Layout#
All UBC ubcpdk.components cells are conveniently combined into the ubcpdk.components module.
import gdsfactory as gf
import ubcpdk
import ubcpdk.components as uc
Fixed Component cells#
Most ubcpdk
components are imported from GDS files as fixed cells.
c = uc.ebeam_crossing4()
c.plot()
2024-04-12 23:30:18.982 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_crossing4.lyp'.
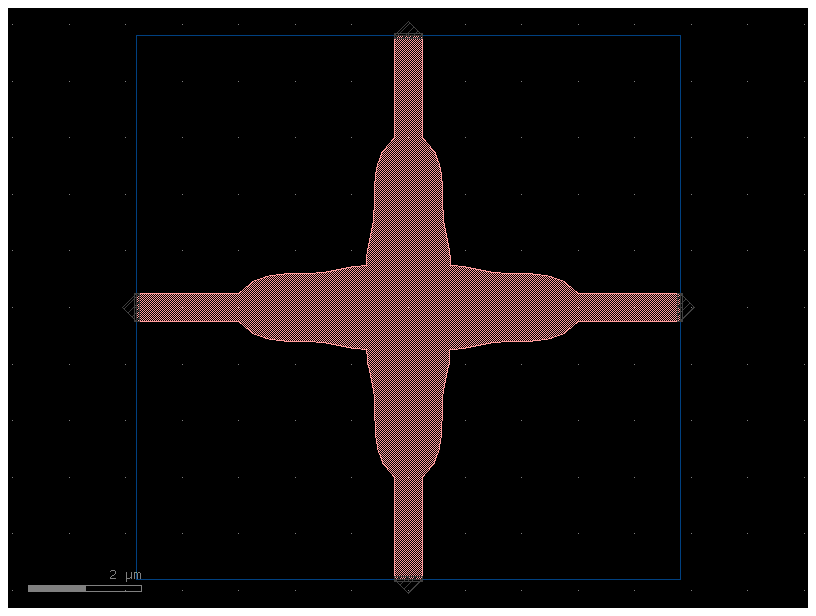
c = uc.ebeam_swg_edgecoupler()
c.plot()
2024-04-12 23:30:19.141 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_swg_edgecoupler.lyp'.
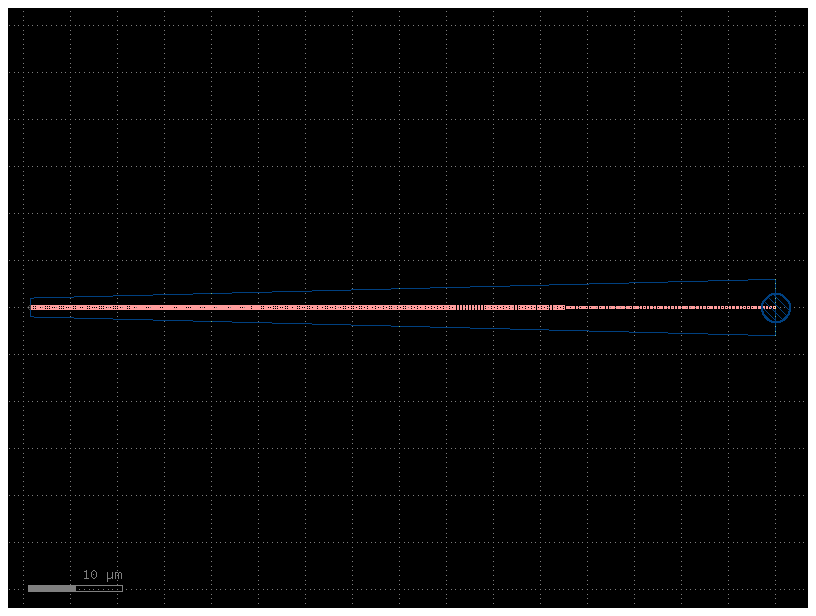
c = uc.ebeam_bdc_te1550()
c.plot()
2024-04-12 23:30:19.327 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_bdc_te1550.lyp'.
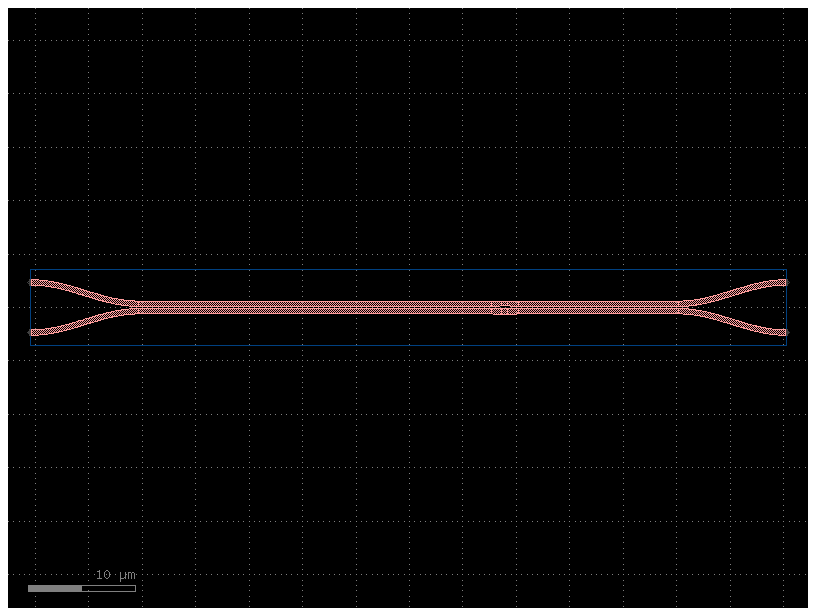
c = uc.ebeam_adiabatic_te1550()
c.plot()
2024-04-12 23:30:19.508 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_adiabatic_te1550.lyp'.
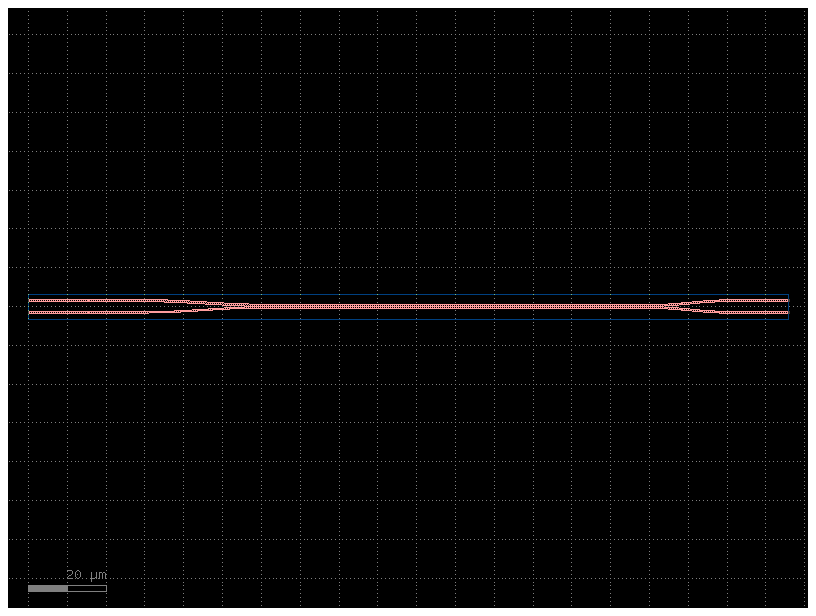
c = uc.ebeam_y_adiabatic()
c.plot()
2024-04-12 23:30:19.686 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_y_adiabatic.lyp'.
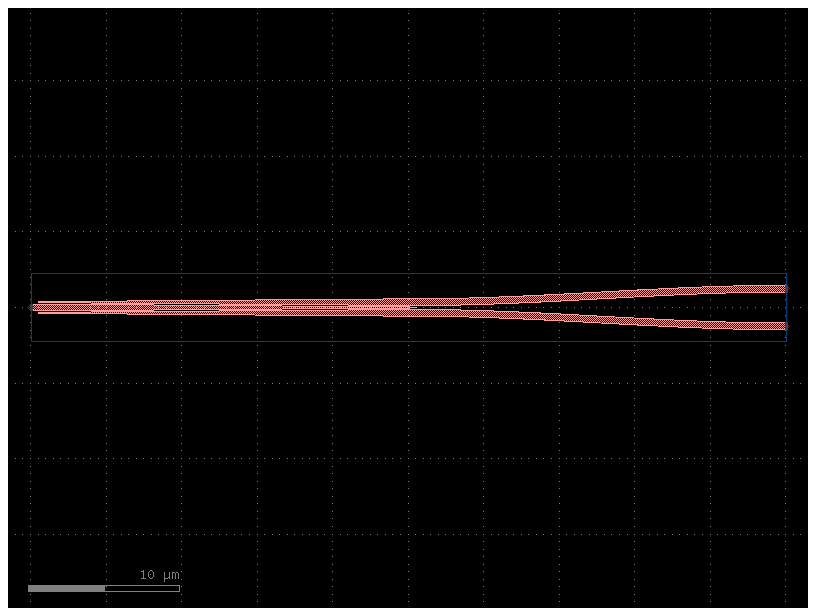
c = uc.ebeam_y_1550()
c.plot()
2024-04-12 23:30:19.860 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ebeam_y_1550.lyp'.
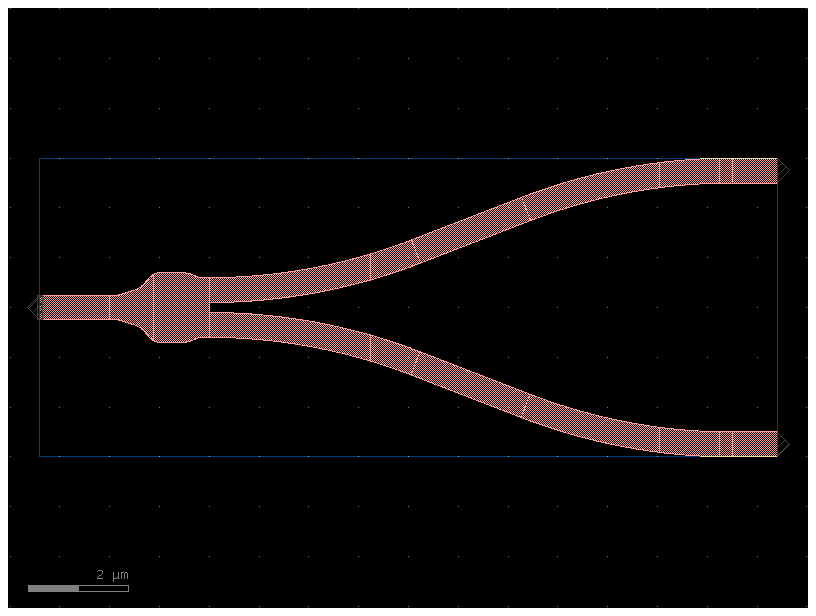
Parametric Component PCells#
You can also define cells adapted from gdsfactory generic pdk.
c = uc.straight(length=2)
c.plot()
2024-04-12 23:30:20.037 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/straight_length2_ubcpdkpcomponents.lyp'.
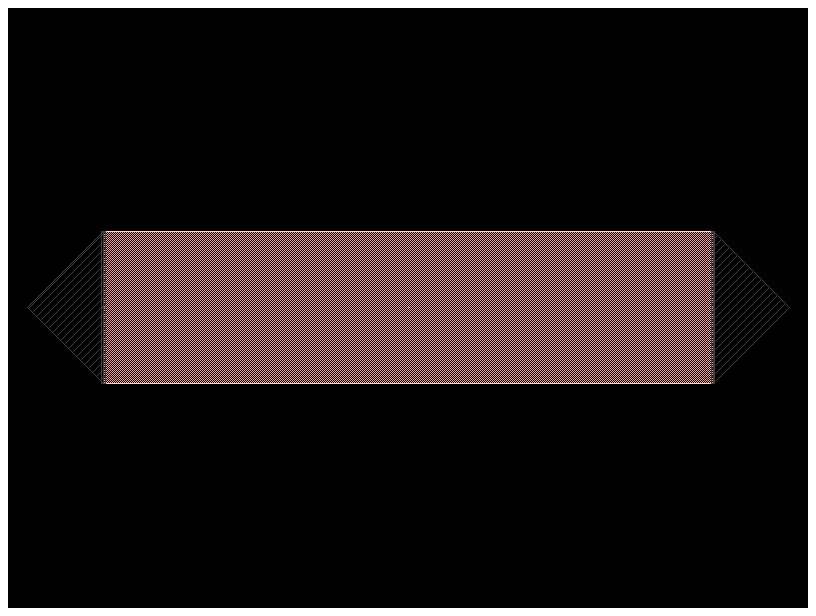
c = uc.bend(radius=5)
c.plot()
2024-04-12 23:30:20.210 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/bend_euler_sc_radius5.lyp'.
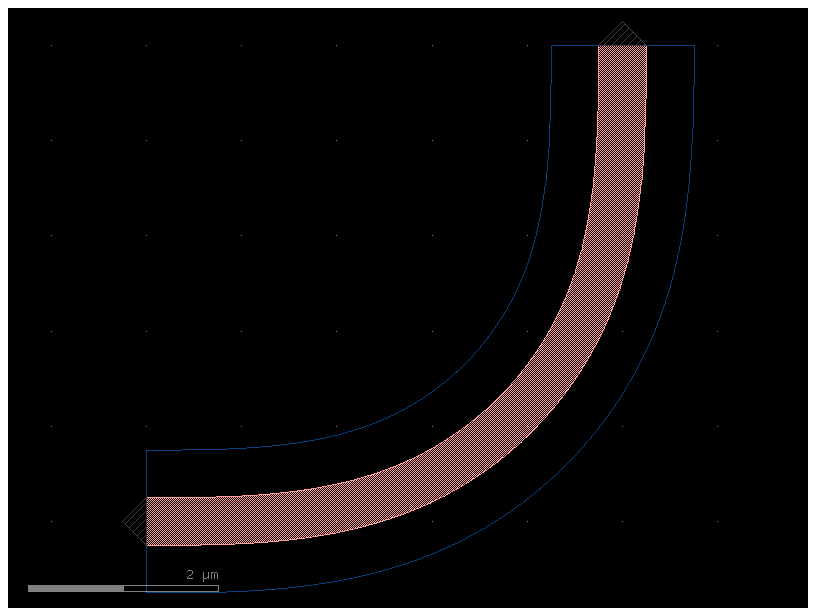
c = uc.ring_with_crossing()
c.plot()
2024-04-12 23:30:20.392 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ring_single_dut_26538df6.lyp'.
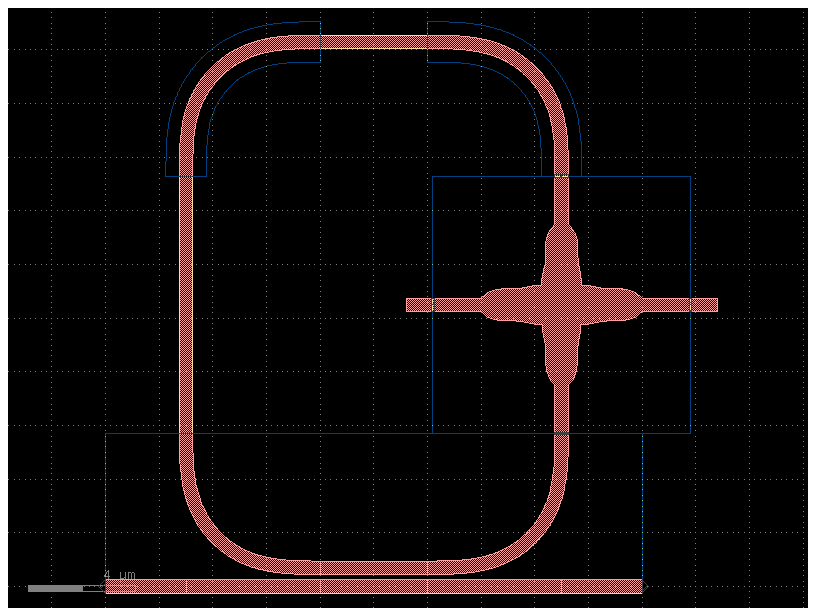
c = uc.dbr()
c.plot()
2024-04-12 23:30:20.534 | WARNING | gdsfactory.component:_write_library:1934 - UserWarning: Component dbr has invalid transformations. Try component.flatten_offgrid_references() first.
2024-04-12 23:30:20.547 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/dbr.lyp'.

c = uc.spiral()
c.plot()
2024-04-12 23:30:20.821 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/spiral_external_io.lyp'.
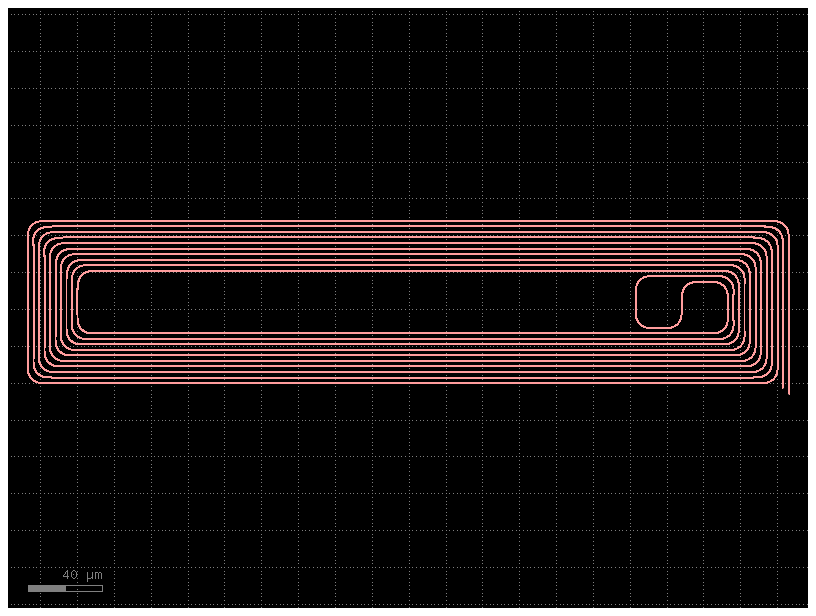
c = uc.mzi_heater()
c.plot()
2024-04-12 23:30:21.048 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/mzi_a1fe9d55.lyp'.

c = uc.ring_single_heater()
c.plot()
2024-04-12 23:30:21.253 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/ring_single_heater_cc91df46.lyp'.
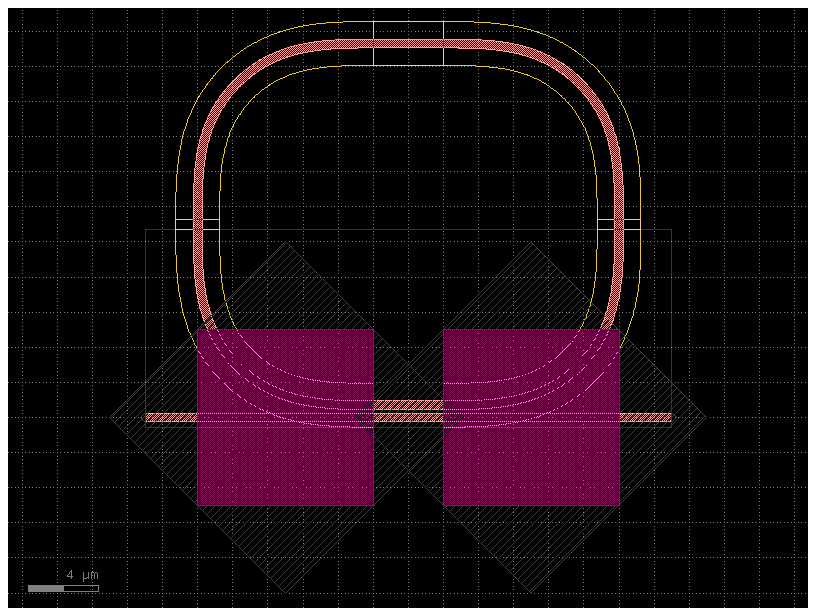
Components with grating couplers#
To test your devices you can add grating couplers. Both for single fibers and for fiber arrays.
splitter = uc.ebeam_y_1550()
mzi = gf.components.mzi(splitter=splitter)
mzi.plot()
2024-04-12 23:30:21.453 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/mzi_splitterebeam_y_1550.lyp'.
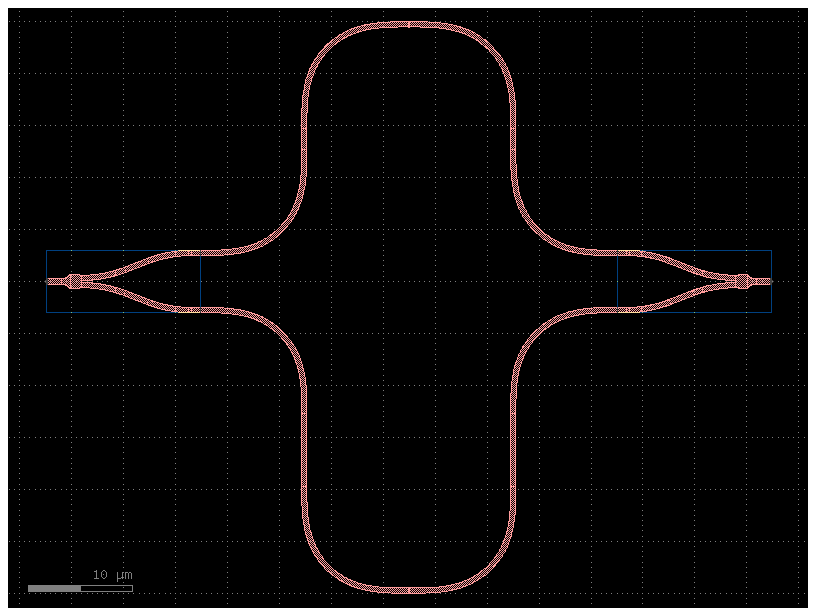
component_fiber_array = uc.add_fiber_array(component=mzi)
component_fiber_array.plot()
2024-04-12 23:30:21.653 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/add_fiber_array_add_fiber_array_3de02987.lyp'.

c = uc.ring_single_heater()
c = uc.add_fiber_array_pads_rf(c)
c.plot()
2024-04-12 23:30:21.864 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/add_fiber_array_add_fiber_array_35c727a6.lyp'.

c = uc.mzi_heater()
c = uc.add_fiber_array_pads_rf(c, optical_routing_type=2)
c.plot()
2024-04-12 23:30:22.086 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/add_fiber_array_add_fiber_array_3c63ffb9.lyp'.

3D rendering#
scene = c.to_3d()
scene.show()
Die assembly#
from functools import partial
from pathlib import Path
import gdsfactory as gf
import ubcpdk
import ubcpdk.components as uc
from ubcpdk import tech
from ubcpdk.tech import LAYER
size = (440, 470)
add_gc = uc.add_fiber_array
@gf.cell
def EBeam_JoaquinMatres_1() -> gf.Component:
"""Add DBR cavities."""
e = [add_gc(uc.straight())]
e += [add_gc(uc.mzi(delta_length=dl)) for dl in [9.32, 93.19]]
e += [
add_gc(uc.ring_single(radius=12, gap=gap, length_x=coupling_length))
for gap in [0.2]
for coupling_length in [2.5, 4.5, 6.5]
]
e += [
uc.dbr_cavity_te(w0=w0, dw=dw)
for w0 in [0.5]
for dw in [50e-3, 100e-3, 150e-3, 200e-3]
]
e += [add_gc(uc.ring_with_crossing())]
e += [add_gc(uc.ring_with_crossing(port_name="o2", with_component=False))]
c = gf.Component()
_ = c << gf.pack(e, max_size=size, spacing=2)[0]
_ = c << gf.components.rectangle(size=size, layer=LAYER.FLOORPLAN)
return c
gf.clear_cache()
c = EBeam_JoaquinMatres_1()
c.show() # show in klayout
c.plot() # plot in notebook
2024-04-12 23:30:23.156 | WARNING | gdsfactory.klive:show:49 - UserWarning: Could not connect to klive server. Is klayout open and klive plugin installed?
2024-04-12 23:30:23.165 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/EBeam_JoaquinMatres_1.lyp'.

@gf.cell
def EBeam_JoaquinMatres_2() -> gf.Component:
"""spirals for extracting straight waveguide loss"""
N = 12
radius = 10
e = [
uc.add_fiber_array(
component=uc.spiral(
N=N,
radius=radius,
y_straight_inner_top=0,
x_inner_length_cutback=0,
)
)
]
e.append(
uc.add_fiber_array(
component=uc.spiral(
N=N,
radius=radius,
y_straight_inner_top=0,
x_inner_length_cutback=185,
)
)
)
c = gf.Component()
_ = c << gf.pack(e, max_size=size, spacing=2)[0]
_ = c << gf.components.rectangle(size=size, layer=LAYER.FLOORPLAN)
return c
c = EBeam_JoaquinMatres_2()
c.show() # show in klayout
c.plot() # plot in notebook
2024-04-12 23:30:23.646 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/EBeam_JoaquinMatres_2.lyp'.
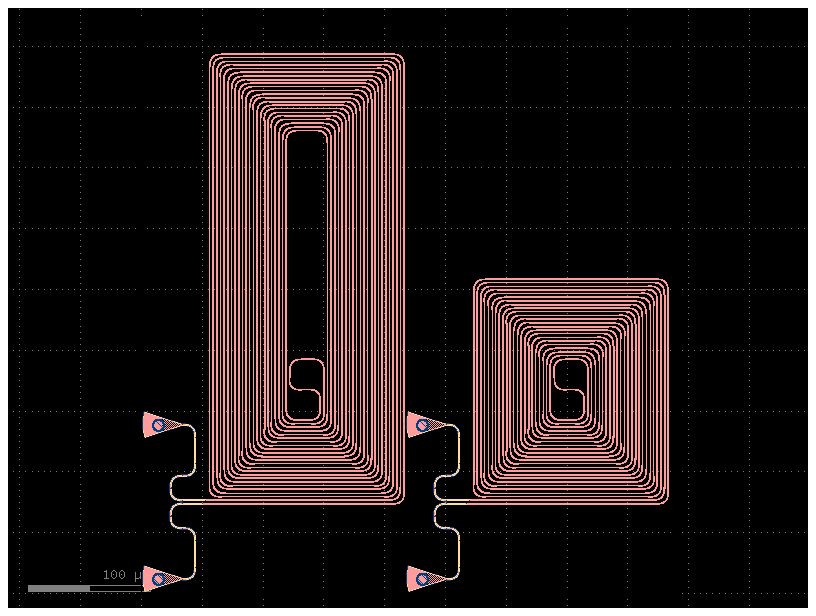
@gf.cell
def EBeam_JoaquinMatres_3() -> gf.Component:
"""contains mirror cavities and structures inside a resonator"""
e = []
e += [add_gc(uc.ebeam_crossing4())]
e += [add_gc(uc.ebeam_adiabatic_te1550(), optical_routing_type=1)]
e += [add_gc(uc.ebeam_bdc_te1550())]
e += [add_gc(uc.ebeam_y_1550(), optical_routing_type=1)]
e += [add_gc(uc.ebeam_y_adiabatic_tapers(), optical_routing_type=1)]
e += [add_gc(uc.straight(), component_name=f"straight_{i}") for i in range(2)]
c = gf.Component()
_ = c << gf.pack(e, max_size=size, spacing=2)[0]
_ = c << gf.components.rectangle(size=size, layer=LAYER.FLOORPLAN)
return c
gf.clear_cache()
c = EBeam_JoaquinMatres_3()
c.show() # show in klayout
c.plot() # plot in notebook
2024-04-12 23:30:24.041 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/EBeam_JoaquinMatres_3.lyp'.
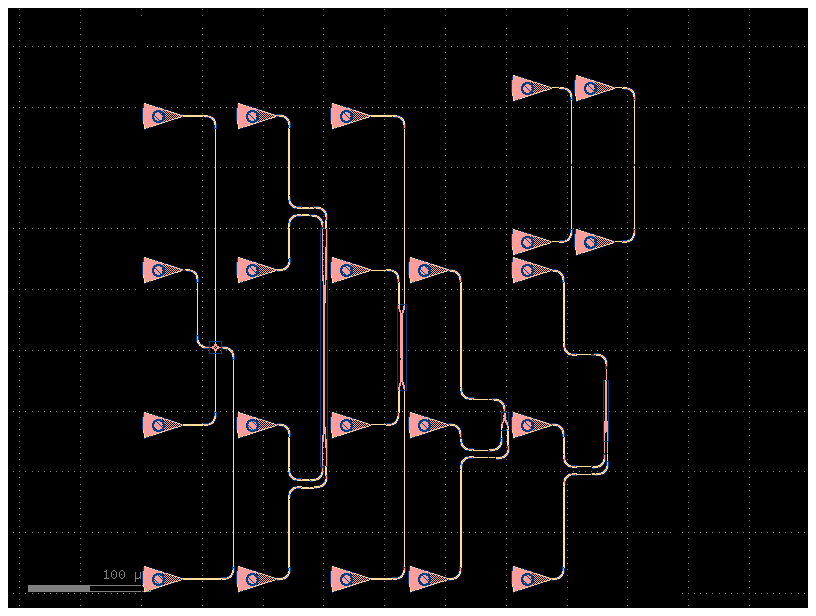
@gf.cell
def EBeam_JoaquinMatres_4() -> gf.Component:
"""MZI interferometers."""
mzi = partial(gf.components.mzi, splitter=uc.ebeam_y_1550)
mzis = [mzi(delta_length=delta_length) for delta_length in [10, 40, 100]]
mzis_gc = [uc.add_fiber_array(mzi) for mzi in mzis]
mzis = [uc.mzi_heater(delta_length=delta_length) for delta_length in [40]]
mzis_heater_gc = [
uc.add_fiber_array_pads_rf(mzi, orientation=90, optical_routing_type=2)
for mzi in mzis
]
e = mzis_gc + mzis_heater_gc
c = gf.Component()
_ = c << gf.pack(e, max_size=size, spacing=2)[0]
_ = c << gf.components.rectangle(size=size, layer=LAYER.FLOORPLAN)
return c
gf.clear_cache()
c = EBeam_JoaquinMatres_4()
c.show() # show in klayout
c.plot() # plot in notebook
2024-04-12 23:30:24.375 | WARNING | gdsfactory.pack:pack:249 - UserWarning: unable to pack in one component, creating 2 components
2024-04-12 23:30:24.387 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/EBeam_JoaquinMatres_4.lyp'.

@gf.cell
def EBeam_JoaquinMatres_5() -> gf.Component:
"""Ring resonators."""
rings = [uc.ring_single_heater(length_x=length_x) for length_x in [4, 6]]
rings = [gf.functions.rotate180(ring) for ring in rings]
rings_gc = [uc.add_fiber_array_pads_rf(ring) for ring in rings]
c = gf.Component()
_ = c << gf.pack(rings_gc, max_size=size, spacing=2)[0]
_ = c << gf.components.rectangle(size=size, layer=LAYER.FLOORPLAN)
return c
gf.clear_cache()
c = EBeam_JoaquinMatres_5()
c.show() # show in klayout
c.plot() # plot in notebook
2024-04-12 23:30:24.639 | INFO | gdsfactory.technology.layer_views:to_lyp:1018 - LayerViews written to '/tmp/gdsfactory/EBeam_JoaquinMatres_5.lyp'.
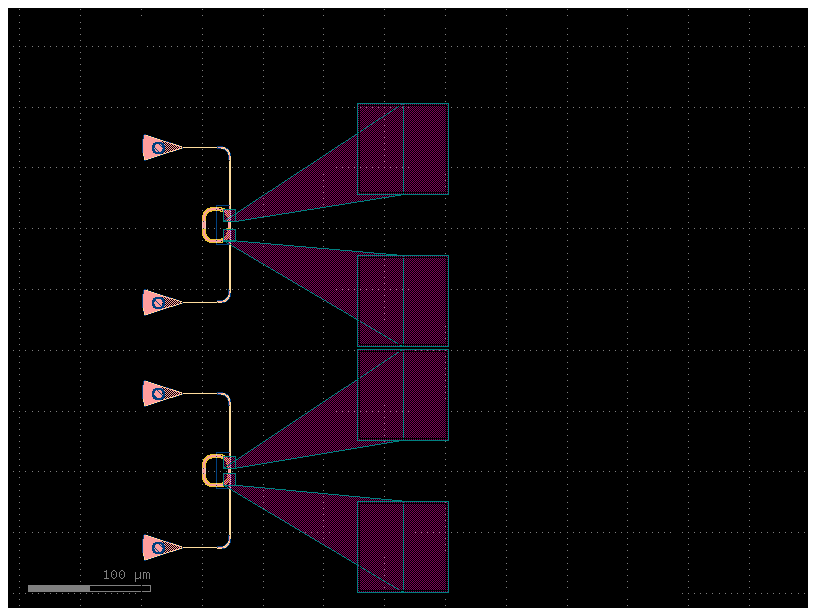