Cells SiN300#
array#
- cspdk.si220.cells.array(component='pad', spacing: tuple[float, float] = (150.0, 150.0), columns: int = 6, rows: int = 1, add_ports: bool = True, size=None, centered: bool = False) Component [source]#
An array of components.
- Parameters:
component – the component of which to create an array
spacing – the x and y spacing by which to place the component
columns – the number of components to place in the x-direction
rows – the number of components to place in the y-direction
add_ports – add ports to the component
size – Optional x, y size. Overrides columns and rows.
centered – center the array around the origin.
import cspdk
c = cspdk.si220.cells.array(component='pad', spacing=(150.0, 150.0), columns=6, rows=1, add_ports=True, centered=False)
c.plot()
(Source code
, png
, hires.png
, pdf
)
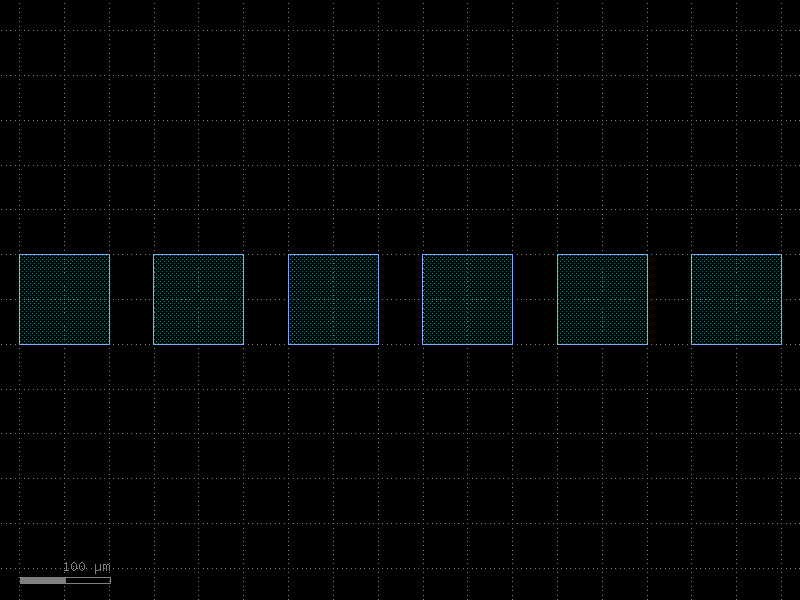
bend_euler#
import cspdk
c = cspdk.si220.cells.bend_euler(angle=90.0, p=0.5, cross_section='xs_nc')
c.plot()
bend_euler_nc#
import cspdk
c = cspdk.si220.cells.bend_euler_nc(angle=90.0, p=0.5, cross_section='xs_nc')
c.plot()
bend_euler_no#
import cspdk
c = cspdk.si220.cells.bend_euler_no(angle=90.0, p=0.5, cross_section='xs_no')
c.plot()
bend_s#
- cspdk.si220.cells.bend_s(size: tuple[float, float] = (11.0, 1.8), cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc', **kwargs) Component [source]#
An S-bend.
- Parameters:
size – the width and height of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the bend_s function.
import cspdk
c = cspdk.si220.cells.bend_s(size=(15.0, 1.8), cross_section='xs_nc')
c.plot()
coupler#
- cspdk.si220.cells.coupler(gap: float = 0.234, length: float = 20.0, dy: float = 4.0, dx: float = 10.0, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
A coupler.
a coupler is a 2x2 splitter
- Parameters:
gap – the gap between the waveguides forming the straight part of the coupler
length – the length of the coupler
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler(gap=0.236, length=20.0, dy=4.0, dx=20.0, cross_section='xs_nc')
c.plot()
coupler_nc#
import cspdk
c = cspdk.si220.cells.coupler_nc(gap=0.236, length=20.0, dy=4.0, dx=20.0, cross_section='xs_nc')
c.plot()
coupler_no#
import cspdk
c = cspdk.si220.cells.coupler_no(gap=0.236, length=20.0, dy=4.0, dx=20.0, cross_section='xs_no')
c.plot()
coupler_straight#
- cspdk.si220.cells.coupler_straight(length: float = 20.0, gap: float = 0.27, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
The straight part of a coupler.
- Parameters:
length – the length of the straight part of the coupler
gap – the gap between the waveguides forming the straight part of the coupler
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_straight(length=20.0, gap=0.236, cross_section='xs_nc')
c.plot()
coupler_symmetric#
- cspdk.si220.cells.coupler_symmetric(gap: float = 0.234, dy: float = 4.0, dx: float = 10.0, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
The part of the coupler that diverges away from each other with s-bends.
- Parameters:
gap – the gap between the s-bends when closest together
dy – the height of the s-bend
dx – the length of the s-bend
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.coupler_symmetric(gap=0.236, dy=4.0, dx=20.0, cross_section='xs_nc')
c.plot()
die#
- cspdk.si220.cells.die(cross_section='xs_sc') Component [source]#
A die template.
- Parameters:
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.die(cross_section='xs_nc')
c.plot()
die_nc#
import cspdk
c = cspdk.si220.cells.die_nc(cross_section='xs_nc')
c.plot()
die_no#
import cspdk
c = cspdk.si220.cells.die_no(cross_section='xs_no')
c.plot()
grating_coupler_array#
- cspdk.si220.cells.grating_coupler_array(pitch: float = 127.0, n: int = 6, centered=True, grating_coupler=None, port_name='o1', with_loopback=False, rotation=-90, straight_to_grating_spacing=10.0, cross_section='xs_sc') Component [source]#
An array of grating couplers.
- Parameters:
pitch – the pitch of the grating couplers
n – the number of grating couplers
centered – if True, centers the array around the origin.
grating_coupler – the name of the grating coupler to use in the array.
port_name – port name
with_loopback – if True, adds a loopback between edge GCs. Only works for rotation = 90 for now.
rotation – rotation angle for each reference.
straight_to_grating_spacing – spacing between the last grating coupler and the loopback.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_array(pitch=127.0, n=6, cross_section='xs_nc', centered=True, port_name='o1', rotation=-90, straight_to_grating_spacing=10.0, with_loopback=False)
c.plot()
(Source code
, png
, hires.png
, pdf
)
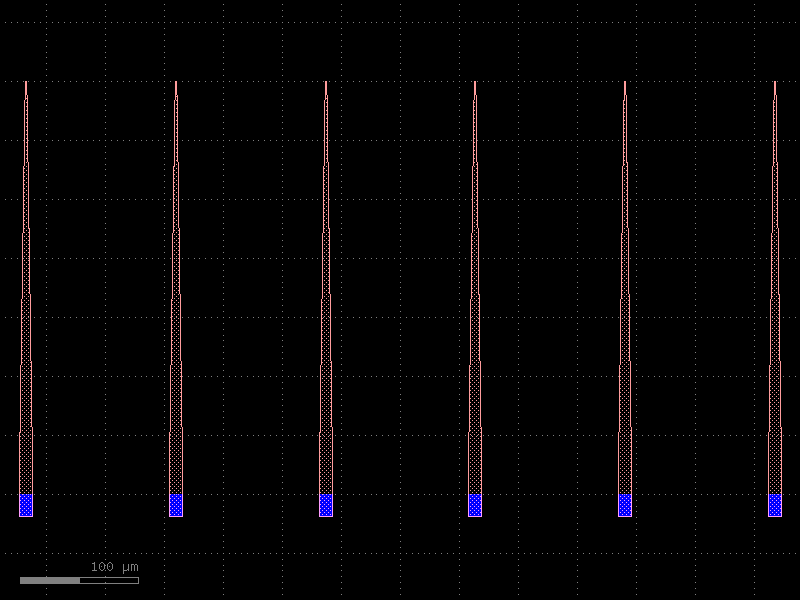
grating_coupler_elliptical#
- cspdk.si220.cells.grating_coupler_elliptical(wavelength: float = 1.55, grating_line_width=0.315, cross_section='xs_sc') Component [source]#
A grating coupler with curved but parallel teeth.
- Parameters:
wavelength – the center wavelength for which the grating is designed
grating_line_width – the line width of the grating
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical(wavelength=1.55, grating_line_width=0.343, cross_section='xs_nc')
c.plot()
grating_coupler_elliptical_nc#
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical_nc(wavelength=1.55, grating_line_width=0.33, cross_section='xs_nc')
c.plot()
grating_coupler_elliptical_no#
import cspdk
c = cspdk.si220.cells.grating_coupler_elliptical_no(wavelength=1.31, grating_line_width=0.482, cross_section='xs_no')
c.plot()
grating_coupler_rectangular#
- cspdk.si220.cells.grating_coupler_rectangular(period=0.63, n_periods: int = 30, length_taper: float = 350.0, wavelength: float = 1.55, cross_section='xs_sc') Component [source]#
A grating coupler with straight and parallel teeth.
- Parameters:
period – the period of the grating
n_periods – the number of grating teeth
length_taper – the length of the taper tapering up to the grating
wavelength – the center wavelength for which the grating is designed
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular(period=0.66, n_periods=30, length_taper=200.0, wavelength=1.55, cross_section='xs_nc')
c.plot()
grating_coupler_rectangular_nc#
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_nc(period=0.66, n_periods=30, length_taper=200.0, wavelength=1.55, cross_section='xs_nc')
c.plot()
grating_coupler_rectangular_no#
import cspdk
c = cspdk.si220.cells.grating_coupler_rectangular_no(period=0.964, n_periods=30, length_taper=200.0, wavelength=1.31, cross_section='xs_no')
c.plot()
mmi1x2#
- cspdk.si220.cells.mmi1x2(width: float | None = None, width_taper=1.5, length_taper=20.0, length_mmi: float = 5.5, width_mmi=6.0, gap_mmi: float = 0.25, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
An mmi1x2.
An mmi1x2 is a splitter that splits a single input to two outputs
- Parameters:
width – the width of the waveguides connecting at the mmi ports.
width_taper – the width at the base of the mmi body.
length_taper – the length of the tapers going towards the mmi body.
length_mmi – the length of the mmi body.
width_mmi – the width of the mmi body.
gap_mmi – the gap between the tapers at the mmi body.
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi1x2(width_mmi=12.0, width_taper=5.5, length_taper=50.0, length_mmi=64.7, gap_mmi=0.4, cross_section='xs_nc')
c.plot()
mmi1x2_nc#
import cspdk
c = cspdk.si220.cells.mmi1x2_nc(width_mmi=12.0, width_taper=5.5, length_taper=50.0, length_mmi=64.7, gap_mmi=0.4, cross_section='xs_nc')
c.plot()
mmi1x2_no#
import cspdk
c = cspdk.si220.cells.mmi1x2_no(width_mmi=12.0, width_taper=5.5, length_taper=50.0, length_mmi=42.0, gap_mmi=0.4, cross_section='xs_no')
c.plot()
mmi2x2#
- cspdk.si220.cells.mmi2x2(width: float | None = None, width_taper: float = 1.5, length_taper: float = 20.0, length_mmi: float = 5.5, width_mmi: float = 6.0, gap_mmi: float = 0.25, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
An mmi2x2.
An mmi2x2 is a 2x2 splitter
- Parameters:
width – the width of the waveguides connecting at the mmi ports
width_taper – the width at the base of the mmi body
length_taper – the length of the tapers going towards the mmi body
length_mmi – the length of the mmi body
width_mmi – the width of the mmi body
gap_mmi – the gap between the tapers at the mmi body
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mmi2x2(width_taper=5.5, length_taper=50.0, length_mmi=5.5, width_mmi=12.0, gap_mmi=0.4, cross_section='xs_nc')
c.plot()
mmi2x2_nc#
import cspdk
c = cspdk.si220.cells.mmi2x2_nc(width_taper=5.5, length_taper=50.0, length_mmi=232.0, width_mmi=12.0, gap_mmi=0.4, cross_section='xs_nc')
c.plot()
mmi2x2_no#
import cspdk
c = cspdk.si220.cells.mmi2x2_no(width_taper=5.5, length_taper=50.0, length_mmi=126.0, width_mmi=12.0, gap_mmi=0.4, cross_section='xs_no')
c.plot()
mzi#
- cspdk.si220.cells.mzi(delta_length: float = 10.0, bend='bend_euler_sc', straight='straight_sc', splitter='mmi1x2_sc', combiner='mmi2x2_sc', cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc') Component [source]#
A Mach-Zehnder Interferometer.
- Parameters:
delta_length – the difference in length between the upper and lower arms of the mzi
bend – the name of the default bend of the mzi
straight – the name of the default straight of the mzi
splitter – the name of the default splitter of the mzi
combiner – the name of the default combiner of the mzi
cross_section – a cross section or its name or a function generating a cross section.
import cspdk
c = cspdk.si220.cells.mzi(delta_length=10.0, bend='bend_euler_nc', straight='straight_nc', splitter='mmi1x2_nc', combiner='mmi2x2_nc', cross_section='xs_nc')
c.plot()
mzi_nc#
import cspdk
c = cspdk.si220.cells.mzi_nc(delta_length=10.0, bend='bend_euler_nc', straight='straight_nc', splitter='mmi1x2_nc', combiner='mmi2x2_nc', cross_section='xs_nc')
c.plot()
mzi_no#
import cspdk
c = cspdk.si220.cells.mzi_no(delta_length=10.0, bend='bend_euler_no', straight='straight_no', splitter='mmi1x2_no', combiner='mmi2x2_no', cross_section='xs_no')
c.plot()
pad#
import cspdk
c = cspdk.si220.cells.pad()
c.plot()
(Source code
, png
, hires.png
, pdf
)
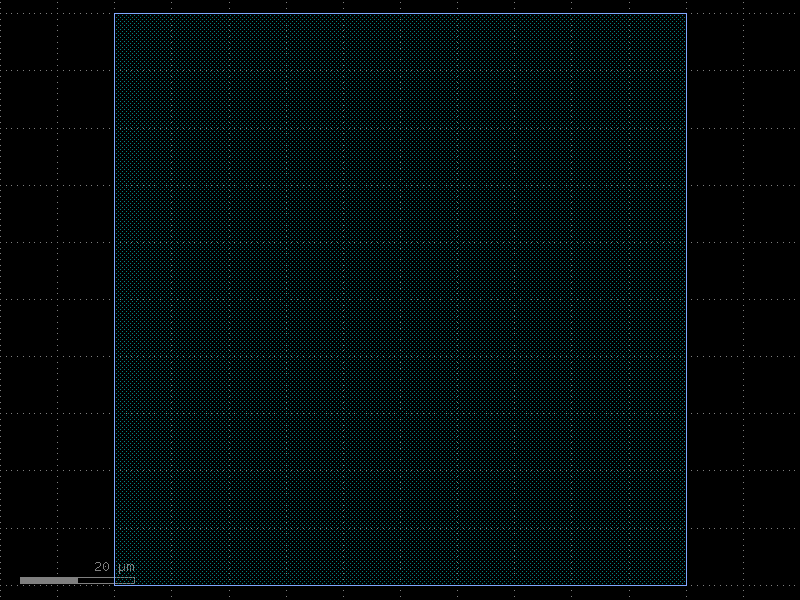
rectangle#
import cspdk
c = cspdk.si220.cells.rectangle()
c.plot()
(Source code
, png
, hires.png
, pdf
)
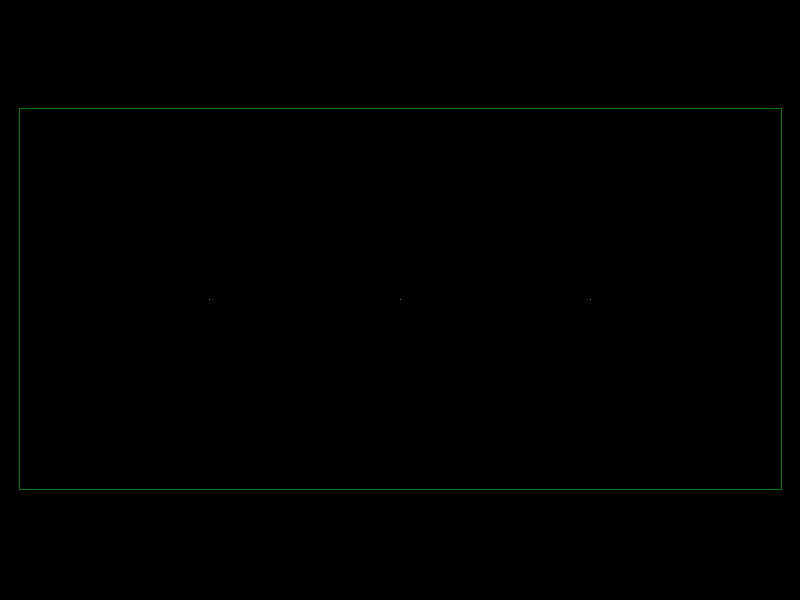
straight#
- cspdk.si220.cells.straight(length: float = 10.0, width: float | None = None, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc', **kwargs) Component [source]#
A straight waveguide.
- Parameters:
length – the length of the waveguide.
width – the width of the waveguide.
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the straight function.
import cspdk
c = cspdk.si220.cells.straight(length=10.0, cross_section='xs_nc')
c.plot()
straight_nc#
import cspdk
c = cspdk.si220.cells.straight_nc(length=10.0, cross_section='xs_nc')
c.plot()
straight_no#
import cspdk
c = cspdk.si220.cells.straight_no(length=10.0, cross_section='xs_no')
c.plot()
taper#
- cspdk.si220.cells.taper(length: float = 10.0, width1: float = 0.45, width2: float | None = None, port: Port | None = None, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_sc', **kwargs) Component [source]#
A taper.
A taper is a transition between two waveguide widths
- Parameters:
length – the length of the taper.
width1 – the input width of the taper.
width2 – the output width of the taper (if not given, use port).
port – the port (with certain width) to taper towards (if not given, use width2).
cross_section – a cross section or its name or a function generating a cross section.
kwargs – additional arguments to pass to the taper function.
import cspdk
c = cspdk.si220.cells.taper(length=10.0, width1=1.2, cross_section='xs_nc')
c.plot()
taper_nc#
import cspdk
c = cspdk.si220.cells.taper_nc(length=10.0, width1=1.2, cross_section='xs_nc')
c.plot()
taper_no#
import cspdk
c = cspdk.si220.cells.taper_no(length=10.0, width1=0.95, cross_section='xs_no')
c.plot()
wire_corner#
- cspdk.si220.cells.wire_corner() Component [source]#
A wire corner.
A wire corner is a bend for electrical routes.
import cspdk
c = cspdk.si220.cells.wire_corner()
c.plot()
(Source code
, png
, hires.png
, pdf
)
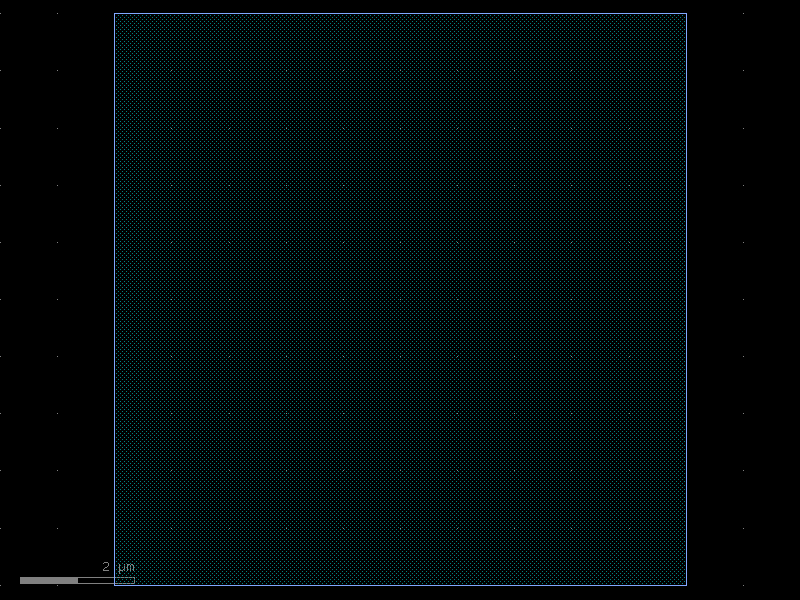