Meshing introduction#
gdsfactory has interfaces to external meshers (currently: gmsh).
Using a gdsfactory Component
and a Layerstack
reflecting the post-fabrication structure, you can automatically generate a 2D or 3D mesh suitable for physical simulation.
Within gdsfactory, this interface is currently used for:
finite-volume simulation through a DEVSIM plugin, for e.g. TCAD simulations
finite-element simulation through the femwell wrapper around scikit-fem, for e.g. mode solving and thermal simulations
Current features include:
GDS postprocessing – common interface for layout and simulation
A generic shapely <–> gmsh translator, which properly reuses gmsh objects, resulting in conformal handling of
lateral interfaces
vertical interfaces
polygon inclusions
polygon “holes”
2D meshing of in-plane cross-sections (e.g. x - y)
2D meshing of out-of-plane cross-sections (e.g. arbitrary xy line - z)
(In progress) 3D meshing
The mesh is returned tagged with LayerStack
label
for each GDS layer according to a specificmesh_order
All interfaces between layer entities are also tagged as
label1___label2
to e.g. implement interfacial boundary conditionsDummy layers can be easily introduced in a component to provide extra lines and polygons with custom labels to e.g. implement boundary conditions, sources, etc.
Coarse resolution setting per label, and around interfaces
Fine resolution setting with callable
[x,y,z,mesh_size]
functions (useful for simulation-driven refinement)
GMSH can be used one of two ways:
The traditional “bottom-up” way, where the user manually defines points, then line segments (from points), then lines (from segments), then closed curves (from lines), then surfaces (from curves), then closed shells (from surfaces), and finally volumes (from shells).
With CAD-type boolean operations (set operations on objects)
While the latter method is much simpler for complex geometries, as of 2022 it does not preserve physical and mesh information, requiring manual “retagging” of the entities after the boolean operations, and driving its complexity back to bottom-up construction (especially for arbitrary geometries).
As such, gdsfactory uses the first approach, where the mask layers and a layer_stack are used as a guide to define the various physical entities, which are returned as tagged objects to the user.
Installation#
You can install the meshing plugins with pip install gplugins[gmsh]
.
Because PyVista does not work properly on headless systems we use Meshio.
Usage#
First, you can start with a gdsfactory Component
import gdsfactory as gf
import meshio
from gdsfactory.generic_tech import LAYER_STACK, get_generic_pdk
from gdsfactory.technology import LayerStack
from skfem.io import from_meshio
from gplugins.gmsh.get_mesh import create_physical_mesh, get_mesh
gf.config.rich_output()
PDK = get_generic_pdk()
PDK.activate()
waveguide = gf.components.straight_pin(length=10, taper=None)
waveguide.plot()
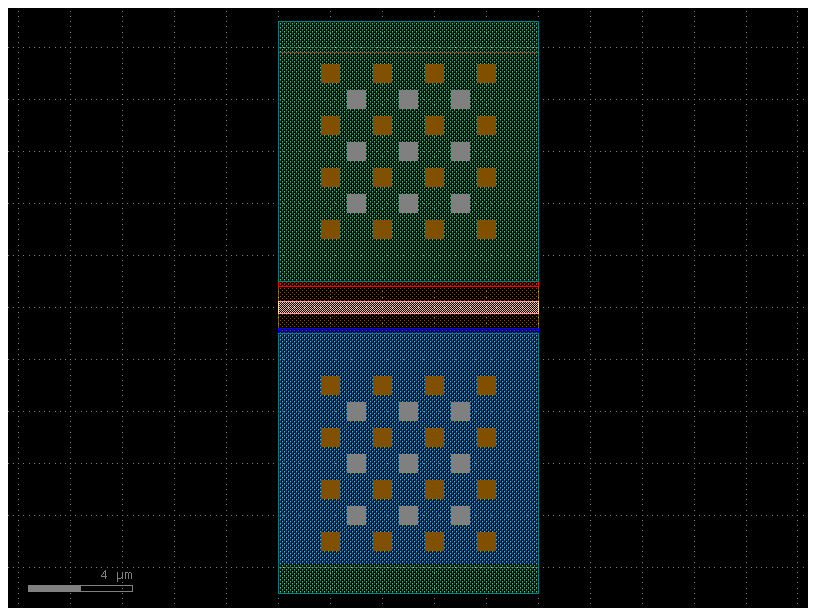
and a LayerStack
. Here, we copy the example from gdsfactory.generic_tech
for clarity).
The info
dict contains miscellaneous information about the layers, including mesh_order
, which determines which layer will appear in the mesh if layers overlap.
We can filter this stack to only focus on some layers:
filtered_layer_stack = LayerStack(
layers={
k: LAYER_STACK.layers[k]
for k in (
"slab90",
"core",
"via_contact",
)
}
)
filename = "mesh"
def mesh_with_physicals(mesh, filename):
mesh_from_file = meshio.read(f"{filename}.msh")
return create_physical_mesh(mesh_from_file, "triangle")
scene = waveguide.to_3d(layer_stack=filtered_layer_stack)
scene.show()
The various processing and meshing functions are located under gplugins.gmsh
and can be called from there, but a shortcut is implemented to mesh directly from a component:
mesh = get_mesh(
component=waveguide,
type="xy",
z=0.09,
layer_stack=filtered_layer_stack,
filename="mesh.msh",
)
Info : [ 0%] Union
Info : [ 10%] Union - Performing intersection of shapes
Info : [ 80%] Union - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference - Performing intersection of shapes
Info : [ 80%] Difference - Building splits of containers
Info : [ 0%] Fragments
Info : [ 10%] Fragments - Performing intersection of shapes
Info : [ 80%] Fragments - Building splits of containers
Info : [ 0%] Difference
Info : [ 10%] Difference
Info : [ 20%] Difference
Info : [ 30%] Difference
Info : [ 40%] Difference
Info : [ 50%] Difference
Info : [ 60%] Difference
Info : [ 70%] Difference
Info : [ 80%] Difference - Making faces
Info : [ 90%] Difference - Adding holes
Info : [ 0%] Fragments
Info : [ 10%] Fragments
Info : [ 20%] Fragments
Info : [ 30%] Fragments
Info : [ 40%] Fragments
Info : [ 50%] Fragments
Info : [ 60%] Fragments
Info : [ 70%] Fragments - Building splits of containers
Info : [ 80%] Fragments - Splitting faces
Info : Meshing 1D...
Info : [ 0%] Meshing curve 1 (Line)
Info : [ 0%] Meshing curve 3 (Line)
Info : [ 0%] Meshing curve 2 (Line)
Info : [ 0%] Meshing curve 4 (Line)
Info : [ 0%] Meshing curve 5 (Line)
Info : [ 0%] Meshing curve 6 (Line)
Info : [ 10%] Meshing curve 7 (Line)
Info : [ 10%] Meshing curve 8 (Line)
Info : [ 10%] Meshing curve 9 (Line)
Info : [ 10%] Meshing curve 10 (Line)
Info : [ 10%] Meshing curve 11 (Line)
Info : [ 10%] Meshing curve 12 (Line)
Info : [ 10%] Meshing curve 13 (Line)
Info : [ 10%] Meshing curve 14 (Line)
Info : [ 10%] Meshing curve 15 (Line)
Info : [ 10%] Meshing curve 16 (Line)
Info : [ 10%] Meshing curve 17 (Line)
Info : [ 10%] Meshing curve 18 (Line)
Info : [ 10%] Meshing curve 19 (Line)
Info : [ 20%] Meshing curve 20 (Line)
Info : [ 20%] Meshing curve 21 (Line)
Info : [ 20%] Meshing curve 22 (Line)
Info : [ 20%] Meshing curve 23 (Line)
Info : [ 20%] Meshing curve 24 (Line)
Info : [ 20%] Meshing curve 25 (Line)
Info : [ 20%] Meshing curve 26 (Line)
Info : [ 20%] Meshing curve 27 (Line)
Info : [ 20%] Meshing curve 28 (Line)
Info : [ 20%] Meshing curve 29 (Line)
Info : [ 20%] Meshing curve 30 (Line)
Info : [ 20%] Meshing curve 31 (Line)
Info : [ 20%] Meshing curve 32 (Line)
Info : [ 20%] Meshing curve 33 (Line)
Info : [ 30%] Meshing curve 34 (Line)
Info : [ 30%] Meshing curve 35 (Line)
Info : [ 30%] Meshing curve 36 (Line)
Info : [ 30%] Meshing curve 37 (Line)
Info : [ 30%] Meshing curve 38 (Line)
Info : [ 30%] Meshing curve 39 (Line)
Info : [ 30%] Meshing curve 40 (Line)
Info : [ 30%] Meshing curve 41 (Line)
Info : [ 30%] Meshing curve 42 (Line)
Info : [ 30%] Meshing curve 43 (Line)
Info : [ 30%] Meshing curve 44 (Line)
Info : [ 30%] Meshing curve 45 (Line)
Info : [ 30%] Meshing curve 46 (Line)
Info : [ 40%] Meshing curve 47 (Line)
Info : [ 40%] Meshing curve 48 (Line)
Info : [ 40%] Meshing curve 49 (Line)
Info : [ 40%] Meshing curve 50 (Line)
Info : [ 40%] Meshing curve 51 (Line)
Info : [ 40%] Meshing curve 52 (Line)
Info : [ 40%] Meshing curve 53 (Line)
Info : [ 40%] Meshing curve 54 (Line)
Info : [ 40%] Meshing curve 55 (Line)
Info : [ 40%] Meshing curve 56 (Line)
Info : [ 40%] Meshing curve 57 (Line)
Info : [ 40%] Meshing curve 58 (Line)
Info : [ 40%] Meshing curve 59 (Line)
Info : [ 40%] Meshing curve 60 (Line)
Info : [ 50%] Meshing curve 61 (Line)
Info : [ 50%] Meshing curve 62 (Line)
Info : [ 50%] Meshing curve 63 (Line)
Info : [ 50%] Meshing curve 64 (Line)
Info : [ 50%] Meshing curve 65 (Line)
Info : [ 50%] Meshing curve 66 (Line)
Info : [ 50%] Meshing curve 67 (Line)
Info : [ 50%] Meshing curve 68 (Line)
Info : [ 50%] Meshing curve 69 (Line)
Info : [ 50%] Meshing curve 70 (Line)
Info : [ 50%] Meshing curve 71 (Line)
Info : [ 50%] Meshing curve 72 (Line)
Info : [ 50%] Meshing curve 73 (Line)
Info : [ 60%] Meshing curve 74 (Line)
Info : [ 60%] Meshing curve 75 (Line)
Info : [ 60%] Meshing curve 76 (Line)
Info : [ 60%] Meshing curve 78 (Line)
Info : [ 60%] Meshing curve 79 (Line)
Info : [ 60%] Meshing curve 80 (Line)
Info : [ 60%] Meshing curve 81 (Line)
Info : [ 60%] Meshing curve 82 (Line)
Info : [ 60%] Meshing curve 83 (Line)
Info : [ 60%] Meshing curve 84 (Line)
Info : [ 60%] Meshing curve 85 (Line)
Info : [ 60%] Meshing curve 86 (Line)
Info : [ 60%] Meshing curve 87 (Line)
Info : [ 60%] Meshing curve 88 (Line)
Info : [ 70%] Meshing curve 89 (Line)
Info : [ 70%] Meshing curve 90 (Line)
Info : [ 70%] Meshing curve 91 (Line)
Info : [ 70%] Meshing curve 92 (Line)
Info : [ 70%] Meshing curve 93 (Line)
Info : [ 70%] Meshing curve 94 (Line)
Info : [ 70%] Meshing curve 95 (Line)
Info : [ 70%] Meshing curve 96 (Line)
Info : [ 70%] Meshing curve 97 (Line)
Info : [ 60%] Meshing curve 77 (Line)
Info : [ 70%] Meshing curve 98 (Line)
Info : [ 70%] Meshing curve 99 (Line)
Info : [ 70%] Meshing curve 100 (Line)
Info : [ 70%] Meshing curve 101 (Line)
Info : [ 80%] Meshing curve 102 (Line)
Info : [ 80%] Meshing curve 103 (Line)
Info : [ 80%] Meshing curve 104 (Line)
Info : [ 80%] Meshing curve 105 (Line)
Info : [ 80%] Meshing curve 106 (Line)
Info : [ 80%] Meshing curve 107 (Line)
Info : [ 80%] Meshing curve 108 (Line)
Info : [ 80%] Meshing curve 109 (Line)
Info : [ 80%] Meshing curve 110 (Line)
Info : [ 80%] Meshing curve 112 (Line)
Info : [ 80%] Meshing curve 111 (Line)
Info : [ 80%] Meshing curve 113 (Line)
Info : [ 80%] Meshing curve 114 (Line)
Info : [ 80%] Meshing curve 116 (Line)
Info : [ 80%] Meshing curve 117 (Line)
Info : [ 80%] Meshing curve 118 (Line)
Info : [ 80%] Meshing curve 119 (Line)
Info : [ 80%] Meshing curve 115 (Line)
Info : [ 80%] Meshing curve 120 (Line)
Info : [ 80%] Meshing curve 121 (Line)
Info : [ 80%] Meshing curve 122 (Line)
Info : [ 80%] Meshing curve 123 (Line)
Info : [ 80%] Meshing curve 124 (Line)
Info : [ 80%] Meshing curve 125 (Line)
Info : [ 80%] Meshing curve 126 (Line)
Info : [ 80%] Meshing curve 127 (Line)
Info : [ 80%] Meshing curve 129 (Line)
Info : [100%] Meshing curve 130 (Line)
Info : [100%] Meshing curve 131 (Line)
Info : [ 80%] Meshing curve 128 (Line)
Info : [100%] Meshing curve 132 (Line)
Info : [100%] Meshing curve 133 (Line)
Info : [100%] Meshing curve 134 (Line)
Info : [100%] Meshing curve 135 (Line)
Info : [100%] Meshing curve 136 (Line)
Info : [100%] Meshing curve 137 (Line)
Info : [100%] Meshing curve 138 (Line)
Info : Done meshing 1D (Wall 0.0210923s, CPU 0.05686s)
Info : Meshing 2D...
Info : [ 0%] Meshing surface 1 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 4 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 3 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 2 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 6 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 7 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 5 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 9 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 10 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 8 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 11 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 12 (Plane, Frontal-Delaunay)
Info : [ 0%] Meshing surface 13 (Plane, Frontal-Delaunay)
Info : [ 30%] Meshing surface 14 (Plane, Frontal-Delaunay)
Info : [ 30%] Meshing surface 15 (Plane, Frontal-Delaunay)
Info : [ 30%] Meshing surface 16 (Plane, Frontal-Delaunay)
Info : [ 40%] Meshing surface 17 (Plane, Frontal-Delaunay)
Info : [ 40%] Meshing surface 18 (Plane, Frontal-Delaunay)
Info : [ 40%] Meshing surface 19 (Plane, Frontal-Delaunay)
Info : [ 50%] Meshing surface 20 (Plane, Frontal-Delaunay)
Info : [ 50%] Meshing surface 21 (Plane, Frontal-Delaunay)
Info : [ 50%] Meshing surface 22 (Plane, Frontal-Delaunay)
Info : [ 60%] Meshing surface 23 (Plane, Frontal-Delaunay)
Info : [ 60%] Meshing surface 24 (Plane, Frontal-Delaunay)
Info : [ 60%] Meshing surface 25 (Plane, Frontal-Delaunay)
Info : [ 70%] Meshing surface 26 (Plane, Frontal-Delaunay)
Info : [ 70%] Meshing surface 27 (Plane, Frontal-Delaunay)
Info : [ 70%] Meshing surface 28 (Plane, Frontal-Delaunay)
Info : [ 80%] Meshing surface 29 (Plane, Frontal-Delaunay)
Info : [ 80%] Meshing surface 30 (Plane, Frontal-Delaunay)
Info : [ 80%] Meshing surface 31 (Plane, Frontal-Delaunay)
Info : [ 90%] Meshing surface 32 (Plane, Frontal-Delaunay)
Info : [ 90%] Meshing surface 33 (Plane, Frontal-Delaunay)
Info : [ 90%] Meshing surface 34 (Plane, Frontal-Delaunay)
Info : [ 90%] Meshing surface 35 (Plane, Frontal-Delaunay)
Info : Done meshing 2D (Wall 0.0341906s, CPU 0.092455s)
Info : 2004 nodes 4438 elements
Info : Writing 'mesh.msh'...
Info : Done writing 'mesh.msh'
Info : Writing '/tmp/tmpi7_eodee/mesh.msh'...
Info : Done writing '/tmp/tmpi7_eodee/mesh.msh'
This returns a gmsh .msh
mesh, also saved in filename
if provided, which can be processed:
mesh.get_cells_type("triangle")
mesh = from_meshio(mesh)
mesh.draw().plot()
[]
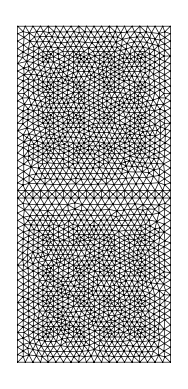
The gmsh
GUI can be used to load and inspect the .msh
file:
meshio can also be used to convert the .msh
to another arbitrary format, to observe for instance with Paraview
. This is useful, for instance to preprocess the msh
file using the create_mesh
utility in order to consolidate entities with the same label:
mesh_from_file = meshio.read("mesh.msh")
triangle_mesh = create_physical_mesh(mesh_from_file, "triangle")
meshio.write("mesh.xdmf", triangle_mesh)
mesh = mesh_with_physicals(triangle_mesh, filename)
mesh = from_meshio(mesh)
mesh.draw().plot()
[]
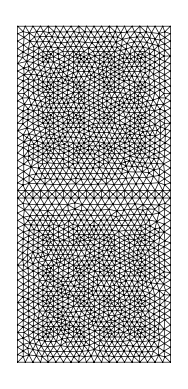
You can opening the mesh.xdmf
in paraview:
line_mesh = create_physical_mesh(mesh_from_file, "line")
meshio.write("facet_mesh.xdmf", line_mesh)
mesh = mesh_with_physicals(line_mesh, filename)
mesh = from_meshio(mesh)
mesh.draw().plot()
[]
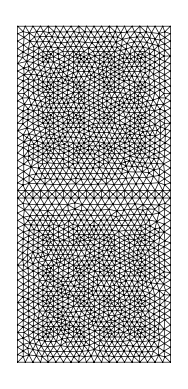
Opening the facet_mesh.xdmf
in paraview:
The xdmf
files with consolidated physical groups can also be opened dynamically in a notebook with pyvista