Here are the components available in the PDK
Cells#
add_fiber_array#
- ubcpdk.components.add_fiber_array(component: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.KCell = <function straight>, component_name: str | None = None, gc_port_name: str = 'o1', with_loopback: bool = False, fanout_length: float = 0.0, grating_coupler: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.KCell = <function gc_te1550>, cross_section: ~collections.abc.Callable[[...], ~gdsfactory.cross_section.CrossSection] | ~gdsfactory.cross_section.CrossSection | dict[str, ~typing.Any] | str | ~gdsfactory.cross_section.Transition = 'strip', straight: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.KCell = 'straight', taper: str | ~collections.abc.Callable[[...], ~gdsfactory.component.Component] | dict[str, ~typing.Any] | ~kfactory.kcell.KCell | None = None, **kwargs) Component [source]#
Returns component with grating couplers and labels on each port.
Routes all component ports south. Can add align_ports loopback reference structure on the edges.
- Parameters:
component – to connect.
component_name – for the label.
gc_port_name – grating coupler input port name ‘o1’.
with_loopback – True, adds loopback structures.
fanout_length – None # if None, automatic calculation of fanout length.
grating_coupler – grating coupler instance, function or list of functions.
cross_section – spec.
straight – straight component.
taper – taper component.
kwargs – cross_section settings.
import ubcpdk
c = ubcpdk.components.add_fiber_array(gc_port_name='o1', with_loopback=False, optical_routing_type=0, fanout_length=0.0, cross_section='xs_sc', layer_label=(10, 0))
c.plot()
add_fiber_array_pads_rf#
- ubcpdk.components.add_fiber_array_pads_rf(component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'ring_single_heater', username: str = 'JoaquinMatres', orientation: float = 0, pad_yspacing: float = 50, component_name: str | None = None, **kwargs) Component [source]#
Returns fiber array with label and electrical pads.
- Parameters:
component – to add fiber array and pads.
username – for the label.
orientation – for adding pads.
pad_yspacing – for adding pads.
component_name – for the label.
kwargs – for add_fiber_array.
import ubcpdk
c = ubcpdk.components.add_fiber_array_pads_rf(component='ring_single_heater', username='JoaquinMatres', orientation=0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
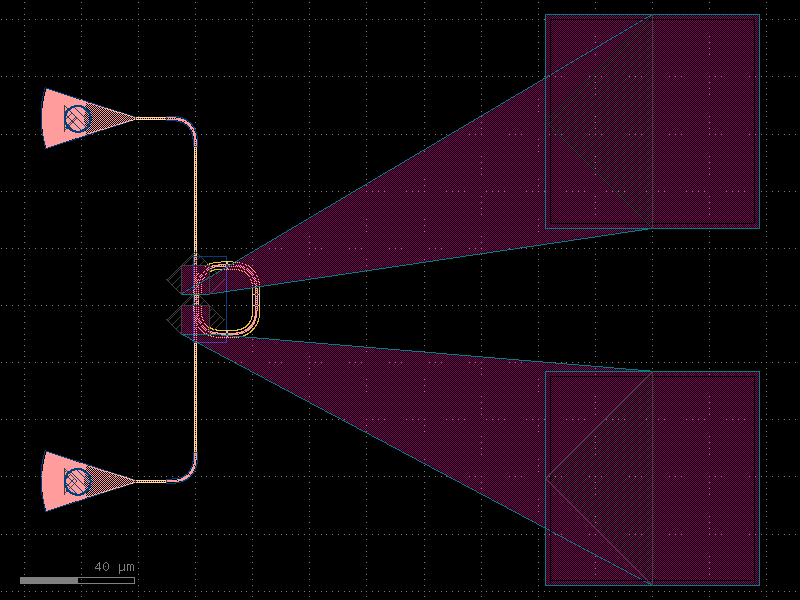
add_pads#
- ubcpdk.components.add_pads(component: str | Callable[[...], Component] | dict[str, Any] | KCell = 'ring_single_heater', username: str = 'JoaquinMatres', **kwargs) Component [source]#
Returns fiber array with label and electrical pads.
- Parameters:
component – to add fiber array and pads.
username – for the label.
kwargs – for add_fiber_array.
import ubcpdk
c = ubcpdk.components.add_pads(component='ring_single_heater', username='JoaquinMatres')
c.plot()
add_pads_dc#
import ubcpdk
c = ubcpdk.components.add_pads_dc(component='ring_single_heater', spacing=(0.0, 100.0))
c.plot()
add_pads_rf#
- ubcpdk.components.add_pads_rf(*, component: ComponentSpec = 'ring_single_heater', direction: str = 'top', spacing: Float2 = (0.0, 100.0), pad_array: ComponentSpec = 'pad_array', select_ports: Callable = functools.partial(<function select_ports>, port_type='electrical'), port_names: Strs | None = None, layer: LayerSpec = 'MTOP', **kwargs) Component #
Returns new component with electrical ports connected to top pad array.
- Parameters:
component – to route.
direction – sets direction of the array (top or right).
spacing – component to pad spacing.
pad_array – function for pad_array.
select_ports – function to select electrical ports.
port_names – optional port names. Overrides select_ports.
layer – for the routes.
kwargs – additional arguments.
- Keyword Arguments:
ports – Dict[str, Port] a port dict {port name: port}.
prefix – select ports with port name prefix.
suffix – select ports with port name suffix.
orientation – select ports with orientation in degrees.
width – select ports with port width.
layers_excluded – List of layers to exclude.
port_type – select ports with port type (optical, electrical, vertical_te).
clockwise – if True, sort ports clockwise, False: counter-clockwise.
import gdsfactory as gf c = gf.components.wire_straight(length=200.) cc = gf.routing.add_electrical_pads_top(component=c, spacing=(-150, 30)) cc.plot()
(
Source code
,png
,hires.png
,pdf
)
import ubcpdk
c = ubcpdk.components.add_pads_rf(component='ring_single_heater', direction='top', spacing=(0.0, 100.0), layer='MTOP')
c.plot()
(Source code
, png
, hires.png
, pdf
)
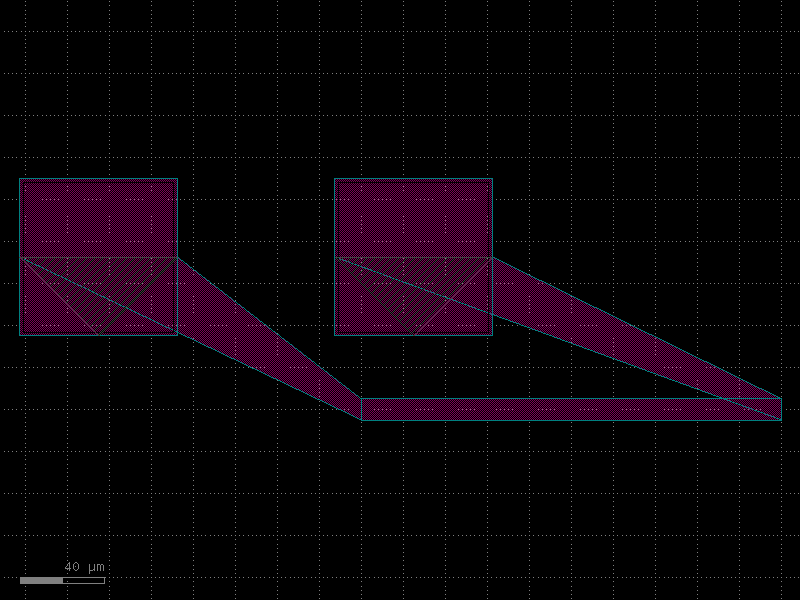
add_pins_bbox_siepic#
- ubcpdk.components.add_pins_bbox_siepic(component: ComponentSpec, *, function: Callable[..., None] = <function _add_pins_bbox_siepic>, **kwargs: Any) Component #
Returns new component with a component reference.
- Parameters:
component – to add to container.
function – function to apply to component.
kwargs – keyword arguments to pass to function.
import ubcpdk
c = ubcpdk.components.add_pins_bbox_siepic(port_type='optical', layer_pin=(1, 10), pin_length=0.01, bbox_layer=(68, 0), padding=0, remove_layers=False)
c.plot()
add_pins_bbox_siepic_remove_layers#
import ubcpdk
c = ubcpdk.components.add_pins_bbox_siepic_remove_layers(port_type='optical', layer_pin=(1, 10), pin_length=0.01, bbox_layer=(68, 0), padding=0, remove_layers=True)
c.plot()
add_pins_siepic_metal#
import ubcpdk
c = ubcpdk.components.add_pins_siepic_metal(port_type='placement', layer_pin=(1, 11), pin_length=0.01)
c.plot()
bend#
- ubcpdk.components.bend(cross_section='strip', **kwargs) Component #
import ubcpdk
c = ubcpdk.components.bend()
c.plot()
(Source code
, png
, hires.png
, pdf
)
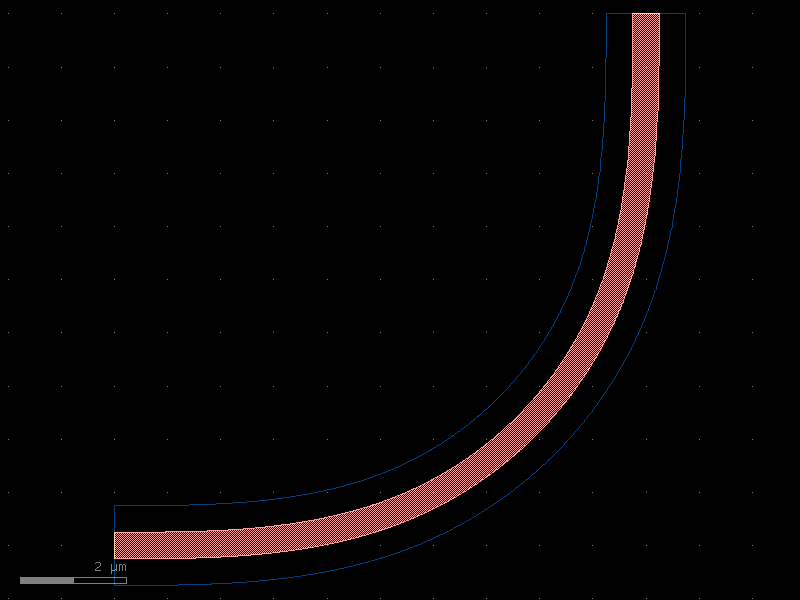
bend_euler180_sc#
import ubcpdk
c = ubcpdk.components.bend_euler180_sc()
c.plot()
bend_euler_sc#
import ubcpdk
c = ubcpdk.components.bend_euler_sc()
c.plot()
bend_s#
import ubcpdk
c = ubcpdk.components.bend_s(size=(11.0, 1.8), npoints=99, cross_section='xs_sc')
c.plot()
coupler#
import ubcpdk
c = ubcpdk.components.coupler(gap=0.236, length=20.0, dy=4.0, dx=10.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
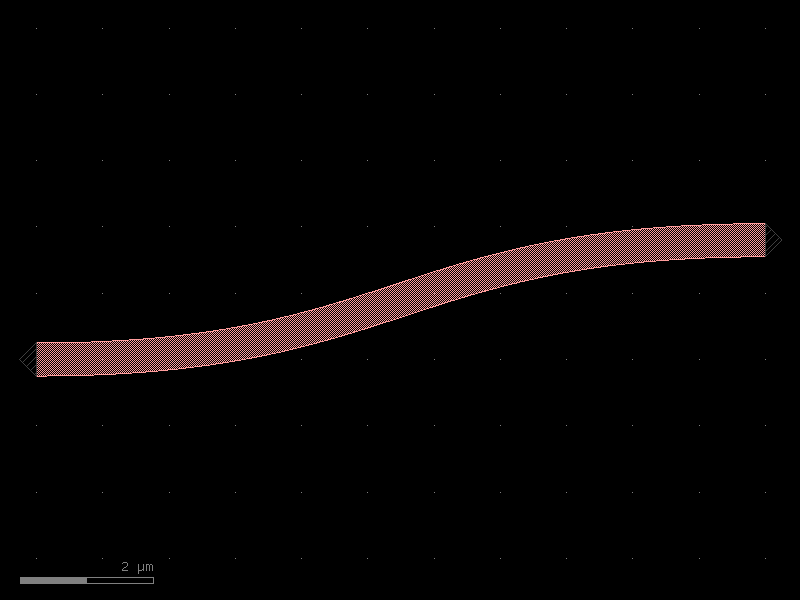
coupler_ring#
- ubcpdk.components.coupler_ring(gap: float = 0.2, radius: float = 10.0, length_x: float = 4.0, length_extension: float = 3, bend=<function bend_euler>, cross_section='strip', **kwargs) Component [source]#
import ubcpdk
c = ubcpdk.components.coupler_ring(gap=0.2, radius=5.0, length_x=4.0, length_extension=3)
c.plot()
(Source code
, png
, hires.png
, pdf
)
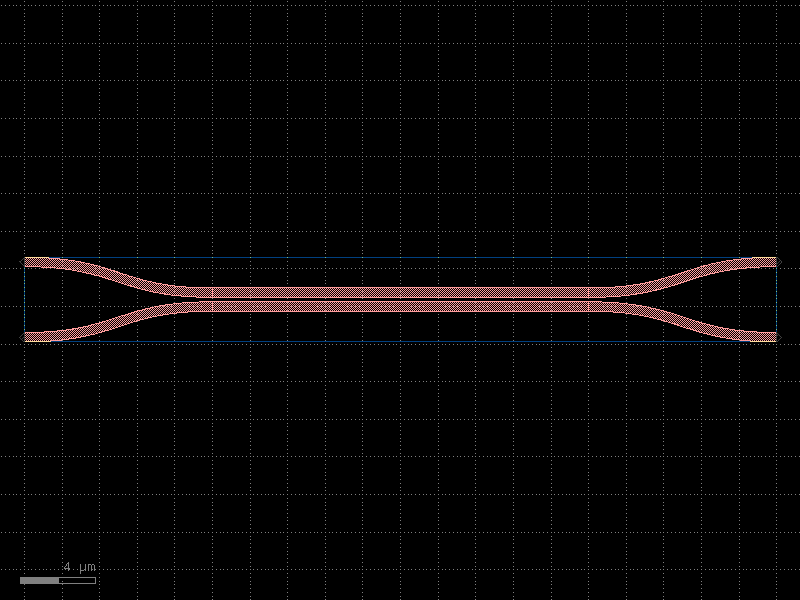
dbg#
- ubcpdk.components.dbg(w0: float = 0.5, dw: float = 0.1, n: int = 100, l1: float = 0.07940573770491803, l2: float = 0.07940573770491803) Component [source]#
Includes two ports.
- Parameters:
w0 – width.
dw – delta width.
n – number of elements.
l1 – length teeth1.
l2 – length teeth2.
import ubcpdk
c = ubcpdk.components.dbg(w0=0.5, dw=0.1, n=100, l1=0.07940573770491803, l2=0.07940573770491803)
c.plot()
dbr#
- ubcpdk.components.dbr(w0: float = 0.5, dw: float = 0.1, n: int = 100, l1: float = 0.07940573770491803, l2: float = 0.07940573770491803, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'strip', **kwargs) Component [source]#
Returns distributed bragg reflector.
- Parameters:
w0 – width.
dw – delta width.
n – number of elements.
l1 – length teeth1.
l2 – length teeth2.
cross_section – spec.
kwargs – cross_section settings.
import ubcpdk
c = ubcpdk.components.dbr(w0=0.5, dw=0.1, n=100, l1=0.07940573770491803, l2=0.07940573770491803)
c.plot()
(Source code
, png
, hires.png
, pdf
)
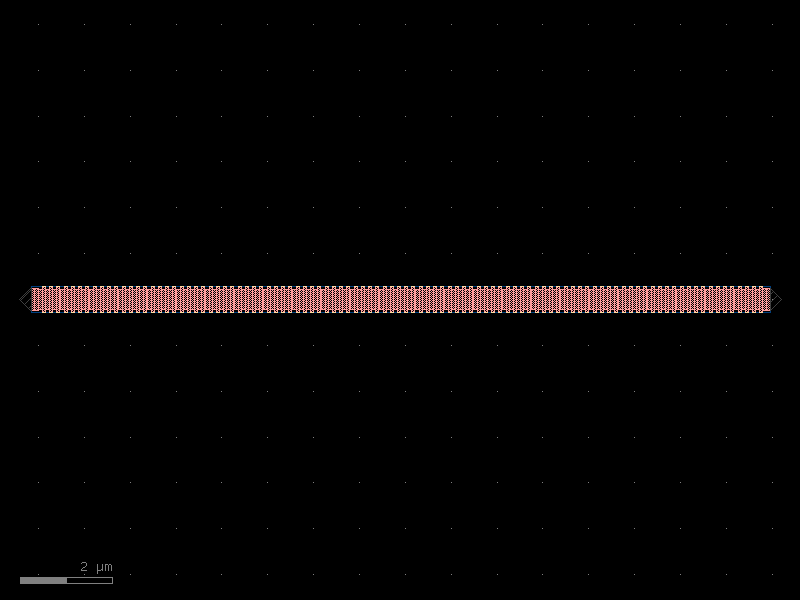
dbr_cavity#
import ubcpdk
c = ubcpdk.components.dbr_cavity()
c.plot()
(Source code
, png
, hires.png
, pdf
)
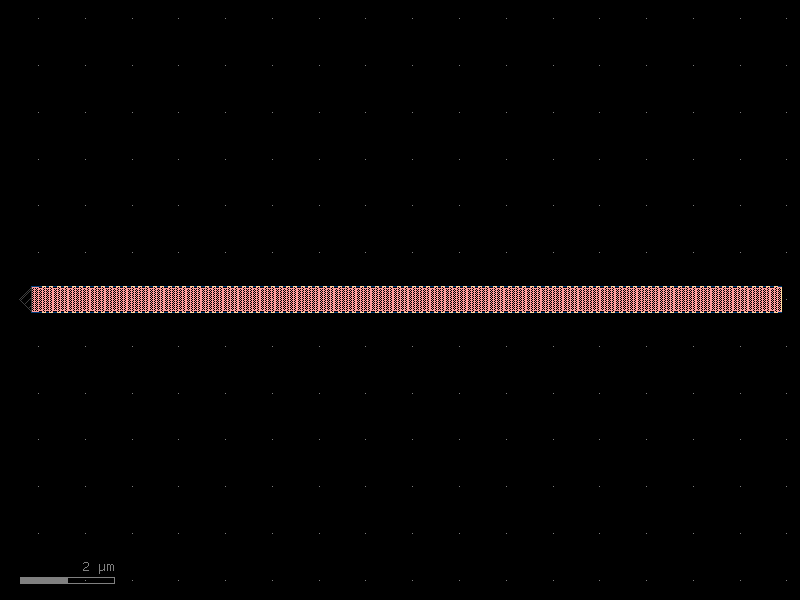
dbr_cavity_te#
import ubcpdk
c = ubcpdk.components.dbr_cavity_te(component='dbr_cavity')
c.plot()
(Source code
, png
, hires.png
, pdf
)
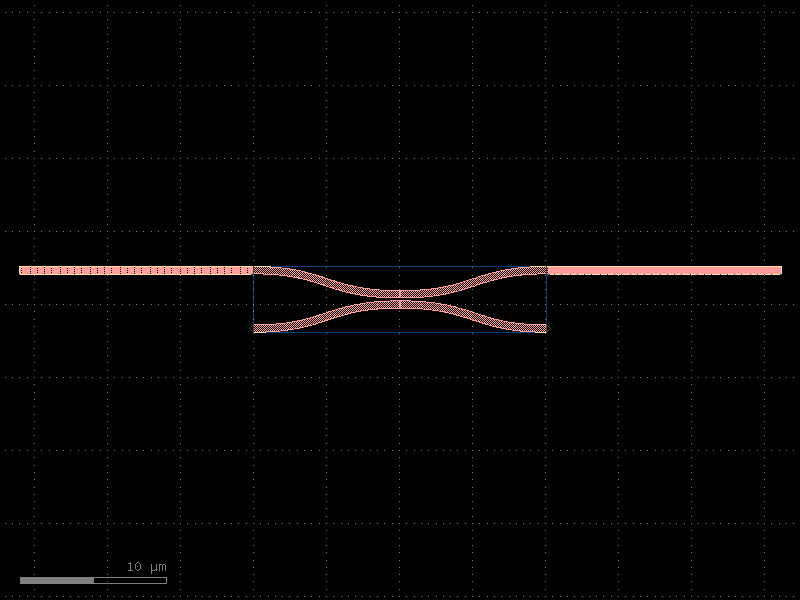
ebeam_BondPad#
import ubcpdk
c = ubcpdk.components.ebeam_BondPad()
c.plot()
(Source code
, png
, hires.png
, pdf
)
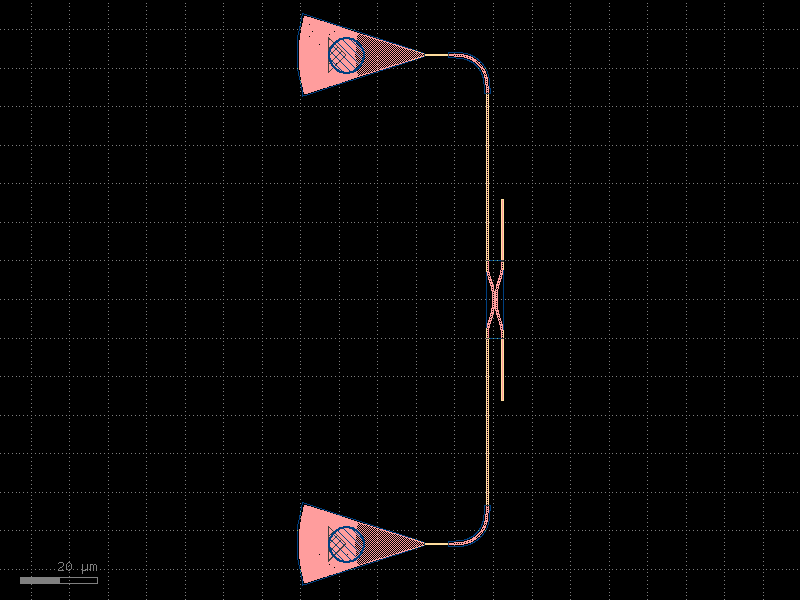
ebeam_adiabatic_te1550#
- ubcpdk.components.ebeam_adiabatic_te1550() Component [source]#
Return ebeam_adiabatic_te1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_adiabatic_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
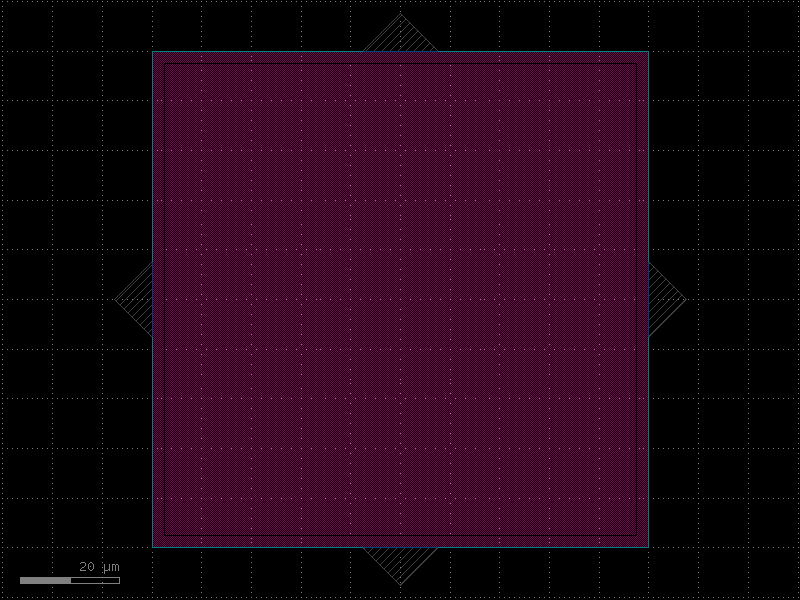
ebeam_adiabatic_tm1550#
- ubcpdk.components.ebeam_adiabatic_tm1550() Component [source]#
Return ebeam_adiabatic_tm1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_adiabatic_tm1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
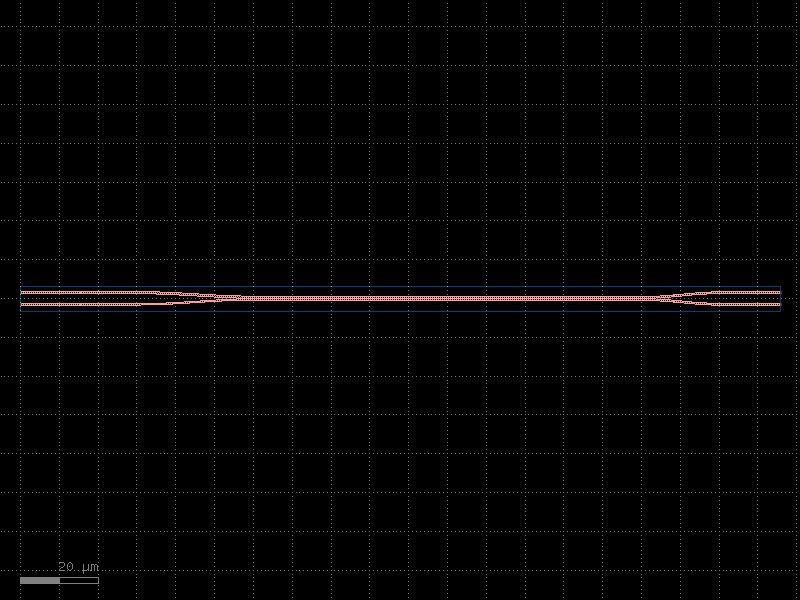
ebeam_bdc_te1550#
import ubcpdk
c = ubcpdk.components.ebeam_bdc_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
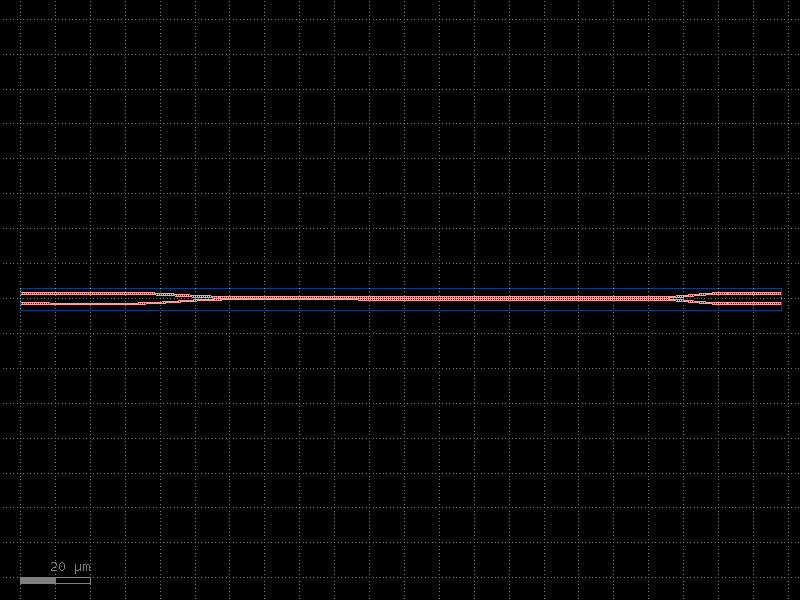
ebeam_bdc_tm1550#
import ubcpdk
c = ubcpdk.components.ebeam_bdc_tm1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
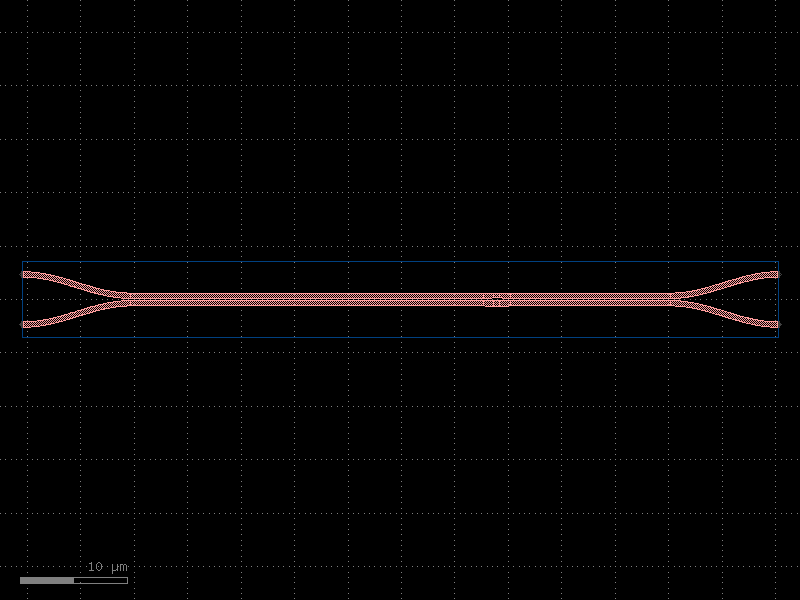
ebeam_crossing4#
import ubcpdk
c = ubcpdk.components.ebeam_crossing4()
c.plot()
(Source code
, png
, hires.png
, pdf
)
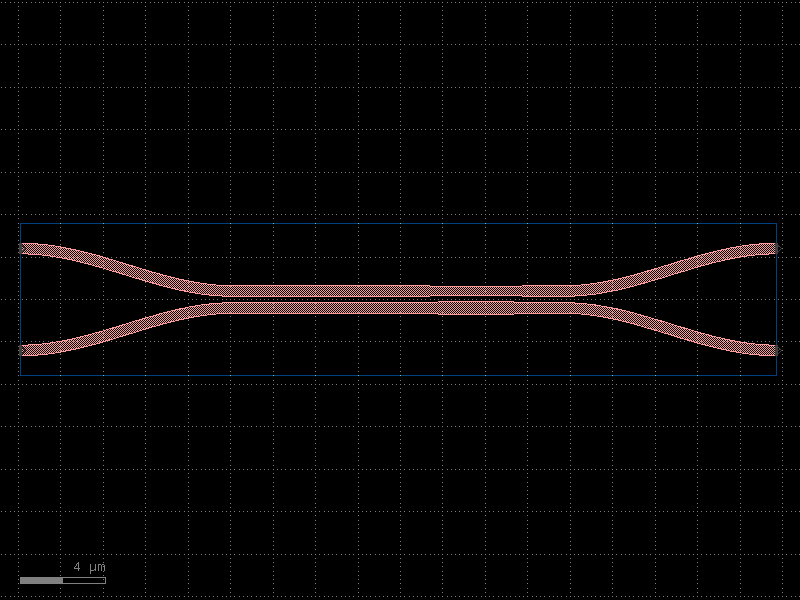
ebeam_crossing4_2ports#
import ubcpdk
c = ubcpdk.components.ebeam_crossing4_2ports()
c.plot()
(Source code
, png
, hires.png
, pdf
)
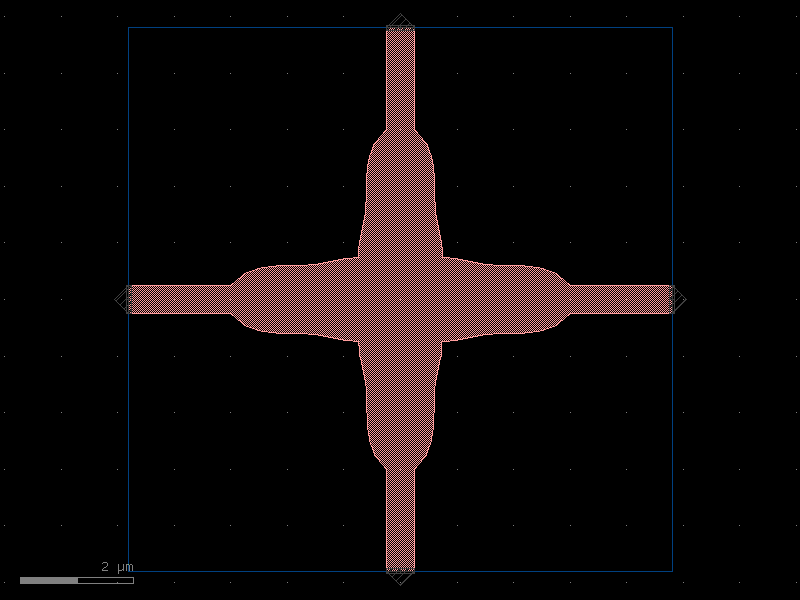
ebeam_dc_halfring_straight#
import ubcpdk
c = ubcpdk.components.ebeam_dc_halfring_straight(gap=0.2, radius=5.0, length_x=4.0, siepic=True, model='ebeam_dc_halfring_straight')
c.plot()
ebeam_dc_te1550#
- ubcpdk.components.ebeam_dc_te1550(gap: float = 0.236, length: float = 20.0, dy: float = 4.0, dx: float = 10.0, cross_section: CrossSectionSpec = 'strip', allow_min_radius_violation: bool = False) Component #
Symmetric coupler.
- Parameters:
gap – between straights in um.
length – of coupling region in um.
dy – port to port vertical spacing in um.
dx – length of bend in x direction in um.
cross_section – spec (CrossSection, string or dict).
allow_min_radius_violation – if True does not check for min bend radius.
dx dx |------| |------| o2 ________ ______o3 \ / | \ length / | ======================= gap | dy / \ | ________/ \_______ | o1 o4 coupler_straight coupler_symmetric
import ubcpdk
c = ubcpdk.components.ebeam_dc_te1550(gap=0.236, length=20.0, dy=4.0, dx=10.0, cross_section='xs_sc')
c.plot()
ebeam_splitter_adiabatic_swg_te1550#
- ubcpdk.components.ebeam_splitter_adiabatic_swg_te1550() Component [source]#
Return ebeam_splitter_adiabatic_swg_te1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_splitter_adiabatic_swg_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
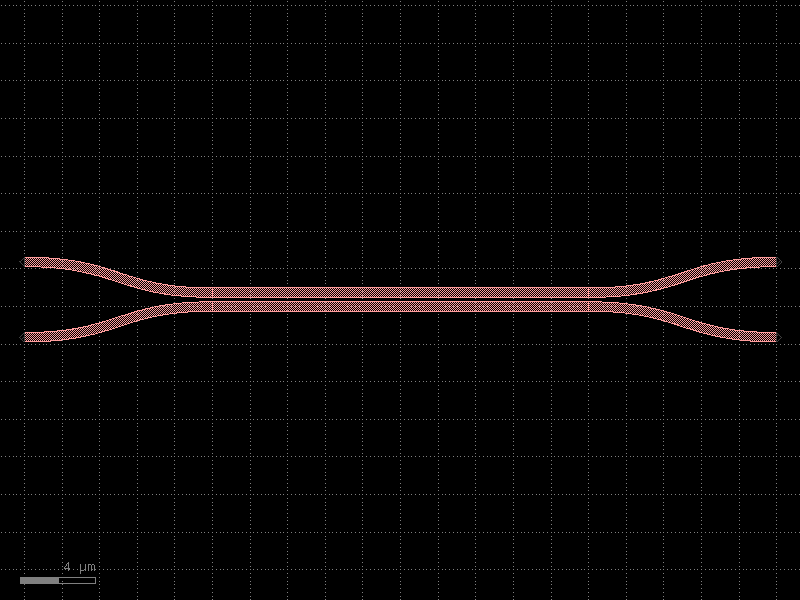
ebeam_splitter_swg_assist_te1310#
- ubcpdk.components.ebeam_splitter_swg_assist_te1310() Component [source]#
Return ebeam_splitter_swg_assist_te1310 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_splitter_swg_assist_te1310()
c.plot()
(Source code
, png
, hires.png
, pdf
)
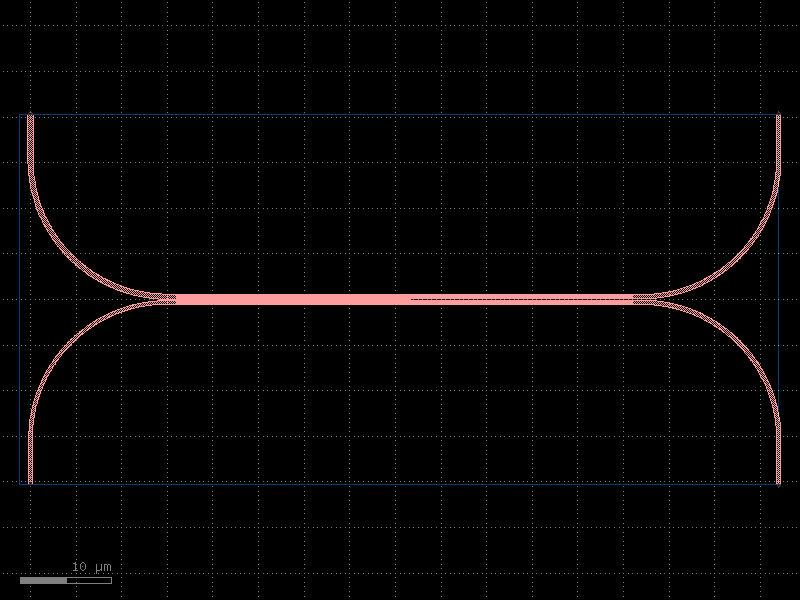
ebeam_splitter_swg_assist_te1550#
- ubcpdk.components.ebeam_splitter_swg_assist_te1550() Component [source]#
Return ebeam_splitter_swg_assist_te1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_splitter_swg_assist_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
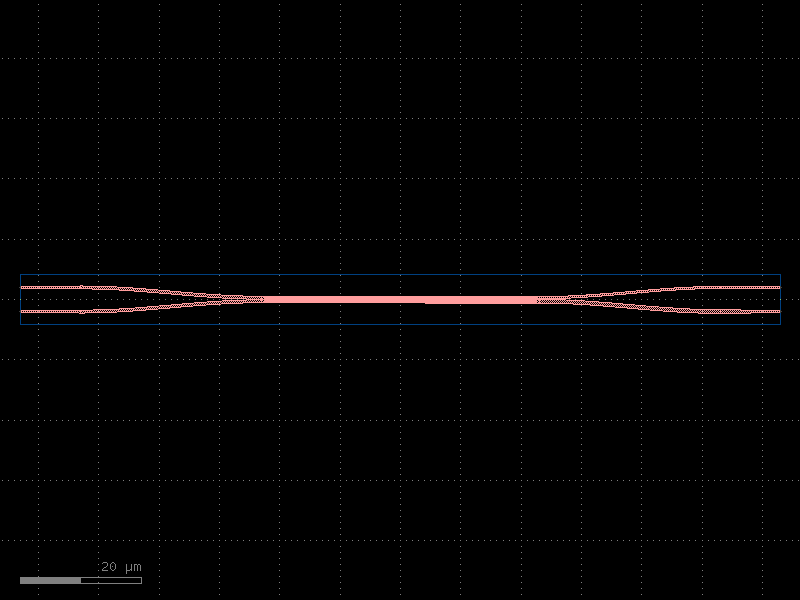
ebeam_swg_edgecoupler#
- ubcpdk.components.ebeam_swg_edgecoupler() Component [source]#
Return ebeam_swg_edgecoupler fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_swg_edgecoupler()
c.plot()
(Source code
, png
, hires.png
, pdf
)
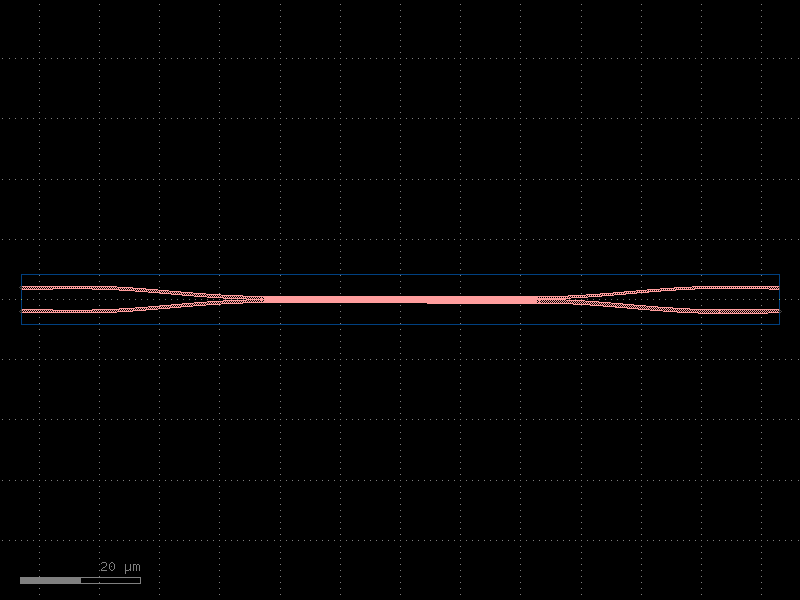
ebeam_terminator_te1310#
- ubcpdk.components.ebeam_terminator_te1310() Component [source]#
Return ebeam_terminator_te1310 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_terminator_te1310()
c.plot()
(Source code
, png
, hires.png
, pdf
)
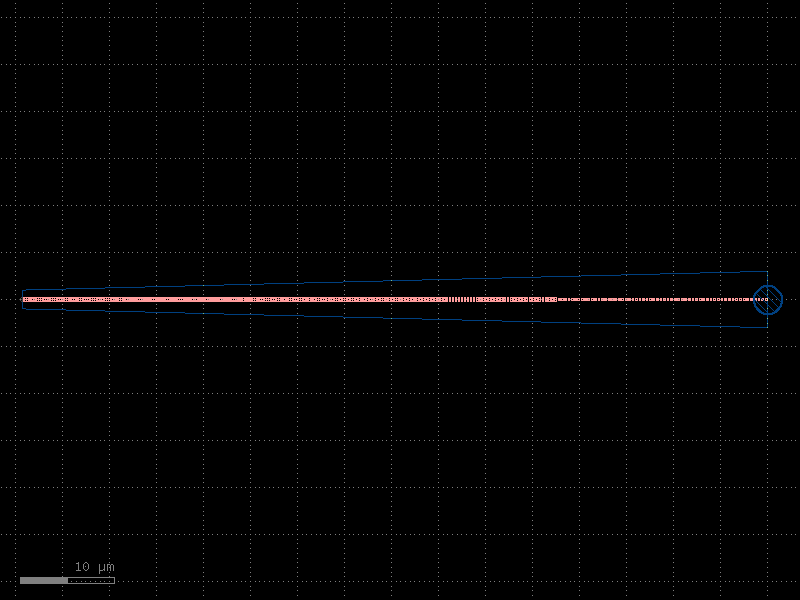
ebeam_terminator_te1550#
- ubcpdk.components.ebeam_terminator_te1550() Component [source]#
Return ebeam_terminator_te1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_terminator_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
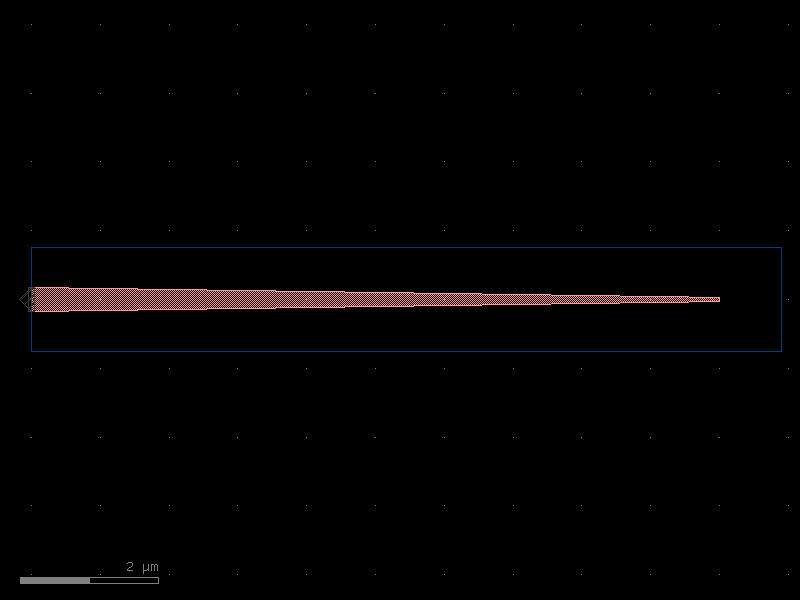
ebeam_terminator_tm1550#
- ubcpdk.components.ebeam_terminator_tm1550() Component [source]#
Return ebeam_terminator_tm1550 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_terminator_tm1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
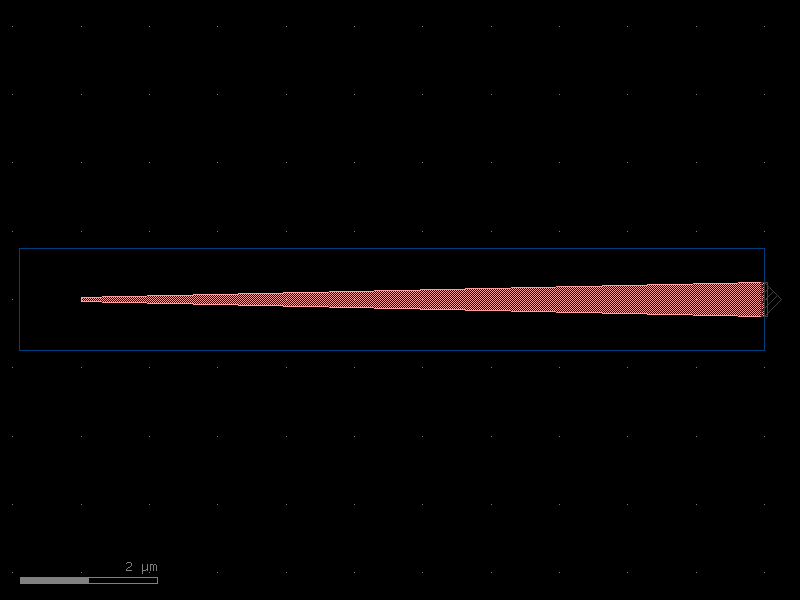
ebeam_y_1550#
import ubcpdk
c = ubcpdk.components.ebeam_y_1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
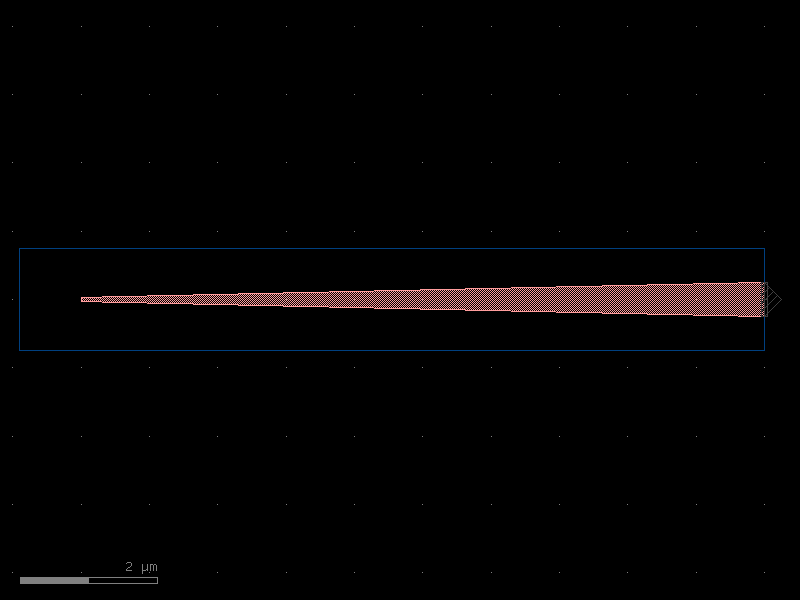
ebeam_y_adiabatic#
import ubcpdk
c = ubcpdk.components.ebeam_y_adiabatic()
c.plot()
(Source code
, png
, hires.png
, pdf
)
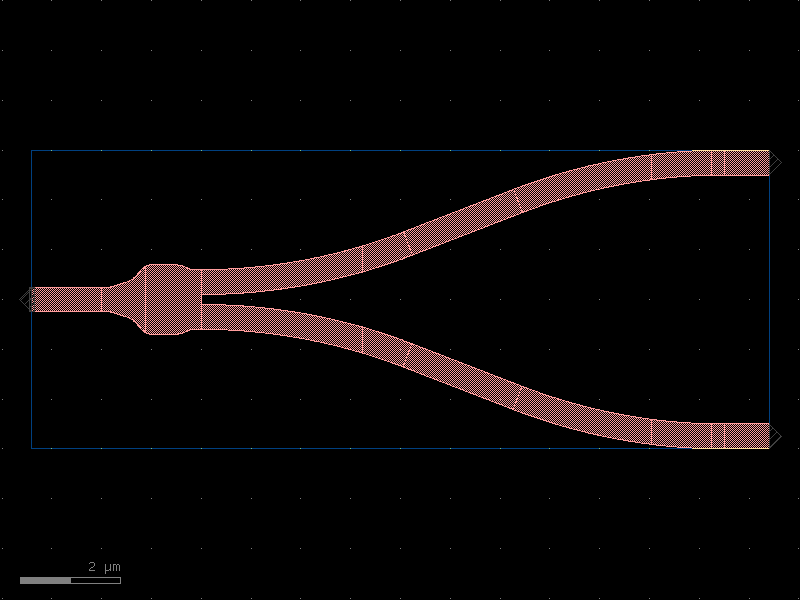
ebeam_y_adiabatic_1310#
- ubcpdk.components.ebeam_y_adiabatic_1310() Component [source]#
Return ebeam_y_adiabatic_1310 fixed cell.
import ubcpdk
c = ubcpdk.components.ebeam_y_adiabatic_1310()
c.plot()
(Source code
, png
, hires.png
, pdf
)
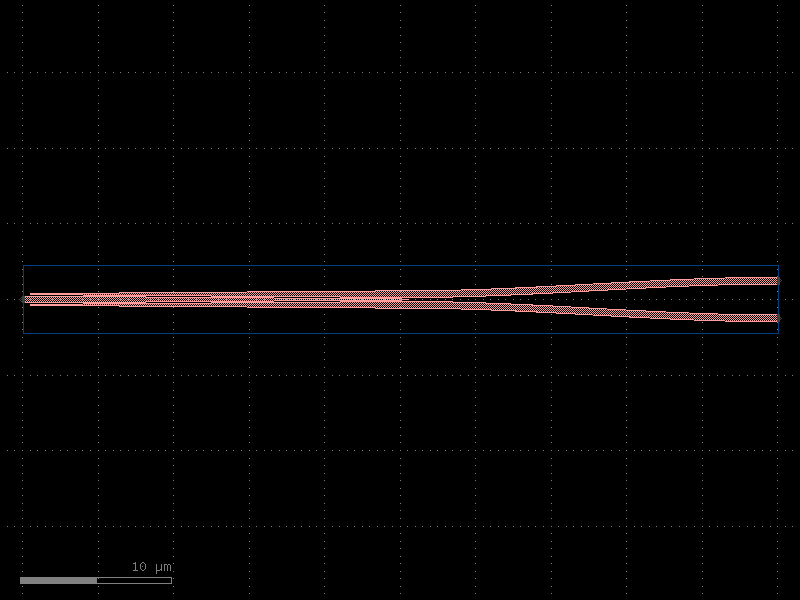
ebeam_y_adiabatic_tapers#
import ubcpdk
c = ubcpdk.components.ebeam_y_adiabatic_tapers()
c.plot()
gc_te1310#
import ubcpdk
c = ubcpdk.components.gc_te1310()
c.plot()
(Source code
, png
, hires.png
, pdf
)
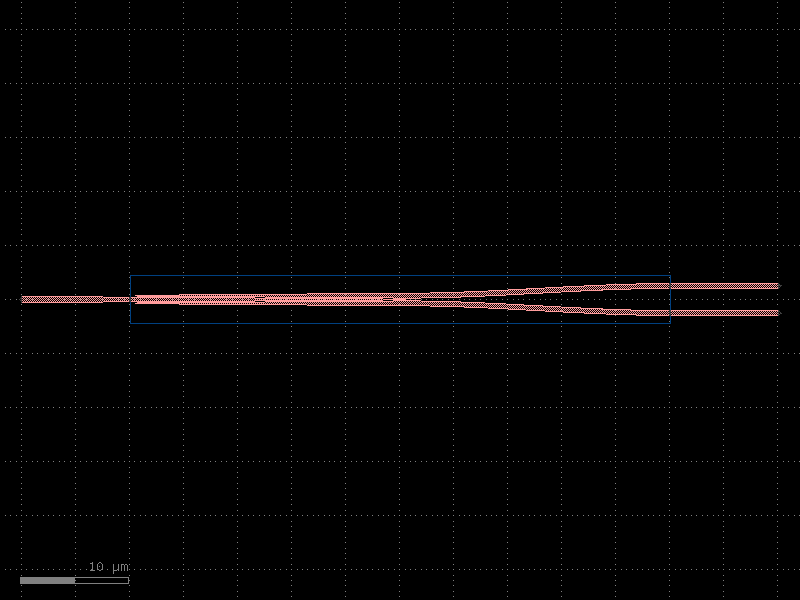
gc_te1310_8deg#
import ubcpdk
c = ubcpdk.components.gc_te1310_8deg()
c.plot()
(Source code
, png
, hires.png
, pdf
)
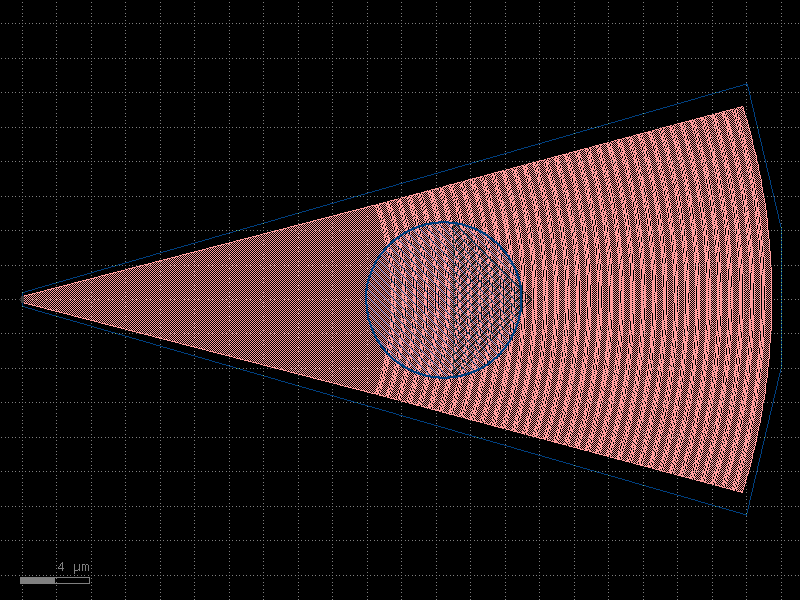
gc_te1310_broadband#
- ubcpdk.components.gc_te1310_broadband() Component [source]#
Return ebeam_gc_te1310_broadband fixed cell.
import ubcpdk
c = ubcpdk.components.gc_te1310_broadband()
c.plot()
(Source code
, png
, hires.png
, pdf
)
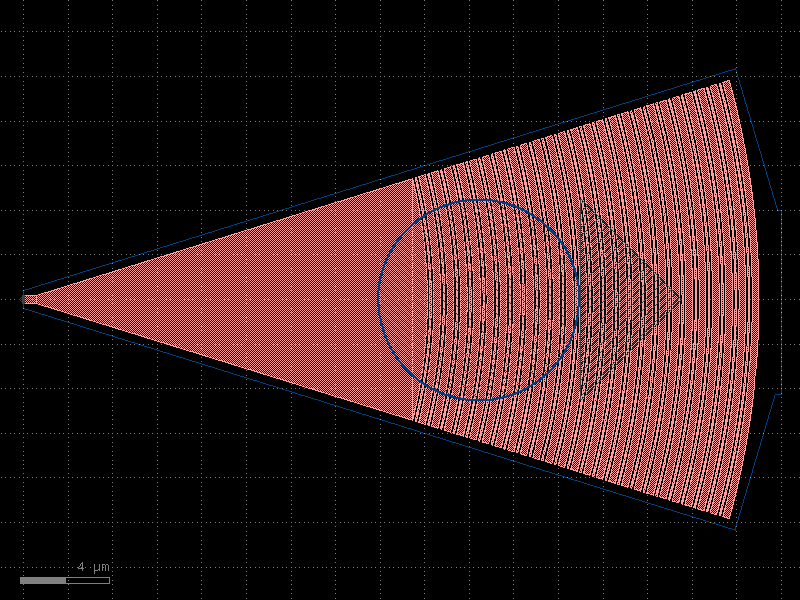
gc_te1550#
import ubcpdk
c = ubcpdk.components.gc_te1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
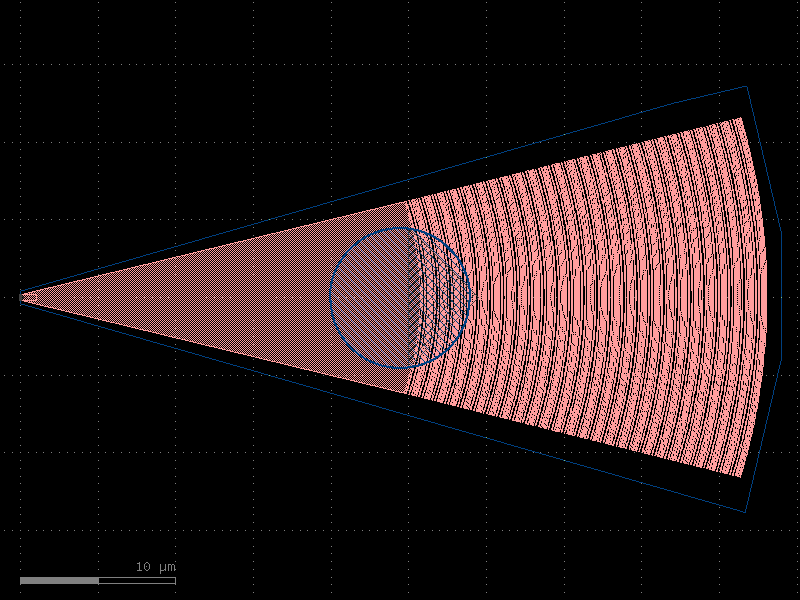
gc_te1550_90nmSlab#
- ubcpdk.components.gc_te1550_90nmSlab() Component [source]#
Return ebeam_gc_te1550_90nmSlab fixed cell.
import ubcpdk
c = ubcpdk.components.gc_te1550_90nmSlab()
c.plot()
(Source code
, png
, hires.png
, pdf
)
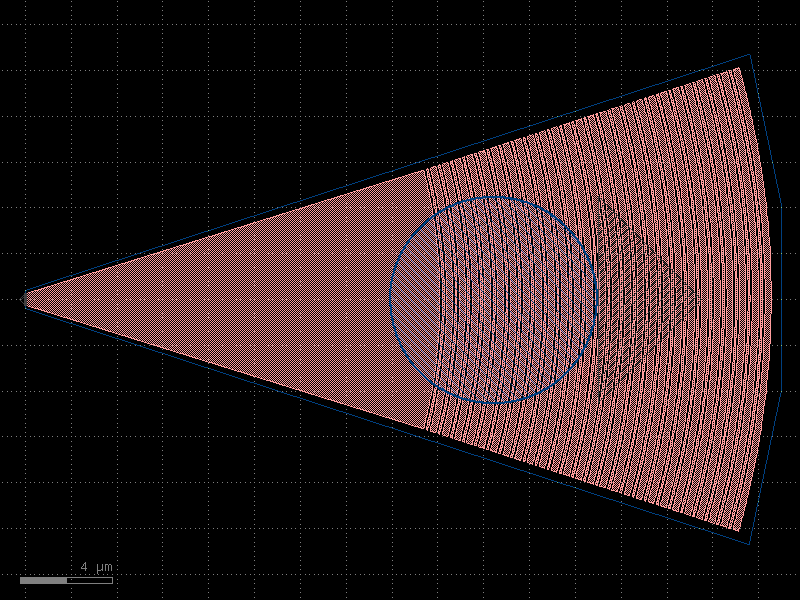
gc_te1550_broadband#
- ubcpdk.components.gc_te1550_broadband() Component [source]#
Return ebeam_gc_te1550_broadband fixed cell.
import ubcpdk
c = ubcpdk.components.gc_te1550_broadband()
c.plot()
(Source code
, png
, hires.png
, pdf
)
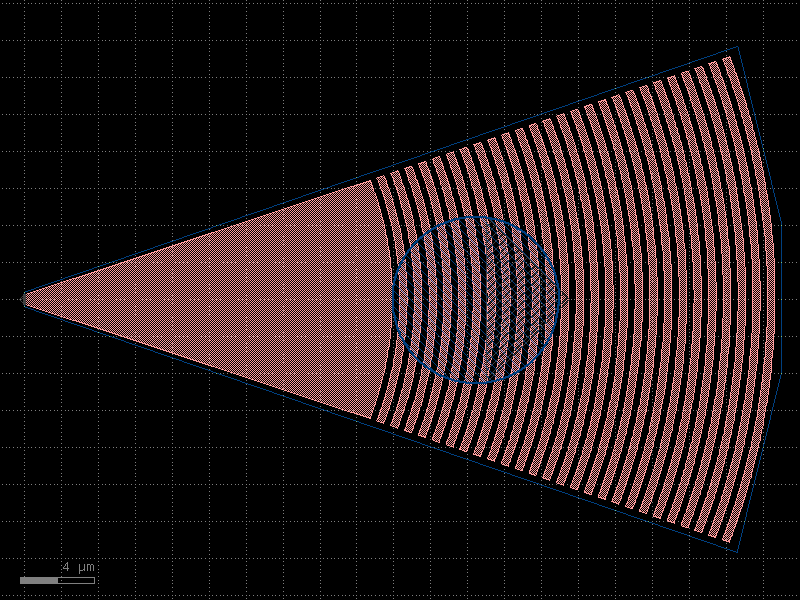
gc_tm1550#
import ubcpdk
c = ubcpdk.components.gc_tm1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
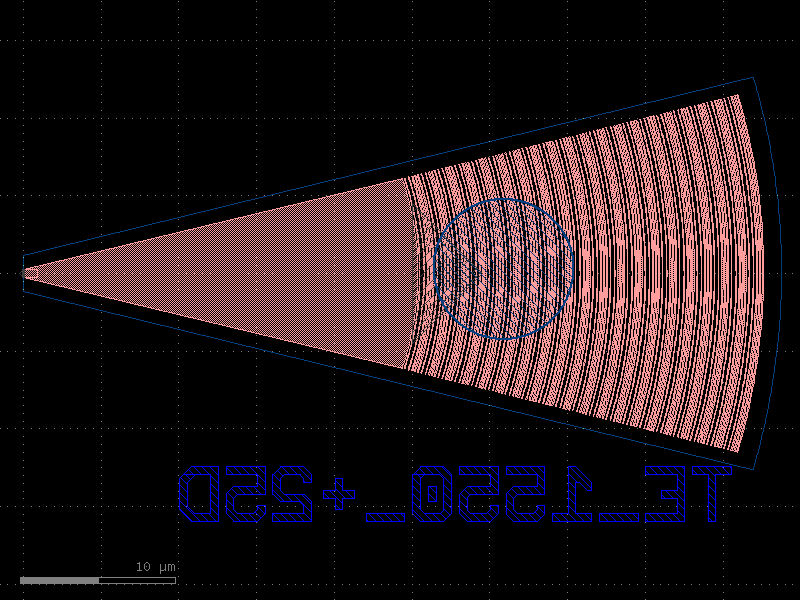
metal_via#
import ubcpdk
c = ubcpdk.components.metal_via()
c.plot()
(Source code
, png
, hires.png
, pdf
)
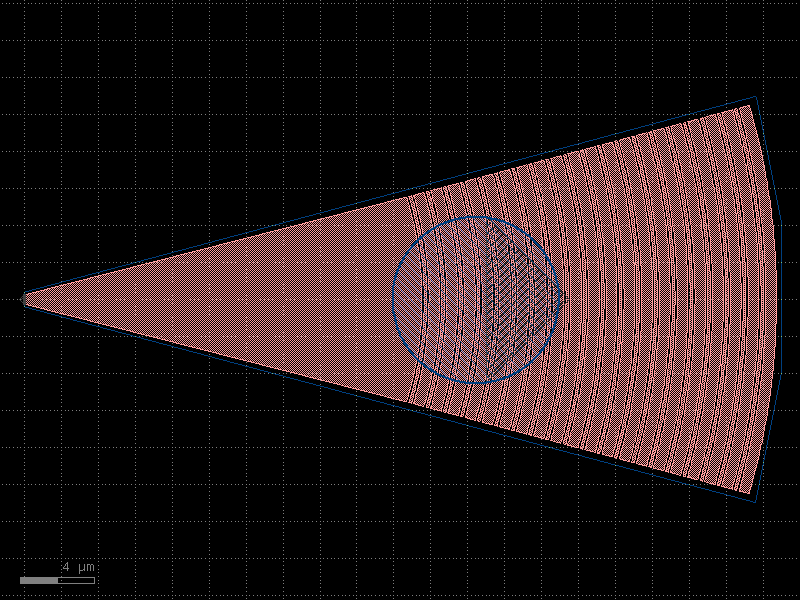
mmi1x2#
import ubcpdk
c = ubcpdk.components.mmi1x2(width_taper=1.0, length_taper=10.0, length_mmi=5.5, width_mmi=2.5, gap_mmi=0.25)
c.plot()
(Source code
, png
, hires.png
, pdf
)
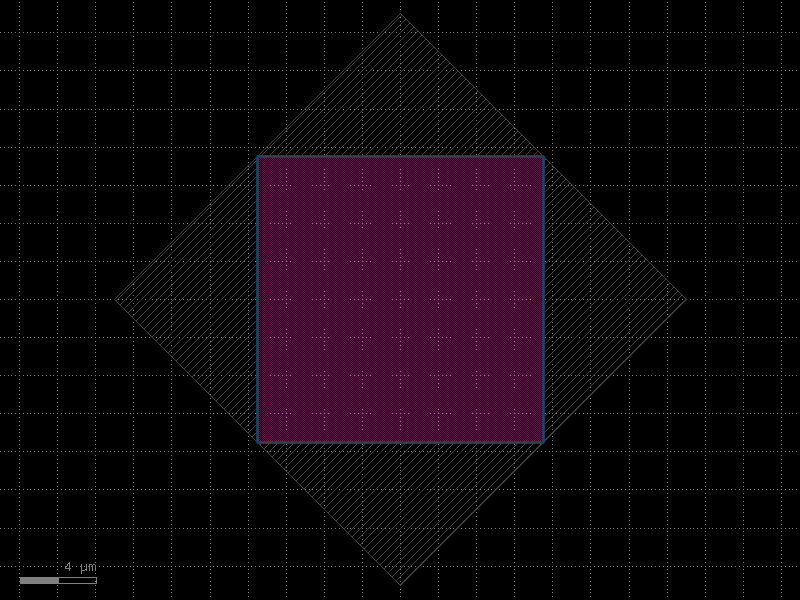
mzi#
- ubcpdk.components.mzi(delta_length: float = 10.0, length_y: float = 2.0, length_x: float | None = 0.1, *, bend: ComponentSpec = <function bend_euler>, straight: ComponentSpec = 'straight', straight_y: ComponentSpec | None = None, straight_x_top: ComponentSpec | None = None, straight_x_bot: ComponentSpec | None = None, splitter: ComponentSpec = <function ebeam_y_1550>, combiner: ComponentSpec | None = None, with_splitter: bool = True, port_e1_splitter: str = 'o2', port_e0_splitter: str = 'o3', port_e1_combiner: str = 'o2', port_e0_combiner: str = 'o3', port1: str = 'o1', port2: str = 'o2', nbends: int = 2, cross_section: CrossSectionSpec = 'strip', cross_section_x_top: CrossSectionSpec | None = None, cross_section_x_bot: CrossSectionSpec | None = None, mirror_bot: bool = False, add_optical_ports_arms: bool = False, min_length: float = 0.01, auto_rename_ports: bool = True) Component #
Mzi.
- Parameters:
delta_length – bottom arm vertical extra length.
length_y – vertical length for both and top arms.
length_x – horizontal length. None uses to the straight_x_bot/top defaults.
bend – 90 degrees bend library.
straight – straight function.
straight_y – straight for length_y and delta_length.
straight_x_top – top straight for length_x.
straight_x_bot – bottom straight for length_x.
splitter – splitter function.
combiner – combiner function.
with_splitter – if False removes splitter.
port_e1_splitter – east top splitter port.
port_e0_splitter – east bot splitter port.
port_e1_combiner – east top combiner port.
port_e0_combiner – east bot combiner port.
port1 – input port name.
port2 – output port name.
nbends – from straight top/bot to combiner (at least 2).
cross_section – for routing (sxtop/sxbot to combiner).
cross_section_x_top – optional top cross_section (defaults to cross_section).
cross_section_x_bot – optional bottom cross_section (defaults to cross_section).
mirror_bot – if true, mirrors the bottom arm.
add_optical_ports_arms – add all other optical ports in the arms with top_ and bot_ prefix.
min_length – minimum length for the straight.
auto_rename_ports – if True, renames ports.
b2______b3 | sxtop | straight_y | | | b1 b4 splitter==| |==combiner b5 b8 | | straight_y | | | delta_length/2 | | | b6__sxbot__b7 Lx
import ubcpdk
c = ubcpdk.components.mzi(delta_length=10.0, length_y=2.0, length_x=0.1, with_splitter=True, port_e1_splitter='o2', port_e0_splitter='o3', port_e1_combiner='o2', port_e0_combiner='o3', nbends=2, cross_section='xs_sc', mirror_bot=False, add_optical_ports_arms=False, add_electrical_ports_bot=True)
c.plot()
mzi_heater#
- ubcpdk.components.mzi_heater(delta_length=10.0, length_x=320) Component [source]#
Returns MZI with heater.
- Parameters:
delta_length – extra length for mzi arms.
import ubcpdk
c = ubcpdk.components.mzi_heater(delta_length=10.0, length_y=2.0, length_x=200, straight_x_top='straight_heater_metal', with_splitter=True, port_e1_splitter='o2', port_e0_splitter='o3', port_e1_combiner='o2', port_e0_combiner='o3', nbends=2, cross_section='xs_sc', mirror_bot=False, add_optical_ports_arms=False, add_electrical_ports_bot=True)
c.plot()
pad#
- ubcpdk.components.pad(*, size: str | Float2 = (75, 75), layer: LayerSpec = <LayerMapUbc.M2_ROUTER: 47>, bbox_layers: tuple[LayerSpec, ...] | None = (<LayerMapUbc.PAD_OPEN: 48>, ), bbox_offsets: tuple[float, ...] | None = (-1.8, ), port_inclusion: float = 0, port_orientation: float | None = 0, port_orientations: Ints | None = (180, 90, 0, -90)) Component #
Returns rectangular pad with ports.
- Parameters:
size – x, y size.
layer – pad layer.
bbox_layers – list of layers.
bbox_offsets – Optional offsets for each layer with respect to size. positive grows, negative shrinks the size.
port_inclusion – from edge.
port_orientation – in degrees.
port_orientations – list of port_orientations to add. None does not add ports.
import ubcpdk
c = ubcpdk.components.pad(size=(75, 75), layer=(12, 0), bbox_layers=((13, 0),), bbox_offsets=(-1.8,), port_inclusion=0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
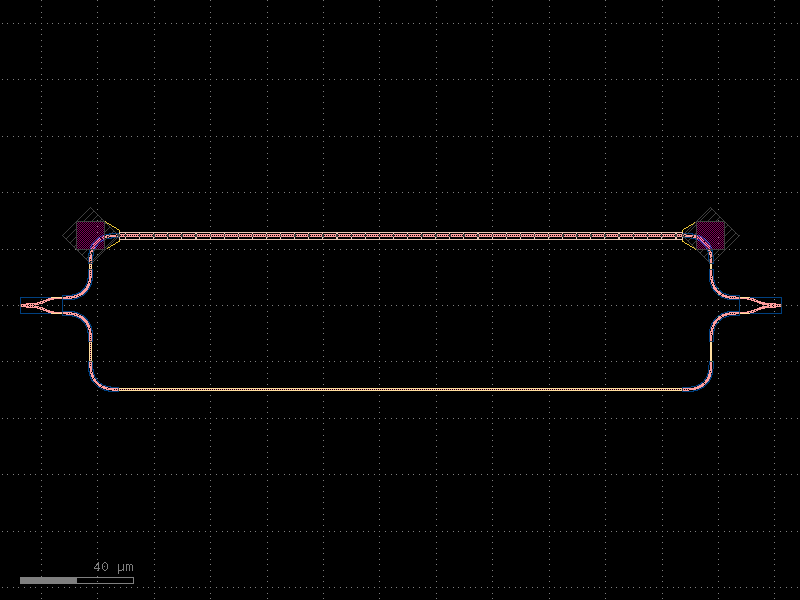
pad_array#
- ubcpdk.components.pad_array(*, pad: ComponentFactory = functools.partial(<function pad>, size=(75, 75), layer=<LayerMapUbc.M2_ROUTER: 47>, bbox_layers=(<LayerMapUbc.PAD_OPEN: 48>, ), bbox_offsets=(-1.8, )), spacing: tuple[float, float] = (125, 125), columns: int = 6, rows: int = 1, port_orientation: float = 0, orientation: float | None = None, size: Float2 = (100.0, 100.0), layer: LayerSpec = 'MTOP', centered_ports: bool = False) Component #
Returns 2D array of pads.
- Parameters:
pad – pad element.
spacing – x, y pitch.
columns – number of columns.
rows – number of rows.
port_orientation – port orientation in deg. None for low speed DC ports.
orientation – Deprecated, use port_orientation.
size – pad size.
layer – pad layer.
centered_ports – True add ports to center. False add ports to the edge.
import ubcpdk
c = ubcpdk.components.pad_array(spacing=(125, 125), columns=6, rows=1, orientation=270)
c.plot()
(Source code
, png
, hires.png
, pdf
)
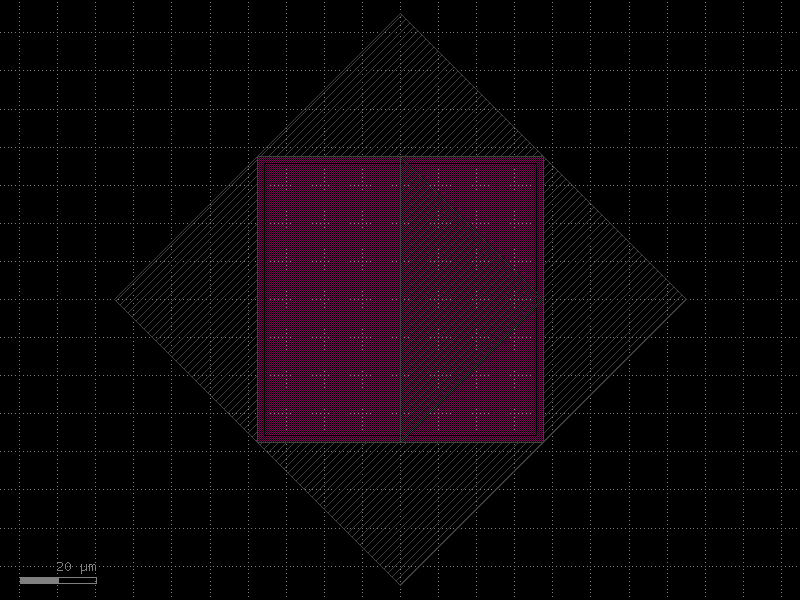
photonic_wirebond_surfacetaper_1310#
- ubcpdk.components.photonic_wirebond_surfacetaper_1310() Component [source]#
Return photonic_wirebond_surfacetaper_1310 fixed cell.
import ubcpdk
c = ubcpdk.components.photonic_wirebond_surfacetaper_1310()
c.plot()
(Source code
, png
, hires.png
, pdf
)
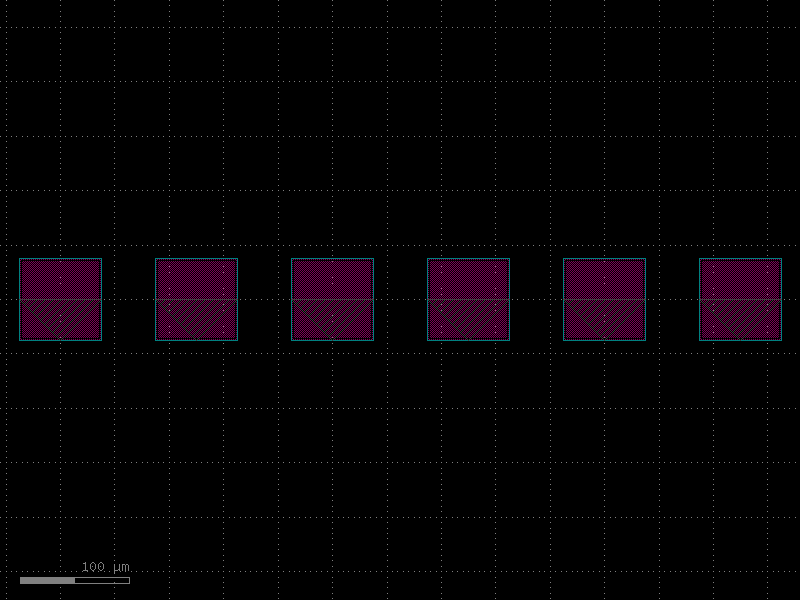
photonic_wirebond_surfacetaper_1550#
- ubcpdk.components.photonic_wirebond_surfacetaper_1550() Component [source]#
Return photonic_wirebond_surfacetaper_1550 fixed cell.
import ubcpdk
c = ubcpdk.components.photonic_wirebond_surfacetaper_1550()
c.plot()
(Source code
, png
, hires.png
, pdf
)
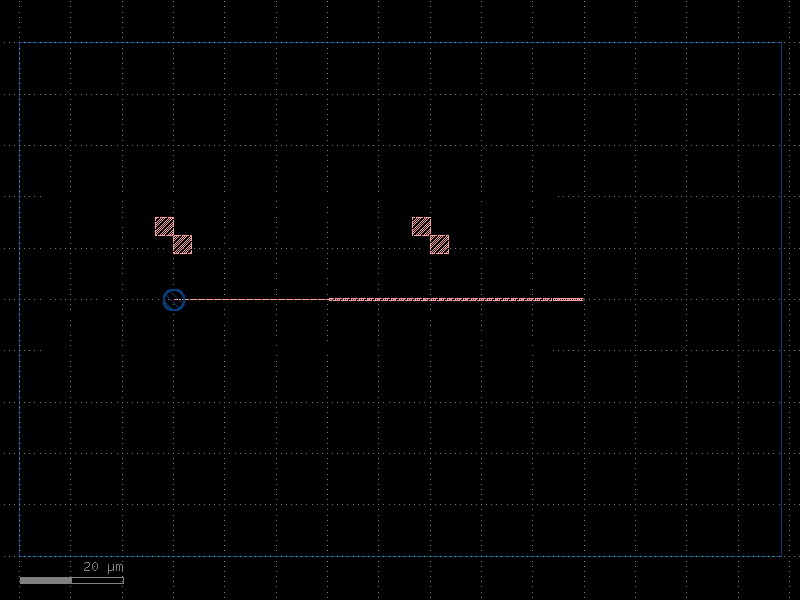
ring_double#
- ubcpdk.components.ring_double(gap: float = 0.2, radius: float = 10.0, length_x: float = 4.0, length_y: float = 0.6) Component [source]#
import ubcpdk
c = ubcpdk.components.ring_double(gap=0.2, radius=10.0, length_x=0.01, length_y=0.01)
c.plot()
(Source code
, png
, hires.png
, pdf
)
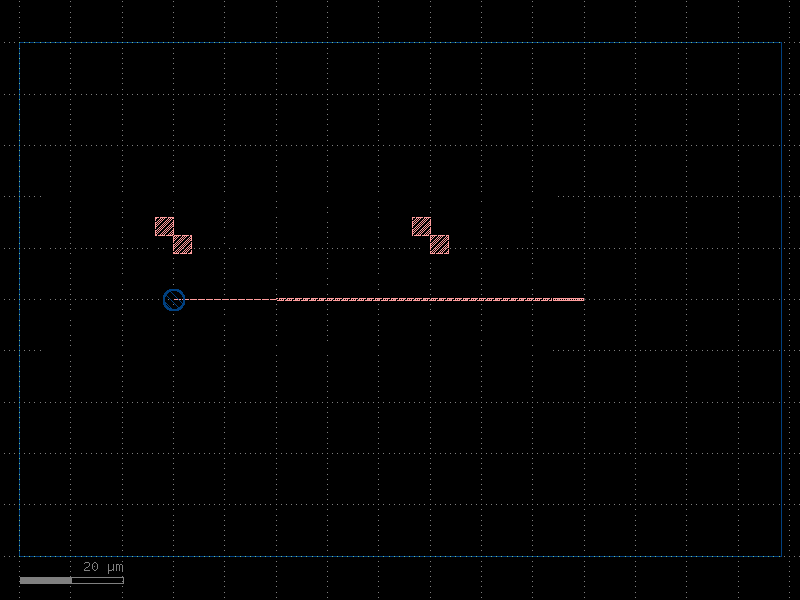
ring_double_heater#
- ubcpdk.components.ring_double_heater(gap: float = 0.2, radius: float = 10.0, length_x: float = 1.0, *, length_y: float = 0.2, coupler_ring: ComponentSpec = <function coupler_ring>, coupler_ring_top: ComponentSpec | None = None, straight: ComponentSpec = <function straight>, bend: ComponentSpec = 'bend_euler', cross_section_heater: CrossSectionSpec = 'heater_metal', cross_section_waveguide_heater: CrossSectionSpec = 'strip_heater_metal', cross_section: CrossSectionSpec = 'strip', via_stack: ComponentSpec = 'via_stack_heater_mtop', port_orientation: float | None = None, via_stack_offset: Float2 = (1, 0), with_drop: bool = True) Component #
Returns a double bus ring with heater on top.
two couplers (ct: top, cb: bottom) connected with two vertical straights (sl: left, sr: right)
- Parameters:
gap – gap between for coupler.
radius – for the bend and coupler.
length_x – ring coupler length.
length_y – vertical straight length.
coupler_ring – ring coupler spec.
coupler_ring_top – ring coupler spec for coupler away from vias (defaults to coupler_ring)
straight – straight spec.
bend – bend spec.
cross_section_heater – for heater.
cross_section_waveguide_heater – for waveguide with heater.
cross_section – for regular waveguide.
via_stack – for heater to routing metal.
port_orientation – for electrical ports to promote from via_stack.
via_stack_offset – x,y offset for via_stack.
with_drop – adds drop ports.
o2──────▲─────────o3 │gap_top xx──────▼─────────xxx xxx xxx xxx xxx xx xxx x xxx xx xx▲ xx xx│length_y xx xx▼ xx xx xx length_x x xx ◄───────────────► x xx xxx xx xxx xxx──────▲─────────xxx │gap o1──────▼─────────o4
import ubcpdk
c = ubcpdk.components.ring_double_heater(gap=0.2, radius=10.0, length_x=1.0, length_y=0.2, cross_section_heater='xs_heater_metal', cross_section_waveguide_heater='xs_sc_heater_metal', via_stack_offset=(1, 0))
c.plot()
ring_single#
- ubcpdk.components.ring_single(gap: float = 0.2, radius: float = 10.0, length_x: float = 4.0, length_y: float = 0.6) Component [source]#
import ubcpdk
c = ubcpdk.components.ring_single(gap=0.2, radius=10.0, length_x=4.0, length_y=0.6, pass_cross_section_to_bend=False)
c.plot()
ring_single_heater#
- ubcpdk.components.ring_single_heater(gap: float = 0.2, radius: float = 10.0, length_x: float = 1.0, length_y: float = 0.01, *, coupler_ring: ComponentSpec = <function coupler_ring>, coupler_ring_top: ComponentSpec | None = None, straight: ComponentSpec = <function straight>, bend: ComponentSpec = 'bend_euler', cross_section_heater: CrossSectionSpec = 'heater_metal', cross_section_waveguide_heater: CrossSectionSpec = 'strip_heater_metal', cross_section: CrossSectionSpec = 'strip', via_stack: ComponentSpec = 'via_stack_heater_mtop', port_orientation: float | None = None, via_stack_offset: Float2 = (1, 0), with_drop: bool = False) Component #
Returns a double bus ring with heater on top.
two couplers (ct: top, cb: bottom) connected with two vertical straights (sl: left, sr: right)
- Parameters:
gap – gap between for coupler.
radius – for the bend and coupler.
length_x – ring coupler length.
length_y – vertical straight length.
coupler_ring – ring coupler spec.
coupler_ring_top – ring coupler spec for coupler away from vias (defaults to coupler_ring)
straight – straight spec.
bend – bend spec.
cross_section_heater – for heater.
cross_section_waveguide_heater – for waveguide with heater.
cross_section – for regular waveguide.
via_stack – for heater to routing metal.
port_orientation – for electrical ports to promote from via_stack.
via_stack_offset – x,y offset for via_stack.
with_drop – adds drop ports.
o2──────▲─────────o3 │gap_top xx──────▼─────────xxx xxx xxx xxx xxx xx xxx x xxx xx xx▲ xx xx│length_y xx xx▼ xx xx xx length_x x xx ◄───────────────► x xx xxx xx xxx xxx──────▲─────────xxx │gap o1──────▼─────────o4
import ubcpdk
c = ubcpdk.components.ring_single_heater(gap=0.2, radius=10.0, length_x=4.0, length_y=0.6, cross_section_waveguide_heater='xs_sc_heater_metal', via_stack_offset=(0, 0))
c.plot()
ring_with_crossing#
- ubcpdk.components.ring_with_crossing(*, component: ComponentSpec = <function ebeam_crossing4_2ports>, gap: float = 0.2, length_x: float = 4, length_y: float = 0, radius: float = 5.0, coupler: ComponentSpec = <function coupler_ring>, bend: ComponentSpec = <function bend_euler>, with_component: bool = True, port_name: str = 'o4', **kwargs) Component #
Single bus ring made of two couplers (ct: top, cb: bottom) connected.
with two vertical straights (wyl: left, wyr: right) (Component Under Test) in the middle to extract loss from quality factor.
- Parameters:
component – device under test.
gap – in um.
length_x – in um.
length_y – in um.
radius – in um.
coupler – coupler function.
bend – bend function.
with_component – True adds component. False adds waveguide.
port_name – for component input.
kwargs – cross_section settings.
with_component – if False changes component for just a straight.
bl-wt-br | | length_y wl component | | --==cb==-- gap length_x
import ubcpdk
c = ubcpdk.components.ring_with_crossing(gap=0.2, length_x=4, length_y=0, radius=5.0, with_component=True, port_name='o4')
c.plot()
(Source code
, png
, hires.png
, pdf
)
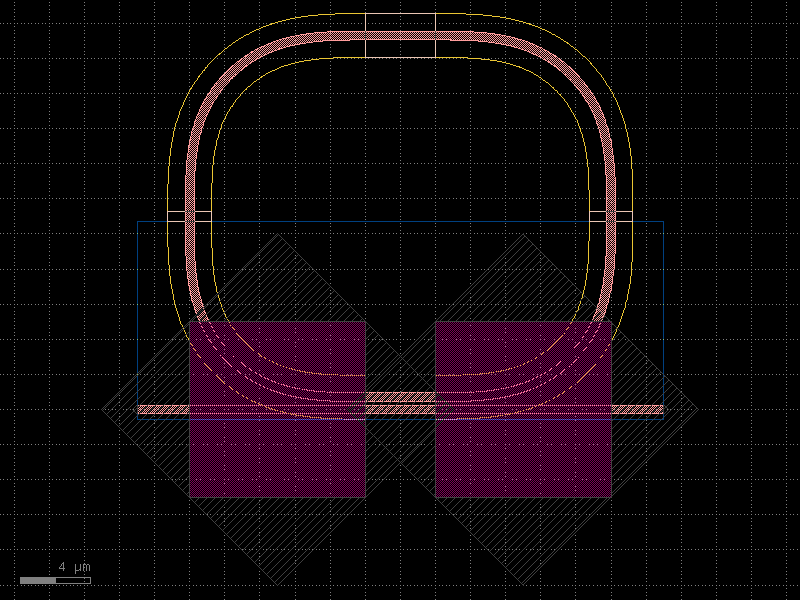
spiral#
- ubcpdk.components.spiral(length: float = 100, spacing: float = 3.0, n_loops: int = 6) Component [source]#
Returns spiral component.
- Parameters:
length – length.
spacing – spacing.
n_loops – number of loops.
import ubcpdk
c = ubcpdk.components.spiral(N=6, x_inner_length_cutback=300.0, x_inner_offset=0.0, y_straight_inner_top=0.0, xspacing=3.0, yspacing=3.0, cross_section='xs_sc', with_inner_ports=False, y_straight_outer_offset=0.0, inner_loop_spacing_offset=0.0, mirror_straight=False)
c.plot()
straight#
- ubcpdk.components.straight(length: float = 10.0, npoints: int = 2, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'strip', **kwargs) Component [source]#
Returns a Straight waveguide.
- Parameters:
length – straight length (um).
npoints – number of points.
cross_section – specification (CrossSection, string or dict).
kwargs – additional cross_section arguments.
o1 -------------- o2 length
import ubcpdk
c = ubcpdk.components.straight(length=10.0, npoints=2, cross_section='xs_sc', post_process=(functools.partial(<function add_padding at 0x7f87372dd440>, layers=((68, 0),), default=0, top=0.5, bottom=0.5),))
c.plot()
straight_heater_metal#
- ubcpdk.components.straight_heater_metal(length: float = 320.0, cross_section='strip') Component [source]#
import ubcpdk
c = ubcpdk.components.straight_heater_metal(length=320.0, length_undercut_spacing=6.0, length_undercut=30.0, length_straight=0.1, length_straight_input=15.0, cross_section='xs_sc', cross_section_heater='xs_heater_metal', cross_section_waveguide_heater='xs_sc_heater_metal', cross_section_heater_undercut='xs_sc_heater_metal_undercut', with_undercut=False, via_stack='via_stack_heater_mtop', heater_taper_length=5.0)
c.plot()
straight_one_pin#
- ubcpdk.components.straight_one_pin(length=1, cross_section=functools.partial(<function cross_section>, radius_min=5, bbox_layers=('DEVREC', ), bbox_offsets=(0.5, ))) Component [source]#
import ubcpdk
c = ubcpdk.components.straight_one_pin(length=1)
c.plot()
(Source code
, png
, hires.png
, pdf
)
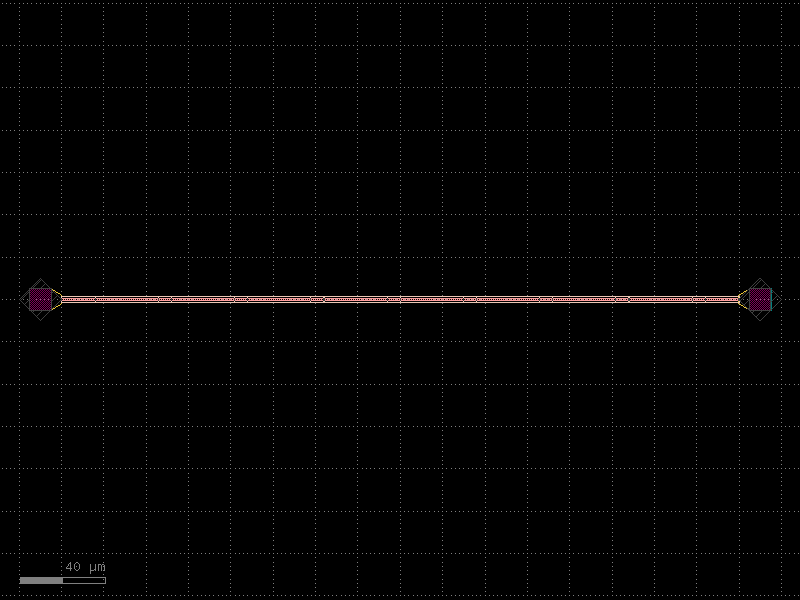
taper#
- ubcpdk.components.taper(length: float = 10.0, width1: float = 0.5, width2: float | None = None, port: Port | None = None, with_two_ports: bool = True, cross_section: CrossSectionSpec = 'strip', port_names: tuple[str, str] = ('o1', 'o2'), port_types: tuple[str, str] = ('optical', 'optical'), with_bbox: bool = True, **kwargs) Component #
Linear taper, which tapers only the main cross section section.
- Parameters:
length – taper length.
width1 – width of the west/left port.
width2 – width of the east/right port. Defaults to width1.
port – can taper from a port instead of defining width1.
with_two_ports – includes a second port. False for terminator and edge coupler fiber interface.
cross_section – specification (CrossSection, string, CrossSectionFactory dict).
port_names – input and output port names. Second name only used if with_two_ports.
port_types – input and output port types. Second type only used if with_two_ports.
with_bbox – box in bbox_layers and bbox_offsets to avoid DRC sharp edges.
kwargs – cross_section settings.
import ubcpdk
c = ubcpdk.components.taper(length=10.0, width1=0.5, with_two_ports=True, cross_section='xs_sc', port_order_name=('o1', 'o2'), port_order_types=('optical', 'optical'))
c.plot()
terminator_short#
import ubcpdk
c = ubcpdk.components.terminator_short()
c.plot()
(Source code
, png
, hires.png
, pdf
)
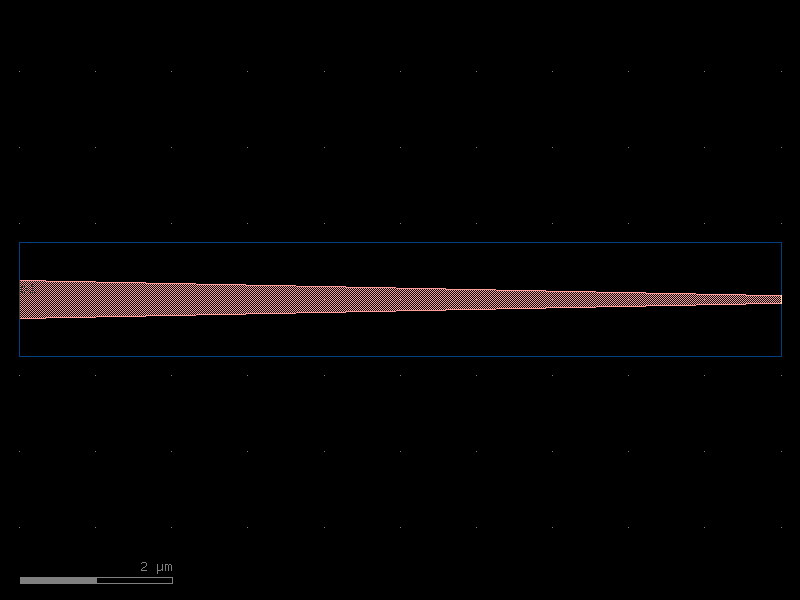
thermal_phase_shifter0#
- ubcpdk.components.thermal_phase_shifter0() Component [source]#
Return thermal_phase_shifters fixed cell.
import ubcpdk
c = ubcpdk.components.thermal_phase_shifter0()
c.plot()
(Source code
, png
, hires.png
, pdf
)
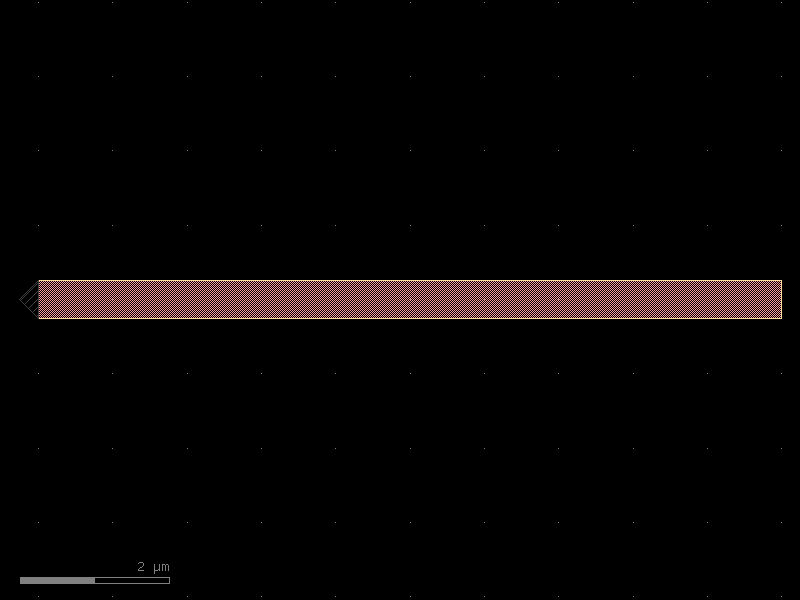
thermal_phase_shifter1#
- ubcpdk.components.thermal_phase_shifter1() Component [source]#
Return thermal_phase_shifters fixed cell.
import ubcpdk
c = ubcpdk.components.thermal_phase_shifter1()
c.plot()
(Source code
, png
, hires.png
, pdf
)
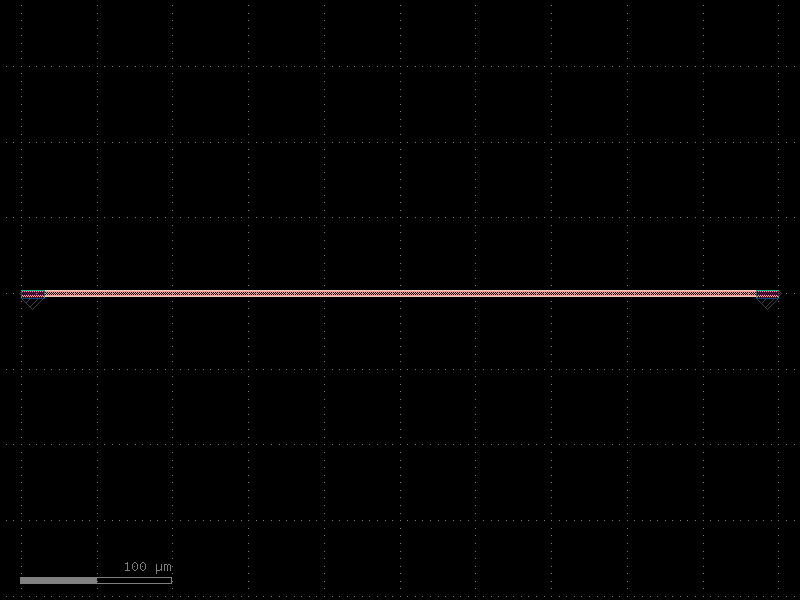
thermal_phase_shifter2#
- ubcpdk.components.thermal_phase_shifter2() Component [source]#
Return thermal_phase_shifters fixed cell.
import ubcpdk
c = ubcpdk.components.thermal_phase_shifter2()
c.plot()
(Source code
, png
, hires.png
, pdf
)
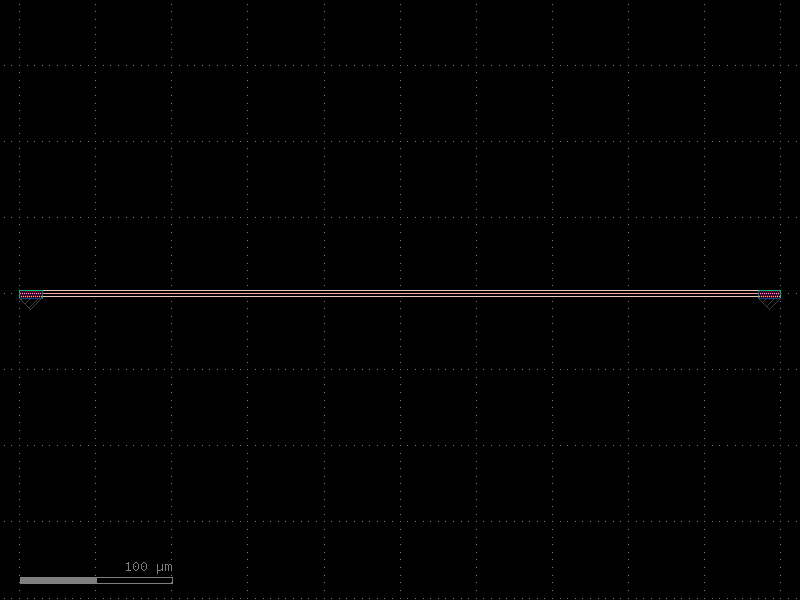
thermal_phase_shifter3#
- ubcpdk.components.thermal_phase_shifter3() Component [source]#
Return thermal_phase_shifters fixed cell.
import ubcpdk
c = ubcpdk.components.thermal_phase_shifter3()
c.plot()
(Source code
, png
, hires.png
, pdf
)
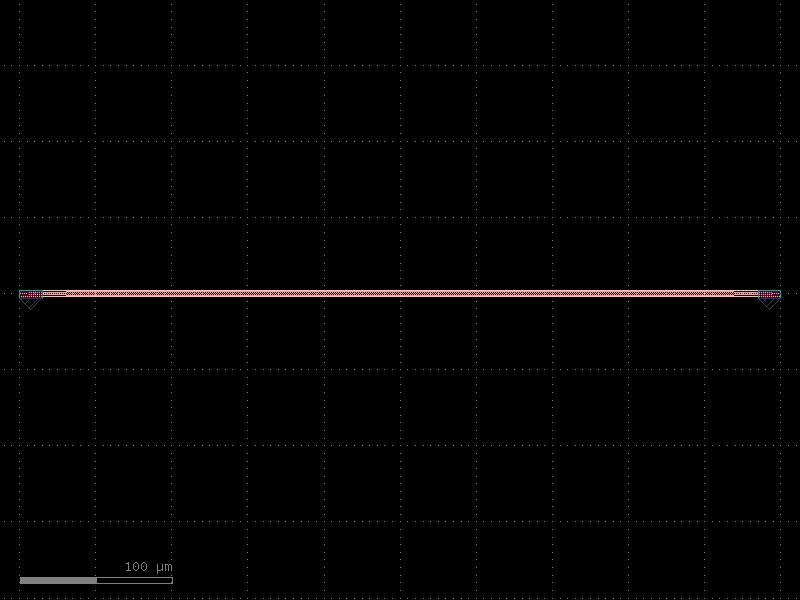
via_stack_heater_mtop#
import ubcpdk
c = ubcpdk.components.via_stack_heater_mtop(size=(10, 10), layers=((11, 0), (12, 0)), vias=(None, None), correct_size=True, slot_horizontal=False, slot_vertical=False)
c.plot()