Here are the parametric cells available in the PDK
Cells#
alter_interdig#
- gf180.alter_interdig(sd_diff=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>), pc1=functools.partial(<function array>, component=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>)), pc2=functools.partial(<function array>, component=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>)), sd_l=0.36, nf=1, pat='', pc_x=0.1, pc_spacing=0.1, label: bool = False, g_label: ~collections.abc.Sequence[str] | None = None, nl: int = 1, patt_label: bool = False) Component [source]#
Returns interdigitation polygons of gate with alternating poly contacts.
- Args :
sd_diff : source/drain diffusion rectangle. pc1 : first poly contact array. pc2 : second poly contact array. sd_l : source/drain length. nf : number of fingers. pat: string of the required pattern. poly_con : component of poly contact. sd_diff_inter : inter source/drain diffusion rectangle. l_gate : gate length. inter_sd_l : inter diffusion length. nf : number of fingers.
import gf180
c = gf180.alter_interdig(sd_l=0.36, nf=1, pat='', pc_x=0.1, pc_spacing=0.1, label=False, nl=1, patt_label=False)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
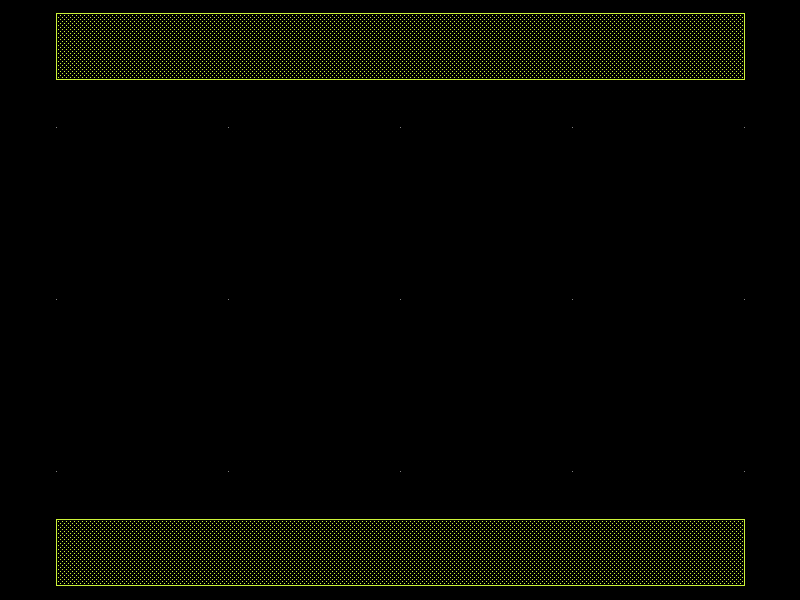
cap_mim#
- gf180.cap_mim(mim_option: str = 'A', metal_level: str = 'M4', lc: float = 2, wc: float = 2, label: bool = False, top_label: str = '', bot_label: str = '') Component [source]#
Return mim cap.
- Parameters:
min_option – MIM-A or MIM-B.
metal_level – metal level. M4, M5, M6.
lc – cap length.
wc – cap width.
label – 1 to add labels.
top_label – top label.
bot_label – bottom label.
import gf180
c = gf180.cap_mim(mim_option='A', metal_level='M4', lc=2, wc=2, label=False, top_label='', bot_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
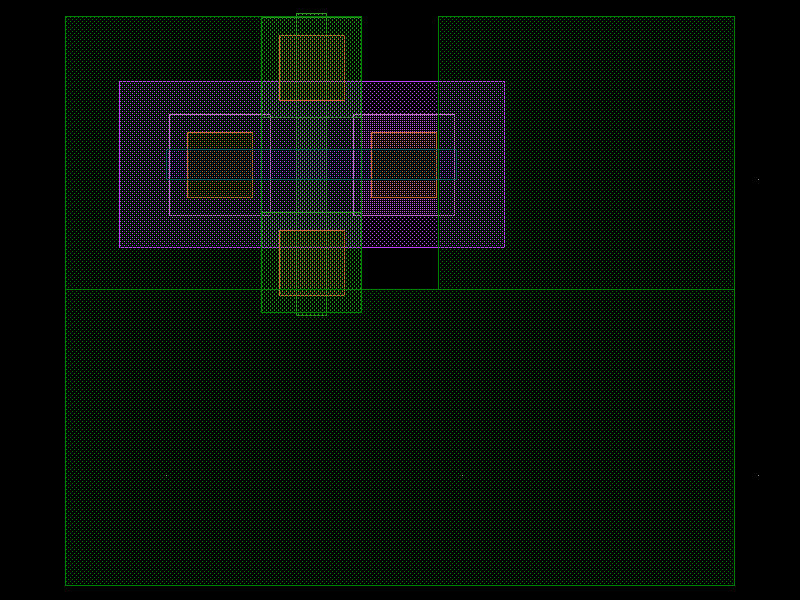
cap_mos#
- gf180.cap_mos(type: str = 'cap_nmos', lc: float = 0.1, wc: float = 0.1, volt: str = '3.3V', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, g_label: str = '', sd_label: str = '') Component [source]#
- Usage:-
used to draw NMOS capacitor (Outside DNWELL) by specifying parameters
- Arguments:-
l : Float of diff length w : Float of diff width.
import gf180
c = gf180.cap_mos(type='cap_nmos', lc=0.1, wc=0.1, volt='3.3V', deepnwell=False, pcmpgr=False, label=False, g_label='', sd_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
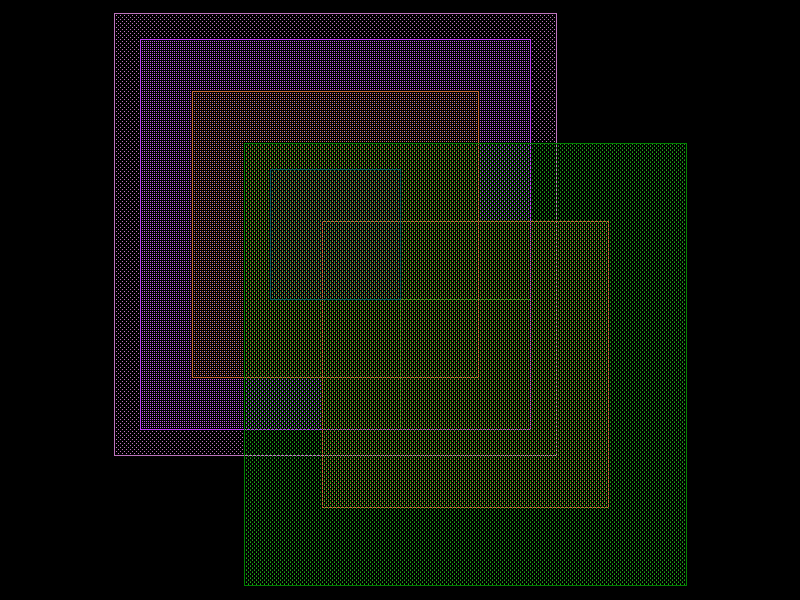
cap_mos_inst#
- gf180.cap_mos_inst(lc: float = 0.1, wc: float = 0.1, cmp_w: float = 0.1, con_w: float = 0.1, pl_l: float = 0.1, cmp_ext: float = 0.1, pl_ext: float = 0.1, implant_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.nplus: 49>, implant_enc: tuple[float, float] = (0.1, 0.1), label: bool = False, g_label: str = '') Component [source]#
Returns mos cap simple instance.
- Parameters:
lc – length of mos_cap.
ws – width of mos_cap.
cmp_w – width of layer[“comp”].
con_w – min width of comp contain contact.
pl_l – length od layer[“poly2”].
cmp_ext – comp extension beyond poly2.
pl_ext – poly2 extension beyond comp.
implant_layer – Layer of implant [nplus,pplus].
implant_enc – enclosure of implant_layer to comp.
label – 1 to add labels.
g_label – gate label.
import gf180
c = gf180.cap_mos_inst(lc=0.1, wc=0.1, cmp_w=0.1, con_w=0.1, pl_l=0.1, cmp_ext=0.1, pl_ext=0.1, implant_layer='nplus', implant_enc=(0.1, 0.1), label=False, g_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
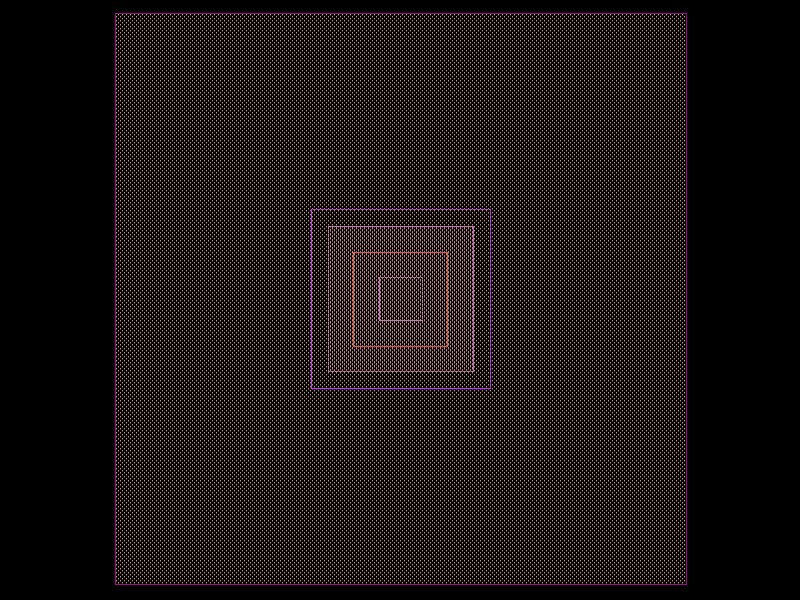
diode_dw2ps#
- gf180.diode_dw2ps(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, volt: str = '3.3V', pcmpgr: bool = False, label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
Used to draw LVPWELL/DNWELL diode by specifying parameters.
- Parameters:
la – anode length.
wa – anode width.
cw – cathode width.
volt – operating voltage of the diode [3.3V, 5V/6V].
pcmpgr – True if pwell guardring is required.
label – True if labels are required.
p_label – label for pwell.
n_label – label for nwell.
import gf180
c = gf180.diode_dw2ps(la=0.1, wa=0.1, cw=0.1, volt='3.3V', pcmpgr=False, label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
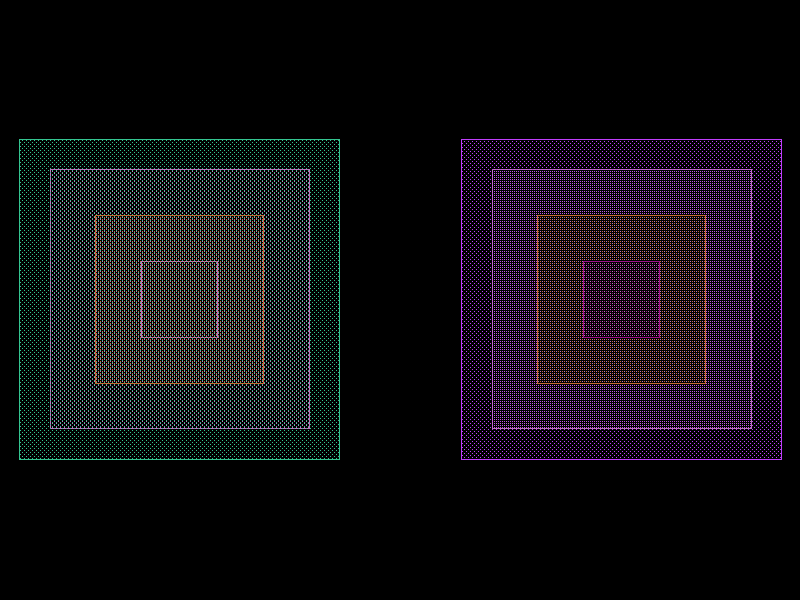
diode_nd2ps#
- gf180.diode_nd2ps(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, volt: str = '3.3V', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
Draw N+/LVPWELL diode (Outside DNWELL) by specifying parameters.
- Args::
la: Float of diff length (anode). wa: Float of diff width (anode). cw: Float of cathode width. volt: String of operating voltage of the diode [3.3V, 5V/6V]. deepnwell: Boolean of using Deep NWELL device. pcmpgr : Boolean of using P+ Guard Ring for Deep NWELL devices only. label: Boolean of adding labels.
import gf180
c = gf180.diode_nd2ps(la=0.1, wa=0.1, cw=0.1, volt='3.3V', deepnwell=False, pcmpgr=False, label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
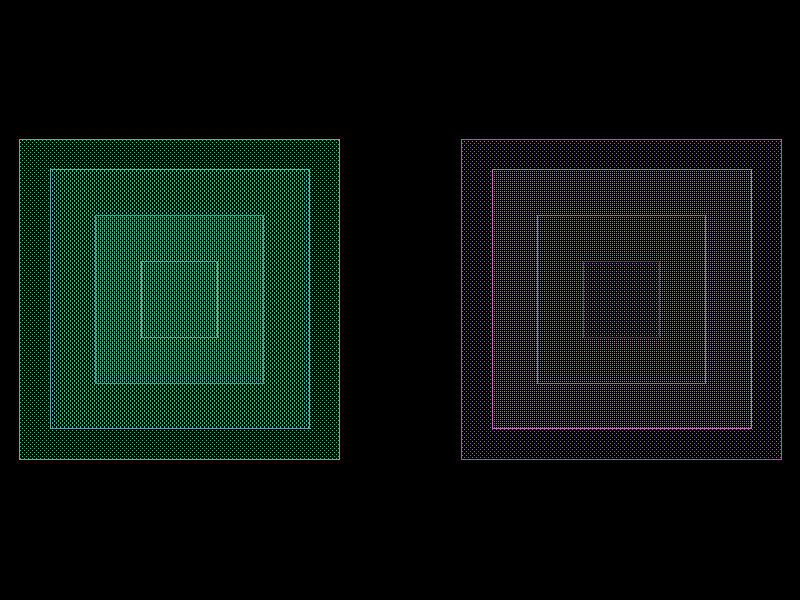
diode_nw2ps#
- gf180.diode_nw2ps(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, volt: str = '3.3V', label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
Used to draw 3.3V Nwell/Psub diode by specifying parameters.
- Parameters:
la – anode length.
wa – anode width.
cw – cathode width.
volt – operating voltage of the diode [3.3V, 5V/6V]
import gf180
c = gf180.diode_nw2ps(la=0.1, wa=0.1, cw=0.1, volt='3.3V', label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
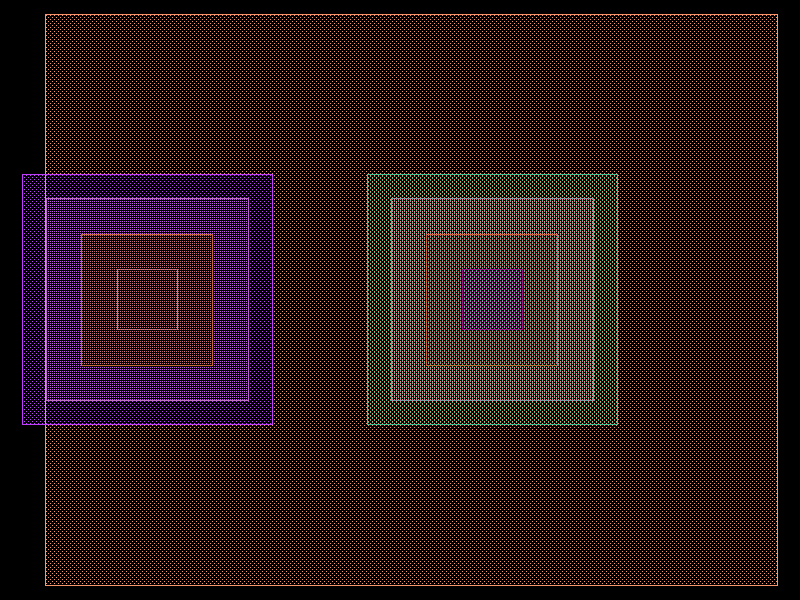
diode_pd2nw#
- gf180.diode_pd2nw(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, volt: str = '3.3V', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
- Usage:-
used to draw 3.3V P+/Nwell diode (Outside DNWELL) by specifying parameters
- Arguments:-
la : Float of diffusion length (anode) wa : Float of diffusion width (anode) volt : String of operating voltage of the diode [3.3V, 5V/6V] deepnwell : Boolean of using Deep NWELL device pcmpgr : Boolean of using P+ Guard Ring for Deep NWELL devices only.
import gf180
c = gf180.diode_pd2nw(la=0.1, wa=0.1, cw=0.1, volt='3.3V', deepnwell=False, pcmpgr=False, label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
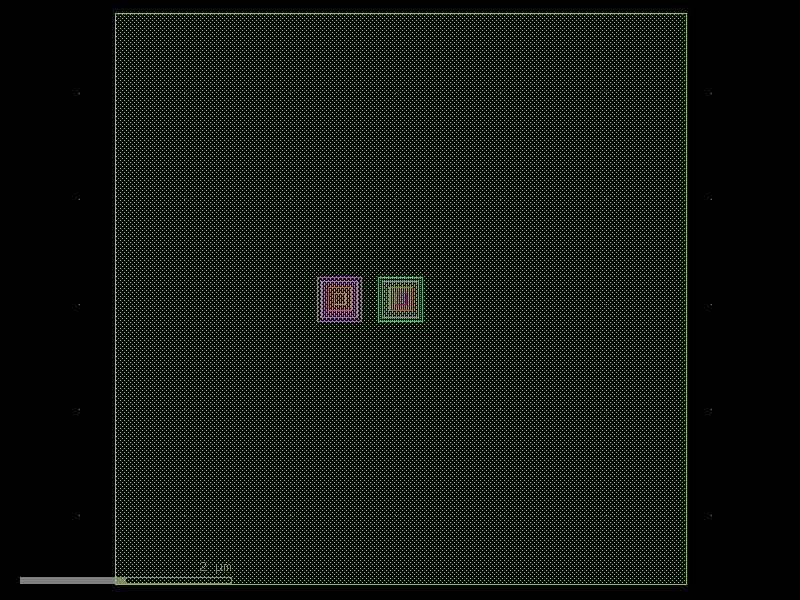
diode_pw2dw#
- gf180.diode_pw2dw(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, volt: str = '3.3V', pcmpgr: bool = False, label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
Used to draw LVPWELL/DNWELL diode by specifying parameters.
- Parameters:
la – anode length.
wa – anode width.
cw – cathode width.
volt – operating voltage of the diode [3.3V, 5V/6V]
pcmpgr – if True, pcmpgr will be added.
label – if True, labels will be added.
p_label – p contact label.
n_label – n contact label.
import gf180
c = gf180.diode_pw2dw(la=0.1, wa=0.1, cw=0.1, volt='3.3V', pcmpgr=False, label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
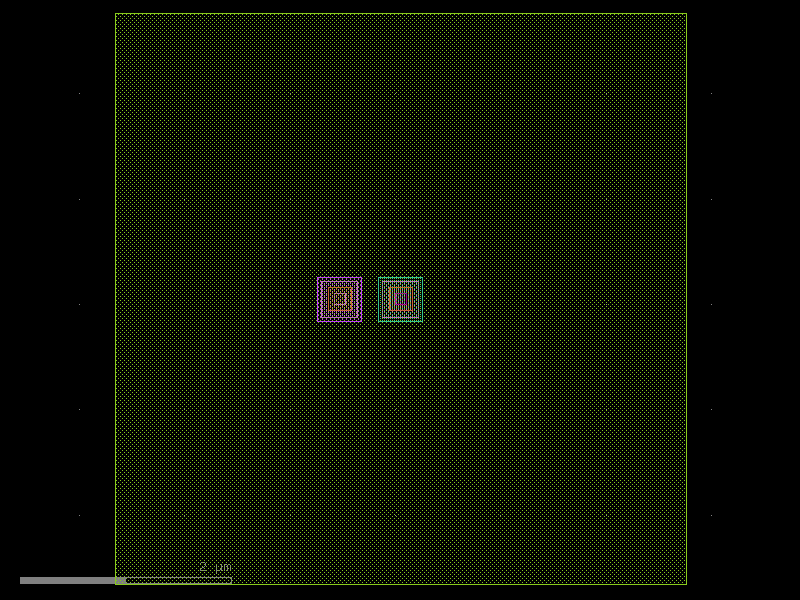
interdigit#
- gf180.interdigit(sd_diff=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>), pc1=functools.partial(<function array>, component=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>)), pc2=functools.partial(<function array>, component=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>)), poly_con=functools.partial(<function rectangle>, layer=<LAYER.comp: 14>), sd_l: float = 0.15, nf=1, patt=[''], gate_con_pos='top', pc_x=0.1, pc_spacing=0.1, label: bool = False, g_label: ~collections.abc.Sequence[str] | None = None, patt_label: bool = False) Component [source]#
Returns interdigitation related polygons.
- Args :
sd_diff : source/drain diffusion rectangle. pc1: first poly contact array. pc2: second poly contact array. poly_con: poly contact. sd_diff_inter : inter source/drain diffusion rectangle. l_gate : gate length. inter_sd_l : inter diffusion length. nf : number of fingers. pat : string of the required pattern. gate_con_pos : position of gate contact.
import gf180
c = gf180.interdigit(sd_l=0.15, nf=1, gate_con_pos='top', pc_x=0.1, pc_spacing=0.1, label=False, patt_label=False)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
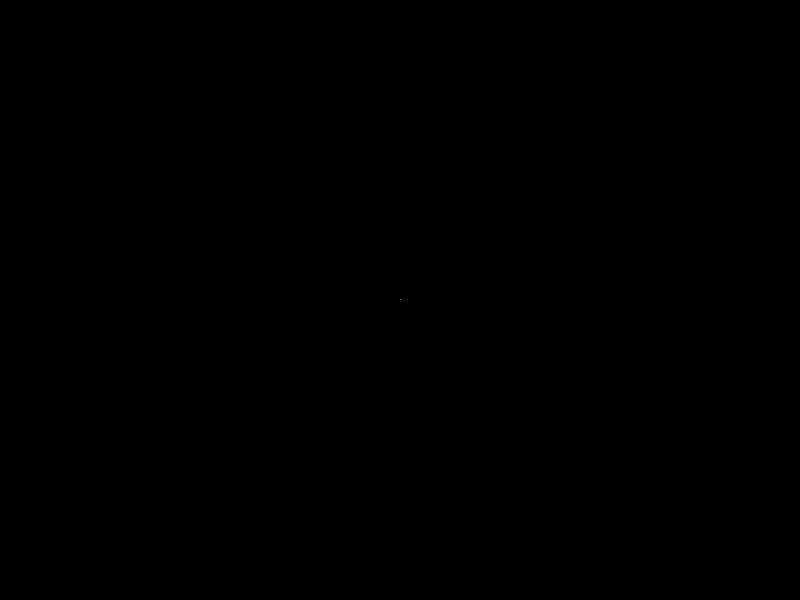
labels_gen#
- gf180.labels_gen(label_str: str = '', position: tuple[float, float] = (0.1, 0.1), layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.metal1_label: 76>, label: bool = False, labels: ~collections.abc.Sequence[str] | None = None, label_valid_len: int = 1, index: int = 0) Component [source]#
Returns labels at given position when label is enabled.
- Args :
label_str : string of the label. position : position of the label. layer : layer of the label. label : boolean of having the label. labels : list of given labels. label_valid_len : valid length of labels.
import gf180
c = gf180.labels_gen(label_str='', position=(0.1, 0.1), layer='metal1_label', label=False, label_valid_len=1, index=0)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
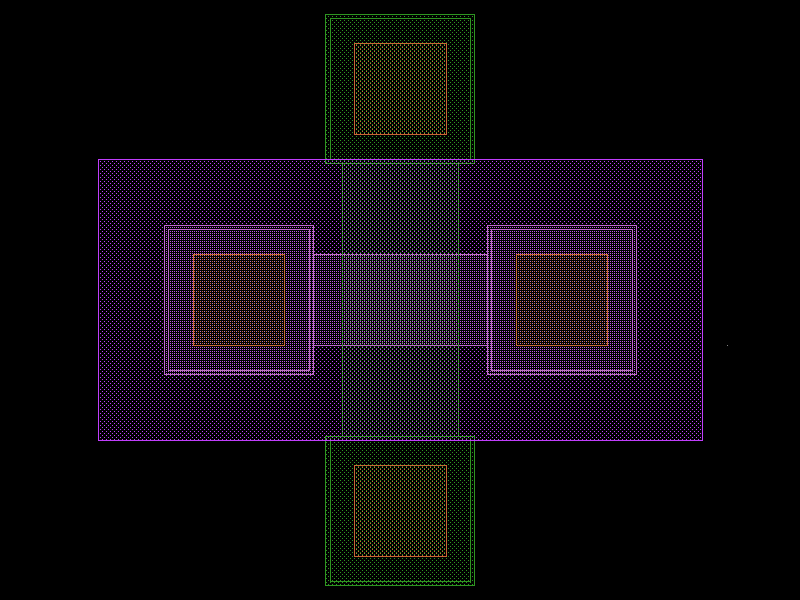
nfet#
- gf180.nfet(l_gate: float = 0.28, w_gate: float = 0.22, sd_con_col: int = 1, inter_sd_l: float = 0.24, nf: int = 1, grw: float = 0.22, volt: str = '3.3V', bulk: str = 'None', con_bet_fin: int = 1, gate_con_pos: str = 'alternating', interdig: int = 0, patt: str = '', deepnwell: int = 0, pcmpgr: int = 0, label: bool = False, sd_label: Sequence[str] | None = [], g_label: Sequence[str] = (), sub_label: str = '', patt_label: bool = False) Component [source]#
Return nfet.
- Parameters:
l – Float of gate length
w – Float of gate width
sd_l – Float of source and drain diffusion length
inter_sd_l – Float of source and drain diffusion length between fingers
nf – integer of number of fingers
M – integer of number of multipliers
grw – guard ring width when enabled
type – string of the device type
bulk – String of bulk connection type (None, Bulk Tie, Guard Ring)
con_bet_fin – boolean of having contacts for diffusion between fingers
gate_con_pos – string of choosing the gate contact position (bottom, top, alternating )
import gf180
c = gf180.nfet(l_gate=0.28, w_gate=0.22, sd_con_col=1, inter_sd_l=0.24, nf=1, grw=0.22, volt='3.3V', bulk='None', con_bet_fin=1, gate_con_pos='alternating', interdig=0, patt='', deepnwell=0, pcmpgr=0, label=False, g_label=(), sub_label='', patt_label=False)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
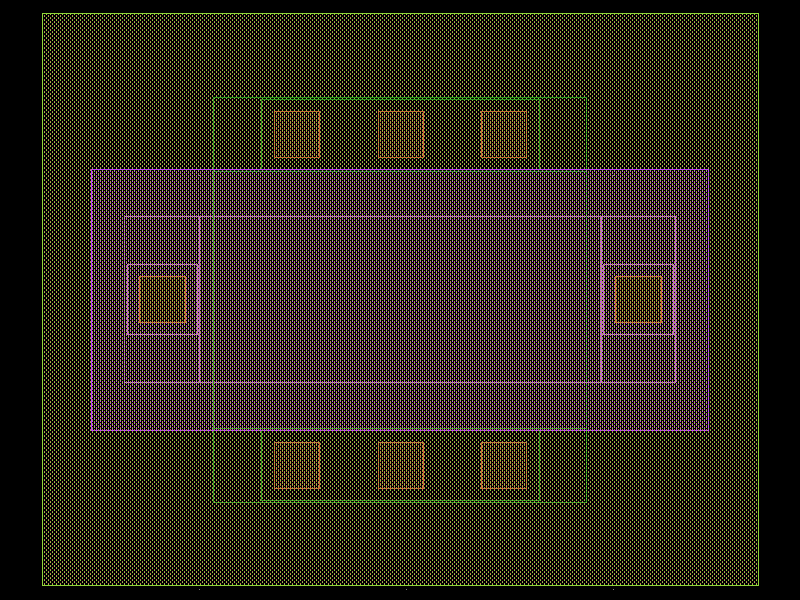
nfet_06v0_nvt#
- gf180.nfet_06v0_nvt(l_gate: float = 1.8, w_gate: float = 0.8, sd_con_col: int = 1, inter_sd_l: float = 0.24, nf: int = 1, grw: float = 0.22, bulk='None', con_bet_fin: int = 1, gate_con_pos='alternating', interdig: int = 0, patt='', label: bool = False, sd_label: Sequence[str] | None = [], g_label: str = [], sub_label: str = '', patt_label: bool = False) Component [source]#
Draw Native NFET 6V transistor by specifying parameters.
- Arg:
l : Float of gate length w : Float of gate width ld : Float of diffusion length nf : Integer of number of fingers grw : Float of guard ring width [If enabled] bulk : String of bulk connection type [None, Bulk Tie, Guard Ring]
import gf180
c = gf180.nfet_06v0_nvt(l_gate=1.8, w_gate=0.8, sd_con_col=1, inter_sd_l=0.24, nf=1, grw=0.22, bulk='None', con_bet_fin=1, gate_con_pos='alternating', interdig=0, patt='', label=False, sub_label='', patt_label=False)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
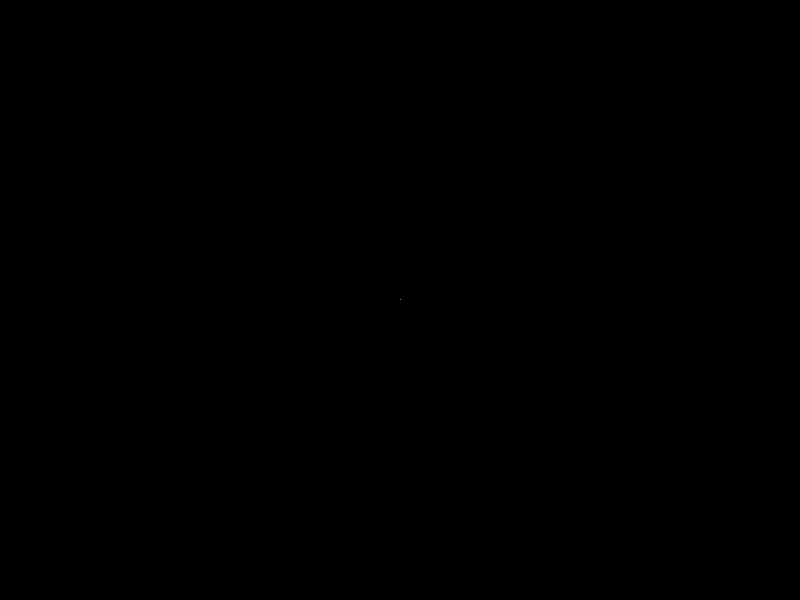
nfet_deep_nwell#
- gf180.nfet_deep_nwell(deepnwell: bool = False, pcmpgr: bool = False, inst_size: tuple[float, float] = (0.1, 0.1), inst_xmin: float = 0.1, inst_ymin: float = 0.1, grw: float = 0.36) Component [source]#
Return nfet deepnwell.
- Args :
deepnwell : boolean of having deepnwell pcmpgr : boolean of having deepnwell guardring inst_size : deepnwell enclosed size inst_xmin : deepnwell enclosed dxmin inst_ymin : deepnwell enclosed dymin grw : guardring width
import gf180
c = gf180.nfet_deep_nwell(deepnwell=False, pcmpgr=False, inst_size=(0.1, 0.1), inst_xmin=0.1, inst_ymin=0.1, grw=0.36)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
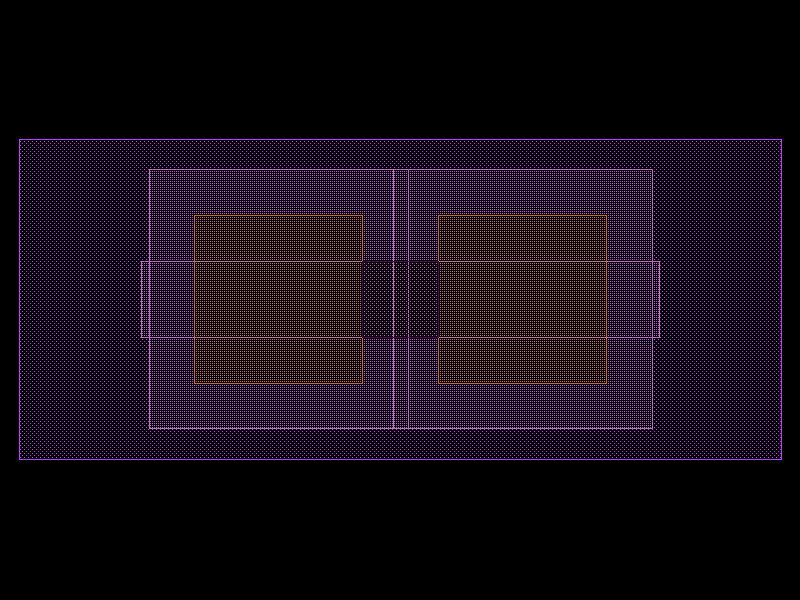
nplus_res#
- gf180.nplus_res(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'nplus_s', sub: bool = False, deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.nplus_res(l_res=0.1, w_res=0.1, res_type='nplus_s', sub=False, deepnwell=False, pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
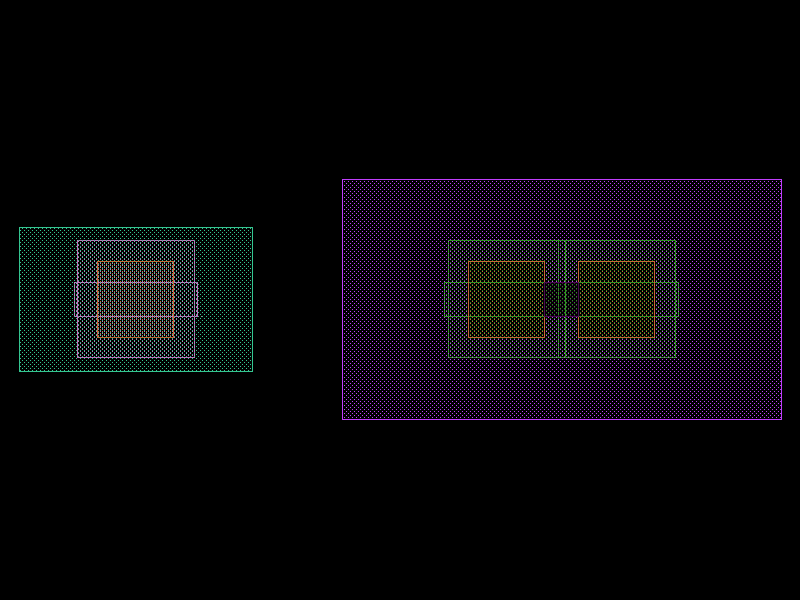
npolyf_res#
- gf180.npolyf_res(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'npolyf_s', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.npolyf_res(l_res=0.1, w_res=0.1, res_type='npolyf_s', deepnwell=False, pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
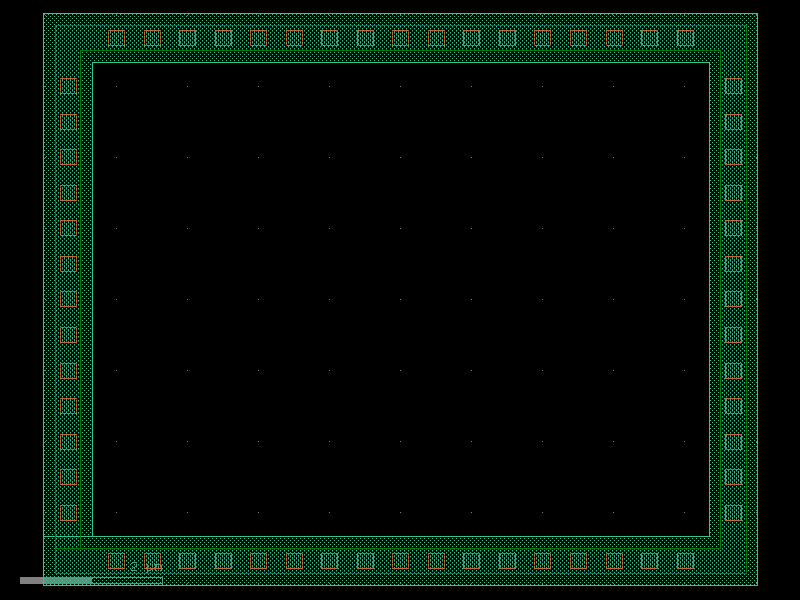
pcmpgr_gen#
- gf180.pcmpgr_gen(dn_rect=functools.partial(<function rectangle>, layer=<LAYER.dnwell: 46>), grw: float = 0.36) Component [source]#
Return deepnwell guardring.
- Parameters:
dn_rect – deepnwell polygon.
grw – guardring width.
import gf180
c = gf180.pcmpgr_gen(grw=0.36)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
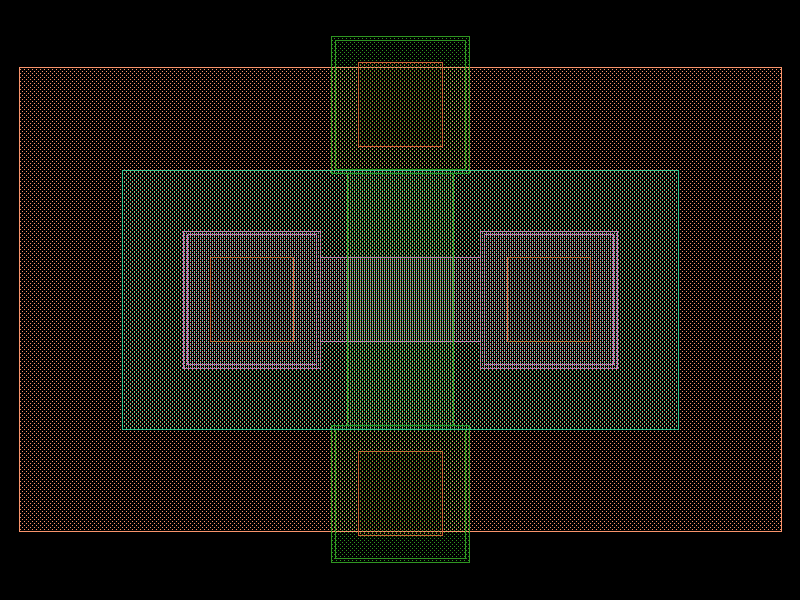
pfet#
- gf180.pfet(l_gate: float = 0.28, w_gate: float = 0.22, sd_con_col: int = 1, inter_sd_l: float = 0.24, nf: int = 1, grw: float = 0.22, volt: str = '3.3V', bulk: str = 'None', con_bet_fin: int = 1, gate_con_pos: str = 'alternating', interdig: int = 0, patt: str = '', deepnwell: int = 0, pcmpgr: int = 0, label: bool = False, sd_label: Sequence[str] | None = (), g_label: Sequence[str] = (), sub_label: str = '', patt_label: bool = False) Component [source]#
Return pfet.
- Parameters:
l – Float of gate length
w – Float of gate width
sd_l – Float of source and drain diffusion length
inter_sd_l – Float of source and drain diffusion length between fingers
nf – integer of number of fingers
M – integer of number of multipliers
grw – guard ring width when enabled
type – string of the device type
bulk – String of bulk connection type (None, Bulk Tie, Guard Ring)
con_bet_fin – boolean of having contacts for diffusion between fingers
gate_con_pos – string of choosing the gate contact position (bottom, top, alternating )
import gf180
c = gf180.pfet(l_gate=0.28, w_gate=0.22, sd_con_col=1, inter_sd_l=0.24, nf=1, grw=0.22, volt='3.3V', bulk='None', con_bet_fin=1, gate_con_pos='alternating', interdig=0, patt='', deepnwell=0, pcmpgr=0, label=False, sd_label=(), g_label=(), sub_label='', patt_label=False)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
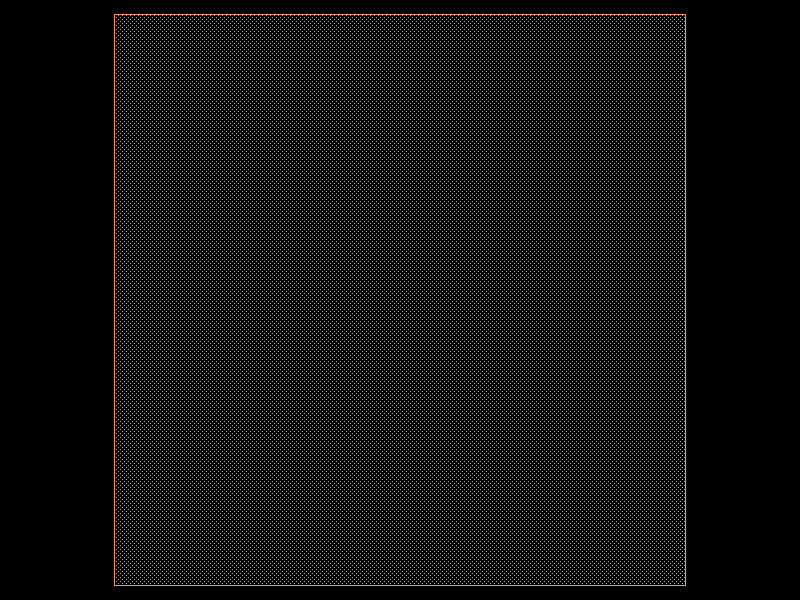
pfet_deep_nwell#
- gf180.pfet_deep_nwell(deepnwell: bool = False, pcmpgr: bool = False, enc_size: tuple[float, float] = (0.1, 0.1), enc_xmin: float = 0.1, enc_ymin: float = 0.1, nw_enc_pcmp: float = 0.1, grw: float = 0.36) Component [source]#
Returns pfet well related polygons.
- Args :
deepnwell : boolaen of having deepnwell pcmpgr : boolean of having deepnwell guardring enc_size : enclosed size enc_xmin : enclosed dxmin enc_ymin : enclosed dymin nw_enc_pcmp : nwell enclosure of pcomp grw : guardring width
import gf180
c = gf180.pfet_deep_nwell(deepnwell=False, pcmpgr=False, enc_size=(0.1, 0.1), enc_xmin=0.1, enc_ymin=0.1, nw_enc_pcmp=0.1, grw=0.36)
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
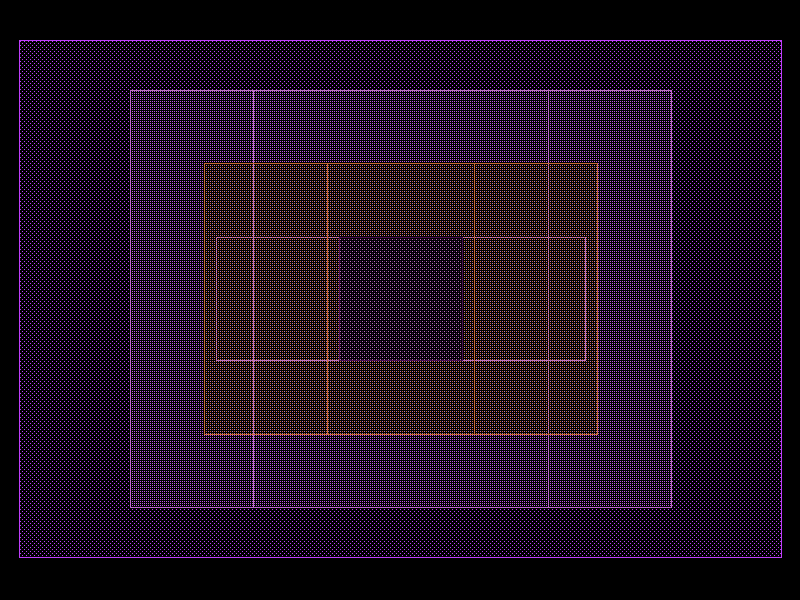
plus_res_inst#
- gf180.plus_res_inst(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'nplus_s', sub: bool = False, cmp_res_ext: float = 0.1, con_enc: float = 0.1, cmp_imp_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.nplus: 49>, sub_imp_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.pplus: 50>, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
Returns 2-terminal Metal resistor by specifying parameters.
import gf180
c = gf180.plus_res_inst(l_res=0.1, w_res=0.1, res_type='nplus_s', sub=False, cmp_res_ext=0.1, con_enc=0.1, cmp_imp_layer='nplus', sub_imp_layer='pplus', label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
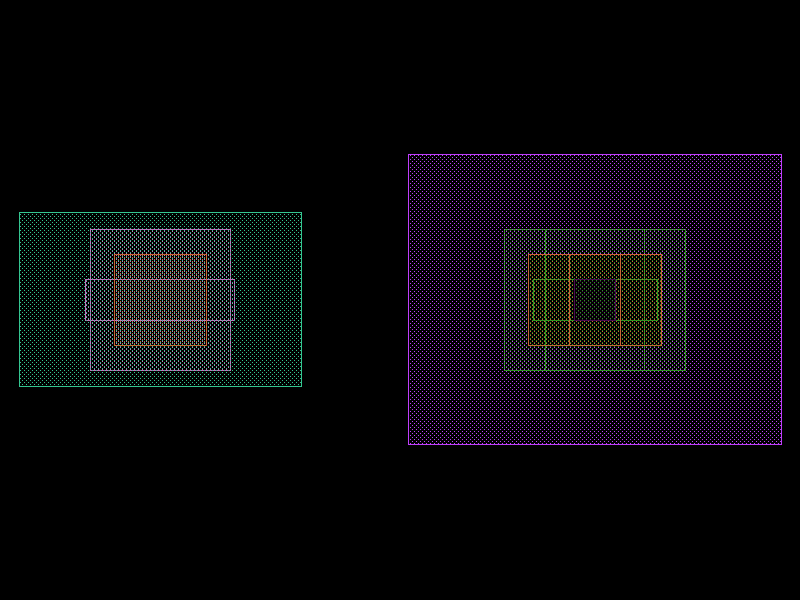
polyf_res_inst#
- gf180.polyf_res_inst(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'npolyf_s', pl_res_ext: float = 0.1, con_enc: float = 0.1, pl_imp_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.nplus: 49>, sub_imp_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.pplus: 50>, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.polyf_res_inst(l_res=0.1, w_res=0.1, res_type='npolyf_s', pl_res_ext=0.1, con_enc=0.1, pl_imp_layer='nplus', sub_imp_layer='pplus', label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
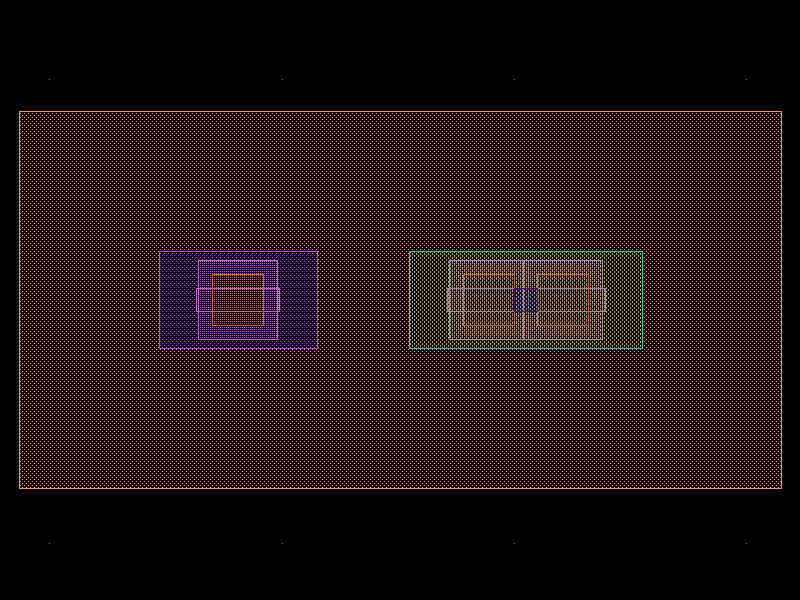
pplus_res#
- gf180.pplus_res(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'pplus_s', sub: bool = False, deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.pplus_res(l_res=0.1, w_res=0.1, res_type='pplus_s', sub=False, deepnwell=False, pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
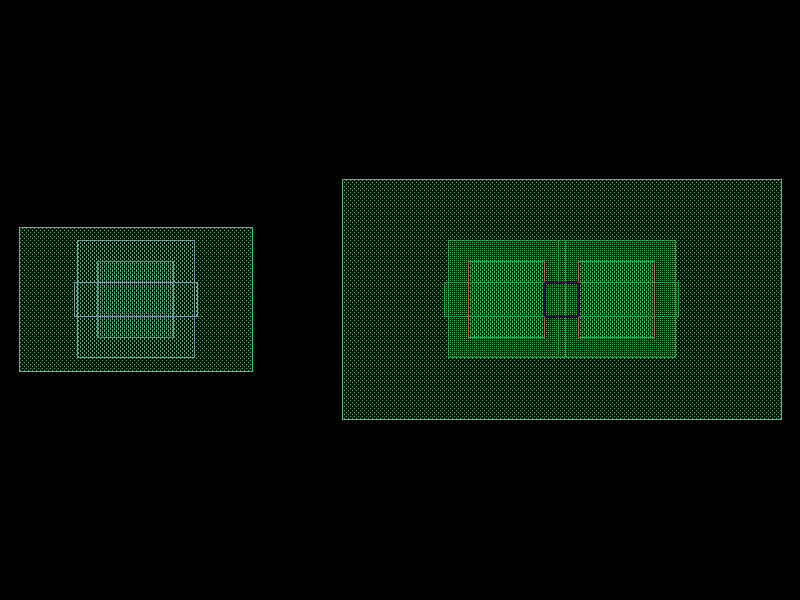
ppolyf_res#
- gf180.ppolyf_res(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'ppolyf_s', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.ppolyf_res(l_res=0.1, w_res=0.1, res_type='ppolyf_s', deepnwell=False, pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
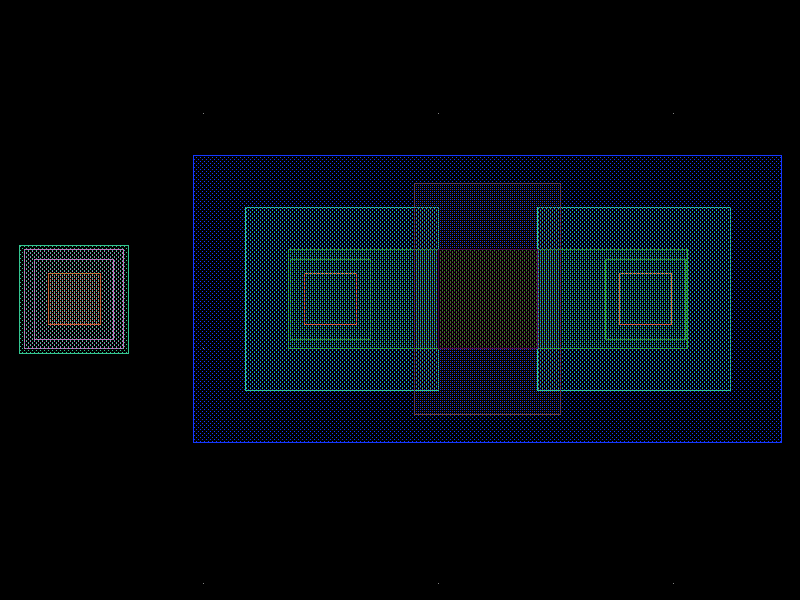
ppolyf_u_high_Rs_res#
- gf180.ppolyf_u_high_Rs_res(l_res: float = 0.42, w_res: float = 0.42, volt: str = '3.3V', deepnwell: bool = False, pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.ppolyf_u_high_Rs_res(l_res=0.42, w_res=0.42, volt='3.3V', deepnwell=False, pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
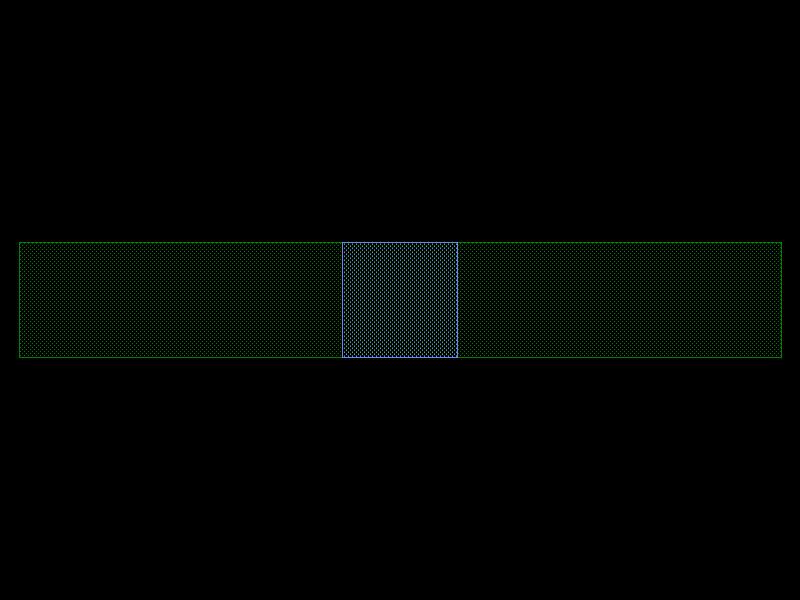
res#
- gf180.res(l_res: float = 0.1, w_res: float = 0.1, res_type: str = 'rm1', label: bool = False, r0_label: str = '', r1_label: str = '') Component [source]#
Returns 2-terminal Metal resistor by specifying parameters.
- Parameters:
l_res – length of resistor.
w_res – width of resistor.
res_type – type of resistor.
label – label generation.
r0_label – label for resistor.
r1_label – label for resistor.
import gf180
c = gf180.res(l_res=0.1, w_res=0.1, res_type='rm1', label=False, r0_label='', r1_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
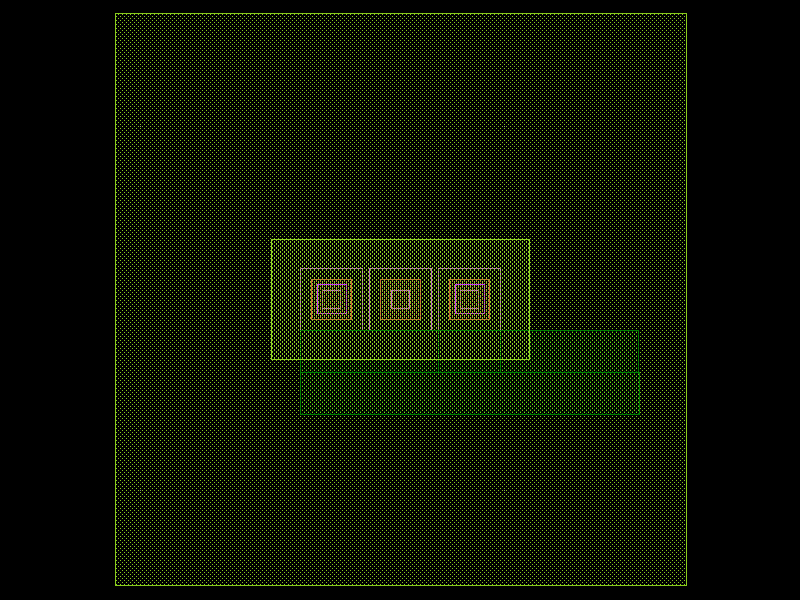
sc_diode#
- gf180.sc_diode(la: float = 0.1, wa: float = 0.1, cw: float = 0.1, m: int = 1, pcmpgr: bool = False, label: bool = False, p_label: str = '', n_label: str = '') Component [source]#
Used to draw N+/LVPWELL diode (Outside DNWELL) by specifying parameters.
- Parameters:
la – Float of diff length (anode)
wa – Float of diff width (anode)
m – Integer of number of fingers
pcmpgr – Boolean of using P+ Guard Ring for Deep NWELL devices only
import gf180
c = gf180.sc_diode(la=0.1, wa=0.1, cw=0.1, m=1, pcmpgr=False, label=False, p_label='', n_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
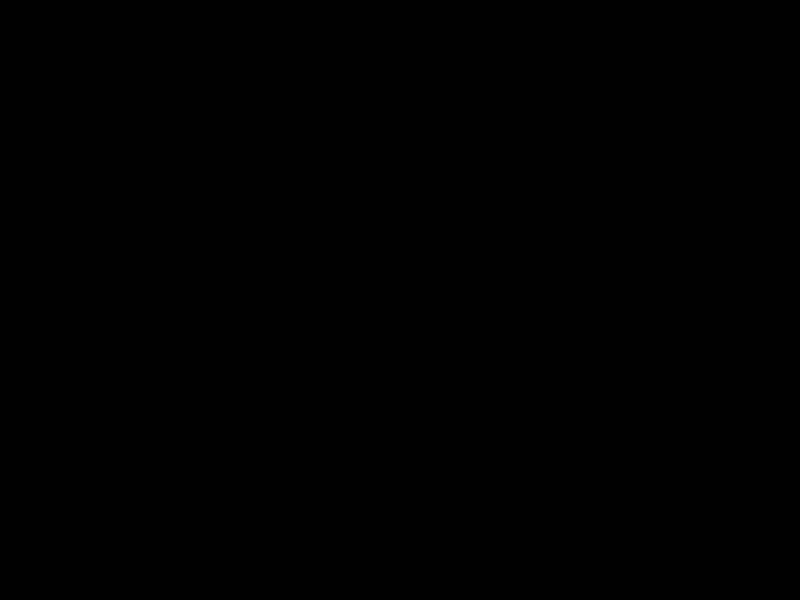
via_generator#
- gf180.via_generator(x_range: tuple[float, float] = (0, 1), y_range: tuple[float, float] = (0, 1), via_size: tuple[float, float] = (0.17, 0.17), via_layer: tuple[int, int] | str | int | LayerEnum = (66, 44), via_enclosure: tuple[float, float] = (0.06, 0.06), via_spacing: tuple[float, float] = (0.17, 0.17)) Component [source]#
Return only vias withen the range xrange and yrange while enclosing by via_enclosure and set number of rows and number of columns according to ranges and via size and spacing.
- Parameters:
x_range – dx range.
y_range – dy range.
via_size – via size.
via_layer – via layer.
via_enclosure – via enclosure.
via_spacing – via spacing.
import gf180
c = gf180.via_generator(x_range=(0, 1), y_range=(0, 1), via_size=(0.17, 0.17), via_layer=(66, 44), via_enclosure=(0.06, 0.06), via_spacing=(0.17, 0.17))
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
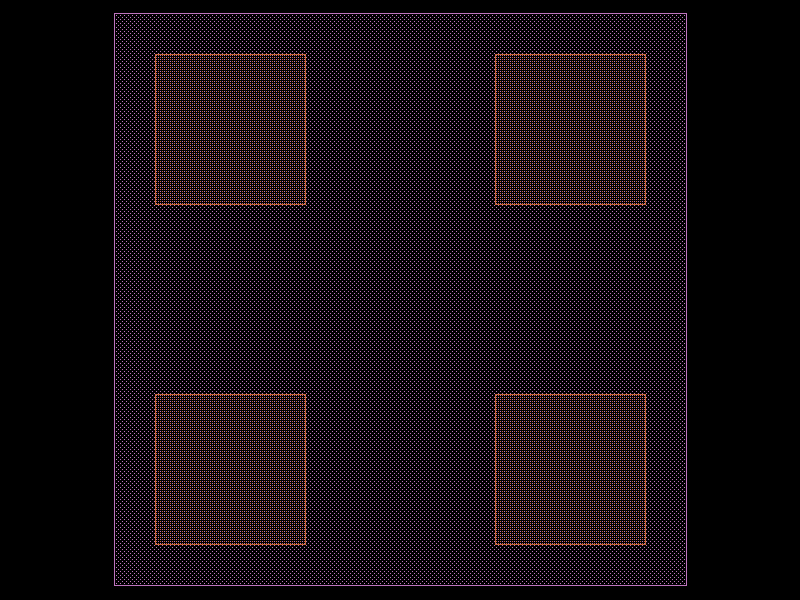
via_stack#
- gf180.via_stack(x_range: tuple[float, float] = (0, 1), y_range: tuple[float, float] = (0, 1), metal_level: int = 1, con_size: tuple[float, float] = (0.22, 0.22), con_enc: float = 0.07, m_enc: float = 0.06, con_spacing: tuple[float, float] = (0.28, 0.28), via_size: tuple[float, float] = (0.22, 0.22), via_spacing: tuple[float, float] = (0.28, 0.28), via_enc: tuple[float, float] = (0.06, 0.06), base_layer: tuple[int, int] | str | int | ~kfactory.layer.LayerEnum = <LAYER.metal1: 10>, **kwargs: ~typing.Any) Component [source]#
Returns a via stack withen the range xrange and yrange and expecting the base_layer to be drawen.
- Parameters:
x_range – dx range.
y_range – dy range.
metal_level – metal level.
con_size – contact size.
con_enc – contact enclosure.
m_enc – metal enclosure.
con_spacing – contact spacing.
via_size – via size.
via_spacing – via spacing.
via_enc – via enclosure.
return via stack till the metal level indicated where : metal_level 1 : till m1 metal_level 2 : till m2 metal_level 3 : till m3 metal_level 4 : till m4 metal_level 5 : till m5 withen the range xrange and yrange and expecting the base_layer to be drawen
import gf180
c = gf180.via_stack(x_range=(0, 1), y_range=(0, 1), metal_level=1, con_size=(0.22, 0.22), con_enc=0.07, m_enc=0.06, con_spacing=(0.28, 0.28), via_size=(0.22, 0.22), via_spacing=(0.28, 0.28), via_enc=(0.06, 0.06), base_layer='metal1')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
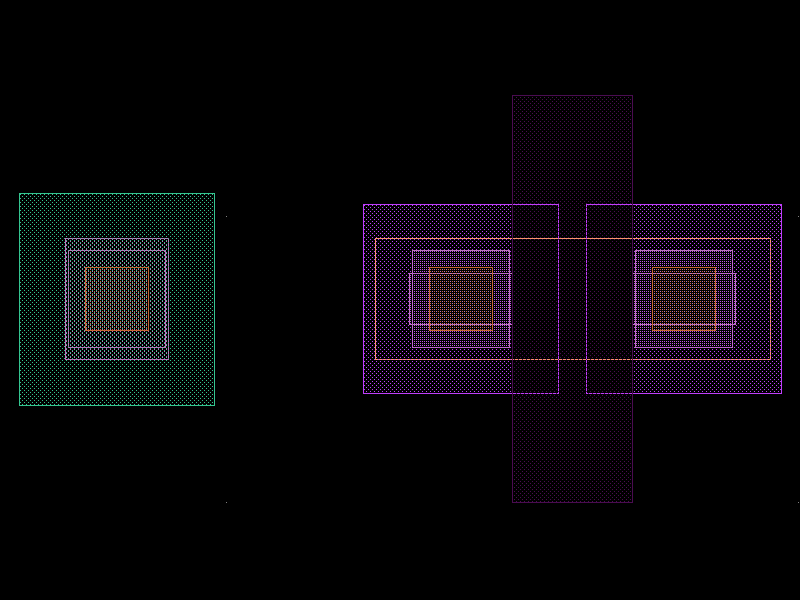
well_res#
- gf180.well_res(l_res: float = 0.42, w_res: float = 0.42, res_type: str = 'nwell', pcmpgr: bool = False, label: bool = False, r0_label: str = '', r1_label: str = '', sub_label: str = '') Component [source]#
import gf180
c = gf180.well_res(l_res=0.42, w_res=0.42, res_type='nwell', pcmpgr=False, label=False, r0_label='', r1_label='', sub_label='')
c = c.copy()
c.draw_ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
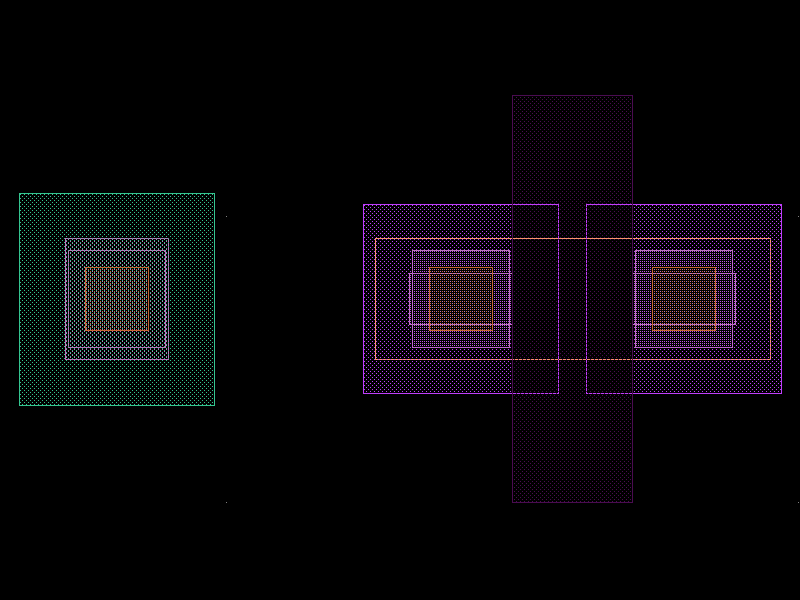