Here are the components available in the PDK
Cells#
bend_euler#
- gvtt.components.bend_euler(angle: float = 90.0, p: float = 1.0, with_arc_floorplan: bool = False, npoints: int | None = None, direction: str = 'ccw', with_bbox: bool = True, cross_section: CrossSectionSpec = functools.partial(<function strip>, width=1.874), **kwargs: Any) Component [source]#
Returns an euler bend that transitions from straight to curved.
By default, radius corresponds to the minimum radius of curvature of the bend. However, if with_arc_floorplan is True, radius corresponds to the effective radius of curvature (making the curve a drop-in replacement for an arc). If p < 1.0, will create a “partial euler” curve as described in Vogelbacher et. al. https://dx.doi.org/10.1364/oe.27.031394
default p = 0.5 based on this paper https://www.osapublishing.org/oe/fulltext.cfm?uri=oe-25-8-9150&id=362937
- Parameters:
angle – total angle of the curve.
p – Proportion of the curve that is an Euler curve.
with_arc_floorplan – If False: radius is the minimum radius of curvature If True: The curve scales such that the endpoints match a bend_circular with parameters radius and angle.
npoints – Number of points used per 360 degrees.
direction – cw (clock-wise) or ccw (counter clock-wise).
with_bbox – add bbox_layers and bbox_offsets to avoid DRC sharp edges.
cross_section – specification (CrossSection, string, CrossSectionFactory dict).
kwargs – cross_section settings.
o2 | / / / o1_____/
import gvtt
c = gvtt.components.bend_euler(angle=90.0, p=1.0, with_arc_floorplan=False, direction='ccw', with_bbox=True)
c.plot()
(Source code
, png
, hires.png
, pdf
)
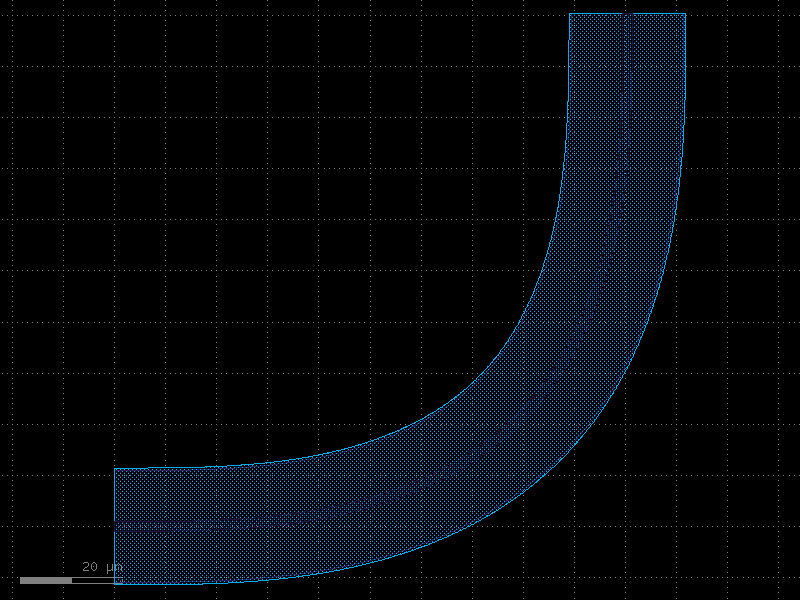
die#
- gvtt.components.die(size: tuple[float, float] = (5000.0, 9500.0), street_width: float = 75.0, street_length: float = 1000.0, die_name: str | None = 'chip99', text_size: float = 100.0, text_location: str | tuple[float, float] = 'SW', layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum | None = None, bbox_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum | None = None, draw_corners: bool = True, draw_dicing_lane: bool = True, text_component: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.DKCell = <function text>) Component [source]#
import gvtt
c = gvtt.components.die(size=(5000.0, 9500.0), street_width=75.0, street_length=1000.0, die_name='chip99', text_size=100.0, text_location='SW', draw_corners=True, draw_dicing_lane=True)
c.plot()
(Source code
, png
, hires.png
, pdf
)
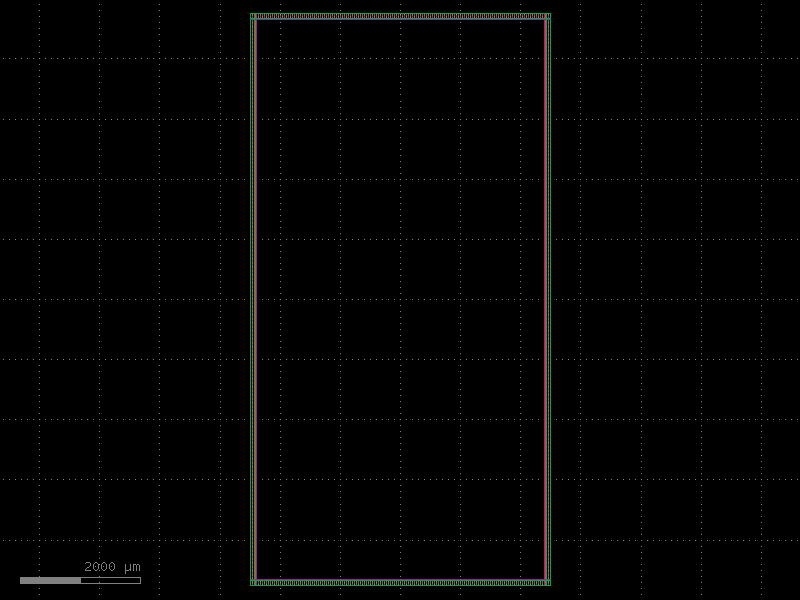
edge_coupler_rib#
- gvtt.components.edge_coupler_rib(edge_coupling_width: float = 3.0, polishing_length: float = 25.0, side: str = 'W', xpos: float = 0.0, ypos: float = 0.0) Component [source]#
Returns a rib waveguide edge coupler.
- Parameters:
edge_coupling_width – width of the edge coupling waveguide.
polishing_length – length of the edge coupling waveguide.
side – side of the edge coupler (W, E, N, S).
xpos – dx position of the edge coupler.
ypos – dy position of the edge coupler.
import gvtt
c = gvtt.components.edge_coupler_rib(edge_coupling_width=3.0, polishing_length=25.0, side='W', xpos=0.0, ypos=0.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
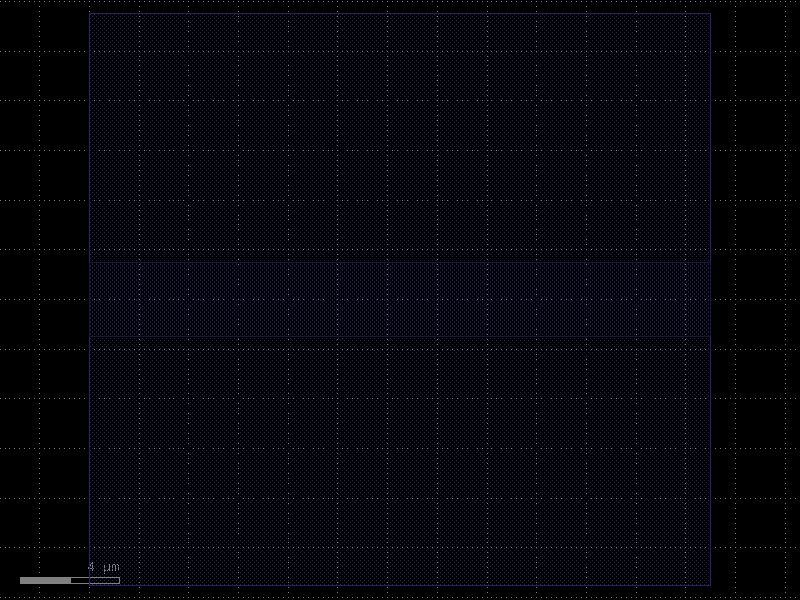
mmi1x2#
- gvtt.components.mmi1x2(width: float = 1.874, width_taper: float = 1.874, length_taper: float = 1.0, length_mmi: float = 43.25, width_mmi: float = 6.25, gap_mmi: float = 1.251, taper: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.DKCell = <function taper>, straight: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.DKCell = <function straight>, cross_section: ~gdsfactory.cross_section.CrossSection | str | dict[str, ~typing.Any] | ~collections.abc.Callable[[...], CrossSection] | ~kfactory.cross_section.SymmetricalCrossSection | ~kfactory.cross_section.DCrossSection = 'xs_sc') Component [source]#
1x2 MultiMode Interferometer (MMI).
- Parameters:
width – input and output straight width. Defaults to cross_section width.
width_taper – interface between input straights and mmi region.
length_taper – into the mmi region.
length_mmi – in x direction.
width_mmi – in y direction.
gap_mmi – gap between tapered wg.
taper – taper function.
straight – straight function.
cross_section – specification (CrossSection, string or dict).
length_mmi <------> ________ | | | \__ | __ o2 __/ /_ _ _ _ o1 __ | _ _ _ _| gap_mmi \ \__ | __ o3 | / |________| <-> length_taper
import gvtt
c = gvtt.components.mmi1x2(width=1.874, width_taper=1.874, length_taper=1.0, length_mmi=43.25, width_mmi=6.25, gap_mmi=1.251, cross_section='xs_sc')
c.plot()
(Source code
, png
, hires.png
, pdf
)
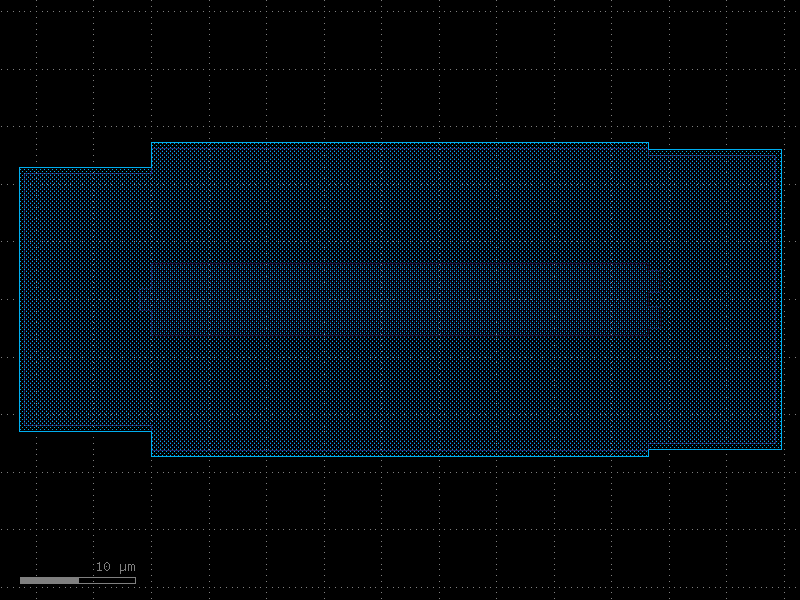
mmi2x2#
- gvtt.components.mmi2x2(width: float | None = None, width_taper: float = 1.874, length_taper: float = 1.0, length_mmi: float = 112.0, width_mmi: float = 5, gap_mmi: float = 1.25, taper: ComponentSpec = <function taper>, straight: ComponentSpec = <function straight>, cross_section: CrossSectionSpec = 'xs_sc') Component [source]#
Mmi 2x2.
- Parameters:
width – input and output straight width.
width_taper – interface between input straights and mmi region.
length_taper – into the mmi region.
length_mmi – in x direction.
width_mmi – in y direction.
gap_mmi – (width_taper + gap between tapered wg)/2.
taper – taper function.
straight – straight function.
cross_section – spec.
length_mmi <------> ________ | | __/ \__ o2 __ __ o3 \ /_ _ _ _ | | _ _ _ _| gap_mmi __/ \__ o1 __ __ o4 \ / |________| <-> length_taper
import gvtt
c = gvtt.components.mmi2x2(width_taper=1.874, length_taper=1.0, length_mmi=112.0, width_mmi=5, gap_mmi=1.25, cross_section='xs_sc')
c.plot()
(Source code
, png
, hires.png
, pdf
)
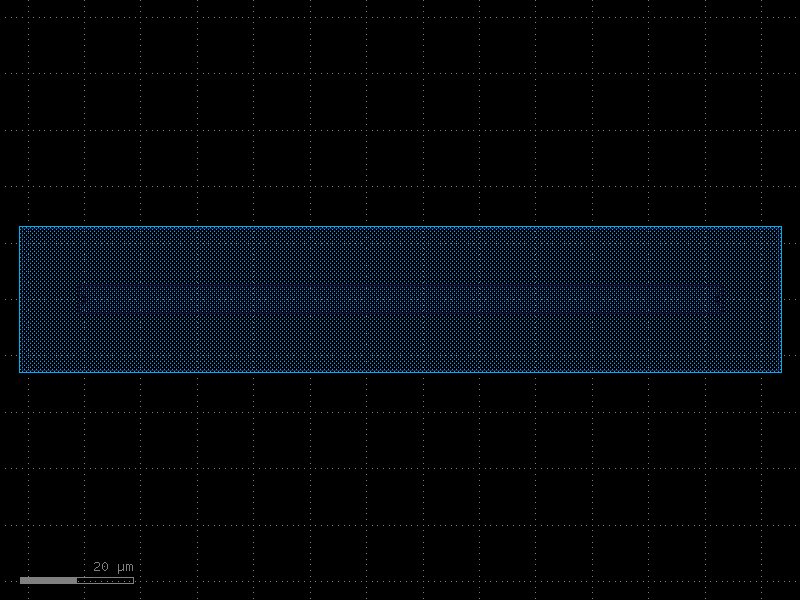
rib_taper#
- gvtt.components.rib_taper(width1: float = 1, width2: float = 1, taper_ratio: float = 50.0, length: float | None = None, **kwargs: Any) Component [source]#
Standard rib-to-strip waveguide converter.
- Parameters:
width1 – initial width.
width2 – final width.
taper_ratio – taper ratio.
length – taper length.
**kwargs – additional arguments.
import gvtt
c = gvtt.components.rib_taper(width1=1, width2=1, taper_ratio=50.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
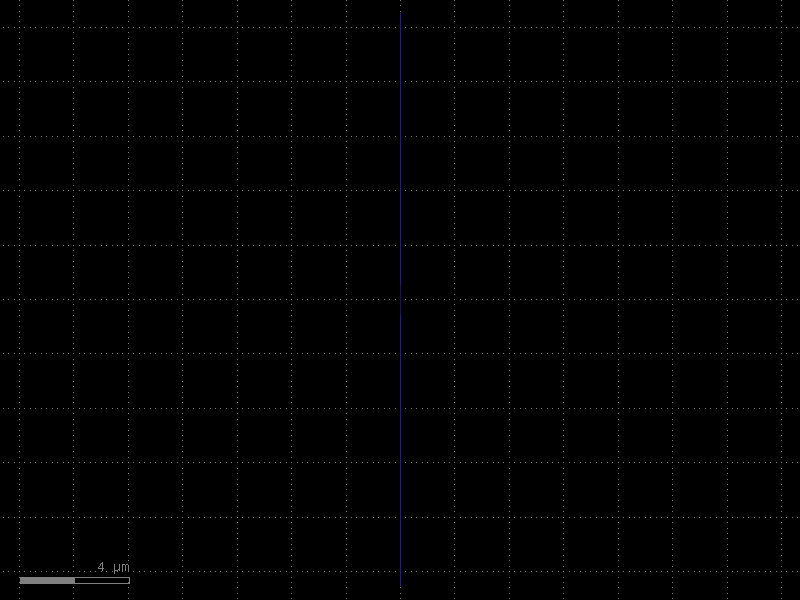
rib_to_strip#
- gvtt.components.rib_to_strip(length: float = 200.0, width1: float = 3.0, width2: float = 3.0) Component [source]#
Standard rib-to-strip waveguide converter.
- Parameters:
length – taper length.
width1 – initial width.
width2 – final width.
import gvtt
c = gvtt.components.rib_to_strip(length=200.0, width1=3.0, width2=3.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
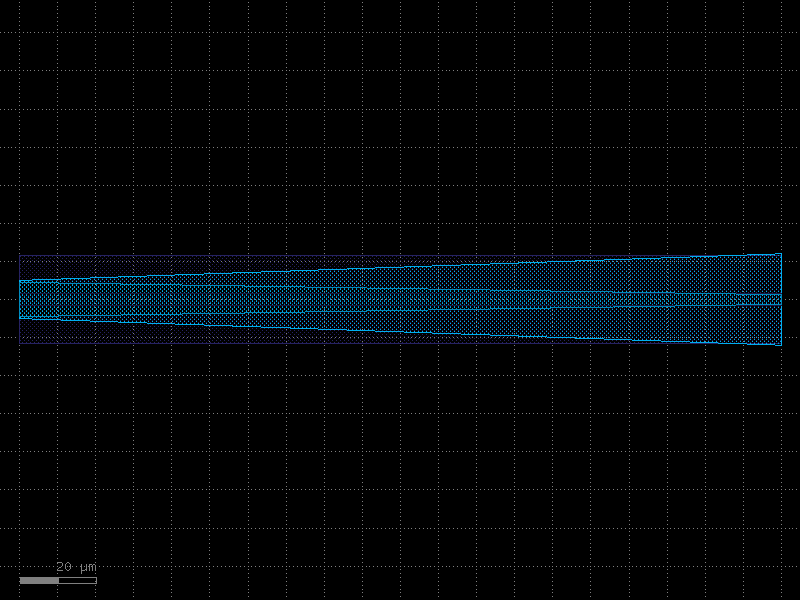
straight#
- gvtt.components.straight(length: float = 10.0, npoints: int = 2, *, cross_section: CrossSectionSpec = functools.partial(<function strip>, width=1.874), width: float | None = None) Component #
Returns a Straight waveguide.
- Parameters:
length – straight length (um).
npoints – number of points.
cross_section – specification (CrossSection, string or dict).
width – width of the waveguide. If None, it will use the width of the cross_section.
o1 -------------- o2 length
import gvtt
c = gvtt.components.straight(length=10.0, npoints=2)
c.plot()
(Source code
, png
, hires.png
, pdf
)
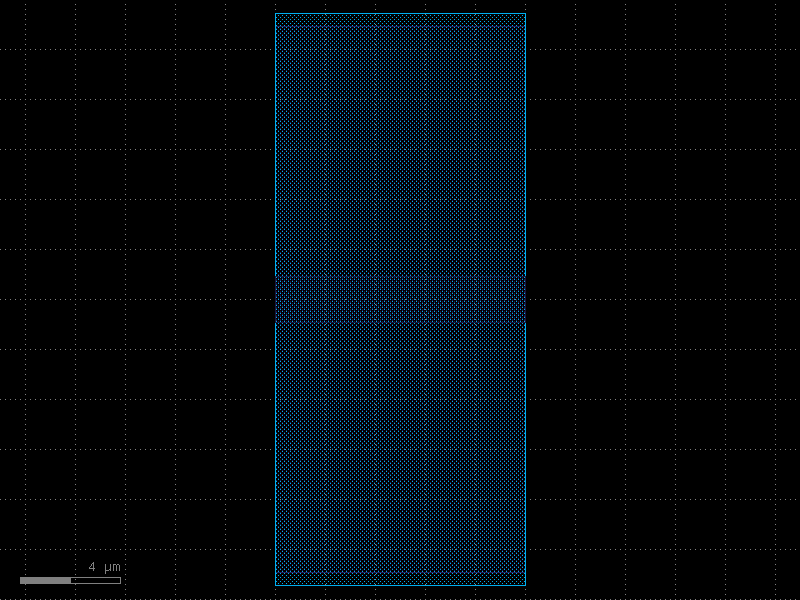
straight_rib#
- gvtt.components.straight_rib(length: float = 10.0, npoints: int = 2, *, cross_section: CrossSectionSpec = CrossSection(sections=(Section(width=2.5, offset=0.0, insets=None, layer=<LayerMap.TYPE_RIB: 46>, port_names=('o1', 'o2'), port_types=('optical', 'optical'), name='_default', hidden=False, simplify=None, width_function=None, offset_function=None), Section(width=2.5, offset=0, insets=None, layer='WG_RIBS_SUB', port_names=(None, None), port_types=('optical', 'optical'), name='WG', hidden=False, simplify=None, width_function=None, offset_function=None), Section(width=22.5, offset=0, insets=None, layer='WG_RIBS_ADD', port_names=(None, None), port_types=('optical', 'optical'), name='trench', hidden=False, simplify=None, width_function=None, offset_function=None)), components_along_path=(), radius=10.0, radius_min=None, bbox_layers=None, bbox_offsets=None), width: float | None = None) Component #
Returns a Straight waveguide.
- Parameters:
length – straight length (um).
npoints – number of points.
cross_section – specification (CrossSection, string or dict).
width – width of the waveguide. If None, it will use the width of the cross_section.
o1 -------------- o2 length
import gvtt
c = gvtt.components.straight_rib(length=10.0, npoints=2)
c.plot()
(Source code
, png
, hires.png
, pdf
)
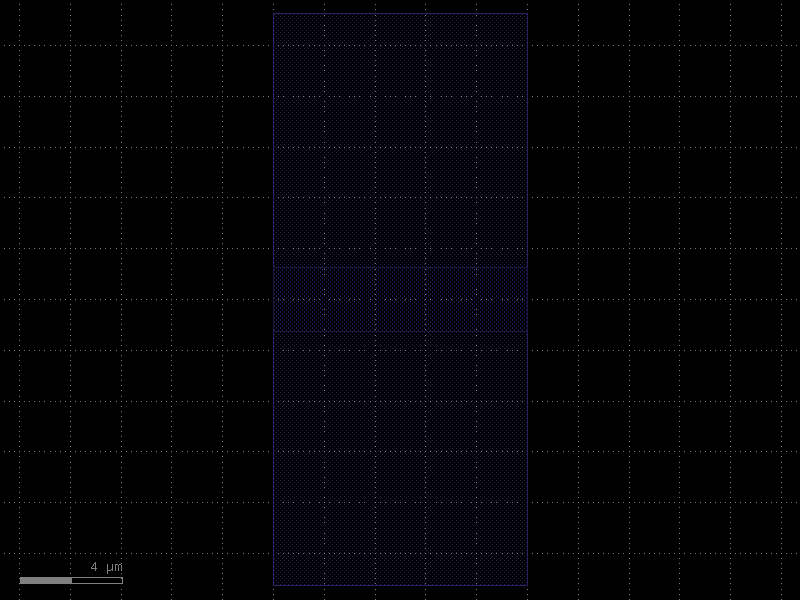
straight_sc#
- gvtt.components.straight_sc(length: float = 10.0, npoints: int = 2, *, cross_section: CrossSectionSpec = functools.partial(<function strip>, width=1.874), width: float | None = None) Component #
Returns a Straight waveguide.
- Parameters:
length – straight length (um).
npoints – number of points.
cross_section – specification (CrossSection, string or dict).
width – width of the waveguide. If None, it will use the width of the cross_section.
o1 -------------- o2 length
import gvtt
c = gvtt.components.straight_sc(length=10.0, npoints=2)
c.plot()
(Source code
, png
, hires.png
, pdf
)
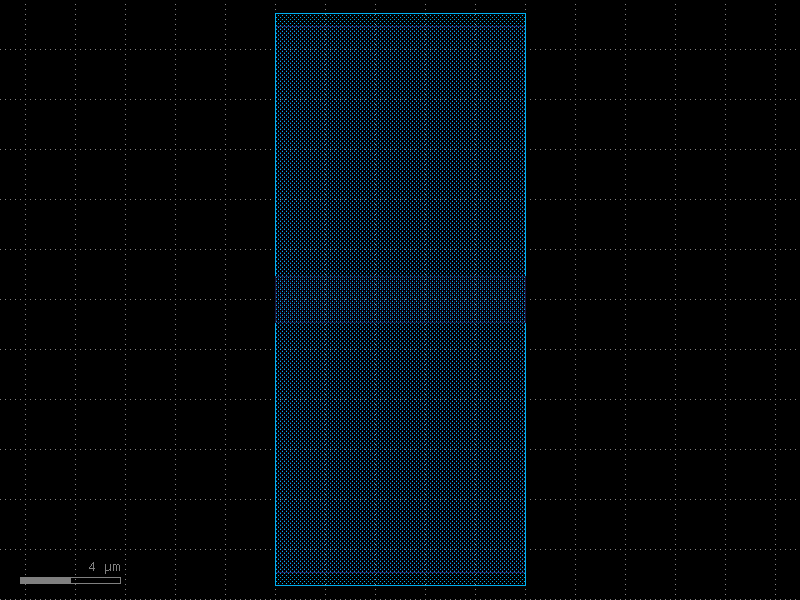
strip_taper#
- gvtt.components.strip_taper(width1: float = 1, width2: float = 1, taper_ratio: float = 25.0, length: float | None = None, **kwargs: Any) Component [source]#
Standard rib-to-strip waveguide converter.
- Parameters:
width1 – initial width.
width2 – final width.
taper_ratio – taper ratio.
length – taper length.
**kwargs – additional arguments.
import gvtt
c = gvtt.components.strip_taper(width1=1, width2=1, taper_ratio=25.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
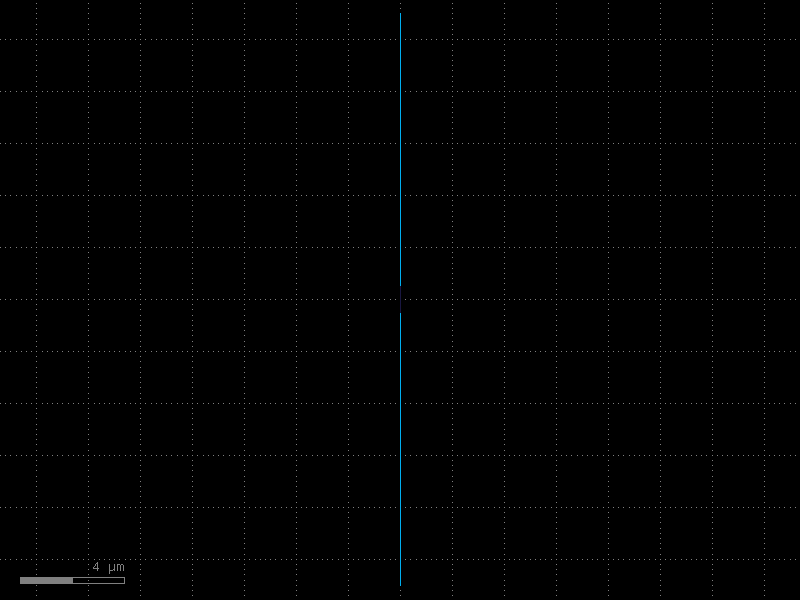
strip_to_rib#
- gvtt.components.strip_to_rib(length: float = 200.0, width1: float = 3.0, width2: float = 3.0) Component [source]#
Returns strip to rib transition.
- Parameters:
length – in um.
import gvtt
c = gvtt.components.strip_to_rib(length=200.0, width1=3.0, width2=3.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
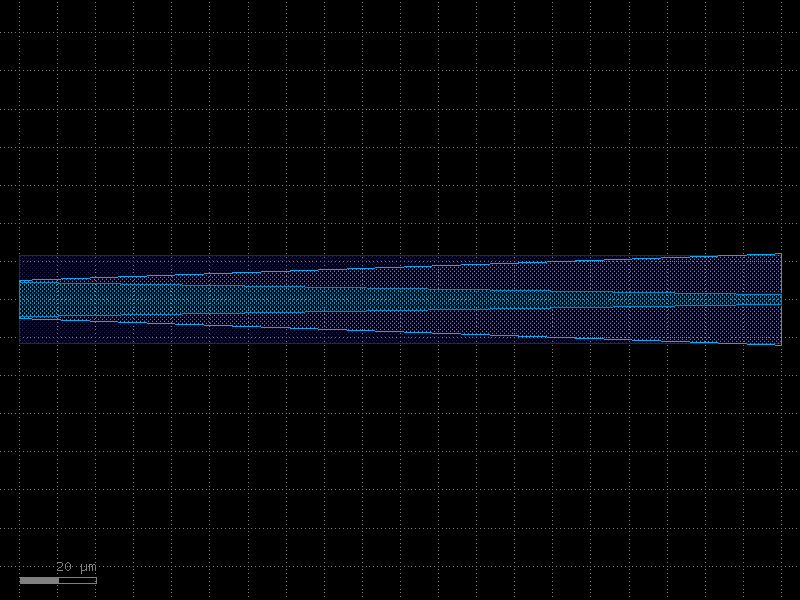