cspdk.si220.cells.bend_metal(radius: float | None = None, angle: float = 90.0, p: float = 0.5, width: float | None = None, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'metal_routing') Component #
An euler bend.
- Parameters:
radius – the effective radius of the bend.
angle – the angle of the bend (usually 90 degrees).
p – the fraction of the bend that’s represented by a polar bend.
width – the width of the waveguide forming the bend.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.bend_metal(angle=90.0, p=0.5, cross_section='metal_routing').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
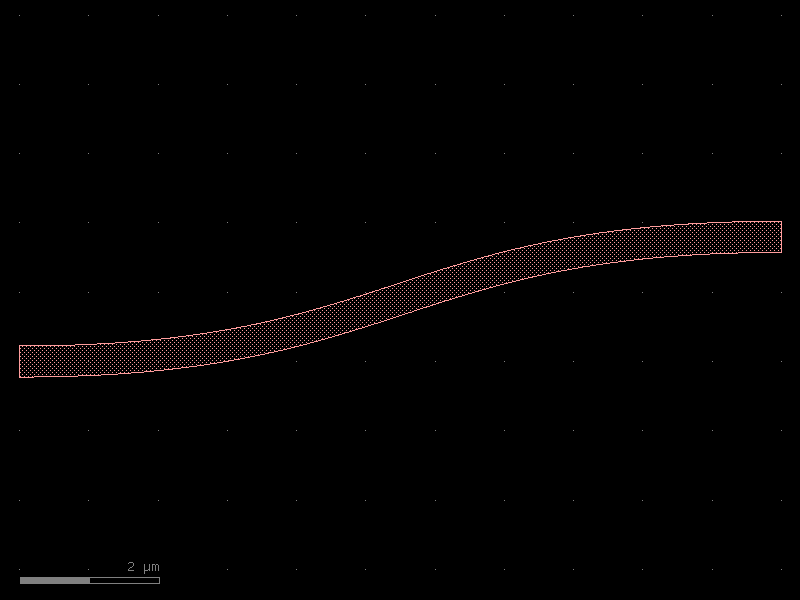
bend_s#
- cspdk.si220.cells.bend_s(size: tuple[float, float] = (11.0, 1.8), cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', **kwargs) Component [source]#
An S-bend.
- Parameters:
size – the width and height of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the bend_s function.
import cspdk
c = cspdk.si220.cells.bend_s(size=(11.0, 1.8), cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
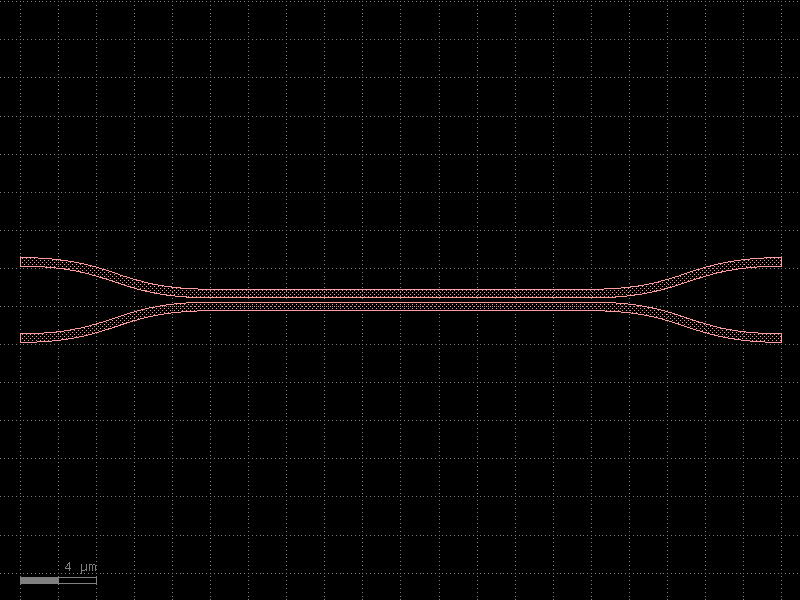
compass#
- cspdk.si220.cells.compass(size: tuple[float, float] = (4.0, 2.0), layer: tuple[int, int] | str | int | LayerEnum = 'PAD', port_type: str | None = 'electrical', port_inclusion: float = 0.0, port_orientations: tuple[int, ...] | list[int] | None = (180, 90, 0, -90), auto_rename_ports: bool = True) Component [source]#
Rectangle with ports on each edge (north, south, east, and west).
- Parameters:
size – rectangle size.
layer – tuple (int, int).
port_type – optical, electrical.
port_inclusion – from edge.
port_orientations – list of port_orientations to add. None does not add ports.
auto_rename_ports – auto rename ports.
import cspdk
c = cspdk.si220.cells.compass(size=(4.0, 2.0), layer='PAD', port_type='electrical', port_inclusion=0.0, port_orientations=(180, 90, 0, -90), auto_rename_ports=True).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
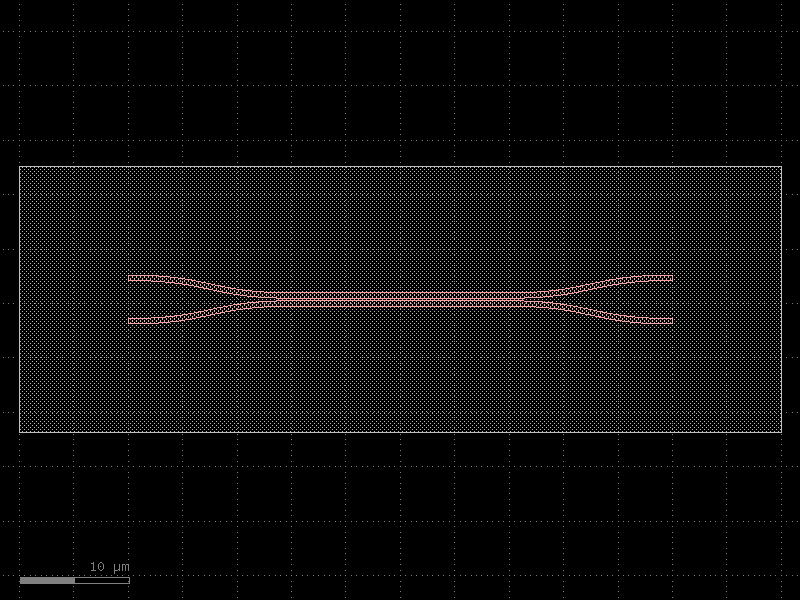
coupler#
- cspdk.si220.cells.coupler(gap: float = 0.234, length: float = 20.0, dy: float = 4.0, dx: float = 10.0, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler(gap=0.234, length=20.0, dy=4.0, dx=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
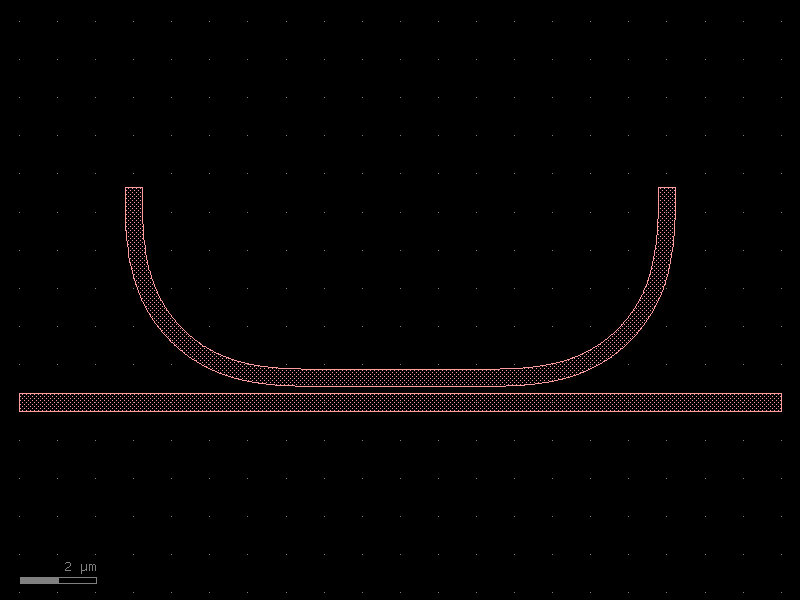
coupler_rc#
- cspdk.si220.cells.coupler_rc(gap: float = 0.234, length: float = 20.0, *, dy: float = 4.0, dx: float = 15, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc') Component #
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_rc(gap=0.234, length=20.0, dy=4.0, dx=15, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
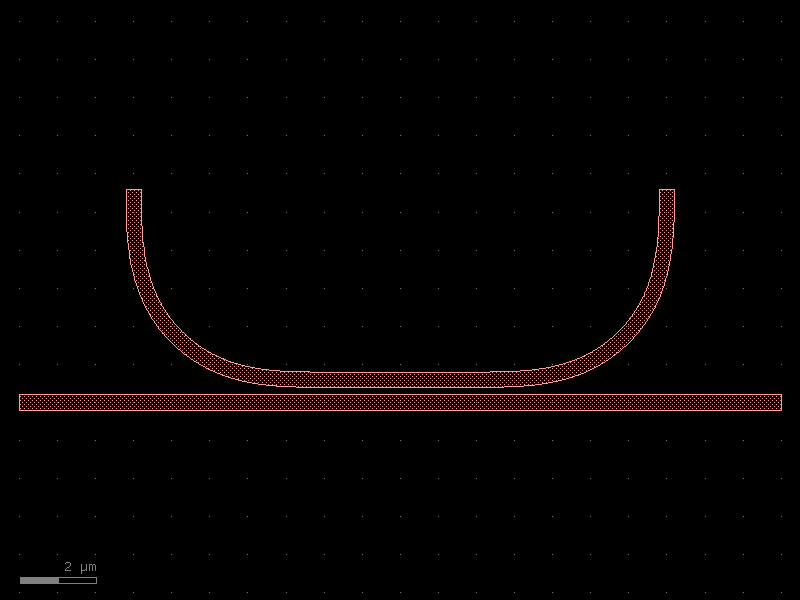
coupler_ring_sc#
- cspdk.si220.cells.coupler_ring_sc(gap: float = 0.2, radius: float = 5.0, length_x: float = 4.0, bend: str | Callable[[...], Component] | dict[str, Any] | KCell = 'bend_euler_sc', straight: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_sc', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', cross_section_bend: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection | None = None, length_extension: float = 3) Component [source]#
Coupler for ring.
- Parameters:
gap – spacing between parallel coupled straight waveguides.
radius – of the bends.
length_x – length of the parallel coupled straight waveguides.
bend – 90 degrees bend spec.
straight – straight spec.
cross_section – cross_section spec.
cross_section_bend – optional bend cross_section spec.
length_extension – for the ports.
o2 o3 | | \ / \ / ---=========--- o1 length_x o4
import cspdk
c = cspdk.si220.cells.coupler_ring_sc(gap=0.2, radius=5.0, length_x=4.0, bend='bend_euler_sc', straight='straight_sc', cross_section='xs_sc', length_extension=3).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
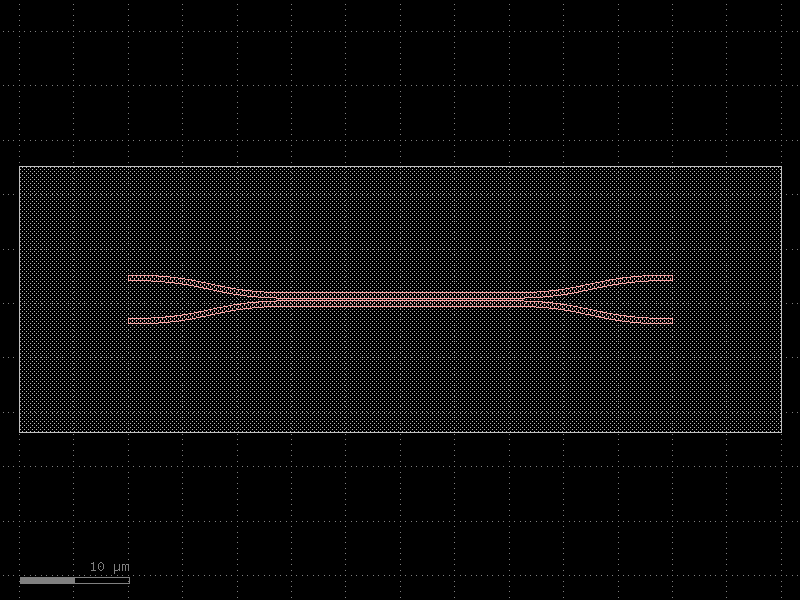
coupler_ring_so#
- cspdk.si220.cells.coupler_ring_so(gap: float = 0.2, radius: float = 5.0, length_x: float = 4.0, *, bend: str | Callable[[...], Component] | dict[str, Any] | KCell = 'bend_euler_so', straight: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_so', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', cross_section_bend: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection | None = None, length_extension: float = 3) Component #
Coupler for ring.
- Parameters:
gap – spacing between parallel coupled straight waveguides.
radius – of the bends.
length_x – length of the parallel coupled straight waveguides.
bend – 90 degrees bend spec.
straight – straight spec.
cross_section – cross_section spec.
cross_section_bend – optional bend cross_section spec.
length_extension – for the ports.
o2 o3 | | \ / \ / ---=========--- o1 length_x o4
import cspdk
c = cspdk.si220.cells.coupler_ring_so(gap=0.2, radius=5.0, length_x=4.0, bend='bend_euler_so', straight='straight_so', cross_section='xs_so', length_extension=3).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
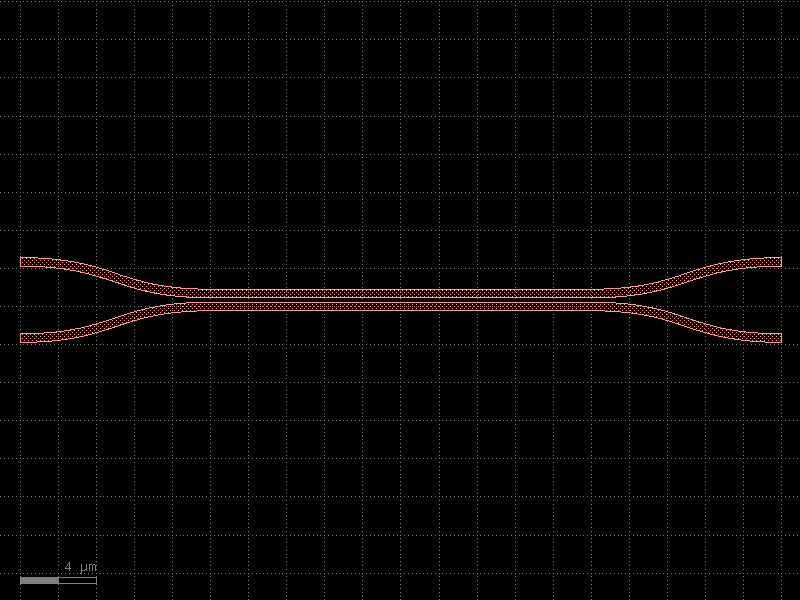
coupler_ro#
- cspdk.si220.cells.coupler_ro(gap: float = 0.234, length: float = 20.0, *, dy: float = 4.0, dx: float = 15, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro') Component #
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_ro(gap=0.234, length=20.0, dy=4.0, dx=15, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
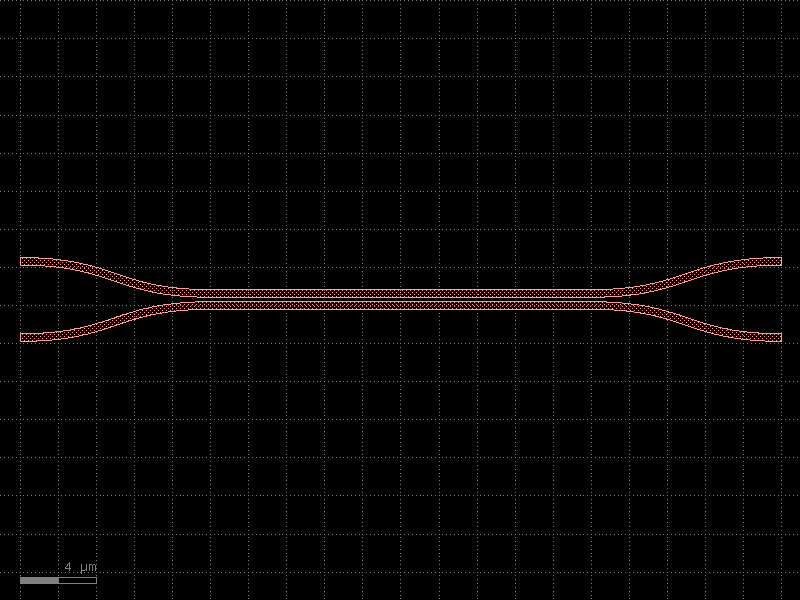
coupler_sc#
- cspdk.si220.cells.coupler_sc(gap: float = 0.234, length: float = 20.0, dy: float = 4.0, dx: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_sc(gap=0.234, length=20.0, dy=4.0, dx=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
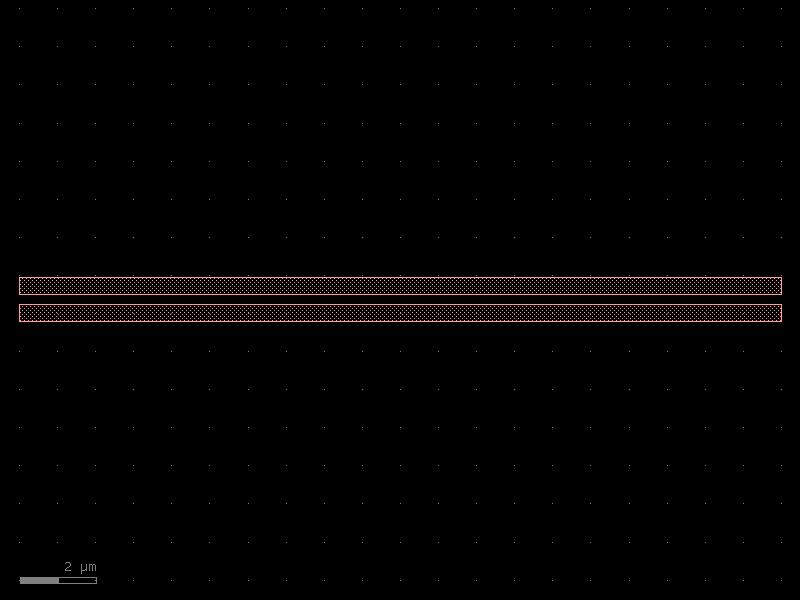
coupler_so#
- cspdk.si220.cells.coupler_so(gap: float = 0.234, length: float = 20.0, dy: float = 4.0, dx: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_so(gap=0.234, length=20.0, dy=4.0, dx=10.0, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
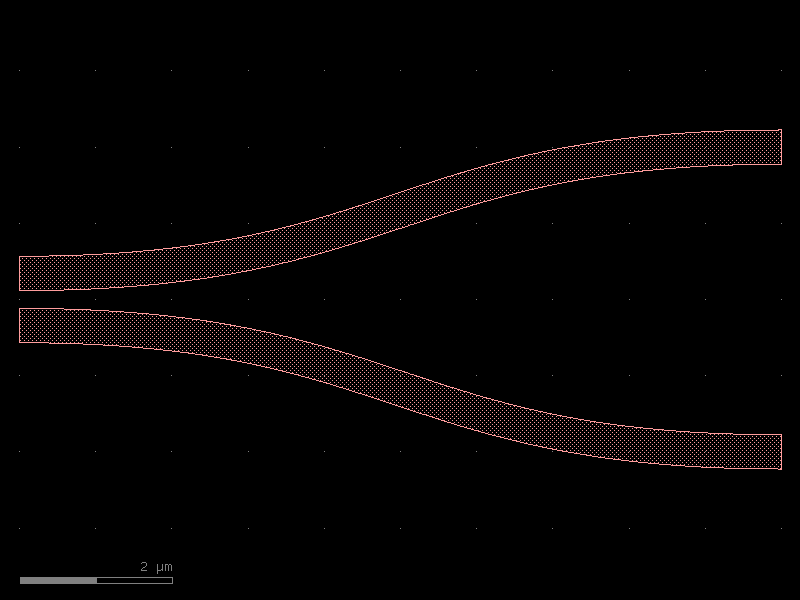
coupler_straight#
- cspdk.si220.cells.coupler_straight(length: float = 20.0, gap: float = 0.27, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
The straight part of a coupler.
- Parameters:
length – the length of the straight part of the coupler.
gap – the gap between the waveguides forming the straight part of the coupler.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_straight(length=20.0, gap=0.27, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
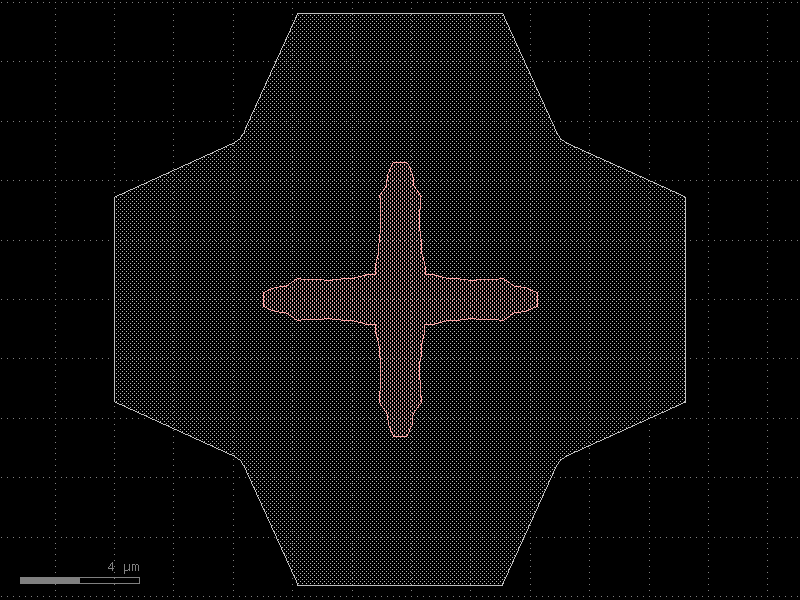
coupler_symmetric#
- cspdk.si220.cells.coupler_symmetric(gap: float = 0.234, dy: float = 4.0, dx: float = 10.0, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
The part of the coupler that diverges away from each other with s-bends.
- Parameters:
gap – the gap between the s-bends when closest together.
dy – the height of the s-bend.
dx – the length of the s-bend.
cross_section – a cross section or its name or a function generating a cross section..
import cspdk
c = cspdk.si220.cells.coupler_symmetric(gap=0.234, dy=4.0, dx=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
crossing_rc#
- cspdk.si220.cells.crossing_rc() Component [source]#
SOI220nm_1550nm_TE_RIB_Waveguide_Crossing fixed cell.
import cspdk
c = cspdk.si220.cells.crossing_rc().dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
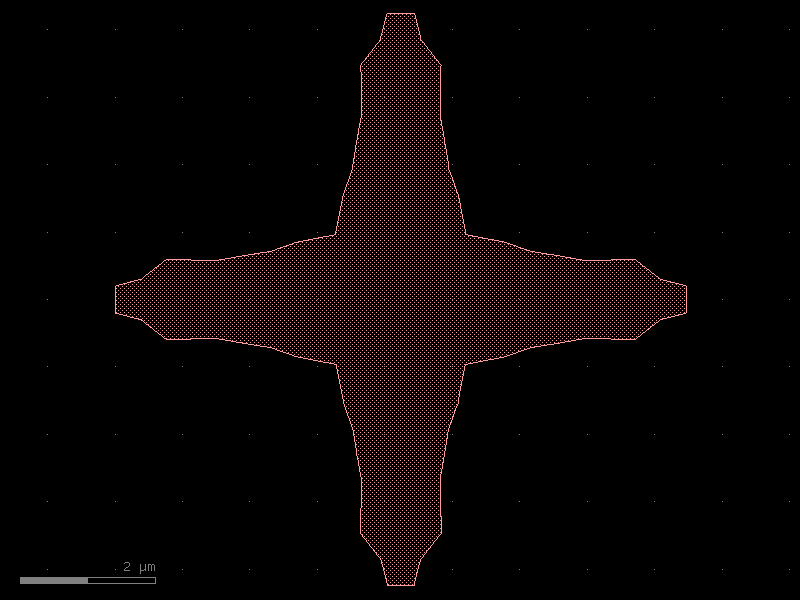
crossing_sc#
- cspdk.si220.cells.crossing_sc() Component [source]#
SOI220nm_1550nm_TE_STRIP_Waveguide_Crossing fixed cell.
import cspdk
c = cspdk.si220.cells.crossing_sc().dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
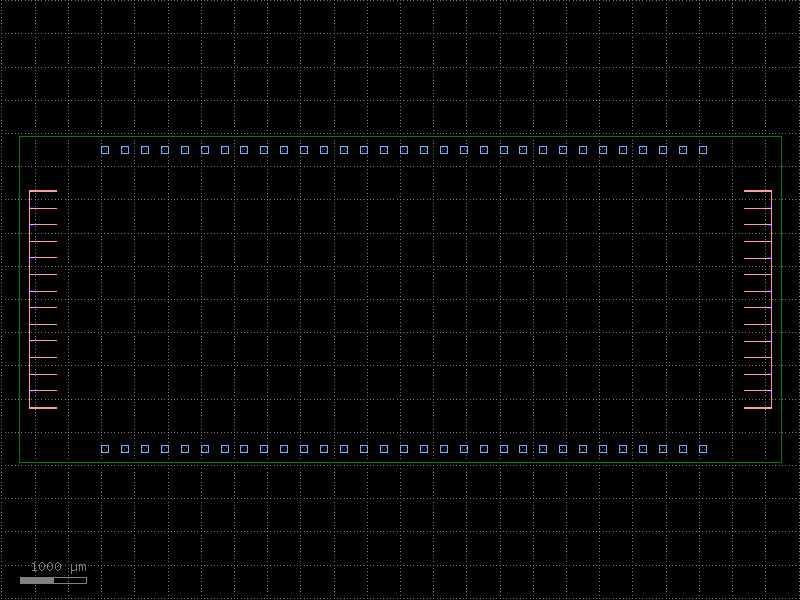
crossing_so#
- cspdk.si220.cells.crossing_so() Component [source]#
SOI220nm_1310nm_TE_STRIP_Waveguide_Crossing fixed cell.
import cspdk
c = cspdk.si220.cells.crossing_so().dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
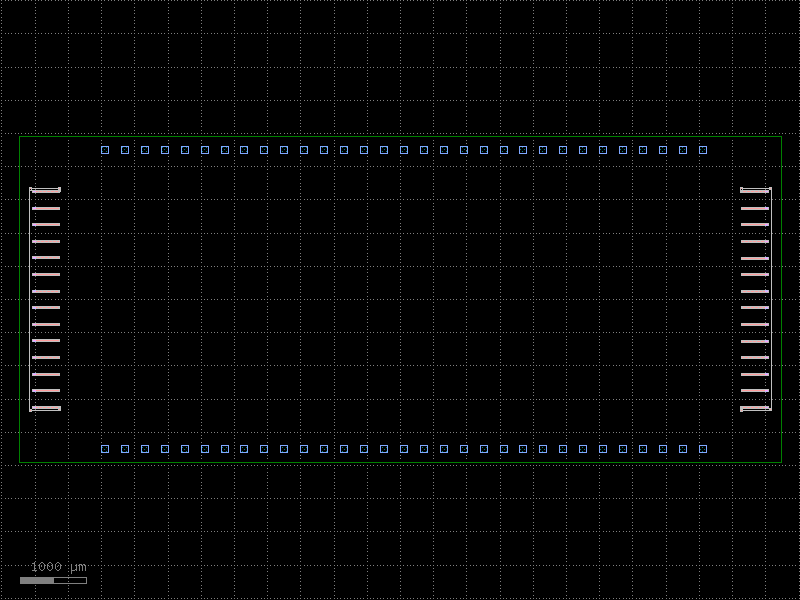
die#
- cspdk.si220.cells.die(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_coupler=None, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_sc') Component [source]#
A die template.
- Parameters:
size – the size of the die.
edge_to_grating_distance – the distance from the edge to the grating couplers.
edge_to_pad_distance – the distance from the edge to the pads.
grating_coupler – the name of the grating coupler to use in the array.
grating_pitch – the pitch of the grating couplers.
layer_floorplan – the layer to use for the floorplan.
ngratings – the number of grating couplers.
npads – the number of pads.
pad – the name of the pad to use in the array.
pad_pitch – the pitch of the pads.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
die_rc#
- cspdk.si220.cells.die_rc(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_coupler=None, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, *, cross_section='xs_rc') Component #
A die template.
- Parameters:
size – the size of the die.
edge_to_grating_distance – the distance from the edge to the grating couplers.
edge_to_pad_distance – the distance from the edge to the pads.
grating_coupler – the name of the grating coupler to use in the array.
grating_pitch – the pitch of the grating couplers.
layer_floorplan – the layer to use for the floorplan.
ngratings – the number of grating couplers.
npads – the number of pads.
pad – the name of the pad to use in the array.
pad_pitch – the pitch of the pads.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die_rc(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
die_ro#
- cspdk.si220.cells.die_ro(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_coupler=None, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, *, cross_section='xs_ro') Component #
A die template.
- Parameters:
size – the size of the die.
edge_to_grating_distance – the distance from the edge to the grating couplers.
edge_to_pad_distance – the distance from the edge to the pads.
grating_coupler – the name of the grating coupler to use in the array.
grating_pitch – the pitch of the grating couplers.
layer_floorplan – the layer to use for the floorplan.
ngratings – the number of grating couplers.
npads – the number of pads.
pad – the name of the pad to use in the array.
pad_pitch – the pitch of the pads.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die_ro(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
die_sc#
- cspdk.si220.cells.die_sc(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_coupler=None, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, *, cross_section='xs_sc') Component #
A die template.
- Parameters:
size – the size of the die.
edge_to_grating_distance – the distance from the edge to the grating couplers.
edge_to_pad_distance – the distance from the edge to the pads.
grating_coupler – the name of the grating coupler to use in the array.
grating_pitch – the pitch of the grating couplers.
layer_floorplan – the layer to use for the floorplan.
ngratings – the number of grating couplers.
npads – the number of pads.
pad – the name of the pad to use in the array.
pad_pitch – the pitch of the pads.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die_sc(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
die_so#
- cspdk.si220.cells.die_so(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_coupler=None, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, *, cross_section='xs_so') Component #
A die template.
- Parameters:
size – the size of the die.
edge_to_grating_distance – the distance from the edge to the grating couplers.
edge_to_pad_distance – the distance from the edge to the pads.
grating_coupler – the name of the grating coupler to use in the array.
grating_pitch – the pitch of the grating couplers.
layer_floorplan – the layer to use for the floorplan.
ngratings – the number of grating couplers.
npads – the number of pads.
pad – the name of the pad to use in the array.
pad_pitch – the pitch of the pads.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die_so(size=(11470.0, 4900.0), edge_to_grating_distance=150.0, edge_to_pad_distance=150.0, grating_pitch=250.0, layer_floorplan=<LayerMapCornerstone.FLOORPLAN: 46>, ngratings=14, npads=31, pad='pad', pad_pitch=300.0, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
grating_coupler_array#
- cspdk.si220.cells.grating_coupler_array(pitch: float = 127.0, n: int = 6, centered=True, grating_coupler=None, port_name='o1', with_loopback=False, rotation=-90, straight_to_grating_spacing=10.0, radius: float | None = None, cross_section='xs_sc') Component [source]#
An array of grating couplers.
- Parameters:
pitch – the pitch of the grating couplers
n – the number of grating couplers
centered – if True, centers the array around the origin.
grating_coupler – the name of the grating coupler to use in the array.
port_name – port name
with_loopback – if True, adds a loopback between edge GCs. Only works for rotation = 90 for now.
rotation – rotation angle for each reference.
straight_to_grating_spacing – spacing between the last grating coupler and the loopback.
radius – optional radius for routing the loopback.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_array(pitch=127.0, n=6, centered=True, port_name='o1', with_loopback=False, rotation=-90, straight_to_grating_spacing=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
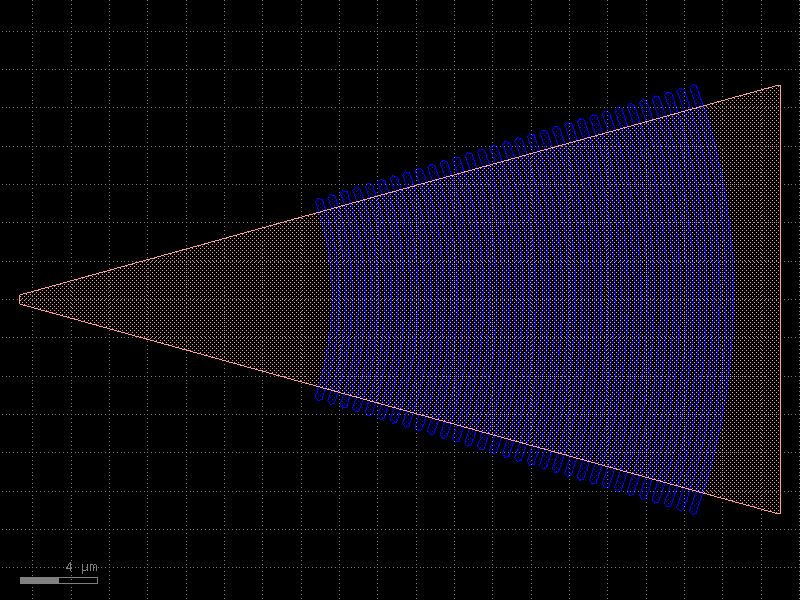
grating_coupler_elliptical#
- cspdk.si220.cells.grating_coupler_elliptical(wavelength: float = 1.55, grating_line_width=0.315, cross_section='xs_sc') Component [source]#
A grating coupler with curved but parallel teeth.
- Parameters:
wavelength – the center wavelength for which the grating is designed
grating_line_width – the line width of the grating
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical(wavelength=1.55, grating_line_width=0.315, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
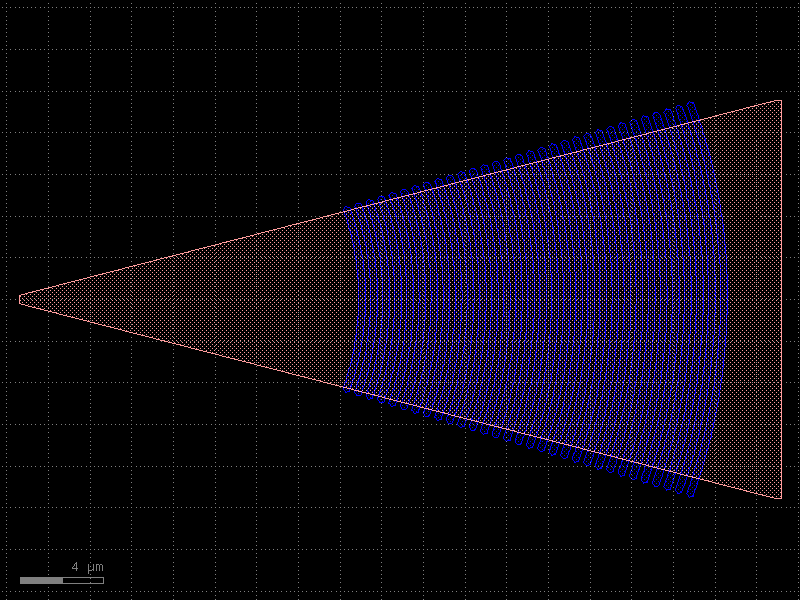
grating_coupler_elliptical_sc#
- cspdk.si220.cells.grating_coupler_elliptical_sc(*, wavelength: float = 1.55, grating_line_width=0.315, cross_section='xs_sc') Component #
A grating coupler with curved but parallel teeth.
- Parameters:
wavelength – the center wavelength for which the grating is designed
grating_line_width – the line width of the grating
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical_sc(wavelength=1.55, grating_line_width=0.315, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
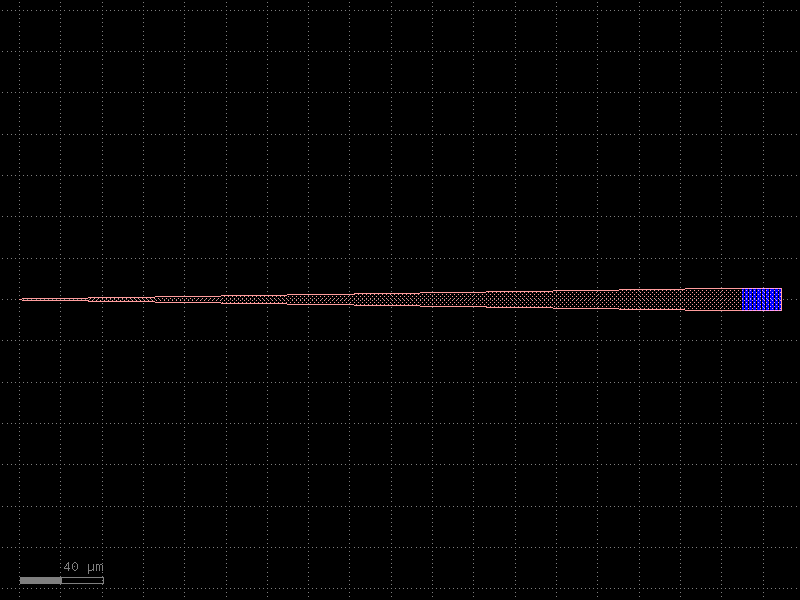
grating_coupler_elliptical_so#
- cspdk.si220.cells.grating_coupler_elliptical_so(*, wavelength: float = 1.31, grating_line_width=0.25, cross_section='xs_so') Component #
A grating coupler with curved but parallel teeth.
- Parameters:
wavelength – the center wavelength for which the grating is designed
grating_line_width – the line width of the grating
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical_so(wavelength=1.31, grating_line_width=0.25, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
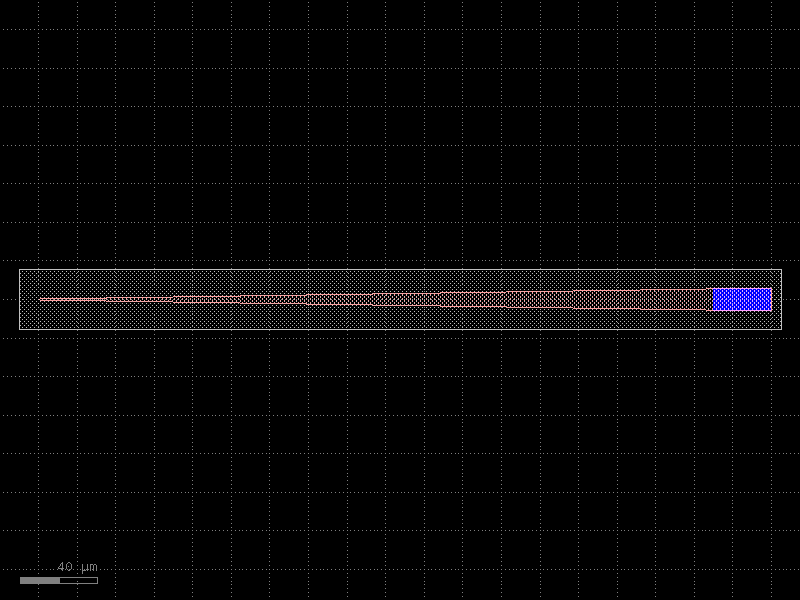
grating_coupler_rectangular#
- cspdk.si220.cells.grating_coupler_rectangular(period=0.63, n_periods: int = 30, length_taper: float = 350.0, wavelength: float = 1.55, cross_section='xs_sc') Component [source]#
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular(period=0.63, n_periods=30, length_taper=350.0, wavelength=1.55, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
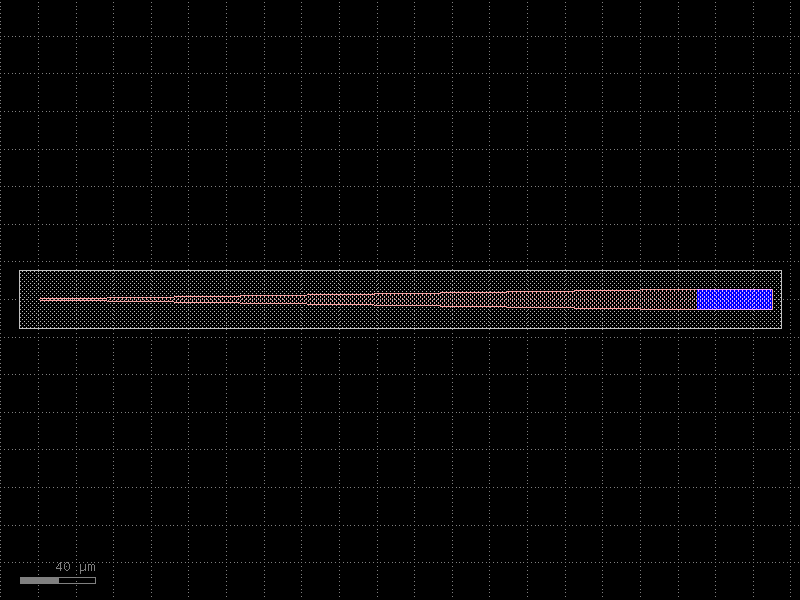
grating_coupler_rectangular_rc#
- cspdk.si220.cells.grating_coupler_rectangular_rc(*, period=0.5, n_periods: int = 60, length_taper: float = 350.0, wavelength: float = 1.55, cross_section='xs_rc') Component #
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_rc(period=0.5, n_periods=60, length_taper=350.0, wavelength=1.55, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
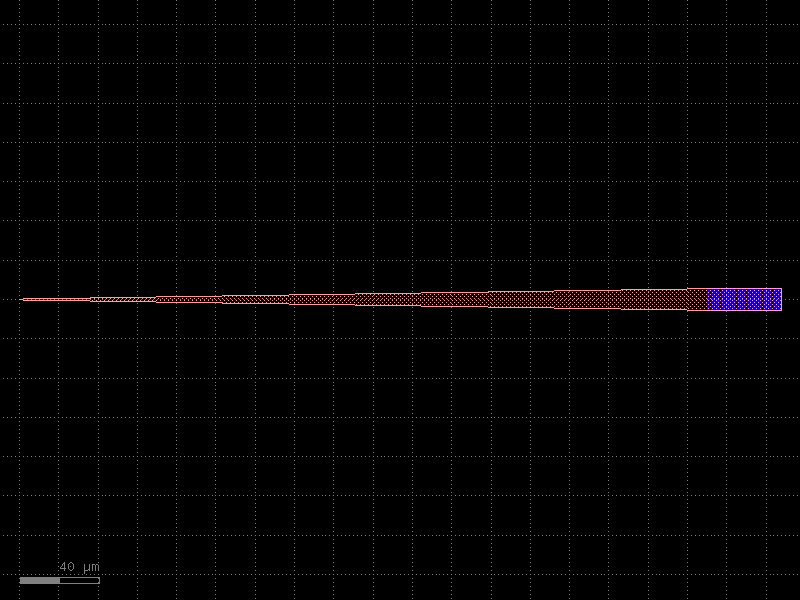
grating_coupler_rectangular_ro#
- cspdk.si220.cells.grating_coupler_rectangular_ro(*, period=0.5, n_periods: int = 80, length_taper: float = 350.0, wavelength: float = 1.55, cross_section='xs_ro') Component #
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_ro(period=0.5, n_periods=80, length_taper=350.0, wavelength=1.55, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
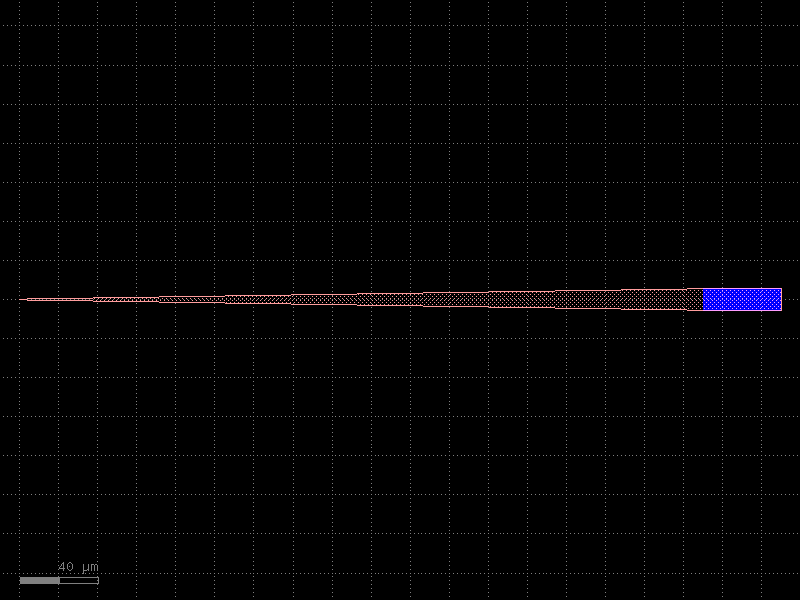
grating_coupler_rectangular_sc#
- cspdk.si220.cells.grating_coupler_rectangular_sc(period=0.63, *, n_periods: int = 60, length_taper: float = 350.0, wavelength: float = 1.55, cross_section='xs_sc') Component #
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_sc(period=0.63, n_periods=60, length_taper=350.0, wavelength=1.55, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
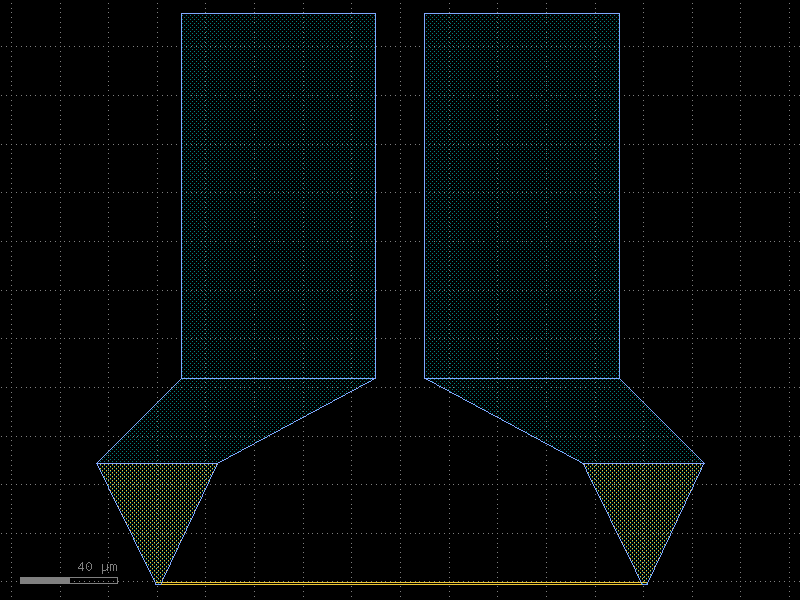
grating_coupler_rectangular_so#
- cspdk.si220.cells.grating_coupler_rectangular_so(*, period=0.5, n_periods: int = 80, length_taper: float = 350.0, wavelength: float = 1.31, cross_section='xs_so') Component #
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_so(period=0.5, n_periods=80, length_taper=350.0, wavelength=1.31, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
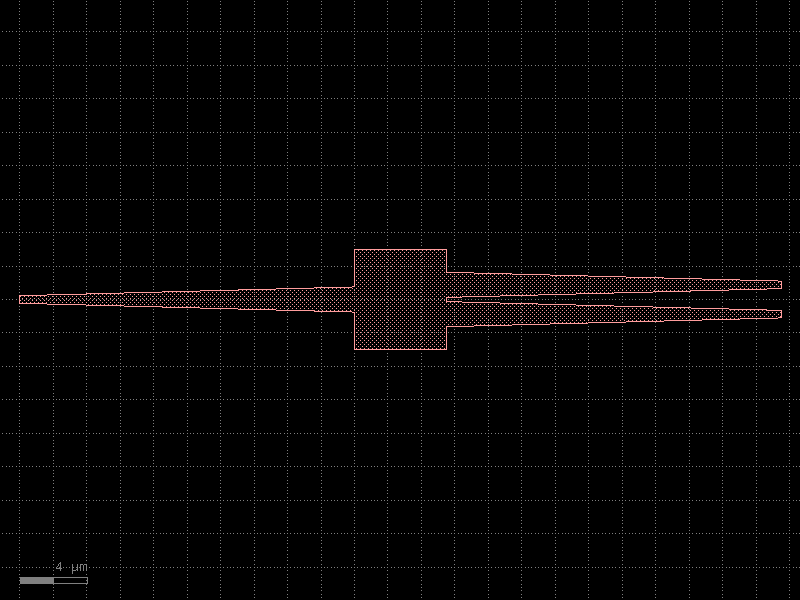
heater#
import cspdk
c = cspdk.si220.cells.heater().dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
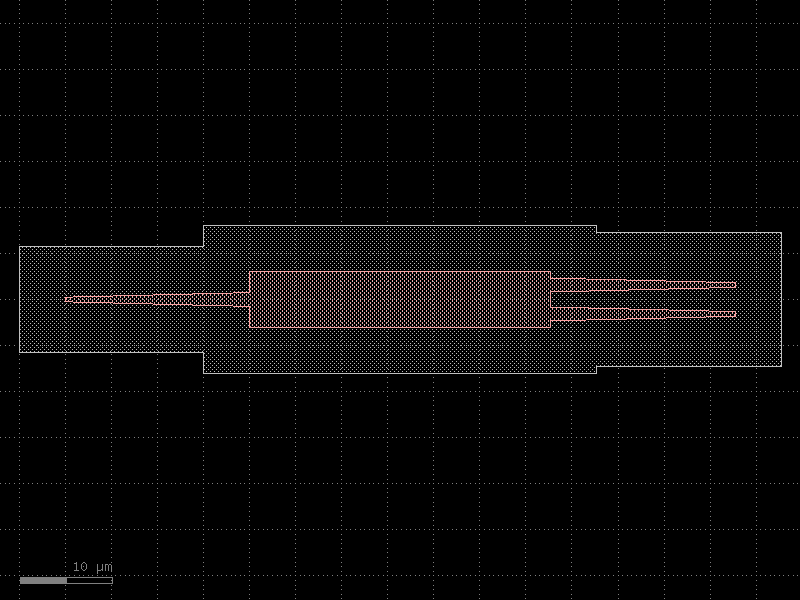
mmi1x2#
- cspdk.si220.cells.mmi1x2(width: float | None = None, width_taper=1.5, length_taper=20.0, length_mmi: float = 5.5, width_mmi=6.0, gap_mmi: float = 0.25, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2(width_taper=1.5, length_taper=20.0, length_mmi=5.5, width_mmi=6.0, gap_mmi=0.25, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
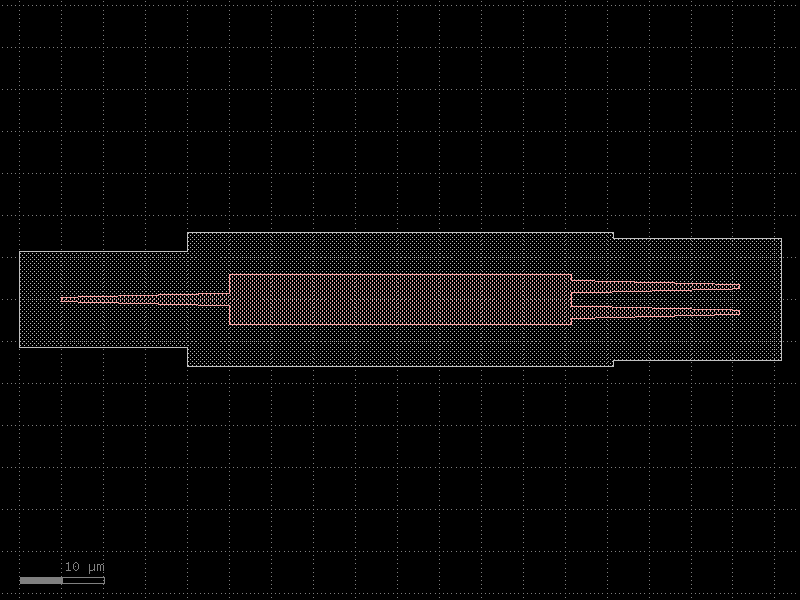
mmi1x2_rc#
- cspdk.si220.cells.mmi1x2_rc(width: float | None = None, width_taper=1.5, length_taper=20.0, *, length_mmi: float = 32.7, width_mmi=6.0, gap_mmi: float = 1.64, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc') Component #
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2_rc(width_taper=1.5, length_taper=20.0, length_mmi=32.7, width_mmi=6.0, gap_mmi=1.64, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
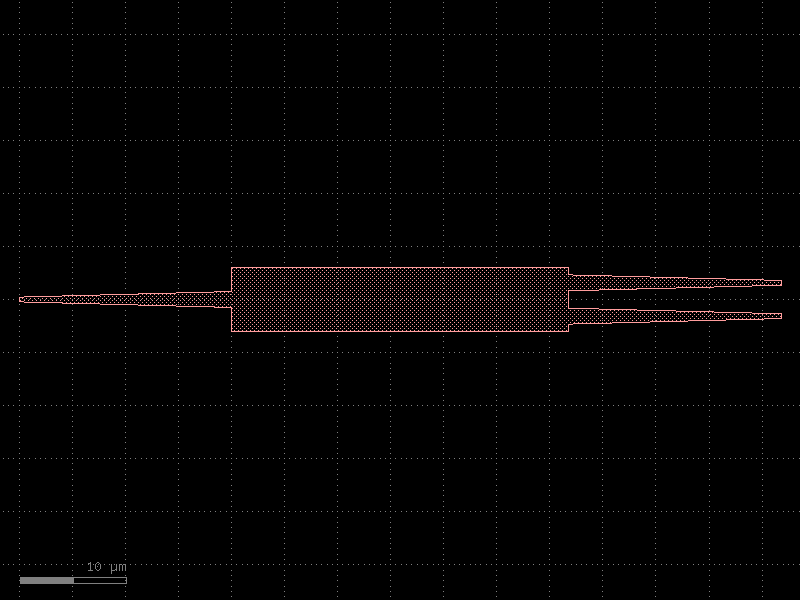
mmi1x2_ro#
- cspdk.si220.cells.mmi1x2_ro(width: float | None = None, width_taper=1.5, length_taper=20.0, *, length_mmi: float = 40.8, width_mmi=6.0, gap_mmi: float = 1.55, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro') Component #
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2_ro(width_taper=1.5, length_taper=20.0, length_mmi=40.8, width_mmi=6.0, gap_mmi=1.55, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
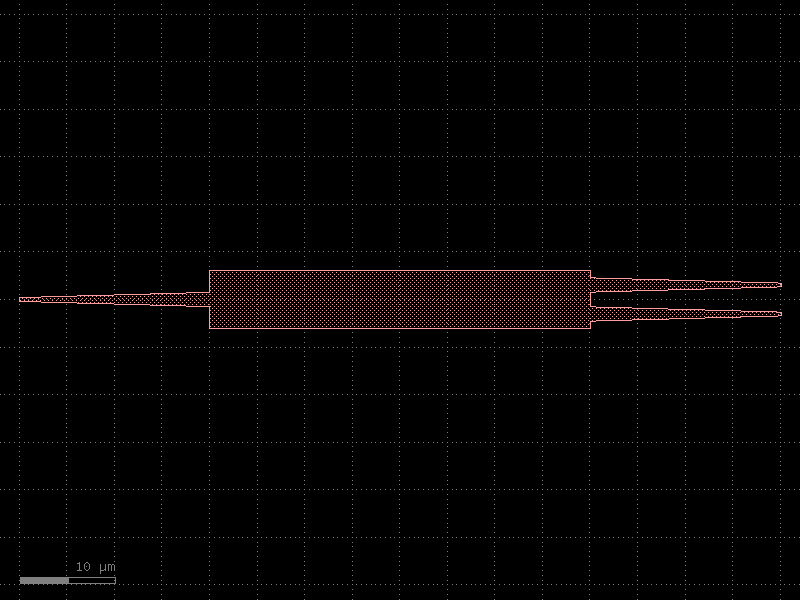
mmi1x2_sc#
- cspdk.si220.cells.mmi1x2_sc(width: float | None = None, width_taper=1.5, length_taper=20.0, *, length_mmi: float = 31.8, width_mmi=6.0, gap_mmi: float = 1.64, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2_sc(width_taper=1.5, length_taper=20.0, length_mmi=31.8, width_mmi=6.0, gap_mmi=1.64, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
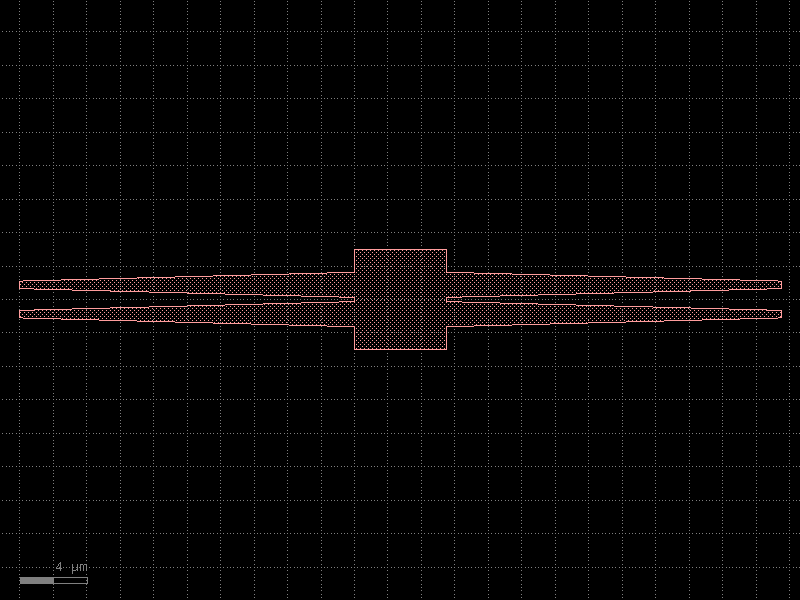
mmi1x2_so#
- cspdk.si220.cells.mmi1x2_so(width: float | None = None, width_taper=1.5, length_taper=20.0, *, length_mmi: float = 40.1, width_mmi=6.0, gap_mmi: float = 1.55, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2_so(width_taper=1.5, length_taper=20.0, length_mmi=40.1, width_mmi=6.0, gap_mmi=1.55, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
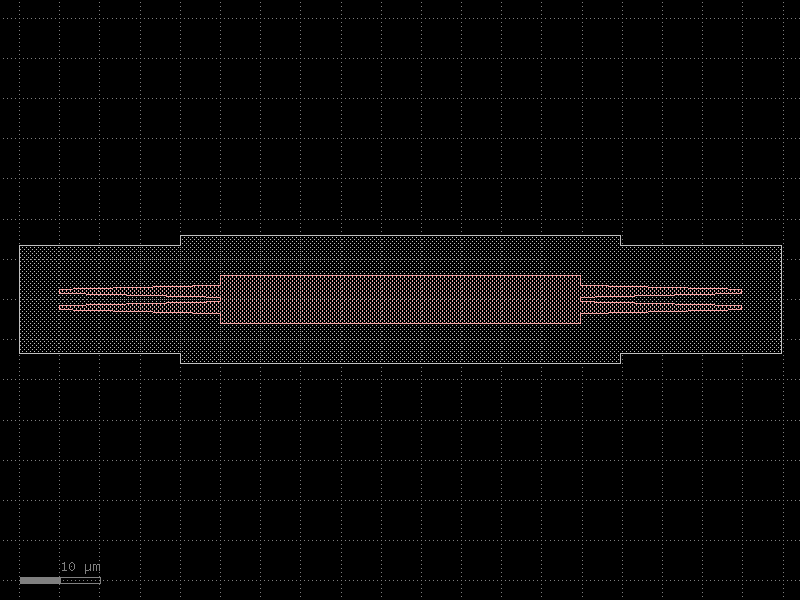
mmi2x2#
- cspdk.si220.cells.mmi2x2(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, length_mmi: float = 5.5, width_mmi: float = 6.0, gap_mmi: float = 0.25, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2(width_taper=1.5, length_taper=20.0, length_mmi=5.5, width_mmi=6.0, gap_mmi=0.25, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
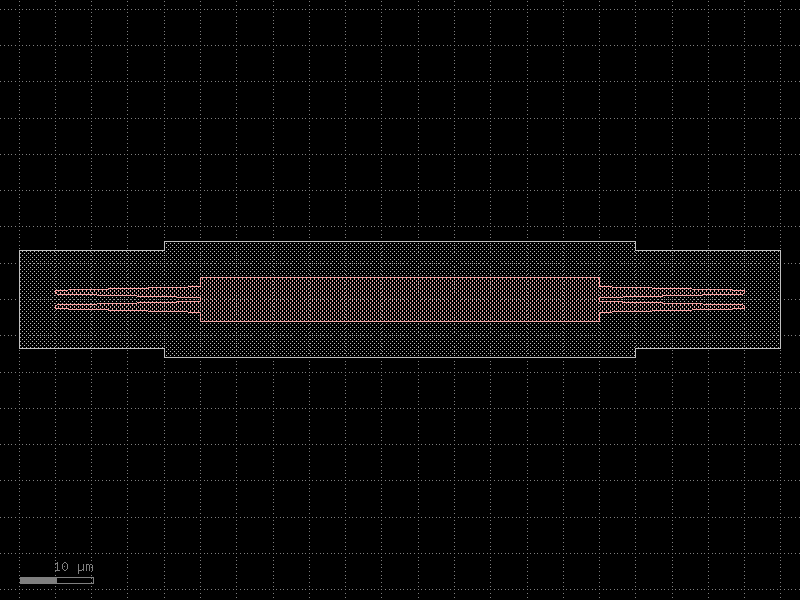
mmi2x2_rc#
- cspdk.si220.cells.mmi2x2_rc(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, *, length_mmi: float = 44.8, width_mmi: float = 6.0, gap_mmi: float = 0.53, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc') Component #
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2_rc(width_taper=1.5, length_taper=20.0, length_mmi=44.8, width_mmi=6.0, gap_mmi=0.53, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
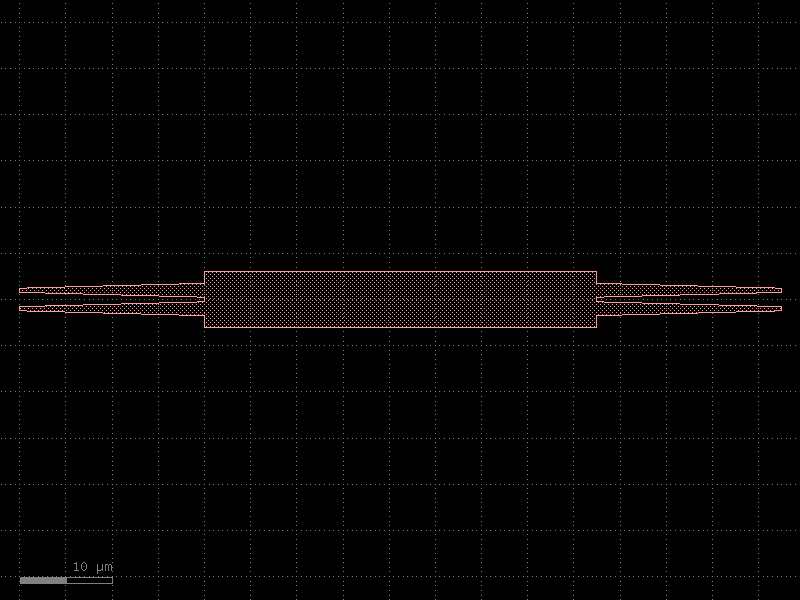
mmi2x2_ro#
- cspdk.si220.cells.mmi2x2_ro(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, *, length_mmi: float = 55.0, width_mmi: float = 6.0, gap_mmi: float = 0.53, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro') Component #
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2_ro(width_taper=1.5, length_taper=20.0, length_mmi=55.0, width_mmi=6.0, gap_mmi=0.53, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
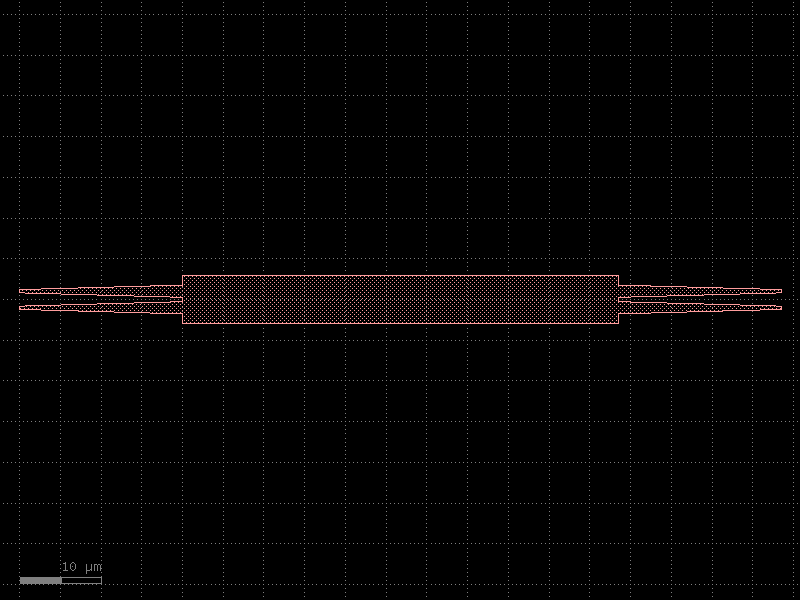
mmi2x2_sc#
- cspdk.si220.cells.mmi2x2_sc(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, *, length_mmi: float = 42.5, width_mmi: float = 6.0, gap_mmi: float = 0.5, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2_sc(width_taper=1.5, length_taper=20.0, length_mmi=42.5, width_mmi=6.0, gap_mmi=0.5, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
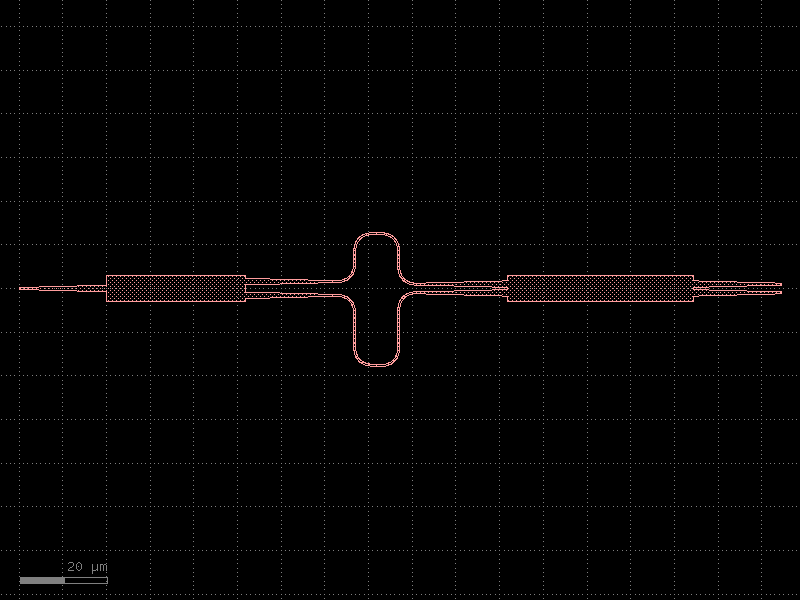
mmi2x2_so#
- cspdk.si220.cells.mmi2x2_so(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, *, length_mmi: float = 53.5, width_mmi: float = 6.0, gap_mmi: float = 0.53, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2_so(width_taper=1.5, length_taper=20.0, length_mmi=53.5, width_mmi=6.0, gap_mmi=0.53, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
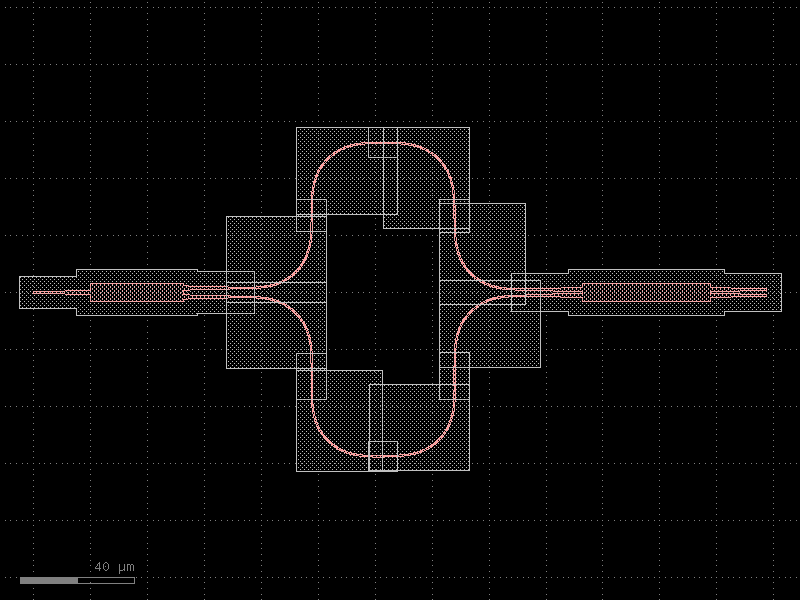
mzi#
- cspdk.si220.cells.mzi(delta_length: float = 10.0, bend='bend_euler_sc', straight='straight_sc', splitter='mmi1x2_sc', combiner='mmi2x2_sc', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi(delta_length=10.0, bend='bend_euler_sc', straight='straight_sc', splitter='mmi1x2_sc', combiner='mmi2x2_sc', cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
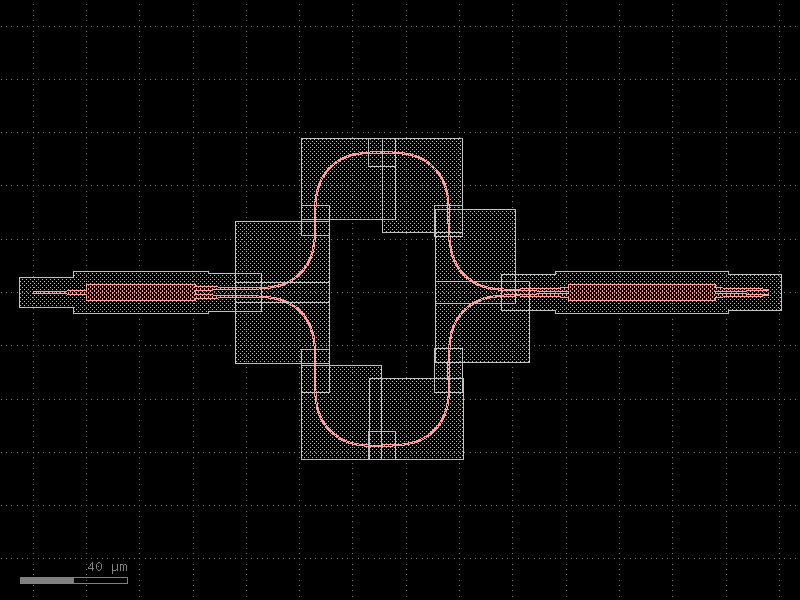
mzi_rc#
- cspdk.si220.cells.mzi_rc(delta_length: float = 10.0, *, bend='bend_euler_rc', straight='straight_rc', splitter='mmi1x2_rc', combiner='mmi2x2_rc', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc') Component #
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi_rc(delta_length=10.0, bend='bend_euler_rc', straight='straight_rc', splitter='mmi1x2_rc', combiner='mmi2x2_rc', cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
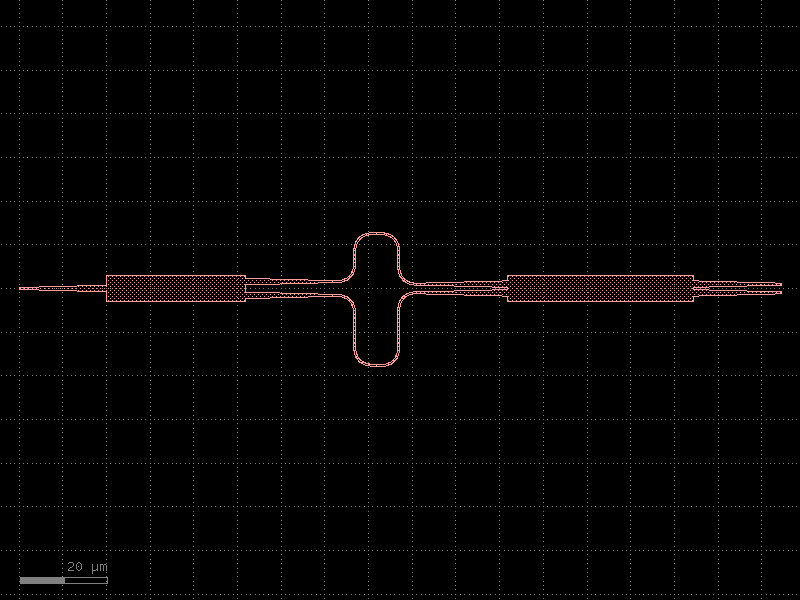
mzi_ro#
- cspdk.si220.cells.mzi_ro(delta_length: float = 10.0, *, bend='bend_euler_ro', straight='straight_ro', splitter='mmi1x2_ro', combiner='mmi2x2_ro', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro') Component #
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi_ro(delta_length=10.0, bend='bend_euler_ro', straight='straight_ro', splitter='mmi1x2_ro', combiner='mmi2x2_ro', cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
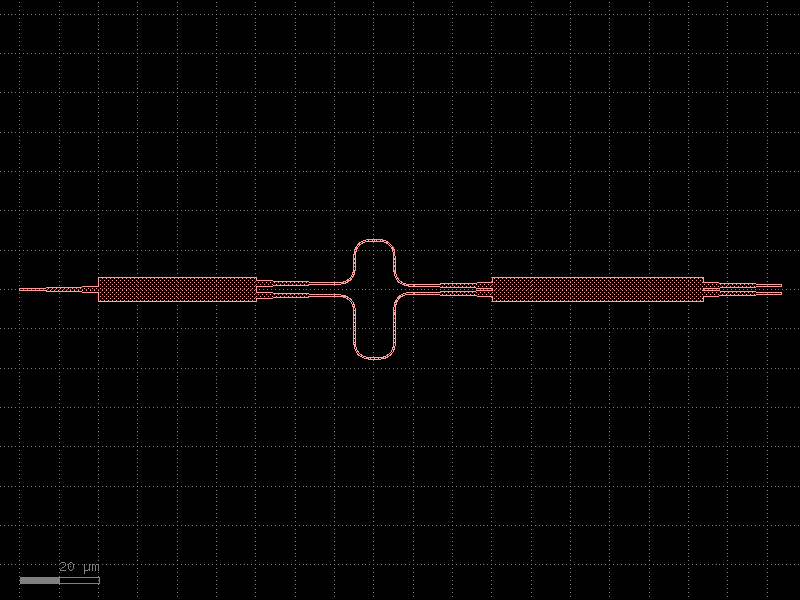
mzi_sc#
- cspdk.si220.cells.mzi_sc(delta_length: float = 10.0, *, bend='bend_euler_sc', straight='straight_sc', splitter='mmi1x2_sc', combiner='mmi2x2_sc', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi_sc(delta_length=10.0, bend='bend_euler_sc', straight='straight_sc', splitter='mmi1x2_sc', combiner='mmi2x2_sc', cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
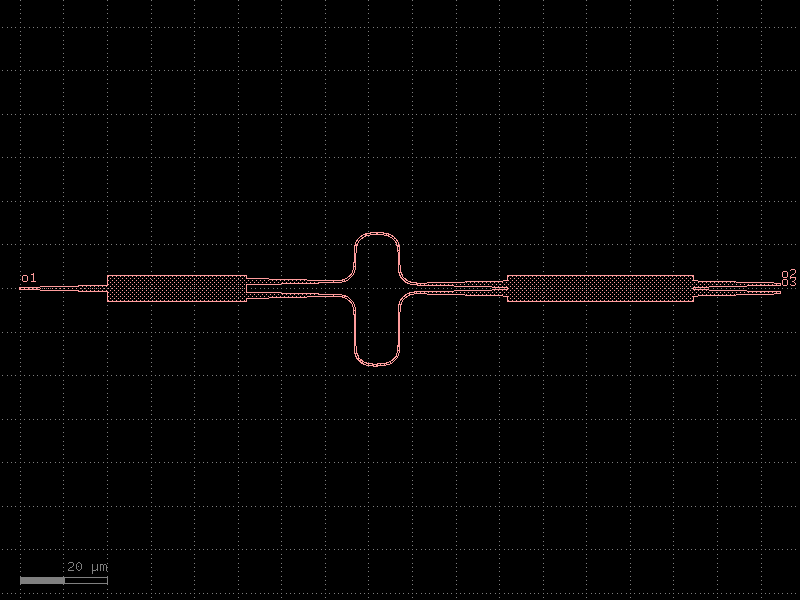
mzi_so#
- cspdk.si220.cells.mzi_so(delta_length: float = 10.0, *, bend='bend_euler_so', straight='straight_so', splitter='mmi1x2_so', combiner='mmi2x2_so', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi_so(delta_length=10.0, bend='bend_euler_so', straight='straight_so', splitter='mmi1x2_so', combiner='mmi2x2_so', cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
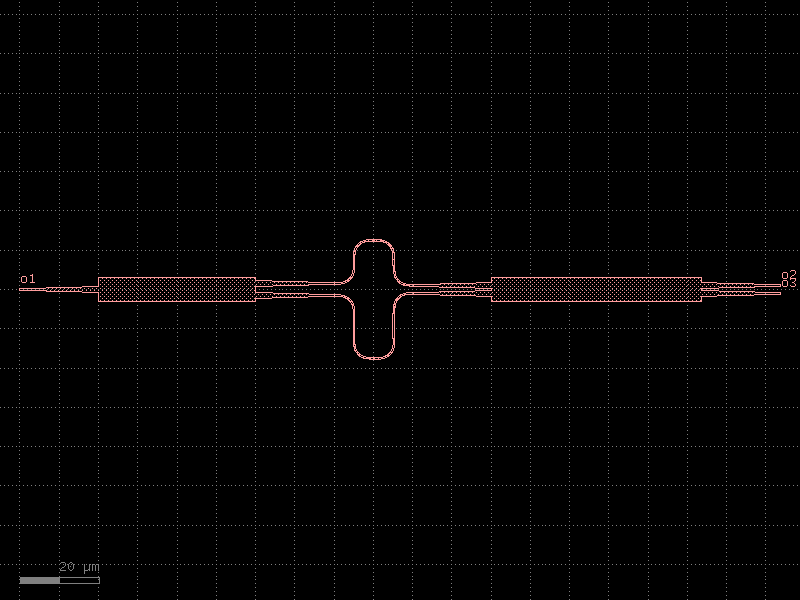
pack_doe#
- cspdk.si220.cells.pack_doe(doe: ComponentSpec, settings: dict[str, Sequence[Any]], do_permutations: bool = False, function: str | Callable[[...], Component] | dict[str, Any] | None = None, **kwargs: Any) Component [source]#
Packs a component DOE (Design of Experiment) using pack.
- Parameters:
doe – function to return Components.
settings – component settings.
do_permutations – for each setting.
function – to apply (add padding, grating couplers).
kwargs – for pack.
- Keyword Arguments:
spacing – Minimum distance between adjacent shapes.
aspect_ratio – (width, height) ratio of the rectangular bin.
max_size – Limits the size into which the shapes will be packed.
sort_by_area – Pre-sorts the shapes by area.
density – Values closer to 1 pack tighter but require more computation.
precision – Desired precision for rounding vertex coordinates.
text – Optional function to add text labels.
text_prefix – for labels. For example. ‘A’ for ‘A1’, ‘A2’…
text_offsets – relative to component size info anchor. Defaults to center.
text_anchors – relative to component (ce cw nc ne nw sc se sw center cc).
name_prefix – for each packed component (avoids the Unnamed cells warning). Note that the suffix contains a uuid so the name will not be deterministic.
rotation – for each component in degrees.
h_mirror – horizontal mirror in y axis (x, 1) (1, 0). This is the most common.
v_mirror – vertical mirror using x axis (1, y) (0, y).
import cspdk
c = cspdk.si220.cells.pack_doe(do_permutations=False).dup()
c.draw_ports()
c.plot()
pack_doe_grid#
- cspdk.si220.cells.pack_doe_grid(doe: ComponentSpec, settings: dict[str, Sequence[Any]], do_permutations: bool = False, function: str | Callable[[...], Component] | dict[str, Any] | None = None, with_text: bool = False, **kwargs: Any) Component [source]#
Packs a component DOE (Design of Experiment) using grid.
- Parameters:
doe – function to return Components.
settings – component settings.
do_permutations – for each setting.
function – to apply to component (add padding, grating couplers).
with_text – includes text label.
kwargs – for grid.
- Keyword Arguments:
spacing – between adjacent elements on the grid, can be a tuple for different distances in height and width.
separation – If True, guarantees elements are separated with fixed spacing if False, elements are spaced evenly along a grid.
shape – x, y shape of the grid (see np.reshape). If no shape and the list is 1D, if np.reshape were run with (1, -1).
align_x – {‘x’, ‘xmin’, ‘xmax’} for x (column) alignment along.
align_y – {‘y’, ‘ymin’, ‘ymax’} for y (row) alignment along.
edge_x – {‘x’, ‘xmin’, ‘xmax’} for x (column) (ignored if separation = True).
edge_y – {‘y’, ‘ymin’, ‘ymax’} for y (row) (ignored if separation = True).
rotation – for each component in degrees.
h_mirror – horizontal mirror y axis (x, 1) (1, 0). most common mirror.
v_mirror – vertical mirror using x axis (1, y) (0, y).
import cspdk
c = cspdk.si220.cells.pack_doe_grid(do_permutations=False, with_text=False).dup()
c.draw_ports()
c.plot()
pad#
import cspdk
c = cspdk.si220.cells.pad().dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
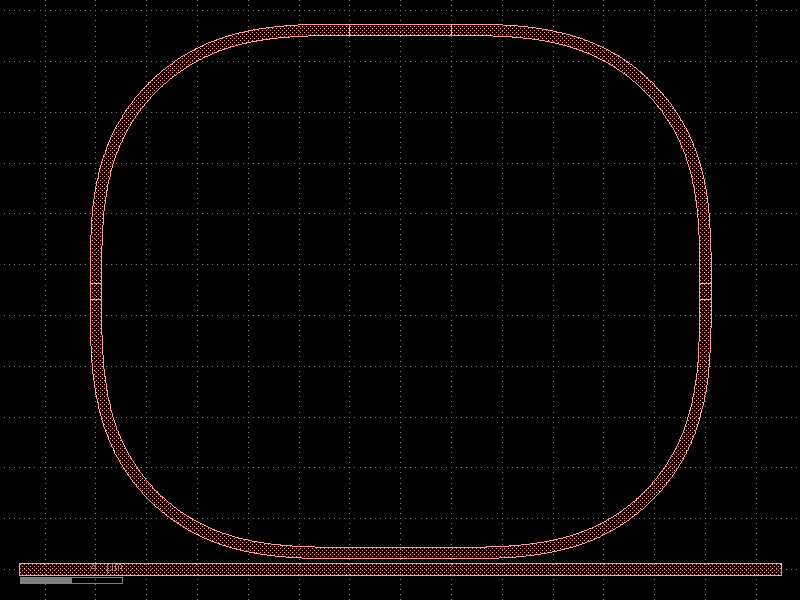
rectangle#
- cspdk.si220.cells.rectangle(layer=<LayerMapCornerstone.FLOORPLAN: 46>, **kwargs) Component [source]#
A rectangle.
import cspdk
c = cspdk.si220.cells.rectangle(layer=<LayerMapCornerstone.FLOORPLAN: 46>).dup()
c.draw_ports()
c.plot()
ring_single_sc#
- cspdk.si220.cells.ring_single_sc(gap: float = 0.2, radius: float = 10.0, length_x: float = 4.0, length_y: float = 0.6, bend: str | Callable[[...], Component] | dict[str, Any] | KCell = 'bend_euler_sc', straight: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_sc', coupler_ring: str | Callable[[...], Component] | dict[str, Any] | KCell = 'coupler_ring_sc', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
Returns a single ring.
ring coupler (cb: bottom) connects to two vertical straights (sl: left, sr: right), two bends (bl, br) and horizontal straight (wg: top)
- Parameters:
gap – gap between for coupler.
radius – for the bend and coupler.
length_x – ring coupler length.
length_y – vertical straight length.
coupler_ring – ring coupler spec.
bend – 90 degrees bend spec.
straight – straight spec.
coupler_ring – ring coupler spec.
cross_section – cross_section spec.
xxxxxxxxxxxxx xxxxx xxxx xxx xxx xxx xxx xx xxx x xxx xx xx▲ xx xx│length_y xx xx▼ xx xx xx length_x x xx ◄───────────────► x xx xxx xx xxx xxx──────▲─────────xxx │gap o1──────▼─────────o2
import cspdk
c = cspdk.si220.cells.ring_single_sc(gap=0.2, radius=10.0, length_x=4.0, length_y=0.6, bend='bend_euler_sc', straight='straight_sc', coupler_ring='coupler_ring_sc', cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
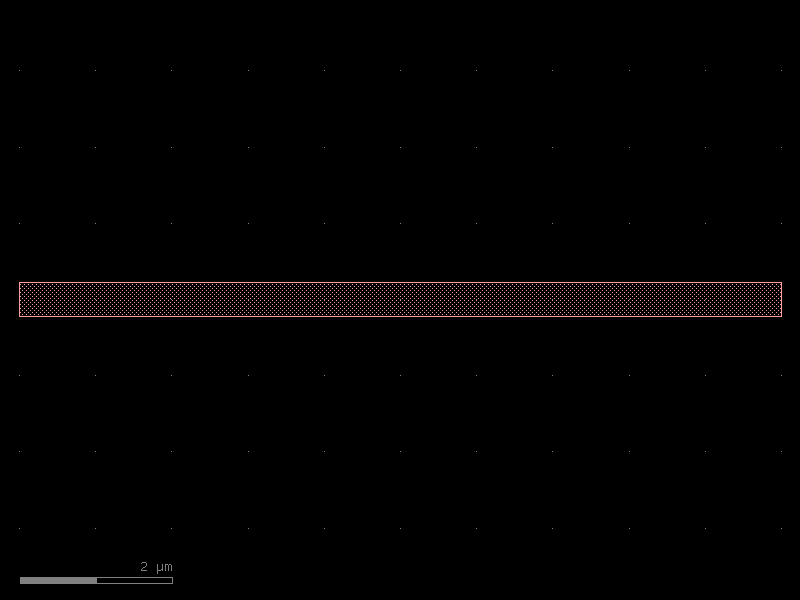
ring_single_so#
- cspdk.si220.cells.ring_single_so(gap: float = 0.2, radius: float = 10.0, length_x: float = 4.0, length_y: float = 0.6, *, bend: str | Callable[[...], Component] | dict[str, Any] | KCell = 'bend_euler_so', straight: str | Callable[[...], Component] | dict[str, Any] | KCell = 'straight_so', coupler_ring: str | Callable[[...], Component] | dict[str, Any] | KCell = 'coupler_ring_so', cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so') Component #
Returns a single ring.
ring coupler (cb: bottom) connects to two vertical straights (sl: left, sr: right), two bends (bl, br) and horizontal straight (wg: top)
- Parameters:
gap – gap between for coupler.
radius – for the bend and coupler.
length_x – ring coupler length.
length_y – vertical straight length.
coupler_ring – ring coupler spec.
bend – 90 degrees bend spec.
straight – straight spec.
coupler_ring – ring coupler spec.
cross_section – cross_section spec.
xxxxxxxxxxxxx xxxxx xxxx xxx xxx xxx xxx xx xxx x xxx xx xx▲ xx xx│length_y xx xx▼ xx xx xx length_x x xx ◄───────────────► x xx xxx xx xxx xxx──────▲─────────xxx │gap o1──────▼─────────o2
import cspdk
c = cspdk.si220.cells.ring_single_so(gap=0.2, radius=10.0, length_x=4.0, length_y=0.6, bend='bend_euler_so', straight='straight_so', coupler_ring='coupler_ring_so', cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
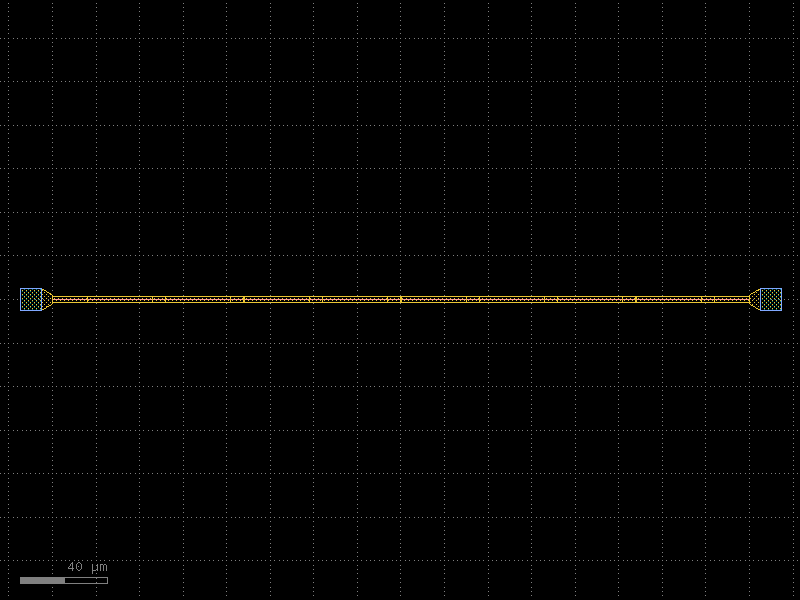
straight#
- cspdk.si220.cells.straight(length: float = 10.0, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', **kwargs) Component [source]#
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight(length=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
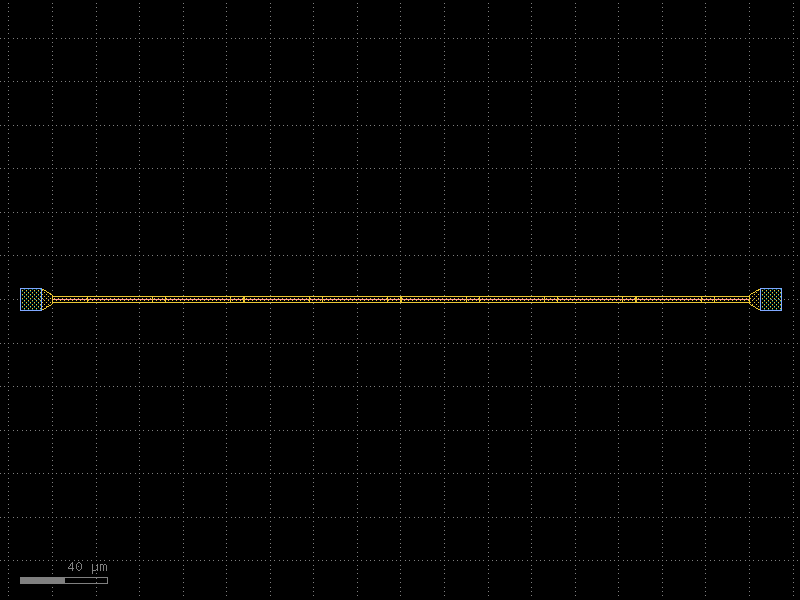
straight_heater_metal_sc#
- cspdk.si220.cells.straight_heater_metal_sc(length: float = 320.0, length_undercut_spacing: float = 6.0, length_undercut: float = 30.0, length_straight: float = 0.1, length_straight_input: float = 15.0, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', cross_section_heater: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'heater_metal', cross_section_waveguide_heater: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc_heater', cross_section_heater_undercut: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc_heater', with_undercut: bool = True, via_stack: str | Callable[[...], Component] | dict[str, Any] | KCell | None = 'via_stack_heater_mtop', port_orientation1: int | None = 180, port_orientation2: int | None = 0, heater_taper_length: float | None = 5.0, ohms_per_square: float | None = None) Component [source]#
Returns a thermal phase shifter.
dimensions from https://doi.org/10.1364/OE.27.010456
- Parameters:
length – of the waveguide.
length_undercut_spacing – from undercut regions.
length_undercut – length of each undercut section.
length_straight – length of the straight waveguide.
length_straight_input – from input port to where trenches start.
cross_section – for waveguide ports.
cross_section_heater – for heated sections. heater metal only.
cross_section_waveguide_heater – for heated sections.
cross_section_heater_undercut – for heated sections with undercut.
with_undercut – isolation trenches for higher efficiency.
via_stack – via stack.
port_orientation1 – left via stack port orientation.
port_orientation2 – right via stack port orientation.
heater_taper_length – minimizes current concentrations from heater to via_stack.
ohms_per_square – to calculate resistance.
import cspdk
c = cspdk.si220.cells.straight_heater_metal_sc(length=320.0, length_undercut_spacing=6.0, length_undercut=30.0, length_straight=0.1, length_straight_input=15.0, cross_section='xs_sc', cross_section_heater='heater_metal', cross_section_waveguide_heater='xs_sc_heater', cross_section_heater_undercut='xs_sc_heater', with_undercut=True, via_stack='via_stack_heater_mtop', port_orientation1=180, port_orientation2=0, heater_taper_length=5.0).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
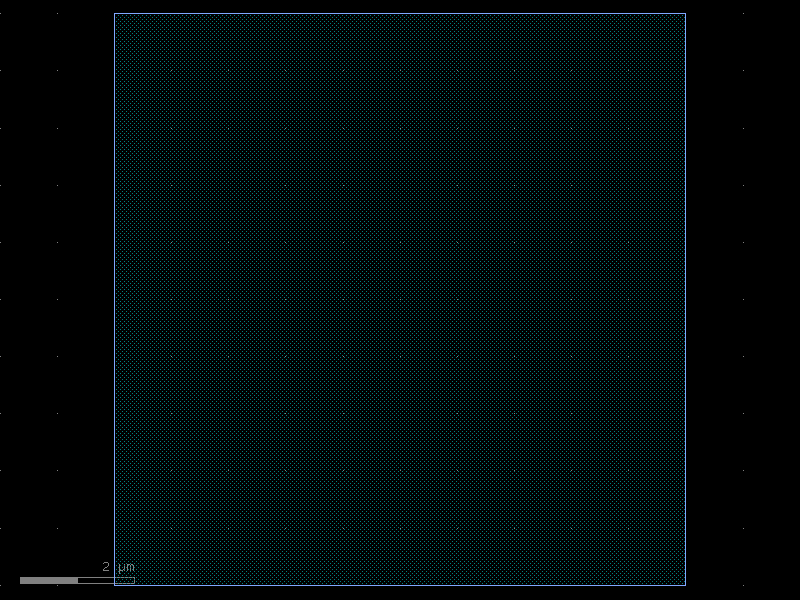
straight_heater_metal_so#
- cspdk.si220.cells.straight_heater_metal_so(length: float = 320.0, length_undercut_spacing: float = 6.0, length_undercut: float = 30.0, length_straight: float = 0.1, length_straight_input: float = 15.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', cross_section_heater: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'heater_metal', cross_section_waveguide_heater: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so_heater', cross_section_heater_undercut: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so_heater', with_undercut: bool = True, via_stack: str | Callable[[...], Component] | dict[str, Any] | KCell | None = 'via_stack_heater_mtop', port_orientation1: int | None = 180, port_orientation2: int | None = 0, heater_taper_length: float | None = 5.0, ohms_per_square: float | None = None) Component #
Returns a thermal phase shifter.
dimensions from https://doi.org/10.1364/OE.27.010456
- Parameters:
length – of the waveguide.
length_undercut_spacing – from undercut regions.
length_undercut – length of each undercut section.
length_straight – length of the straight waveguide.
length_straight_input – from input port to where trenches start.
cross_section – for waveguide ports.
cross_section_heater – for heated sections. heater metal only.
cross_section_waveguide_heater – for heated sections.
cross_section_heater_undercut – for heated sections with undercut.
with_undercut – isolation trenches for higher efficiency.
via_stack – via stack.
port_orientation1 – left via stack port orientation.
port_orientation2 – right via stack port orientation.
heater_taper_length – minimizes current concentrations from heater to via_stack.
ohms_per_square – to calculate resistance.
import cspdk
c = cspdk.si220.cells.straight_heater_metal_so(length=320.0, length_undercut_spacing=6.0, length_undercut=30.0, length_straight=0.1, length_straight_input=15.0, cross_section='xs_so', cross_section_heater='heater_metal', cross_section_waveguide_heater='xs_so_heater', cross_section_heater_undercut='xs_so_heater', with_undercut=True, via_stack='via_stack_heater_mtop', port_orientation1=180, port_orientation2=0, heater_taper_length=5.0).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
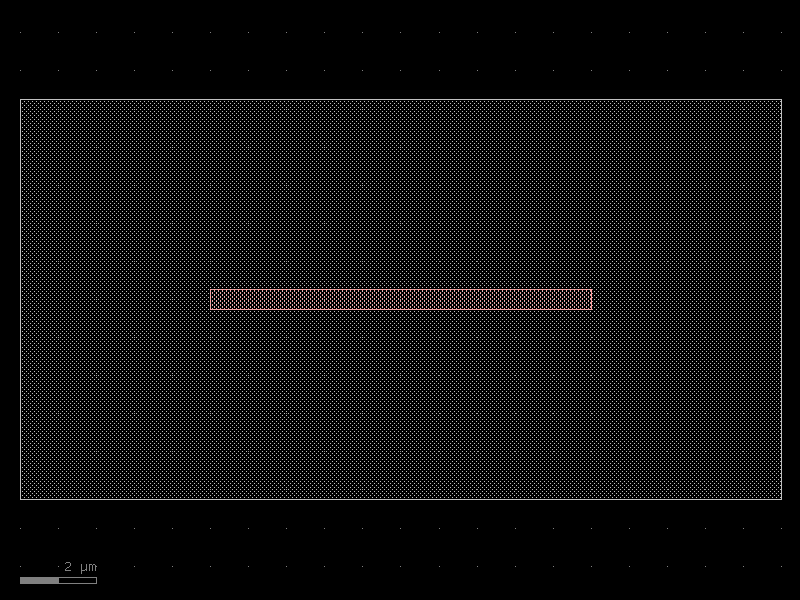
straight_metal#
- cspdk.si220.cells.straight_metal(length: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'metal_routing', **kwargs) Component #
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight_metal(length=10.0, cross_section='metal_routing').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
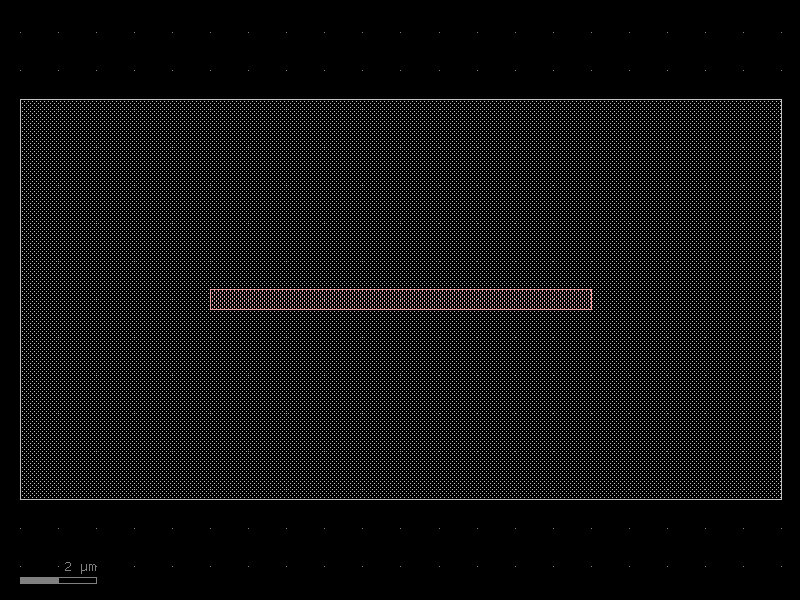
straight_rc#
- cspdk.si220.cells.straight_rc(length: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc', **kwargs) Component #
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight_rc(length=10.0, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
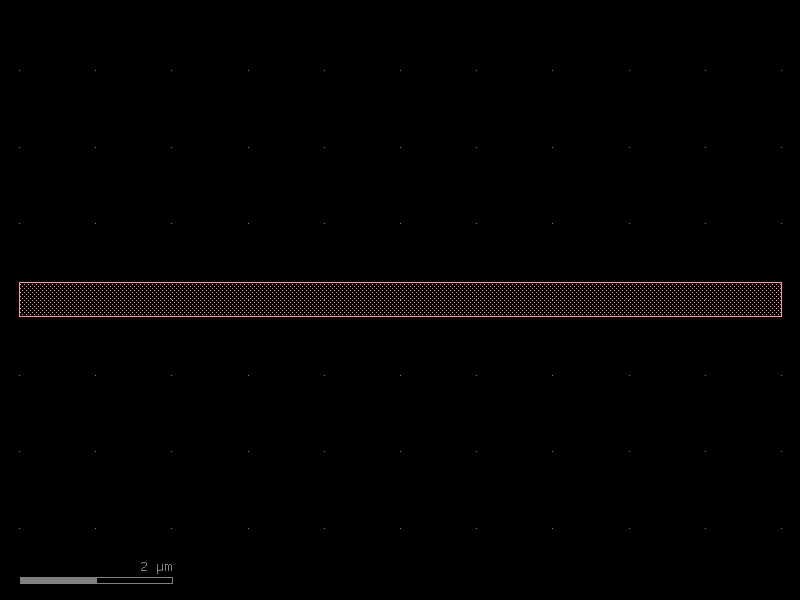
straight_ro#
- cspdk.si220.cells.straight_ro(length: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro', **kwargs) Component #
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight_ro(length=10.0, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
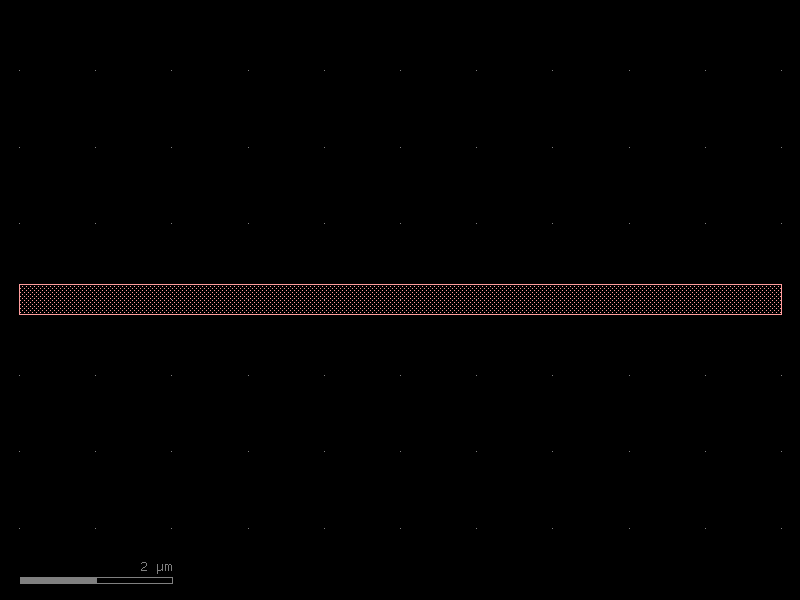
straight_sc#
- cspdk.si220.cells.straight_sc(length: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', **kwargs) Component #
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight_sc(length=10.0, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
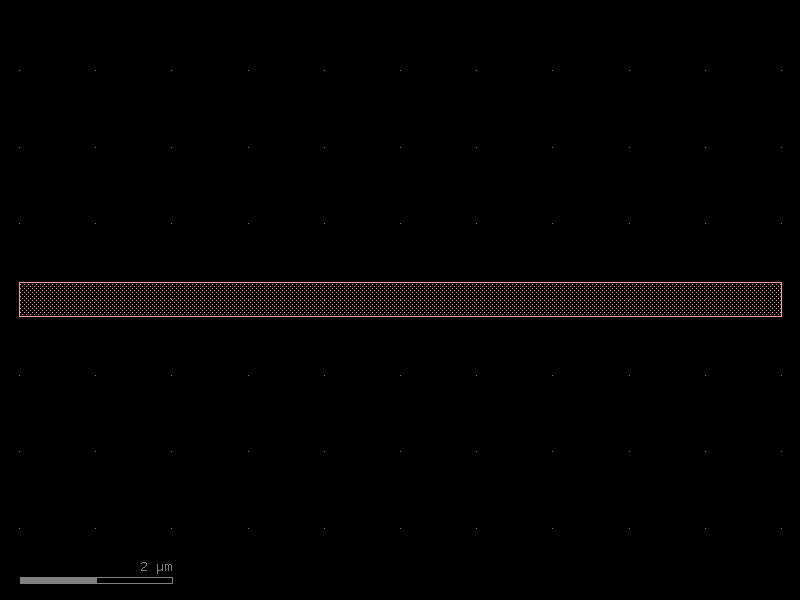
straight_so#
- cspdk.si220.cells.straight_so(length: float = 10.0, *, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', **kwargs) Component #
A straight waveguide.
- Parameters:
length – the length of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight_so(length=10.0, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
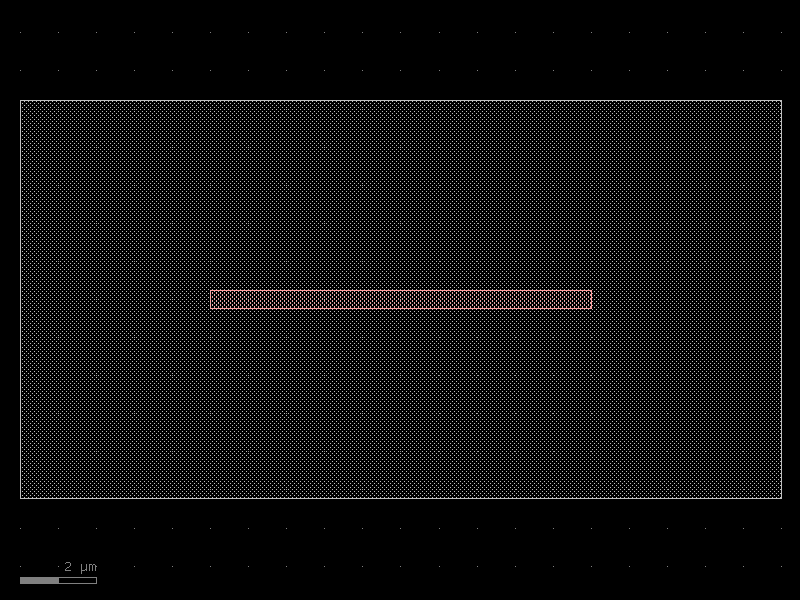
taper#
- cspdk.si220.cells.taper(length: float = 10.0, width1: float = 0.45, width2: float | None = None, port: Port | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', **kwargs) Component [source]#
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper(length=10.0, width1=0.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
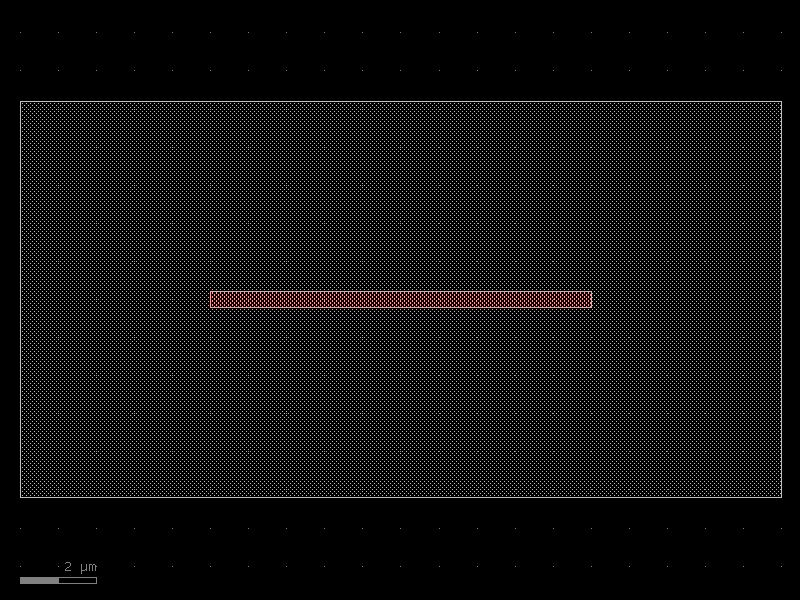
taper_rc#
- cspdk.si220.cells.taper_rc(*, length: float = 10.0, width1: float = 0.5, width2: float | None = None, port: Port | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_rc', **kwargs) Component #
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper_rc(length=10.0, width1=0.5, cross_section='xs_rc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
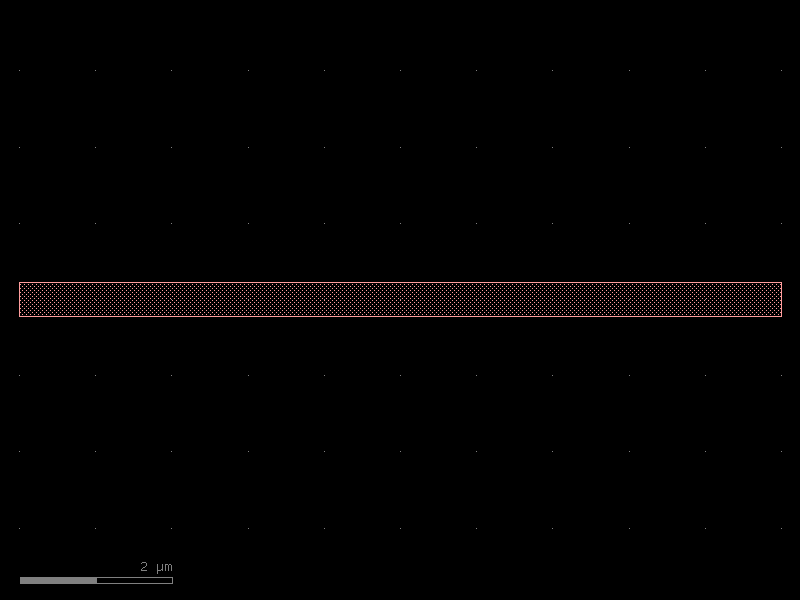
taper_ro#
- cspdk.si220.cells.taper_ro(*, length: float = 10.0, width1: float = 0.5, width2: float | None = None, port: Port | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_ro', **kwargs) Component #
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper_ro(length=10.0, width1=0.5, cross_section='xs_ro').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
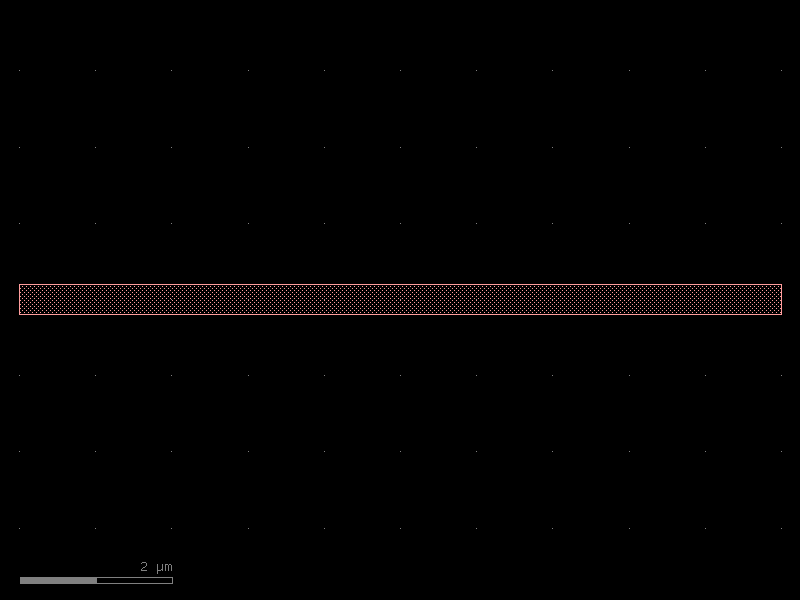
taper_sc#
- cspdk.si220.cells.taper_sc(*, length: float = 10.0, width1: float = 0.45, width2: float | None = None, port: Port | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc', **kwargs) Component #
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper_sc(length=10.0, width1=0.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
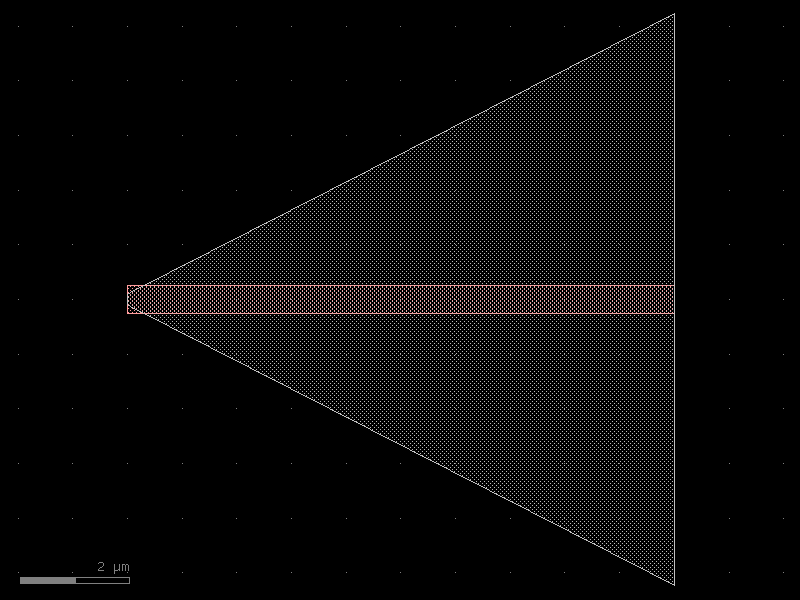
taper_so#
- cspdk.si220.cells.taper_so(*, length: float = 10.0, width1: float = 0.4, width2: float | None = None, port: Port | None = None, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_so', **kwargs) Component #
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper_so(length=10.0, width1=0.4, cross_section='xs_so').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
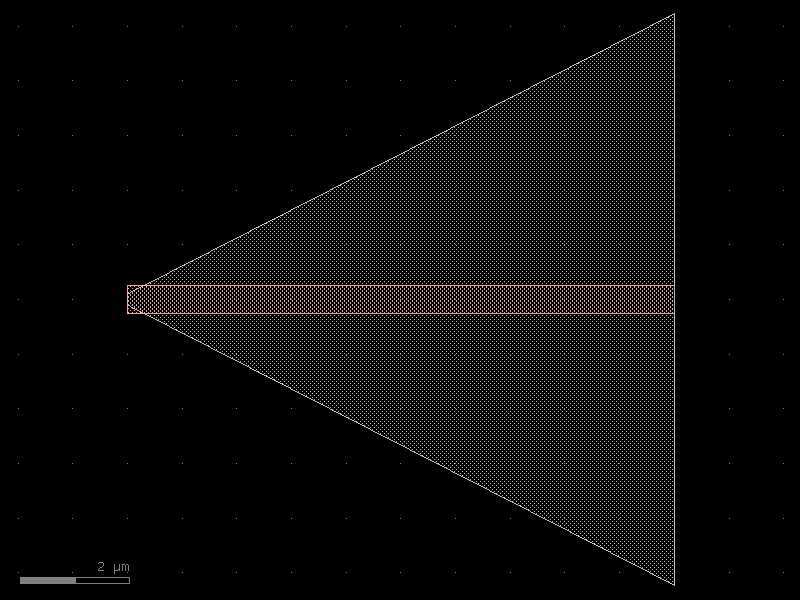
taper_strip_to_ridge#
- cspdk.si220.cells.taper_strip_to_ridge(length: float = 10.0, width1: float = 0.5, width2: float = 0.5, w_slab1: float = 0.2, w_slab2: float = 10.45, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component [source]#
A taper between strip and ridge.
This is a transition between two distinct cross sections
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper.
w_slab1 – the input slab width of the taper.
w_slab2 – the output slab width of the taper.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.taper_strip_to_ridge(length=10.0, width1=0.5, width2=0.5, w_slab1=0.2, w_slab2=10.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
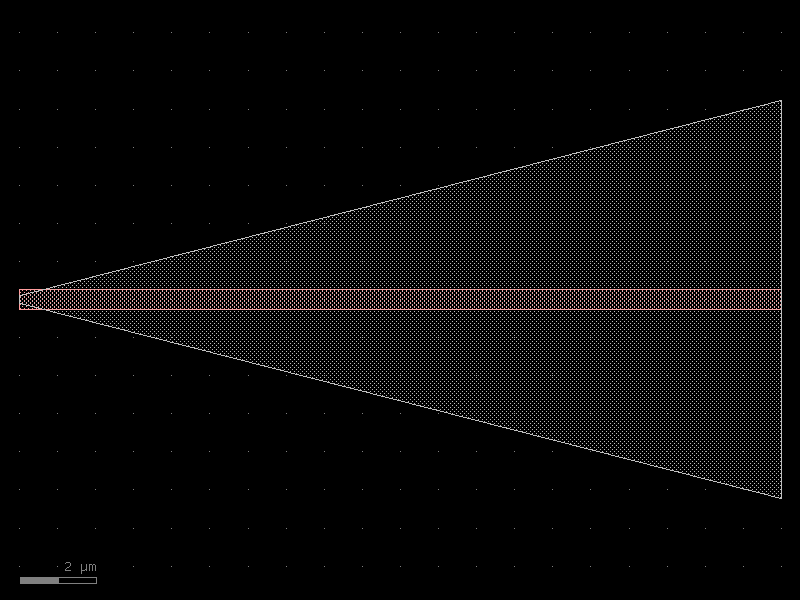
trans_sc_rc10#
- cspdk.si220.cells.trans_sc_rc10(*, length: float = 10, width1: float = 0.5, width2: float = 0.5, w_slab1: float = 0.2, w_slab2: float = 10.45, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
A taper between strip and ridge.
This is a transition between two distinct cross sections
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper.
w_slab1 – the input slab width of the taper.
w_slab2 – the output slab width of the taper.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.trans_sc_rc10(length=10, width1=0.5, width2=0.5, w_slab1=0.2, w_slab2=10.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
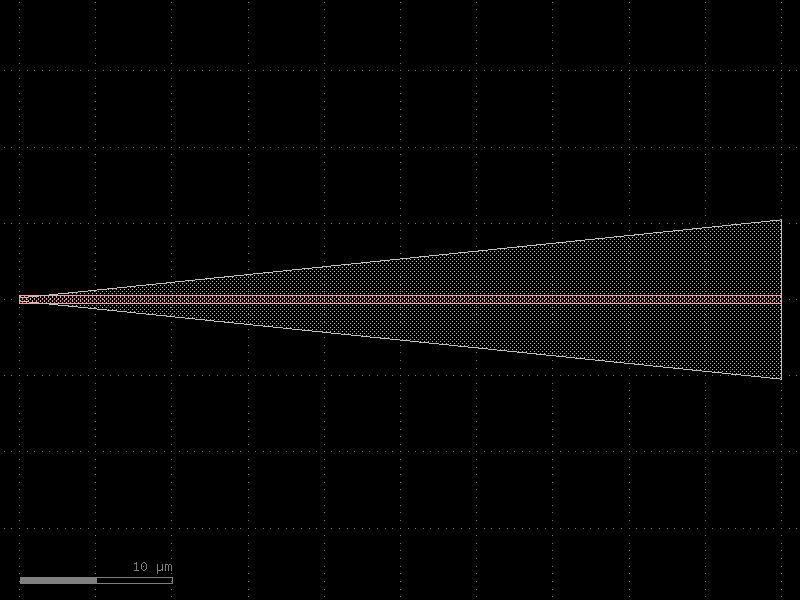
trans_sc_rc20#
- cspdk.si220.cells.trans_sc_rc20(*, length: float = 20, width1: float = 0.5, width2: float = 0.5, w_slab1: float = 0.2, w_slab2: float = 10.45, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
A taper between strip and ridge.
This is a transition between two distinct cross sections
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper.
w_slab1 – the input slab width of the taper.
w_slab2 – the output slab width of the taper.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.trans_sc_rc20(length=20, width1=0.5, width2=0.5, w_slab1=0.2, w_slab2=10.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
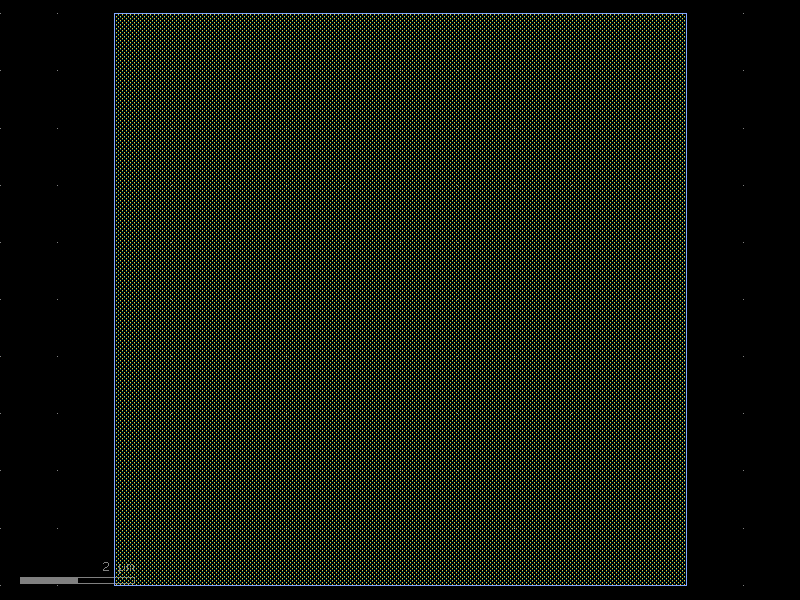
trans_sc_rc50#
- cspdk.si220.cells.trans_sc_rc50(*, length: float = 50, width1: float = 0.5, width2: float = 0.5, w_slab1: float = 0.2, w_slab2: float = 10.45, cross_section: CrossSection | str | dict[str, Any] | Callable[[...], CrossSection] | SymmetricalCrossSection = 'xs_sc') Component #
A taper between strip and ridge.
This is a transition between two distinct cross sections
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper.
w_slab1 – the input slab width of the taper.
w_slab2 – the output slab width of the taper.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.trans_sc_rc50(length=50, width1=0.5, width2=0.5, w_slab1=0.2, w_slab2=10.45, cross_section='xs_sc').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
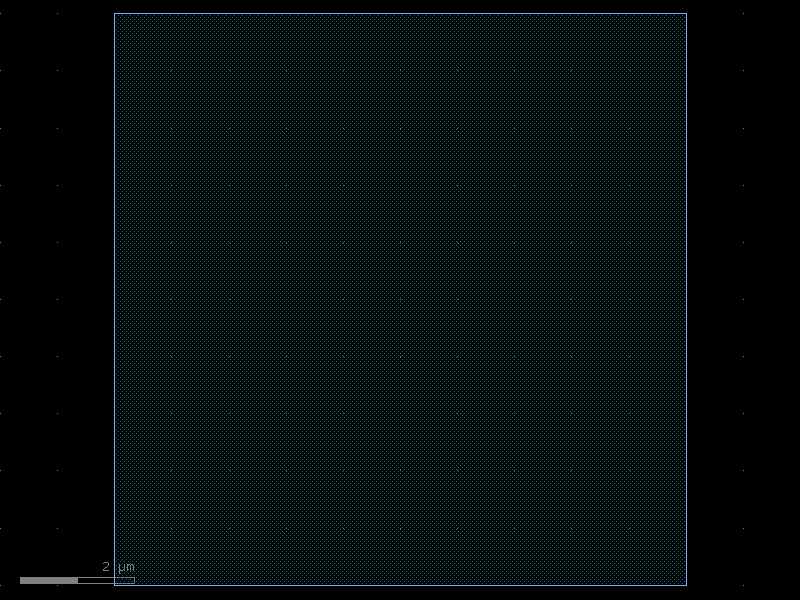
via_stack_heater_mtop#
- cspdk.si220.cells.via_stack_heater_mtop(size=(10, 10)) Component [source]#
Returns a via stack for the heater.
import cspdk
c = cspdk.si220.cells.via_stack_heater_mtop(size=(10, 10)).dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
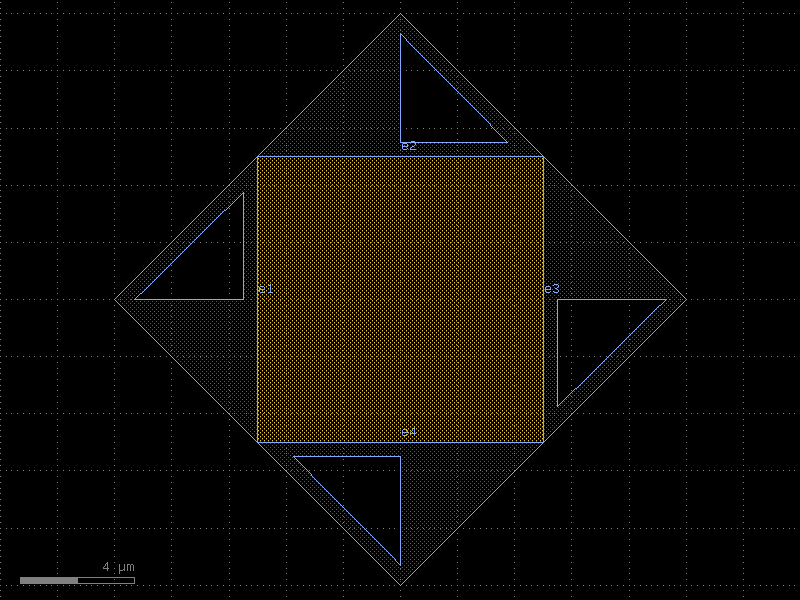
wire_corner#
- cspdk.si220.cells.wire_corner(cross_section='metal_routing', **kwargs) Component [source]#
A wire corner.
A wire corner is a bend for electrical routes.
import cspdk
c = cspdk.si220.cells.wire_corner(cross_section='metal_routing').dup()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
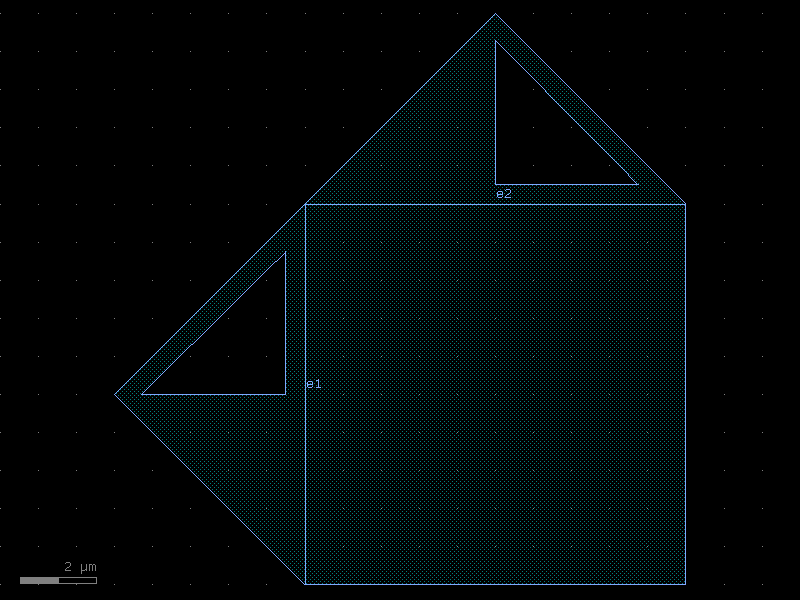