Netlist extractor SPICE#
This notebook demonstrates how to extract the SPICE netlist of a Component or a GDS file using gdsfactory. It uses the :func:~get_netlist
and :func:~get_l2n
functions from the gplugins.klayout
module to extract the netlist and connectivity mapping, respectively. It also uses the plot_nets
function to visualize the connectivity.
import pathlib
from gdsfactory.samples.demo.lvs import pads_correct, pads_shorted
from gplugins.klayout.get_netlist import get_l2n, get_netlist
from gplugins.klayout.netlist_spice_reader import (
GdsfactorySpiceReader,
)
from gplugins.klayout.plot_nets import plot_nets
Sample layouts#
We generate a sample layout using pads_correct
and write a GDS file.
c = pads_correct()
gdspath = c.write_gds()
c.show()
c.plot()
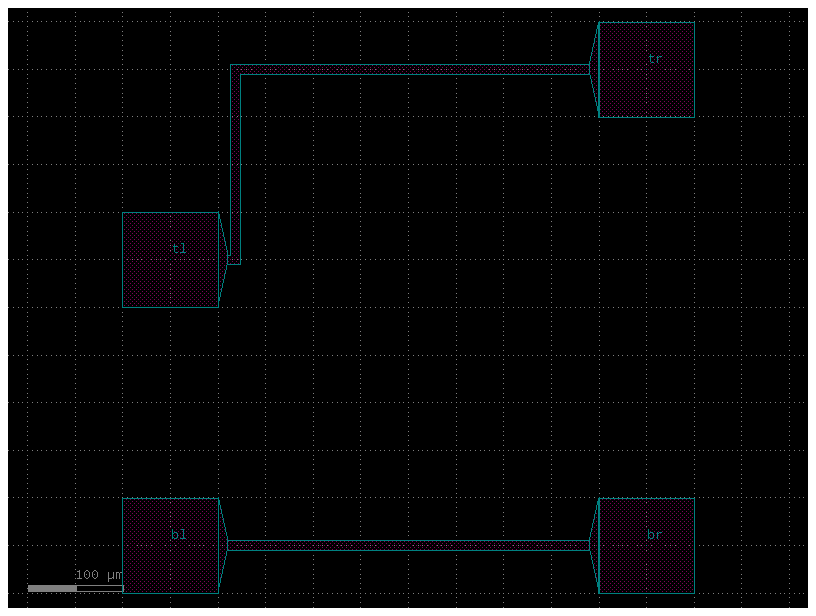
We obtain the netlist using KLayout by simply calling the :func:~get_netlist
function from the gplugins.klayout
module. The function takes the path to the GDS file as an argument and returns the netlist as a kdb.Netlist
object.
netlist = get_netlist(gdspath)
print(netlist)
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/layers.lyp'
/home/runner/work/gplugins/gplugins/gplugins/klayout/d25/generic.lyd25
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/tech.lyt'
circuit taper_L10_W100_W10_LNon_43bc52bd ($1=$1);
end;
circuit pad_S100_100_LMTOP_BLNo_163fd346 ($1=$1,$2=$2);
end;
circuit pads_correct_Ppad_CSmetal3 ();
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $1 ($1='tl,tr',$2=tl);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $2 ($1='tl,tr');
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $3 ($1='tl,tr',$2=tr);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $4 ($1='tl,tr');
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $5 ($1='bl,br',$2=br);
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $6 ($1='bl,br',$2=bl);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $7 ($1='bl,br');
subcircuit taper_L10_W100_W10_LNon_43bc52bd $8 ($1='bl,br');
end;
The connectivity between the components in the GDS file can be visualized using the :func:~plot_nets
function from the gplugins.klayout
module. The function takes the path to the GDS file as an argument and plots the connectivity between the components in the GDS file.
l2n = get_l2n(gdspath)
cwd = pathlib.Path.cwd()
filepath = cwd / f"{c.name}.txt"
l2n.write_l2n(str(filepath))
plt = plot_nets(
filepath, spice_reader=GdsfactorySpiceReader(components_as_subcircuits=[c.name])
)
plt.savefig(f"{c.name}.png")
plt.show()
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/layers.lyp'
/home/runner/work/gplugins/gplugins/gplugins/klayout/d25/generic.lyd25
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/tech.lyt'
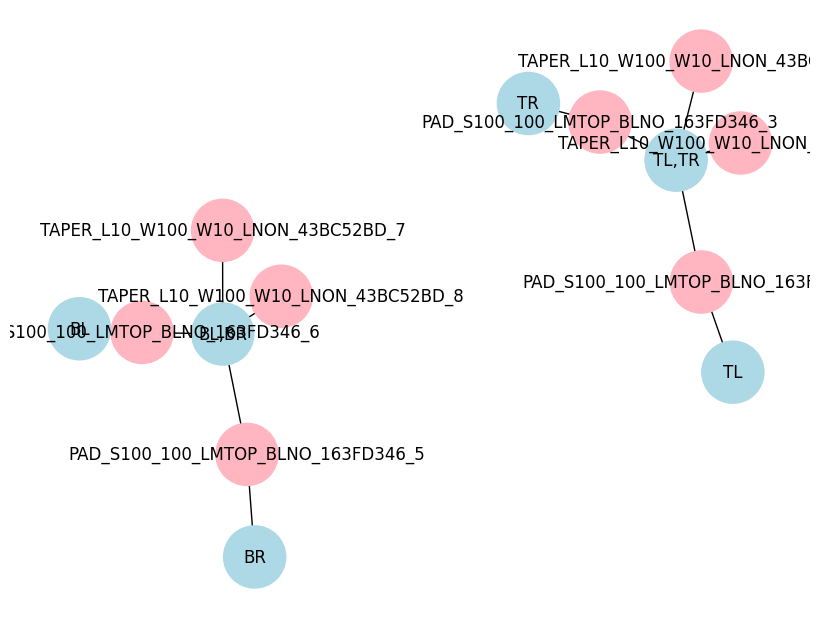
The same steps as above are done for a shorted case.
c = pads_shorted()
gdspath = c.write_gds()
c.plot()
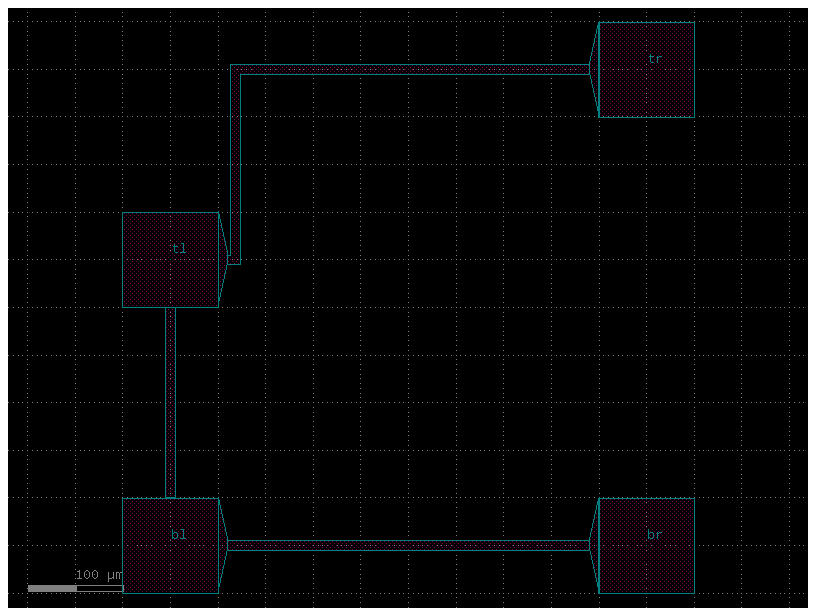
netlist = get_netlist(gdspath)
print(netlist)
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/layers.lyp'
/home/runner/work/gplugins/gplugins/gplugins/klayout/d25/generic.lyd25
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/tech.lyt'
circuit taper_L10_W100_W10_LNon_43bc52bd ($1=$1);
end;
circuit pad_S100_100_LMTOP_BLNo_163fd346 ($1=$1,$2=$2);
end;
circuit pads_shorted_Ppad_CSmetal3 ();
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $1 ($1='bl,br,tl,tr',$2=tl);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $2 ($1='bl,br,tl,tr');
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $3 ($1='bl,br,tl,tr',$2=br);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $4 ($1='bl,br,tl,tr');
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $5 ($1='bl,br,tl,tr',$2=tr);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $6 ($1='bl,br,tl,tr');
subcircuit pad_S100_100_LMTOP_BLNo_163fd346 $7 ($1='bl,br,tl,tr',$2=bl);
subcircuit taper_L10_W100_W10_LNon_43bc52bd $8 ($1='bl,br,tl,tr');
end;
l2n = get_l2n(gdspath)
filepath = cwd / f"{c.name}.txt"
l2n.write_l2n(str(filepath))
plt = plot_nets(
filepath, spice_reader=GdsfactorySpiceReader(components_as_subcircuits=[c.name])
)
plt.savefig(f"{c.name}.png")
plt.show()
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/layers.lyp'
/home/runner/work/gplugins/gplugins/gplugins/klayout/d25/generic.lyd25
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/tech.lyt'
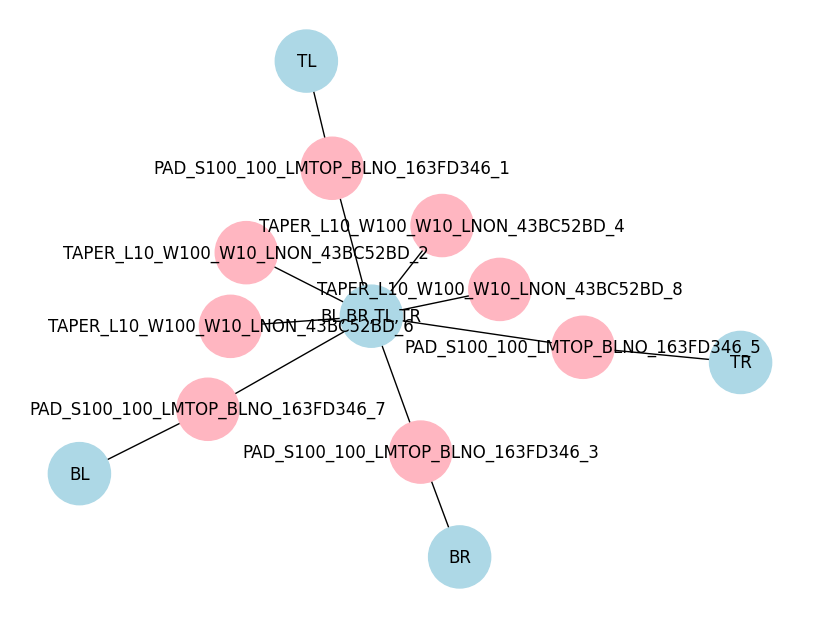