2D meshing: uz cross-section#
You can mesh an out of plane Component
cross-section by supplying the argument type="uz"
and a xsection_bounds
under the form [[x1,y1], [x2,y2]]
, which parametrizes a line in u
-coordinates
import gdsfactory as gf
import meshio
from gdsfactory.generic_tech import get_generic_pdk
from gdsfactory.pdk import get_layer_stack
from gdsfactory.technology import LayerStack
from skfem.io import from_meshio
from gplugins.gmsh.get_mesh import create_physical_mesh, get_mesh
gf.config.rich_output()
PDK = get_generic_pdk()
PDK.activate()
waveguide = gf.components.straight_pin(length=10, taper=None).dup()
waveguide.trim(left=3, right=5, top=+4, bottom=-4)
waveguide.plot()
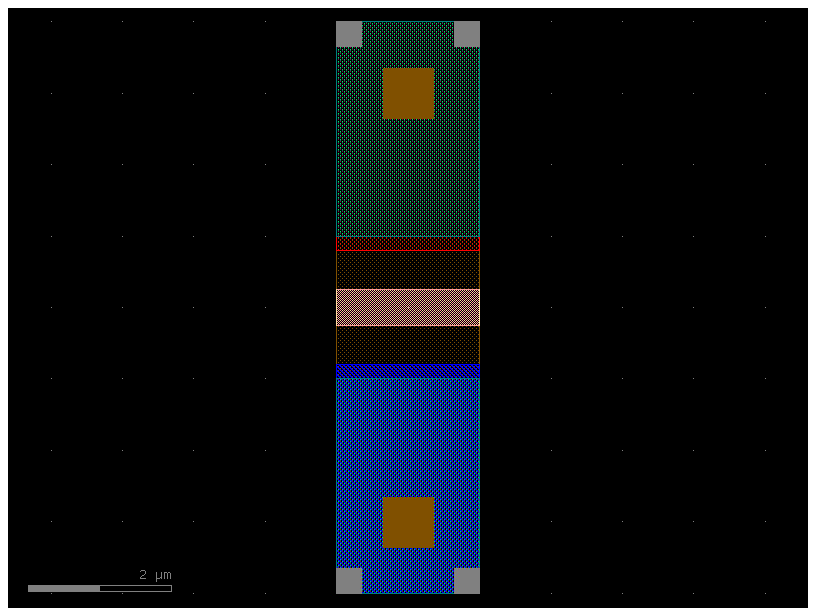
filtered_layer_stack = LayerStack(
layers={
k: get_layer_stack().layers[k]
for k in (
"slab90",
"core",
"via_contact",
)
}
)
filename = "mesh"
def mesh_with_physicals(mesh, filename):
mesh_from_file = meshio.read(f"{filename}.msh")
return create_physical_mesh(mesh_from_file, "triangle")
Choosing the line going from y=-4
to y=4
at x=4
, which crosses slab, via, and core:
mesh = get_mesh(
component=waveguide,
type="uz",
xsection_bounds=[(4, -4), (4, 4)],
layer_stack=filtered_layer_stack,
filename=f"{filename}.msh",
)
mesh = mesh_with_physicals(mesh, filename)
mesh = from_meshio(mesh)
mesh.draw().plot()
Info : [ 0%] Union
Info : [ 10%] Union - Performing intersection of shapes
Info : [ 80%] Union - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference - Performing intersection of shapes
Info : [ 80%] Difference - Building splits of containers
Info : [ 0%] Fragments
Info : [ 10%] Fragments - Performing intersection of shapes
Info : [ 80%] Fragments - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference
Info : [ 20%] Difference
Info : [ 30%] Difference
Info : [ 40%] Difference
Info : [ 50%] Difference
Info : [ 60%] Difference
Info : [ 70%] Difference - Filling splits of edges
Info : [ 80%] Difference - Making faces
Info : [ 90%] Difference
Info : [ 0%] Fragments
Info : [ 10%] Fragments
Info : [ 20%] Fragments
Info : [ 30%] Fragments
Info : [ 40%] Fragments
Info : [ 50%] Fragments
Info : [ 60%] Fragments
Info : [ 70%] Fragments - Filling splits of edges
Info : [ 80%] Fragments - Splitting faces
Info : Meshing 1D...
Info : [ 0%] Meshing curve 4 (Line)
Info : [ 0%] Meshing curve 3 (Line)
Info : [ 0%] Meshing curve 2 (Line)
Info : [ 10%] Meshing curve 5 (Line)
Info : [ 10%] Meshing curve 6 (Line)
Info : [ 10%] Meshing curve 7 (Line)
Info : [ 10%] Meshing curve 8 (Line)
Info : [ 30%] Meshing curve 15 (Line)
Info : [ 30%] Meshing curve 16 (Line)
Info : [ 30%] Meshing curve 17 (Line)
Info : [ 40%] Meshing curve 18 (Line)
Info : [ 40%] Meshing curve 19 (Line)
Info : [ 40%] Meshing curve 20 (Line)
Info : [ 50%] Meshing curve 21 (Line)
Info : [ 50%] Meshing curve 22 (Line)
Info : [ 50%] Meshing curve 23 (Line)
Info : [ 60%] Meshing curve 24 (Line)
Info : [ 60%] Meshing curve 25 (Line)
Info : [ 60%] Meshing curve 26 (Line)
Info : [ 80%] Meshing curve 27 (Line)
Info : [ 80%] Meshing curve 28 (Line)
Info : [ 80%] Meshing curve 29 (Line)
Info : [ 90%] Meshing curve 30 (Line)
Info : [ 0%] Meshing curve 1 (Line)
Info : Done meshing 1D (Wall 0.0030724s, CPU 0.008079s)
Info : Meshing 2D...
Info : [ 0%] Meshing surface 4 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 2 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 1 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 3 (Plane, Frontal-Delaunay)
Info : [ 30%] Meshing surface 5 (Plane, Frontal-Delaunay)
Info : Done meshing 2D (Wall 0.000706426s, CPU 0.002368s)
Info : 69 nodes 162 elements
Info : Writing 'mesh.msh'...
Info : Done writing 'mesh.msh'
Info : Writing '/tmp/tmpefqnlaze/mesh.msh'...
Info : Done writing '/tmp/tmpefqnlaze/mesh.msh'
[]

We can plot as usual (note that the u-z
coordinates we are working in still map to xy
for the plotter):
Mesh background#
You can add a convenience argument to add a background mesh around the geometry (instead of defining a dummy polygon and layer in the layer_stack with low mesh_order):
mesh = get_mesh(
component=waveguide,
type="uz",
xsection_bounds=[(4, -4), (4, 4)],
layer_stack=filtered_layer_stack,
filename=f"{filename}.msh",
background_tag="oxide",
background_padding=(2.0, 2.0, 2.0, 2.0),
)
mesh = mesh_with_physicals(mesh, filename)
mesh = from_meshio(mesh)
mesh.draw().plot()
Info : [ 0%] Union
Info : [ 10%] Union - Performing intersection of shapes
Info : [ 80%] Union - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference - Performing intersection of shapes
Info : [ 80%] Difference - Building splits of containers
Info : [ 0%] Fragments
Info : [ 10%] Fragments - Performing intersection of shapes
Info : [ 80%] Fragments - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference
Info : [ 20%] Difference
Info : [ 30%] Difference
Info : [ 40%] Difference
Info : [ 50%] Difference
Info : [ 60%] Difference
Info : [ 70%] Difference - Filling splits of edges
Info : [ 80%] Difference - Making faces
Info : [ 90%] Difference
Info : [ 0%] Fragments
Info : [ 10%] Fragments
Info : [ 20%] Fragments
Info : [ 30%] Fragments
Info : [ 40%] Fragments
Info : [ 50%] Fragments
Info : [ 60%] Fragments
Info : [ 70%] Fragments - Filling splits of edges
Info : [ 80%] Fragments - Splitting faces
Info : [ 0%] Difference
Info : [ 10%] Difference
Info : [ 20%] Difference
Info : [ 30%] Difference
Info : [ 40%] Difference
Info : [ 50%] Difference
Info : [ 60%] Difference
Info : [ 70%] Difference - Filling splits of edges
Info : [ 80%] Difference - Making faces
Info : [ 90%] Difference - Adding holes
Info : [ 0%] Fragments
Info : [ 10%] Fragments
Info : [ 20%] Fragments
Info : [ 30%] Fragments
Info : [ 40%] Fragments
Info : [ 50%] Fragments
Info : [ 60%] Fragments
Info : [ 70%] Fragments - Building splits of containers
Info : [ 80%] Fragments - Splitting faces
Info : Meshing 1D...
Info : [ 0%] Meshing curve 1 (Line)
Info : [ 0%] Meshing curve 2 (Line)
Info : [ 10%] Meshing curve 3 (Line)
Info : [ 10%] Meshing curve 4 (Line)
Info : [ 20%] Meshing curve 5 (Line)
Info : [ 20%] Meshing curve 6 (Line)
Info : [ 20%] Meshing curve 7 (Line)
Info : [ 20%] Meshing curve 8 (Line)
Info : [ 30%] Meshing curve 15 (Line)
Info : [ 30%] Meshing curve 16 (Line)
Info : [ 40%] Meshing curve 17 (Line)
Info : [ 40%] Meshing curve 18 (Line)
Info : [ 40%] Meshing curve 19 (Line)
Info : [ 40%] Meshing curve 20 (Line)
Info : [ 50%] Meshing curve 21 (Line)
Info : [ 50%] Meshing curve 22 (Line)
Info : [ 60%] Meshing curve 23 (Line)
Info : [ 60%] Meshing curve 24 (Line)
Info : [ 60%] Meshing curve 25 (Line)
Info : [ 70%] Meshing curve 27 (Line)
Info : [ 70%] Meshing curve 28 (Line)
Info : [ 70%] Meshing curve 29 (Line)
Info : [ 70%] Meshing curve 30 (Line)
Info : [ 70%] Meshing curve 31 (Line)
Info : [ 70%] Meshing curve 32 (Line)
Info : [ 70%] Meshing curve 33 (Line)
Info : [ 70%] Meshing curve 34 (Line)
Info : [ 70%] Meshing curve 26 (Line)
Info : Done meshing 1D (Wall 0.00210198s, CPU 0.004386s)
Info : Meshing 2D...
Info : [ 0%] Meshing surface 1 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 2 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 3 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 4 (Plane, Frontal-Delaunay)
Info : [ 20%] Meshing surface 5 (Plane, Frontal-Delaunay)
Info : [ 20%] Meshing surface 6 (Plane, Frontal-Delaunay)
Info : Done meshing 2D (Wall 0.0137733s, CPU 0.039273s)
Info : 504 nodes 1093 elements
Info : Writing 'mesh.msh'...
Info : Done writing 'mesh.msh'
Info : Writing '/tmp/tmprabjwt65/mesh.msh'...
Info : Done writing '/tmp/tmprabjwt65/mesh.msh'
[]
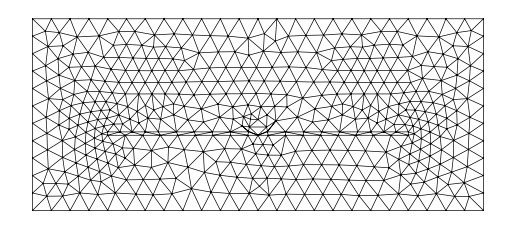