VLSIR#
Thanks to VLSIR you can parse for KLayout’s gds-extracted netlist’s and convert it to Spectre, Spice and Xyce simulator schematic file formats
Simulator and Analysis Support#
Each spice-class simulator includes its own netlist syntax and opinions about the specification for analyses. See
Analysis |
Spectre |
Xyce |
NgSpice |
---|---|---|---|
Op |
✅ |
✅ |
✅ |
Dc |
✅ |
✅ |
|
Tran |
✅ |
✅ |
✅ |
Ac |
✅ |
✅ |
✅ |
Noise |
✅ |
||
Sweep |
|||
Monte Carlo |
|||
Custom |
from io import StringIO
from gdsfactory.samples.demo.lvs import pads_correct
import gplugins.vlsir as gs
from gplugins.klayout.get_netlist import get_netlist
c = pads_correct()
c.plot()
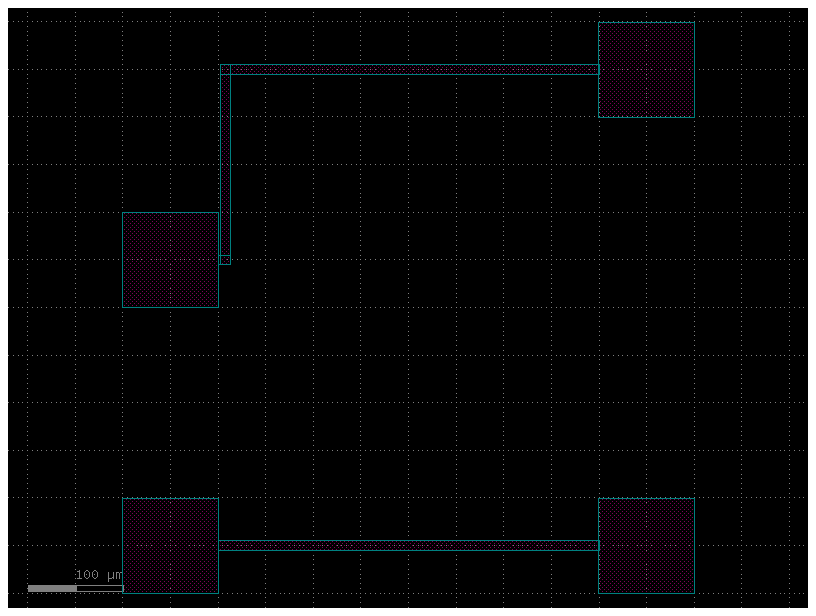
gdspath = c.write_gds()
# get the netlist
kdbnetlist = get_netlist(gdspath)
# convert it to a VLSIR Package
pkg = gs.kdb_vlsir(kdbnetlist, domain="gplugins.klayout.example")
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/layers.lyp'
/home/runner/work/gplugins/gplugins/gplugins/klayout/d25/generic.lyd25
Wrote '/home/runner/work/gplugins/gplugins/gplugins/klayout/tech.lyt'
Spectre RF#
out = StringIO()
gs.export_netlist(pkg, dest=out, fmt="spectre")
print(out.getvalue())
// `circuit.Package` `gplugins.klayout.example`
// Generated by `vlsirtools.SpectreNetlister`
//
subckt straight_L387_N2_CSmetal3_W10
+ 1
+ // No parameters
ends
subckt straight_L190_N2_CSmetal3_W10
+ 1
+ // No parameters
ends
subckt straight_L3_N2_CSmetal3_W10
+ 1
+ // No parameters
ends
subckt wire_corner_CSmetal3_RNone
+ 1
+ // No parameters
ends
subckt straight_L400_N2_CSmetal3_W10
+ 1
+ // No parameters
ends
subckt pad_S100_100_LMTOP_BLNo_8d51f10c
+ 1 2
+ // No parameters
ends
subckt pads_correct_Ppad_CSmetal3
+ // No ports
+ // No parameters
1
+ // Ports:
+ ( tl,tr tl )
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ // No parameters
2
+ // Ports:
+ ( tl,tr tr )
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ // No parameters
3
+ // Ports:
+ ( bl,br br )
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ // No parameters
4
+ // Ports:
+ ( bl,br bl )
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ // No parameters
5
+ // Ports:
+ ( tl,tr )
+ straight_L3_N2_CSmetal3_W10
+ // No parameters
6
+ // Ports:
+ ( tl,tr )
+ wire_corner_CSmetal3_RNone
+ // No parameters
7
+ // Ports:
+ ( tl,tr )
+ straight_L190_N2_CSmetal3_W10
+ // No parameters
8
+ // Ports:
+ ( tl,tr )
+ wire_corner_CSmetal3_RNone
+ // No parameters
9
+ // Ports:
+ ( tl,tr )
+ straight_L387_N2_CSmetal3_W10
+ // No parameters
10
+ // Ports:
+ ( bl,br )
+ straight_L400_N2_CSmetal3_W10
+ // No parameters
ends
Xyce#
out = StringIO()
gs.export_netlist(pkg, dest=out, fmt="xyce")
print(out.getvalue())
; `circuit.Package` `gplugins.klayout.example`
; Generated by `vlsirtools.XyceNetlister`
;
.SUBCKT straight_L387_N2_CSmetal3_W10
+ 1
; No parameters
.ENDS
.SUBCKT straight_L190_N2_CSmetal3_W10
+ 1
; No parameters
.ENDS
.SUBCKT straight_L3_N2_CSmetal3_W10
+ 1
; No parameters
.ENDS
.SUBCKT wire_corner_CSmetal3_RNone
+ 1
; No parameters
.ENDS
.SUBCKT straight_L400_N2_CSmetal3_W10
+ 1
; No parameters
.ENDS
.SUBCKT pad_S100_100_LMTOP_BLNo_8d51f10c
+ 1 2
; No parameters
.ENDS
.SUBCKT pads_correct_Ppad_CSmetal3
; No ports
; No parameters
x1
+ tl,tr tl
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ ; No parameters
x2
+ tl,tr tr
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ ; No parameters
x3
+ bl,br br
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ ; No parameters
x4
+ bl,br bl
+ pad_S100_100_LMTOP_BLNo_8d51f10c
+ ; No parameters
x5
+ tl,tr
+ straight_L3_N2_CSmetal3_W10
+ ; No parameters
x6
+ tl,tr
+ wire_corner_CSmetal3_RNone
+ ; No parameters
x7
+ tl,tr
+ straight_L190_N2_CSmetal3_W10
+ ; No parameters
x8
+ tl,tr
+ wire_corner_CSmetal3_RNone
+ ; No parameters
x9
+ tl,tr
+ straight_L387_N2_CSmetal3_W10
+ ; No parameters
x10
+ bl,br
+ straight_L400_N2_CSmetal3_W10
+ ; No parameters
.ENDS
ngspice#
out = StringIO()
gs.export_netlist(pkg, dest=out, fmt="spice")
print(out.getvalue())
* `circuit.Package` `gplugins.klayout.example`
* Generated by `vlsirtools.SpiceNetlister`
*
.SUBCKT straight_L387_N2_CSmetal3_W10
+ 1
* No parameters
.ENDS
.SUBCKT straight_L190_N2_CSmetal3_W10
+ 1
* No parameters
.ENDS
.SUBCKT straight_L3_N2_CSmetal3_W10
+ 1
* No parameters
.ENDS
.SUBCKT wire_corner_CSmetal3_RNone
+ 1
* No parameters
.ENDS
.SUBCKT straight_L400_N2_CSmetal3_W10
+ 1
* No parameters
.ENDS
.SUBCKT pad_S100_100_LMTOP_BLNo_8d51f10c
+ 1 2
* No parameters
.ENDS
.SUBCKT pads_correct_Ppad_CSmetal3
* No ports
* No parameters
x1
+ tl,tr tl
+ pad_S100_100_LMTOP_BLNo_8d51f10c
* No parameters
x2
+ tl,tr tr
+ pad_S100_100_LMTOP_BLNo_8d51f10c
* No parameters
x3
+ bl,br br
+ pad_S100_100_LMTOP_BLNo_8d51f10c
* No parameters
x4
+ bl,br bl
+ pad_S100_100_LMTOP_BLNo_8d51f10c
* No parameters
x5
+ tl,tr
+ straight_L3_N2_CSmetal3_W10
* No parameters
x6
+ tl,tr
+ wire_corner_CSmetal3_RNone
* No parameters
x7
+ tl,tr
+ straight_L190_N2_CSmetal3_W10
* No parameters
x8
+ tl,tr
+ wire_corner_CSmetal3_RNone
* No parameters
x9
+ tl,tr
+ straight_L387_N2_CSmetal3_W10
* No parameters
x10
+ bl,br
+ straight_L400_N2_CSmetal3_W10
* No parameters
.ENDS